A Comprehensive Guide to Framer Motion: Powerful Animations for React
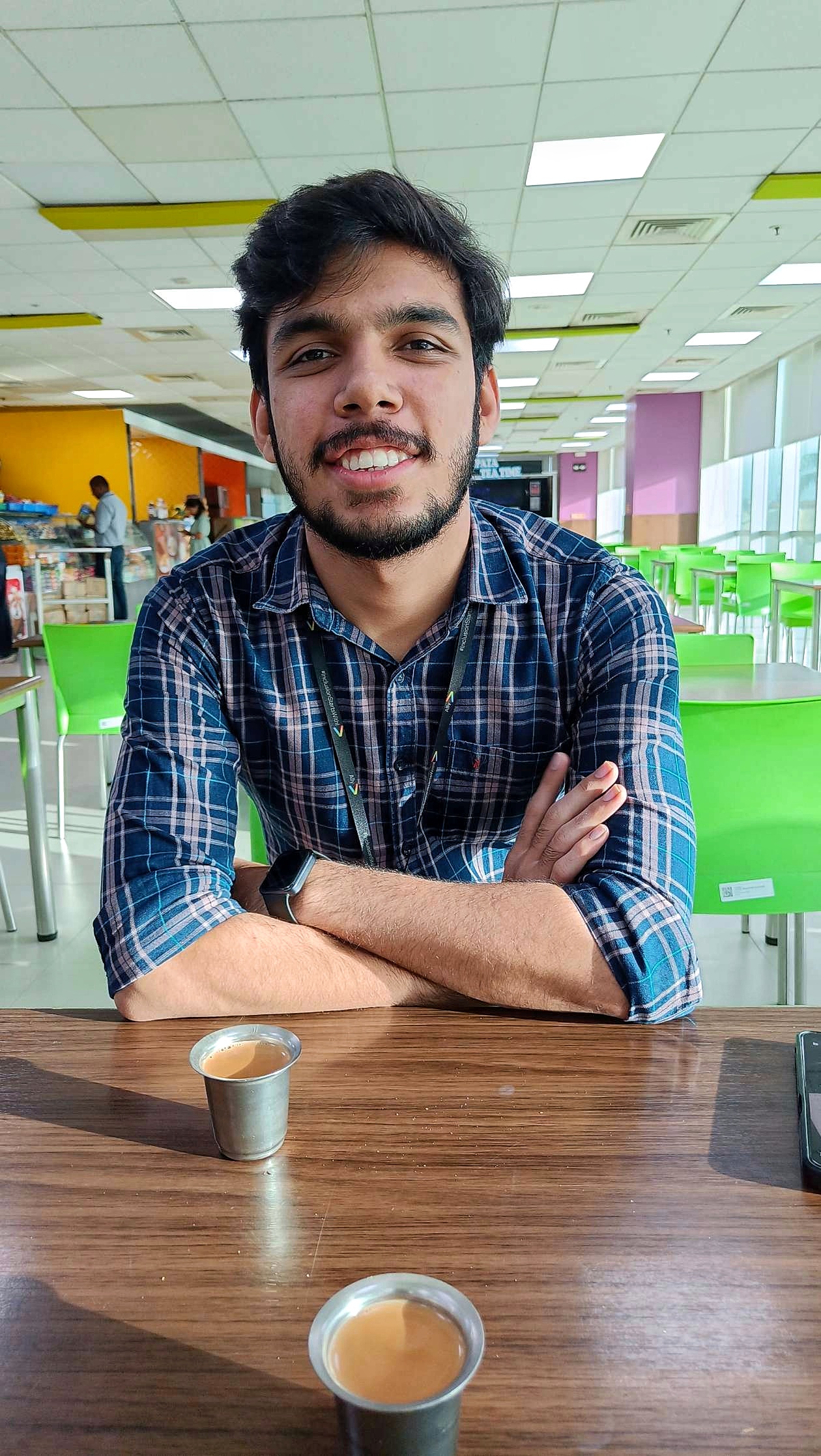
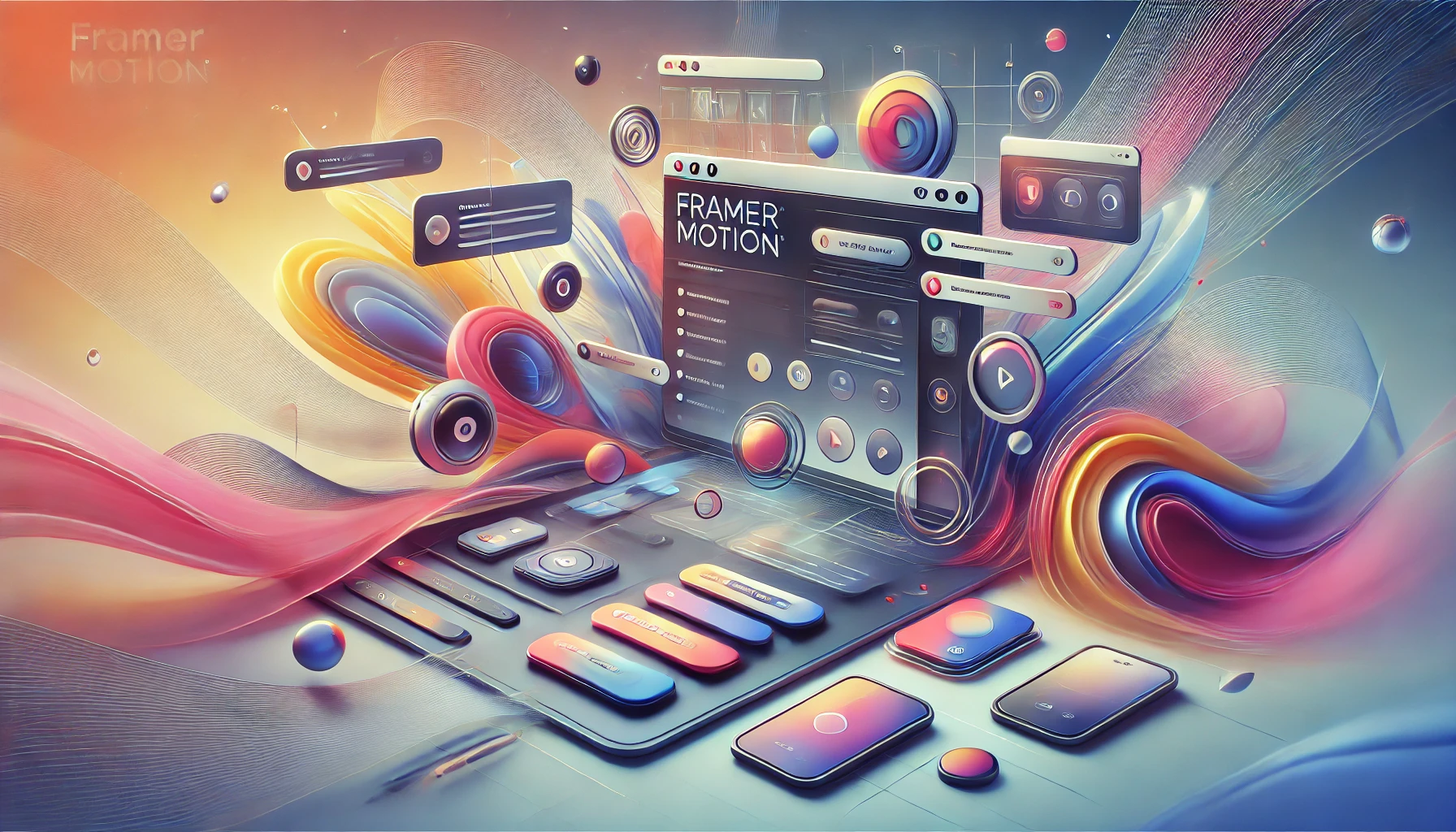
In modern web development, user interfaces are all about engagement and interactivity. Animations play a key role in creating visually appealing and intuitive experiences, enhancing both usability and user satisfaction. Framer Motion is a leading animation library for React that makes crafting such experiences not only simple but also powerful. In this blog, we’ll dive deep into what Framer Motion is, why it’s so popular, and how you can use it to animate your React components.
What is Framer Motion?
Framer Motion is a popular and powerful library for creating smooth animations in React applications. It provides developers with an easy-to-use API to implement complex animations while maintaining excellent performance. Framer Motion is based on Framer, a design tool, but is specifically optimized for React applications. Whether you're looking to add simple transitions or build highly interactive UI elements, Framer Motion is a great choice.
Why Use Framer Motion?
Declarative Animations: Instead of manually manipulating CSS or DOM elements, you define your animations directly within your React components using props. This makes the code more readable and easier to manage.
Smooth and Performant: Framer Motion leverages requestAnimationFrame under the hood, ensuring animations are smooth and optimized for performance.
Highly Customizable: You can define animation duration, easing functions, keyframes, spring physics, and much more. It offers granular control over how your elements animate.
Gestures Support: Framer Motion also provides support for interactive animations like dragging, hovering, and tapping, making it easy to create engaging user experiences.
Layout Animations: Framer Motion supports layout animations that help automatically animate the transition between layout changes, making it incredibly powerful for dynamic UI elements.
Getting Started with Framer Motion
Installation
To get started with Framer Motion, you need to install it in your React project:
npm install framer-motion
Or with yarn:
yarn add framer-motion
Basic Usage Example: Animating an Element
A basic example of animating an element with Framer Motion involves using the motion component.
import { motion } from "framer-motion";
function App() {
return (
<motion.div
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
transition={{ duration: 1 }}
>
<h1>Hello, Framer Motion!</h1>
</motion.div>
);
}
export default App;
Explanation:
initial: Defines the starting state of the animation. Here, we set the initial opacity to 0 (completely invisible).
animate: Defines the final state of the animation, which in this case is an opacity of 1 (fully visible).
transition: Specifies the timing for the animation, including duration and easing.
In this example, the text fades in over 1 second as the page loads.
Animating Multiple Elements
Framer Motion simplifies the creation of animations that affect multiple elements sequentially. For instance, you can create a staggered list animation where each item appears one after the other
import { motion } from "framer-motion";
function StaggeredList() {
const list = {
hidden: { opacity: 0 },
visible: {
opacity: 1,
transition: {
when: "beforeChildren",
staggerChildren: 0.3,
},
},
};
const listItem = {
hidden: { opacity: 0 },
visible: { opacity: 1 },
};
return (
<motion.ul variants={list} initial="hidden" animate="visible">
<motion.li variants={listItem}>Item 1</motion.li>
<motion.li variants={listItem}>Item 2</motion.li>
<motion.li variants={listItem}>Item 3</motion.li>
</motion.ul>
);
}
export default StaggeredList;
Explanation:
Variants: A powerful feature that allows you to define reusable sets of animations. In the example above, both the list and list items have their own variants.
staggerChildren: Allows you to stagger the animations of child elements, creating a delay between them.
The list fades in, and then each list item fades in with a slight delay between them.
Gesture-Based Animations: Drag, Tap, Hover
One of Framer Motion's standout features is its support for gestures like dragging, tapping, and hovering. Here's an example that makes a box draggable:
Drag Example
import { motion } from "framer-motion";
function DraggableBox() {
return (
<motion.div
drag
style={{
width: 100,
height: 100,
backgroundColor: "tomato",
display: "flex",
justifyContent: "center",
alignItems: "center",
borderRadius: 20,
}}
>
Drag Me
</motion.div>
);
}
export default DraggableBox;
Explanation:
drag: When this prop is added to a motion.div, it enables dragging of that element.
The box in the example is now draggable, and Framer Motion takes care of the drag logic (such as boundaries, physics, etc.) for you.
Tap and Hover Animations:
import { motion } from "framer-motion";
function InteractiveButton() {
return (
<motion.button
whileHover={{ scale: 1.2 }}
whileTap={{ scale: 0.9 }}
style={{
padding: "10px 20px",
fontSize: "16px",
borderRadius: "5px",
border: "none",
backgroundColor: "royalblue",
color: "white",
}}
>
Click Me
</motion.button>
);
}
export default InteractiveButton;
Explanation:
whileHover: Defines an animation that plays while the element is being hovered. In this case, the button will scale up when hovered.
whileTap: Defines an animation that plays when the element is tapped or clicked. Here, the button scales down when clicked.
Key Features of Framer Motion
Variants for Reusability
Variants allow you to define different animation states for your components, making it easier to manage complex animations across multiple elements. Variants allow you to create reusable animation definitions that are extremely useful when you want to keep your code clean and maintainable.
const boxVariants = {
hidden: { opacity: 0, scale: 0.5 },
visible: { opacity: 1, scale: 1 },
};
<motion.div variants={boxVariants} initial="hidden" animate="visible" />;
Animating Between Layout Changes (Layout Animations)
Framer Motion makes it simple to animate elements when they change their size or position in the layout, such as when an element expands or shrinks based on user interaction. This allows for smooth transitions when elements are added, removed, or resized, without manually defining the transitions.
import { useState } from "react";
import { motion } from "framer-motion";
function ExpandableCard() {
const [isOpen, setIsOpen] = useState(false);
return (
<motion.div
layout
onClick={() => setIsOpen(!isOpen)}
style={{
backgroundColor: "lightblue",
padding: "20px",
borderRadius: "10px",
cursor: "pointer",
}}
>
<motion.h2 layout>{isOpen ? "Click to Close" : "Click to Expand"}</motion.h2>
{isOpen && (
<motion.p layout>
This is some additional content that shows up when the card is expanded.
</motion.p>
)}
</motion.div>
);
}
export default ExpandableCard;
layout: Automatically animates the layout changes as the component resizes or changes position.
In this example, when the card is clicked, it expands to show additional content, and Framer Motion animates the transition smoothly.
Exit Animations
You can define how an element should animate out of the DOM by using the exit prop.
<motion.div
initial={{ opacity: 0 }}
animate={{ opacity: 1 }}
exit={{ opacity: 0 }}
/>
This makes it easy to handle entering and exiting elements with seamless animations.
Spring Animations
One of the best features of Framer Motion is the ability to create spring animations that simulate real-world physics. By default, Framer Motion uses spring animations for smooth and natural movement.jsx
<motion.div
animate={{ x: 100 }}
transition={{ type: "spring", stiffness: 300 }}
/>
type: "spring": Specifies a spring animation.
stiffness: Determines how stiff the spring is. Higher values make the spring animation snap faster, while lower values make it feel more bouncy.
Conclusion
Framer Motion is a game-changer when it comes to animating React applications. Its declarative API makes it easy to build sophisticated animations while maintaining clean and readable code. From simple transitions to complex gesture-driven interactions, Framer Motion handles it all with exceptional performance.
Whether you’re building a polished web app, a mobile-friendly interface, or a visually stunning landing page, incorporating animations through Framer Motion will take your React projects to the next level.
Subscribe to my newsletter
Read articles from Abhishek Sadhwani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
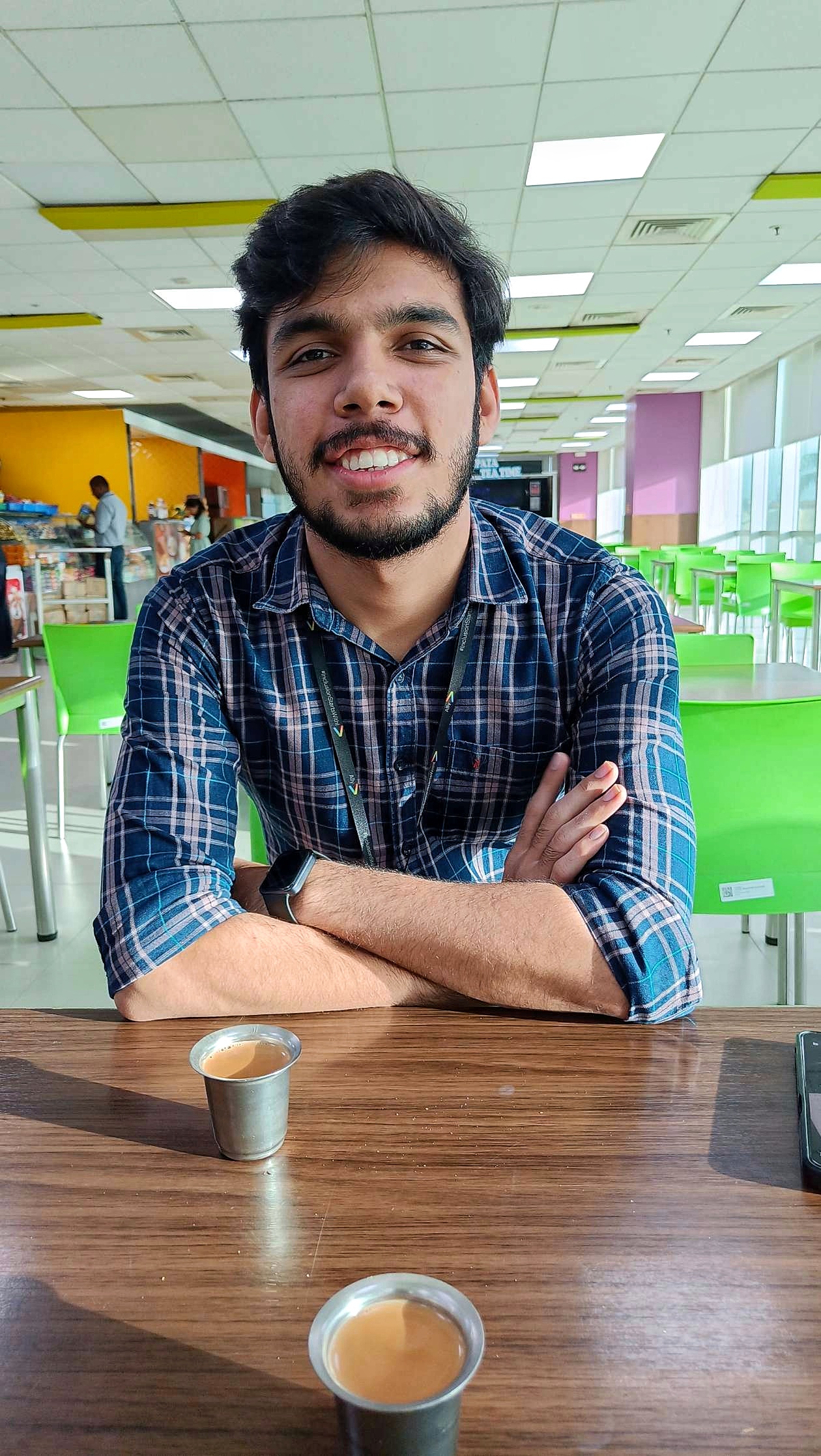