Security Best Practices in PHP Laravel

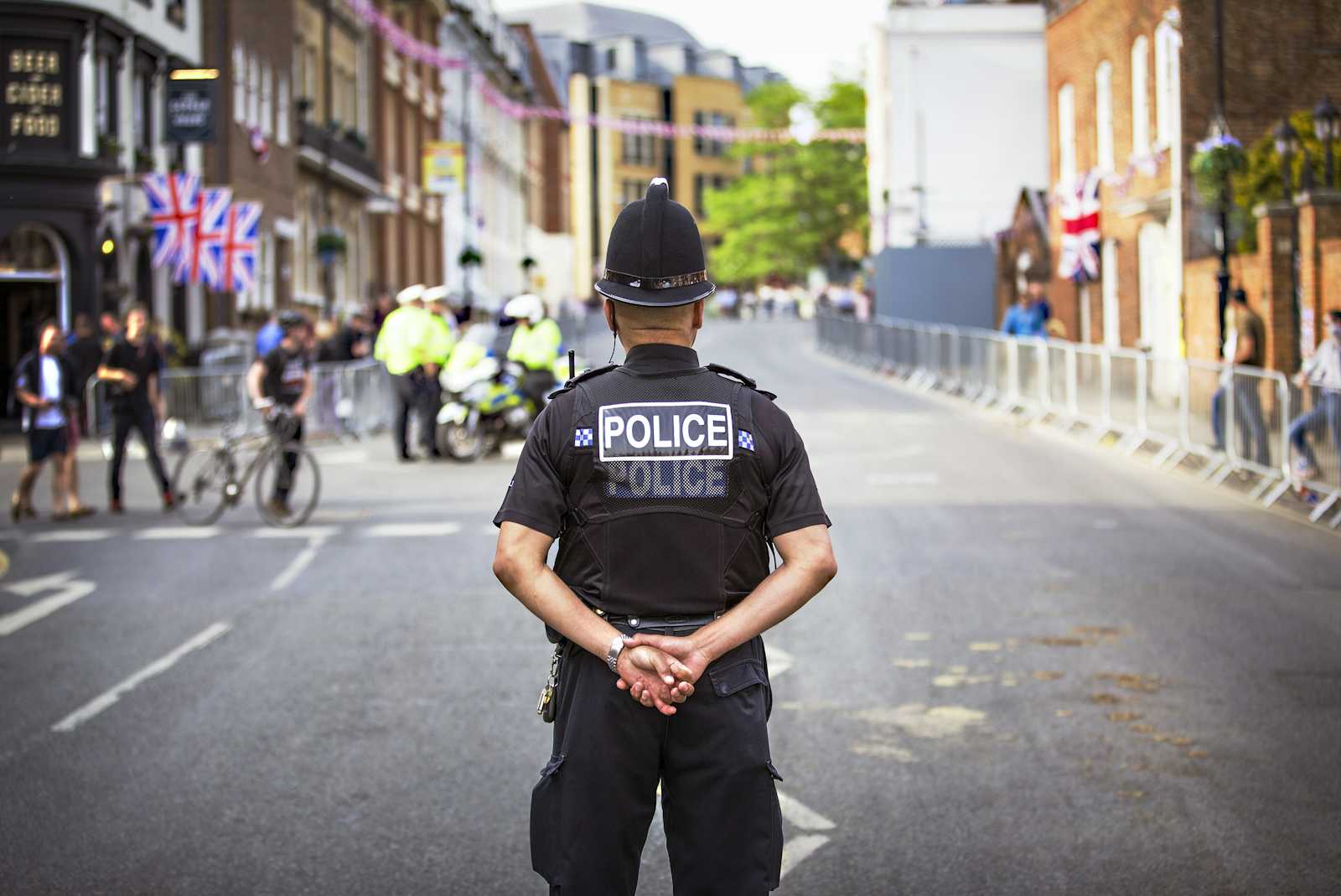
OWASP Top Ten
The OWASP Top Ten is a list of the most common and critical web application security risks. Here's a breakdown of each risk with real-life examples and mitigations in PHP/Laravel.
1. Broken Access Control
Occurs when users can access resources or functionalities that they should not be allowed to.
Example: A user without admin privileges can access an admin page by manipulating URLs (e.g.,
/admin/users
).Mitigation in Laravel: Use middleware for access control (e.g., restricting routes based on roles).
// In routes/web.php Route::middleware('can:isAdmin')->group(function () { Route::get('/admin/users', [AdminController::class, 'index']); }); // Middleware: Verify user is an admin public function handle($request, Closure $next) { if (auth()->user()->role !== 'admin') { return redirect('/home'); } return $next($request); }
2. Cryptographic Failures
Refers to improper implementation of encryption, which can lead to exposure of sensitive data.
Example: Storing passwords in plaintext in the database.
Mitigation in Laravel: Always hash sensitive data (like passwords) before storing it.
// Secure password hashing $hashedPassword = Hash::make($password);
3. Injection
Occurs when untrusted data is sent to an interpreter as part of a query or command, leading to unintended actions.
SQL Injection Example: A malicious user sends
"' OR 1=1; --"
in a form field, causing the database to return all records.Mitigation in Laravel: Use Eloquent ORM or Laravel's Query Builder to automatically prevent SQL injection by binding parameters.
// Safe query using Eloquent ORM $users = User::where('email', $request->email)->get();
4. Insecure Design
Refers to design flaws in applications that result in insecure behavior.
Example: Not validating user input before executing critical actions like payments or purchases.
Mitigation in Laravel: Validate user inputs and business logic through form requests or validation rules.
// Using Laravel validation $request->validate([ 'amount' => 'required|numeric|min:1', ]);
5. Security Misconfiguration
Occurs when the application is improperly configured, leaving it vulnerable to attacks.
Example: Exposing sensitive debug information because
APP_DEBUG=true
in a production environment.Mitigation in Laravel: Always set
APP_DEBUG=false
in production and ensure that sensitive keys are stored in.env
files.APP_DEBUG=false
6. Vulnerable and Outdated Components
Using outdated software libraries or components that have known vulnerabilities.
Example: Using an outdated version of a package that contains known vulnerabilities.
Mitigation in Laravel: Keep Composer dependencies updated regularly and check for vulnerabilities.
composer update composer audit # Check for known vulnerabilities
7. Identification and Authentication Failures
Weaknesses in the authentication system that allow attackers to assume other users' identities.
Example: Allowing weak passwords or not implementing account lockouts after multiple failed login attempts.
Mitigation in Laravel: Use Laravel Breeze or Laravel Fortify for secure authentication and enforce password policies.
// Example of enforcing strong password validation $request->validate([ 'password' => ['required', 'string', 'min:8', 'regex:/[a-z]/', 'regex:/[A-Z]/', 'regex:/[0-9]/', 'confirmed'], ]);
8. Software and Data Integrity Failures
Occurs when integrity checks (e.g., digital signatures) are missing or vulnerable components are loaded dynamically without verification.
Example: Allowing third-party dependencies without verifying their integrity.
Mitigation in Laravel: Use Composer's
"composer.lock"
file to lock down exact versions of dependencies and ensure code integrity.composer install --prefer-dist --no-dev --optimize-autoloader --no-scripts
9. Security Logging and Monitoring Failures
Failure to log security-relevant events and ensure proper monitoring of logs can lead to undetected security incidents.
Example: A failed login attempt is not logged, allowing brute-force attacks to go unnoticed.
Mitigation in Laravel: Log important events like login attempts, exceptions, and errors using Laravel’s logging system.
Log::info('User login attempt failed', ['user' => $request->email]);
10. Server-Side Request Forgery (SSRF)
Occurs when a server makes HTTP requests on behalf of a user, potentially exposing internal systems to attackers.
Example: A vulnerable API allows attackers to force the server to make requests to internal resources, potentially leaking sensitive data.
Mitigation in Laravel: Validate and sanitize all URLs used in HTTP requests.
// Always validate URLs before making requests $validated = $request->validate([ 'url' => 'required|url' ]); // Making safe external HTTP requests $response = Http::get($validated['url']);
Summary of OWASP Top Ten Risks:
Risk | Example in Laravel/PHP | Mitigation |
Broken Access Control | Unauthorized user accessing admin pages | Use role-based access and middleware |
Cryptographic Failures | Storing passwords in plaintext | Use Hash::make() for password encryption |
Injection | SQL injection via query parameters | Use Eloquent ORM or Query Builder |
Insecure Design | Not validating critical actions (e.g., payments) | Use Laravel validation rules |
Security Misconfiguration | APP_DEBUG=true in production | Set APP_DEBUG=false in production |
Vulnerable Components | Using outdated libraries | Regularly update dependencies via Composer |
Authentication Failures | Weak passwords, lack of account lockouts | Use Laravel Fortify, enforce strong passwords |
Data Integrity Failures | Loading unverified third-party code | Use Composer to lock dependencies |
Logging and Monitoring Failures | No logs of failed login attempts | Log critical security events |
SSRF | Allowing unvalidated URLs for server requests | Sanitize and validate URLs |
These OWASP Top Ten risks are important for securing any PHP/Laravel application. By implementing best practices and the built-in security features in Laravel, you can protect your application against these common vulnerabilities.
Authentication & Authorization
JWT (JSON Web Token): A token-based authentication mechanism where a client receives a signed token upon successful login. This token is then used in subsequent requests for authentication.
- Example in Laravel: You can implement JWT authentication using the "laravel/passport" package.
composer require laravel/passport
OAuth2: An authorization framework that enables third-party applications to obtain limited access to an HTTP service.
- Example in Laravel: OAuth2 can be implemented using Laravel Passport, which handles API authentication.
Passport::routes();
SAML (Security Assertion Markup Language): A standard for single sign-on (SSO) that exchanges authentication data between an identity provider and a service provider.
- Example in Laravel: SAML is not built-in but can be implemented using third-party libraries like "aacotroneo/laravel-saml2".
RBAC (Role-Based Access Control): A system for restricting access based on the roles of individual users within an organization. Each role has specific permissions.
- Example in Laravel: Using spatie/laravel-permission to manage roles and permissions.
// Assigning a Role
$user->assignRole('admin');
// Checking Permission
if ($user->hasPermissionTo('edit articles')) {
// Grant access
}
Data Encryption
Encryption Mechanisms: Encrypting sensitive data both during transit and at rest is essential for protecting it from unauthorized access.
In Transit: Use SSL/TLS to secure data in transit. Laravel uses HTTPS by default for production environments.
// In your .env file, ensure the app URL is HTTPS for secure transmission APP_URL=https://yourapp.com
At Rest: Laravel provides built-in encryption mechanisms using the encrypt() and decrypt() functions.
use Illuminate\Support\Facades\Crypt; // Encrypt Data $encrypted = Crypt::encryptString('Sensitive Data'); // Decrypt Data $decrypted = Crypt::decryptString($encrypted);
Hashing Passwords: Laravel uses the bcrypt algorithm by default to hash passwords securely.
// Hashing a password $hashedPassword = Hash::make('password'); // Verifying the hashed password if (Hash::check('password', $hashedPassword)) { // Password is valid }
These security practices are crucial for developing robust and secure web applications, especially when handling sensitive user data. Integrating them into your development flow significantly reduces vulnerabilities in your applications.
Subscribe to my newsletter
Read articles from Dale Lanto directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Dale Lanto
Dale Lanto
A passionate Full Stack and Backend Web Developer with 7+ years of experience, specializing in PHP, Laravel, and a range of modern web technologies. I enjoy solving complex problems, optimizing systems, and delivering efficient, maintainable code. Check out some of my work at dalelanto.netlify.app or explore my GitHub at github.com/dalelantowork.