How to Effortlessly Render Slack Blocks in React with ‘slack-blocks-to-jsx

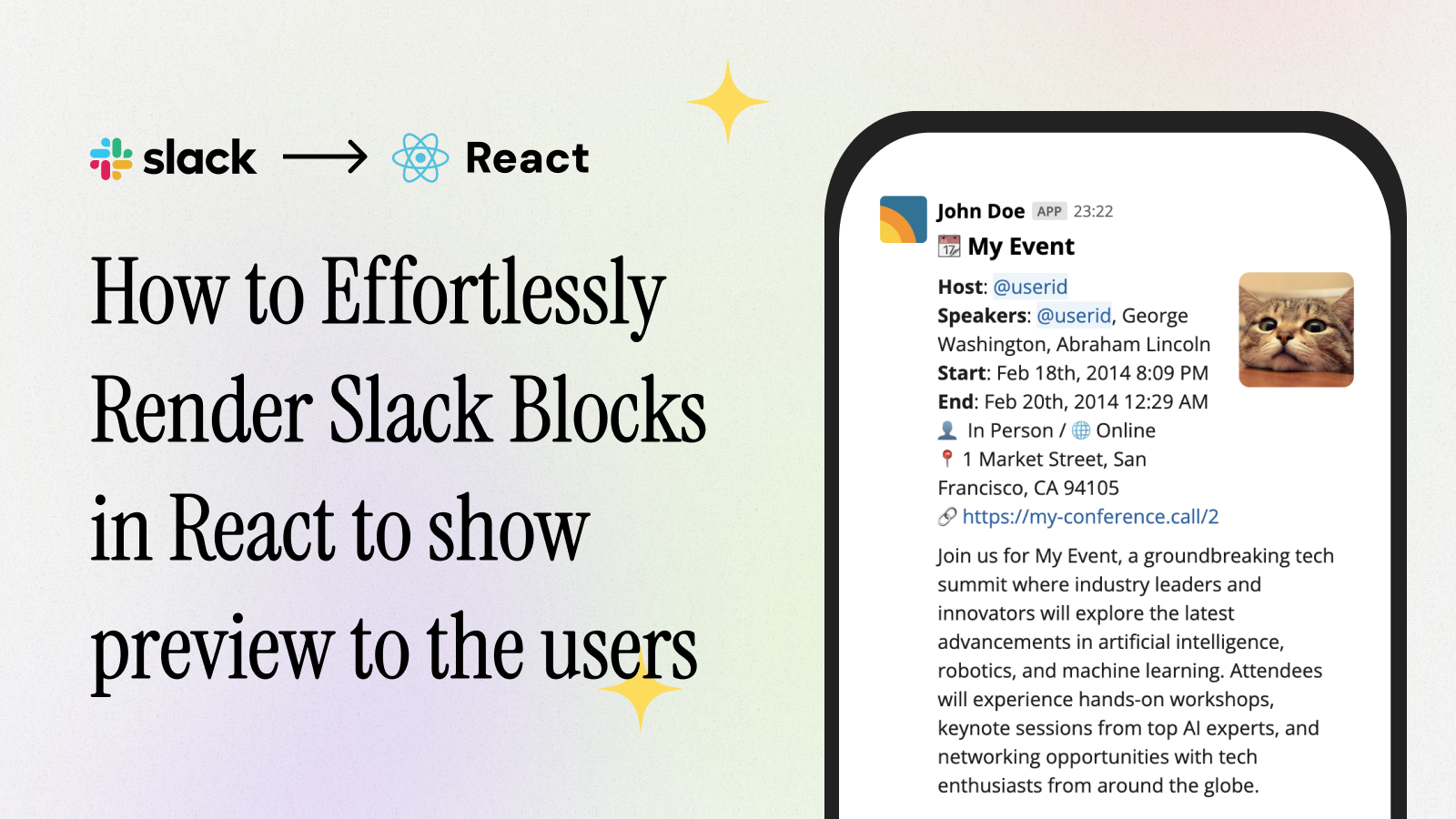
If you’ve ever worked with Slack’s Block Kit, you know it offers a great way to create interactive and visually appealing messages. But what if you want to replicate that same look and feel in a React application? That’s where the slack-blocks-to-jsx
library comes to the rescue. This handy tool allows you to render Slack blocks directly in React, maintaining the authentic Slack look and behavior. In this post, we'll explore how to leverage slack-blocks-to-jsx
to render Slack blocks/messages in React and make your web applications more engaging.
🔗 Want to try it out right now? Head over to the Playground and see the library in action!
🔍 What is slack-blocks-to-jsx
?
The slack-blocks-to-jsx
library allows you to render Slack blocks as React components with ease. It takes the JSON data structure that Slack's Block Kit uses and converts it into JSX, keeping the interactive and visual elements intact.
You don’t have to worry about recreating the styles or elements from scratch—the library ensures your Slack blocks appear just as they do within Slack. Plus, it offers customization options, so you can tweak the appearance as needed.
💡 Why Use This Library?
Quick and easy integration: No need to create custom components from scratch.
Authentic Slack experience: Visual fidelity that matches Slack's interface.
Interactive elements: Support for user mentions, channels, emojis, and more.
Customizable styling: Override styles to fit your brand or app design.
🚀 Getting Started
Step 1: Installation
To get started, you first need to install the library in your project. You can do this using either npm or yarn.
npm install slack-blocks-to-jsx
or
yarn add slack-blocks-to-jsx
Step 2: Import the Styles
To ensure that your rendered Slack blocks have the correct styles, import the library's CSS file in your main entry point (e.g., index.js
or App.js
).
import "slack-blocks-to-jsx/dist/style.css";
This step is essential for the components to mimic Slack's visual design accurately.
Step 3: Rendering Slack Blocks
Now that everything is set up, let's render some Slack blocks using the Message
component from the library.
import React from 'react';
import { Message } from 'slack-blocks-to-jsx';
const App = () => {
const blocks = [
{
type: 'section',
text: {
type: 'mrkdwn',
text: 'Hello, world! This is a Slack block rendered in React!',
},
},
{
type: 'divider',
},
{
type: 'section',
text: {
type: 'mrkdwn',
text: '*Did you know?* You can render Slack blocks effortlessly using this library! 🚀',
},
},
];
return (
<div className="App">
<Message
time={new Date()}
name="Slack Blocks Renderer"
logo="https://your-logo-url.com/logo.png"
blocks={blocks}
/>
</div>
);
};
export default App;
In the example above, we're passing an array of Slack blocks (blocks
) to the Message
component. The Message
component takes care of rendering these blocks as JSX, maintaining the look and feel of Slack.
Step 4: Explore a Detailed Example
Explore an example of a complex message rendered using slack-blocks-to-jsx
. This message features various interactive elements, highlighting the library's powerful capabilities.
🔧 Advanced Customization
One of the powerful features of slack-blocks-to-jsx
is its ability to handle more than just basic rendering. You can customize the behavior and appearance using various props.
Using Hooks for Custom Mentions
The library allows you to customize the way mentions, channels, and emojis are rendered using the hooks
prop.
<Message
blocks={blocks}
hooks={{
user: ({ id, name }) => <span style={{ color: 'blue' }}>@{name}</span>,
channel: ({ id, name }) => <span style={{ color: 'green' }}>#{name}</span>,
}}
/>
With the hooks
prop, you gain full control over how user mentions, channels, and other elements are rendered, making it easy to align the appearance with your app's design language.
Using the data
Prop for Automatic Replacements
You can also pass in predefined arrays of users, channels, and user groups using the data
prop. This allows automatic replacement of these mentions without the need for manual handling.
<Message
blocks={blocks}
data={{
users: [{ id: 'U123', name: 'John Doe' }],
channels: [{ id: 'C123', name: 'general' }],
}}
/>
🤩 Bonus Tool: Temlist – Ready-Made Templates
If you're looking for ready-made message templates for Slack (and soon, Microsoft Teams), check out Temlist. This handy tool offers pre-designed templates for welcome messages, introductions, community updates, surveys, and more.
You can copy and paste these templates directly into your app and render them using slack-blocks-to-jsx
. Temlist is a fantastic time-saver, especially when you want to quickly implement professional and engaging messages.
🛠️ Pro Tips
Test in Slack's Block Kit Builder: Use Slack's Block Kit Builder to craft your messages before implementing them in React. This ensures you get the structure and design just right.
Style Overrides: The library offers a set of CSS classes that you can override to customize the appearance of the blocks. Feel free to tweak the styles to match your app's theme.
Debugging: If you encounter any issues with rendering, set the
showBlockKitDebug
prop totrue
on theMessage
component to access a link for debugging in Slack's Block Kit Builder.
🌟 Wrapping It Up
Using the slack-blocks-to-jsx
library is a fantastic way to render Slack messages in React without the hassle of recreating components from scratch. It saves time, maintains the Slack aesthetic, and offers the flexibility you need to customize and interact with messages.
Combine this with ready-made templates from Temlist, and you’ll have a powerful toolkit for creating engaging and interactive Slack-like experiences in your React applications.
📢 Get Involved!
If you have any suggestions, feature requests, or bug reports, feel free to contribute to the GitHub repository or reach out via email. Let’s build something amazing together!
GitHub Repository Issues: Submit an Issue
Happy coding! 🎉
Subscribe to my newsletter
Read articles from Manish Panwar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Manish Panwar
Manish Panwar
I’m a self-taught Full Stack Developer who genuinely enjoys building solutions that make a difference and support business growth. In my journey, I’ve developed complex applications, shaped product strategies, and even dived into sales and marketing to help companies succeed. As a Founding Engineer at Wrenly AI, I helped expand our market by creating an MS Teams Bot that brought in 5+ new customers. But I’m not just about coding—I love mentoring junior developers, sharing ideas on product direction, and contributing to overall growth strategies. I’m also passionate about open-source projects and have created tools that other developers find helpful. If you’re looking to connect with someone who brings a mix of technical skills, strategic thinking, and a genuine team spirit, I’d love to chat!