JSON Web Token (JWT) Authentication with Django Rest Framework - #DRF06

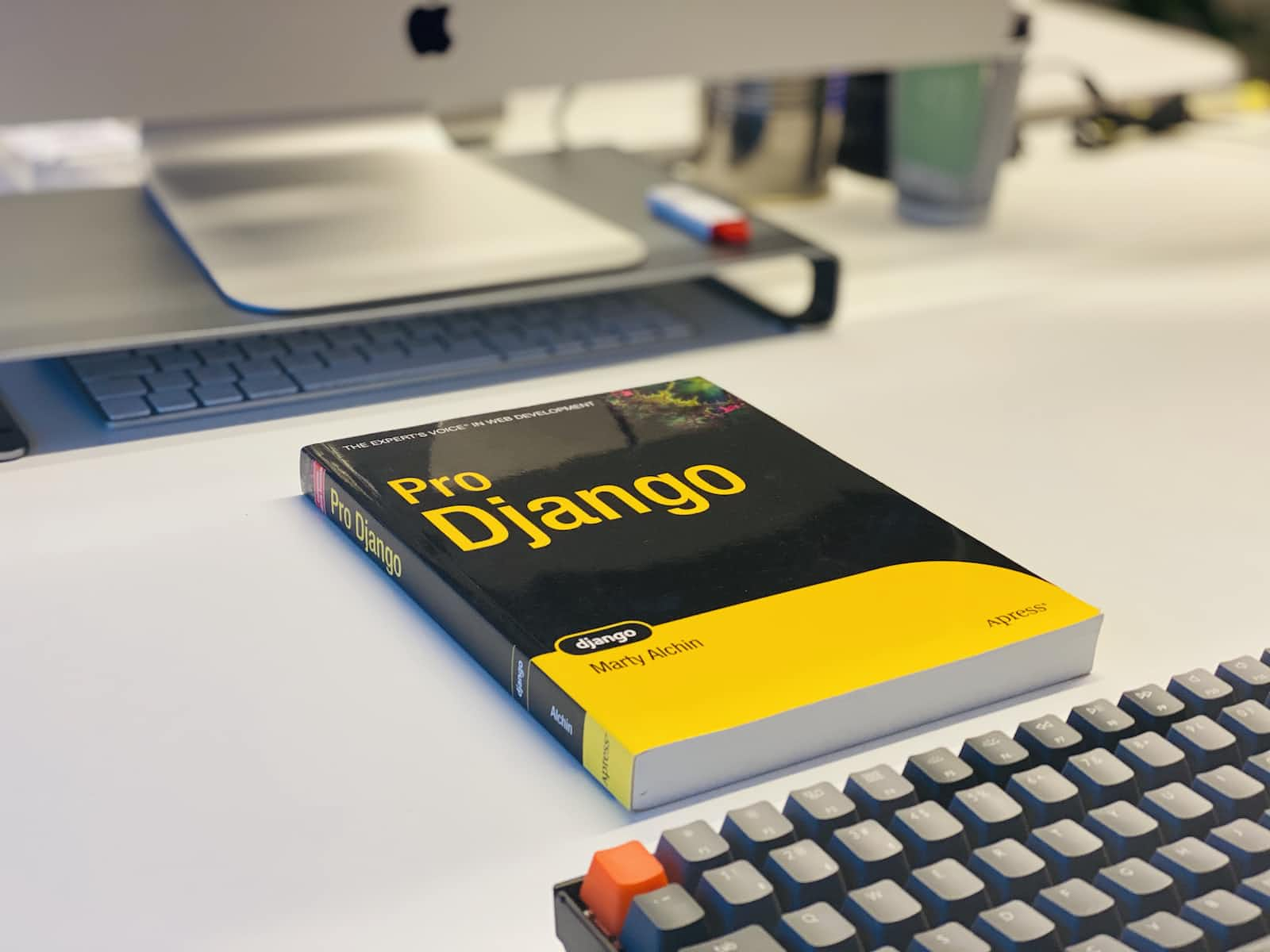
Welcome to another article in our Django Rest Framework series. In this article, we will be discussing how to use JSON Web Tokens (JWT) to authenticate users in Django Rest Framework.
Introduction to JSON Web Tokens
JSON Web Tokens (JWTs) are a compact, URL-safe means of representing claims to be transferred between two parties. JWTs are often used for authentication and authorization purposes. A JWT consists of three parts: the header, the payload, and the signature.
Header
The header typically consists of two parts: the type of the token, which is JWT, and the signing algorithm being used, such as HMAC SHA256 or RSA.
Payload
The payload contains the claims, which are statements about an entity (typically, the user) and additional data. There are three types of claims: registered, public, and private claims.
Signature
The signature is used to verify that the sender of the JWT is who it says it is and to ensure that the message wasn't changed along the way.
Installing Django Rest Framework JWT
To use JWT authentication with Django Rest Framework, we need to install the djangorestframework-jwt
package. We can install it using pip:
pip install djangorestframework-jwt
Configuring JWT Authentication in Django Rest Framework
To enable JWT authentication, we need to add the following settings to our Django settings file:
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': (
'rest_framework_jwt.authentication.JSONWebTokenAuthentication',
),
}
This tells Django Rest Framework to use JWT authentication as the default authentication class.
Generating JWT Tokens
To generate a JWT token, we need to make a POST request to the /api-token-auth/
endpoint with the user's username and password. The response will contain the JWT token:
POST /api-token-auth/ HTTP/1.1
Host: example.com
Content-Type: application/json
{
"username": "johndoe",
"password": "password123"
}
HTTP/1.1 200 OK
Content-Type: application/json
{
"token": "eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyX2lkIjoyLCJ1c2VybmFtZSI6ImpvaG5kb2UiLCJleHAiOjE1NzY4NzYzMDZ9.o92ZptDlSxkKjQ2g61OiW8EnH1XGzNp-DenKKZJwW8c"
}
Authenticating Requests with JWT Tokens
To authenticate a request with a JWT token, we need to include the token in the Authorization header of the request:
GET /api/v1/users/ HTTP/1.1
Host: example.com
Authorization: JWT eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzI1NiJ9.eyJ1c2VyX2lkIjoyLCJ1c2VybmFtZSI6ImpvaG5kb2UiLCJleHAiOjE1NzY4NzYzMDZ9.o92ZptDlSxkKjQ2g61OiW8EnH1XGzNp-DenKKZJwW8c
If the token is valid, Django Rest Framework will authenticate the user and allow the request to proceed.
Subscribe to my newsletter
Read articles from Excited Public directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Excited Public
Excited Public
Generating Contents with AI to make learning easy.