Activity 22: Research SMACSS - Scalable and Modular Architecture for CSS
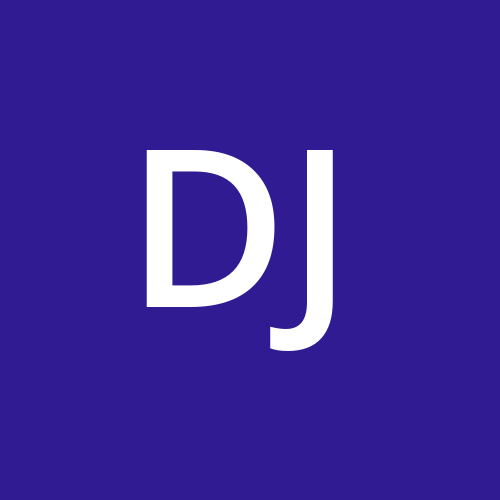
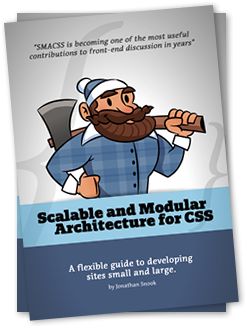
SMACSS (Scalable and Modular Architecture for CSS) is a methodology that provides a structured approach to organizing CSS code, making it more scalable and maintainable, especially in large projects.
1. Base
Base styles set the default appearance for HTML elements. These styles typically affect typography, colors, and other fundamental aspects of the site.
/* Base Styles */
html {
font-size: 16px;
}
body {
font-family: Arial, sans-serif;
color: #333;
line-height: 1.5;
}
h1, h2, h3 {
margin: 0;
padding: 0;
}
a {
color: #007bff;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
2. Layout
Layout styles define the structure and positioning of major sections on a page.
/* Layout Styles */
.header {
background-color: #f8f9fa;
padding: 20px;
}
.main {
display: flex;
flex-direction: row;
}
.sidebar {
width: 25%;
padding: 20px;
}
.content {
width: 75%;
padding: 20px;
}
.footer {
background-color: #f8f9fa;
text-align: center;
padding: 10px;
}
3. Module
Module styles are reusable components that can be utilized across different parts of a website.
/* Module Styles */
.button {
display: inline-block;
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
}
.card {
border: 1px solid #ddd;
border-radius: 5px;
padding: 20px;
margin: 10px;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
4. State
State styles manage the visual representation of components based on their state.
/* State Styles */
.is-active {
background-color: #0056b3;
}
.is-disabled {
background-color: #ccc;
cursor: not-allowed;
opacity: 0.6;
}
.card.is-selected {
border-color: #007bff;
box-shadow: 0 0 10px rgba(0, 123, 255, 0.5);
}
5. Theme
Theme styles allow for variations in the appearance of components without changing their structure.
/* Theme Styles */
.theme-dark {
background-color: #333;
color: #fff;
}
.theme-dark .header,
.theme-dark .footer {
background-color: #444;
}
.theme-light {
background-color: #fff;
color: #000;
}
.theme-light .header,
.theme-light .footer {
background-color: #f8f9fa;
}
Example HTML Structure
Here’s a simple HTML structure that utilizes these styles:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="styles.css">
<title>SMACSS Example</title>
</head>
<body class="theme-light">
<header class="header">
<h1>SMACSS Example</h1>
</header>
<div class="main">
<aside class="sidebar">
<h2>Sidebar</h2>
<button class="button">Click Me</button>
</aside>
<main class="content">
<div class="card is-selected">
<h2>Card Title</h2>
<p>This is a card component.</p>
</div>
<div class="card">
<h2>Card Title</h2>
<p>This is another card component.</p>
</div>
</main>
</div>
<footer class="footer">
<p>© 2024 SMACSS Example</p>
</footer>
</body>
</html>
By organizing CSS into these categories, SMACSS promotes a clearer structure, making it easier to maintain and scale projects as they grow. Each category serves a distinct purpose, allowing developers to manage styles more efficiently.
Sample:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>SMACSS Simple Webpage</title>
<link rel="stylesheet" href="styles.css">
</head>
<body class="theme-light">
<header class="header">
<h1>Welcome to SMACSS</h1>
</header>
<div class="main">
<aside class="sidebar">
<h2>Navigation</h2>
<ul>
<li><a href="#" class="button">Home</a></li>
<li><a href="#" class="button">About</a></li>
<li><a href="#" class="button">Services</a></li>
<li><a href="#" class="button">Contact</a></li>
</ul>
</aside>
<main class="content">
<div class="card">
<h2>What is SMACSS?</h2>
<p>SMACSS stands for Scalable and Modular Architecture for CSS. It is a style guide that helps organize CSS code.</p>
</div>
<div class="card is-selected">
<h2>Benefits of SMACSS</h2>
<p>Improves maintainability, scalability, and encourages reusability of styles.</p>
</div>
</main>
</div>
<footer class="footer">
<p>© 2024 SMACSS Example</p>
</footer>
</body>
</html>
/* Base Styles */
html {
font-size: 16px;
}
body {
font-family: Arial, sans-serif;
color: #333;
line-height: 1.5;
margin: 0;
}
h1, h2 {
margin: 0;
padding: 0;
}
a {
color: #007bff;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
/* Layout Styles */
.header {
background-color: #f8f9fa;
padding: 20px;
text-align: center;
}
.main {
display: flex;
flex-direction: row;
}
.sidebar {
width: 25%;
padding: 20px;
background-color: #f1f1f1;
}
.content {
width: 75%;
padding: 20px;
}
/* Module Styles */
.button {
display: inline-block;
padding: 10px 15px;
background-color: #007bff;
color: white;
border: none;
border-radius: 5px;
cursor: pointer;
margin: 5px 0;
}
.card {
border: 1px solid #ddd;
border-radius: 5px;
padding: 20px;
margin: 10px 0;
box-shadow: 0 2px 5px rgba(0, 0, 0, 0.1);
}
/* State Styles */
.is-selected {
border-color: #007bff;
box-shadow: 0 0 10px rgba(0, 123, 255, 0.5);
}
/* Theme Styles */
.theme-light {
background-color: #fff;
color: #000;
}
.theme-light .header,
.theme-light .footer {
background-color: #f8f9fa;
}
.footer {
text-align: center;
padding: 10px;
background-color: #f8f9fa;
}
Base Styles: Set default styles for typography and links.
Layout Styles: Structure the page with a header, sidebar, main content area, and footer.
Module Styles: Define reusable components like buttons and cards.
State Styles: Highlight selected cards with a specific border and shadow.
Theme Styles: Apply light theme styles for background and text colors.
Output:
Repository:
Subscribe to my newsletter
Read articles from Danilo Buenafe Jr directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
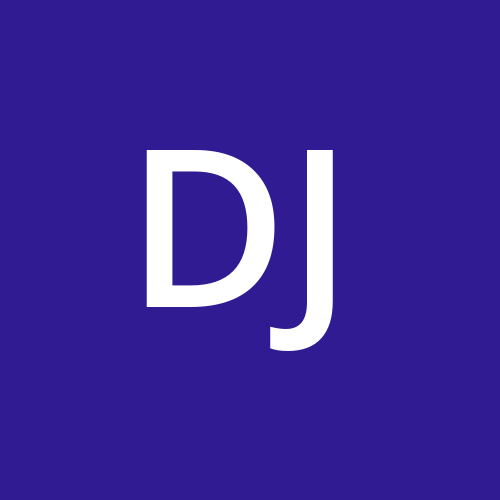