Understanding Session-Based and Token-Based Authentication in API Design
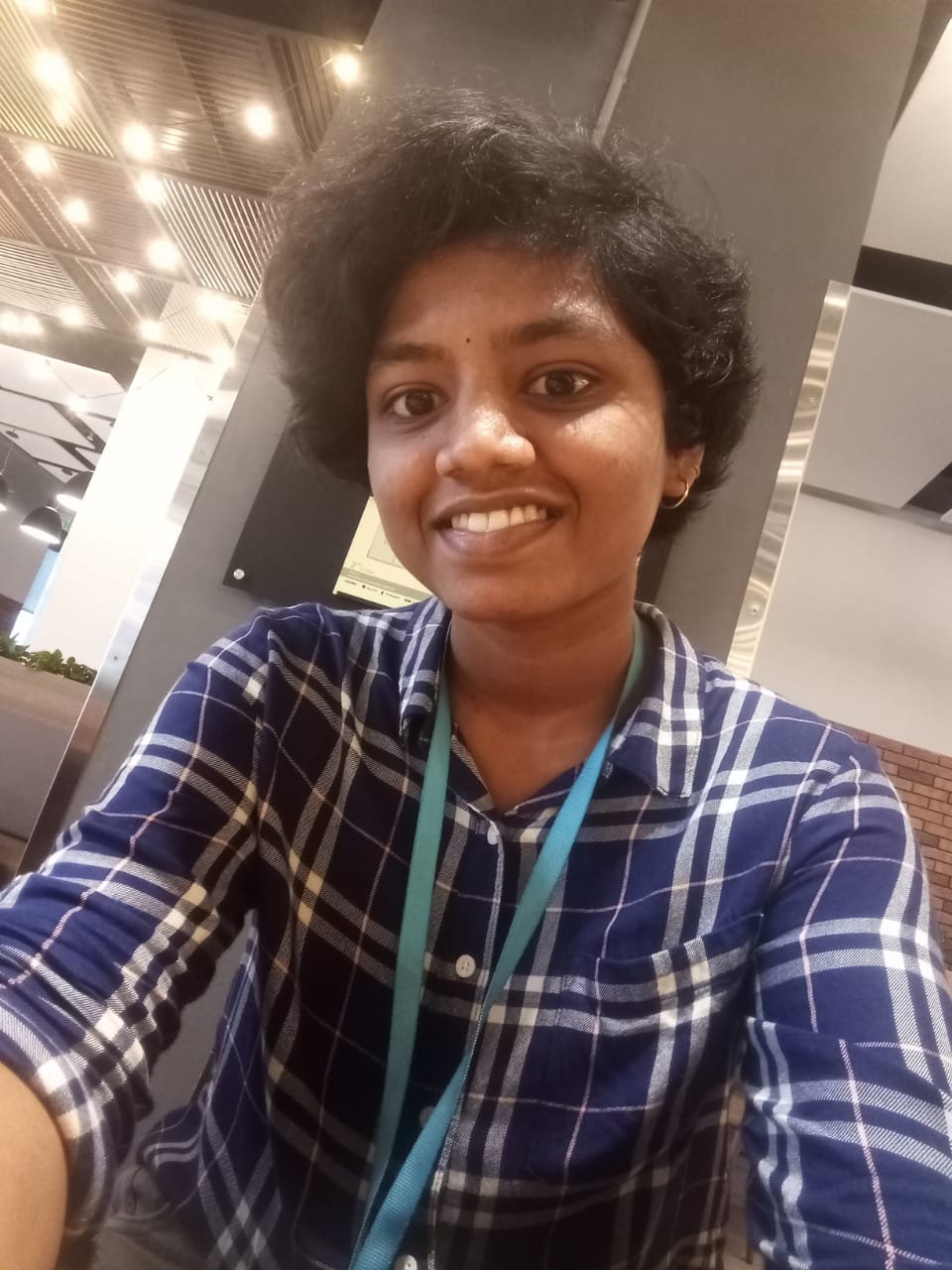
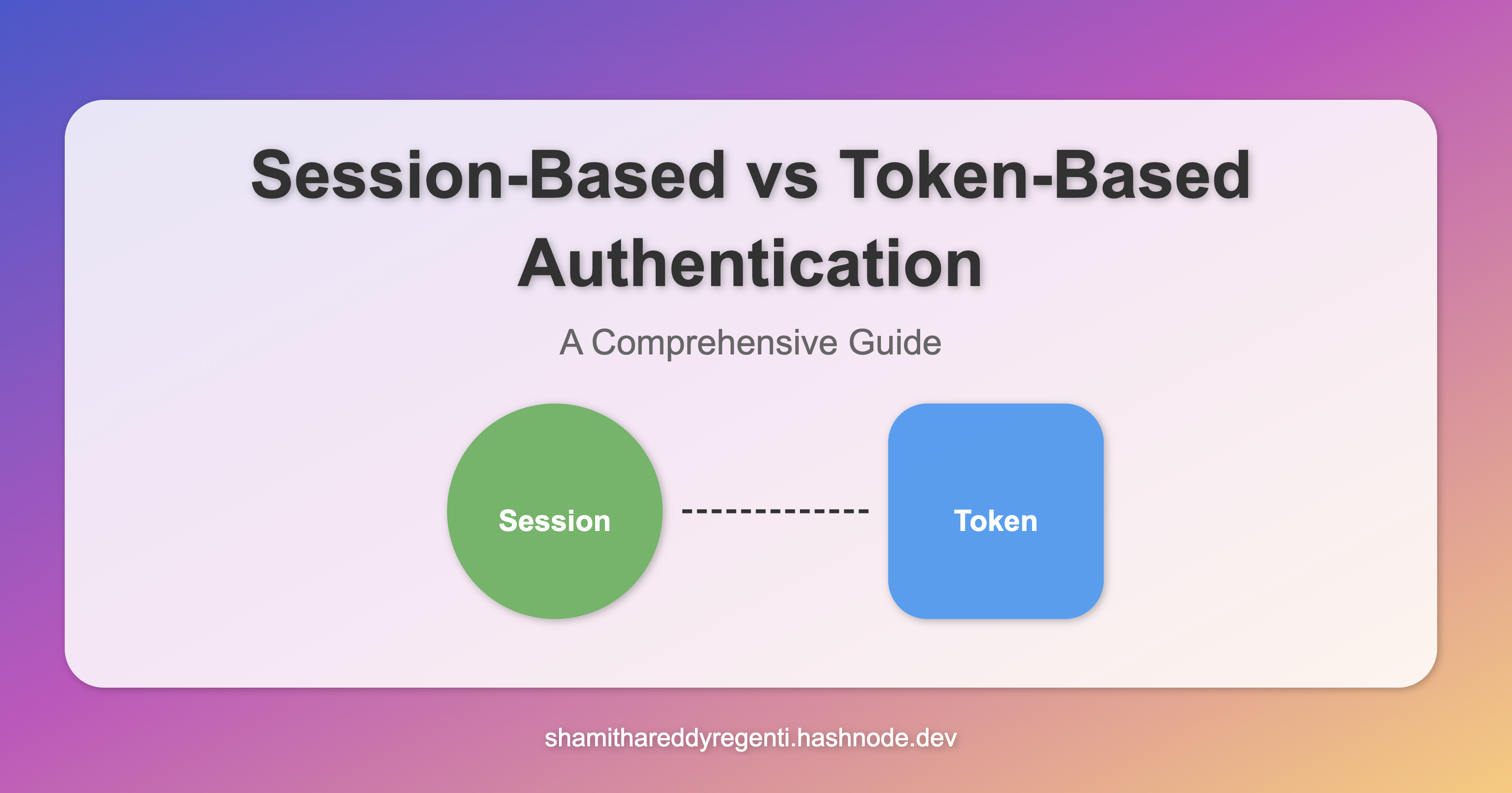
Ever wondered what happens when you try to authenticate into a website? what happens internally? what are all the internal authentication techniques one could apply as a backend engineer?
There are many ways to authenticate a user, listing some of the methods below:
๐ Types of Authentication:
Session-Based Authentication
Token-Based Authentication
Multi-factor Authentication (MFA): Requiring an additional layer of authentication, like an OTP or an authentication app ( Example: Microsoft Authenticator ).
Knowledge-based Authentication: Something the user knows (e.g., passwords, PINs)
Certificate-based Authentication: Uses digital certificates to identify users or devices.
Biometric Authentication: Uses unique physical characteristics for identification.
Single Sign-On (SSO): Allows users to access multiple applications with one set of credentials.
OAuth and OpenID Connect: Provides a framework to web applications to access limited user information from 3rd party authorizers like Google, FaceBook etc.
While in this article ill be explaining session-based and token-based authentication with examples in Java, but iโll also touch on other authentication types, providing an overview with helpful diagrams.
Stay tuned and Follow for future articles where we'll dive deeper into Single Sign-On (SSO), OAuth, and more advanced authentication methods๐
Session-Based Authentication:
Think of it like getting a wristband at an amusement park. You show your ticket (credentials) once, get a wristband (session ID), and use it for all rides (server requests).
Usecase: Traditional web applications like forums or simple e-commerce sites
User logs in with credentials.
Server verifies credentials.
If valid, server generates a unique session ID.
Session ID is stored on the server (in memory or database) along with user data.
Server sends the session ID to the client as a cookie.
Client includes this cookie in subsequent requests.
Server validates the session ID for each request.
How are the session Idโs generated?
๐ using cryptographic techniques, most commonly used is the UUID generators
๐จโ๐ป Java code example:
import java.util.UUID;
public class SessionIdGenerator {
public static String generateSessionId() {
return UUID.randomUUID().toString();
}
public static void main(String[] args) {
String sessionId = generateSessionId();
System.out.println("Generated Session ID: " + sessionId);
}
}
what happens if the If a user erases their cookies?
The session ID is lost on the client-side.
The server can't associate the user with their session.
The user will need to log in again to obtain a new session ID.
Disadvantages:
- Scalability issues due to server-side storage.
Token-Based Authentication:
This is more like having a VIP pass. You get a special pass (token) that contains info about you and what you're allowed to do. You show this pass every time you want to access something.
Usecase: Single-page applications (SPAs) and mobile apps
User logs in with credentials.
Server verifies credentials.
If valid, server generates a token (e.g., JWT).
Token is sent to the client.
Client stores the token (usually in local storage or a cookie).
Client includes the token in the Authorization header of subsequent requests.
Server validates the token for each request.
๐ Different Types of Tokens and their usecases:
Token Type | Explanation | Use Case |
JWT (JSON Web Token) | A secure way to send information between parties as a JSON object | Logging into a website and staying logged in while you navigate |
Access Token | Short-lived token that grants access to a protected resource | Third-party application accessing user data (e.g., "Login with Google") |
Refresh Token | A long-lived token that can get you a new access token when the old one expires | Staying logged into a mobile app for weeks without re-entering your password |
ID Token | A token that contains user profile information | Getting your name and email after logging in with "Sign in with Google" |
Java Example for JWT Token:
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import java.util.Date;
public class JWTGenerator {
private static final String SECRET_KEY = "yourSecretKey";
public static String generateJWT(String userId) {
return Jwts.builder()
.setSubject(userId)
.setIssuedAt(new Date())
.setExpiration(new Date(System.currentTimeMillis() + 3600000)) // 1 hour
.signWith(SignatureAlgorithm.HS256, SECRET_KEY)
.compact();
}
public static void main(String[] args) {
String jwt = generateJWT("user123");
System.out.println("Generated JWT: " + jwt);
}
}
Session-Based vs Token-Based Authentication:
Feature | Session-Based | Token-Based |
Storage | Server-side | Client-side |
Scalability | Limited | Better |
Stateless | No | Yes |
Cross-domain | Challenging | Easier |
Mobile-friendly | Less | More |
Multi-factor Authentication:
Requiring an additional layer of authentication, like an OTP or an authentication app ( Example: Microsoft Authenticator ), adds extra security.
The MFA comes under Possession-based Authentication which means something the user possesses, such as:
Security tokens
Smart cards
Mobile devices (for push notifications or authenticator apps)
๐ If you wanted to read more about MFA using Cloud, do checkout this article https://shamithareddyregenti.hashnode.dev/iam-in-cloud-and-setting-up-mfa-for-an-iam-user
Certificate-based Authentication:
Uses digital certificates to identify users or devices. This authentication is commonly used in scenarios which requires high security.
UseCase: SSL/TLS for web servers, VPN access for remote employees etc.
Biometric Authentication:
Uses unique physical characteristics for identification. This comes under the Inherence-based Authentication category which uses physical characteristics of the user.
UseCase: Smartphone unlock, Time and attendance systems in workplaces etc.
Single Sign-On (SSO):
Allows users to access multiple applications with one set of credentials.
UseCase: A company employee logging into their work computer and automatically gaining access to various internal tools.
OAuth and OpenID Connect:
OAuth 2.0 is an authorization framework that enables applications to obtain limited access to user accounts on an HTTP service.
OpenID Connect is an identity layer built on top of OAuth 2.0, adding authentication capabilities.
Use case: A common use case is "Login with Google/Facebook/Twitter" functionality in web and mobile applications. For example:
User wants to sign up for a new service
Service offers "Login with Google" option
User clicks and is redirected to Google's authentication page
User logs in with Google credentials
Google asks user to grant permissions to the service
User approves, and is redirected back to the service
Service receives an access token to retrieve basic profile information
references:
https://cheatsheetseries.owasp.org/cheatsheets/Authentication_Cheat_Sheet.html
https://developer.mozilla.org/en-US/docs/Web/HTTP/Authentication
Subscribe to my newsletter
Read articles from Shamitha Reddy Regenti directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
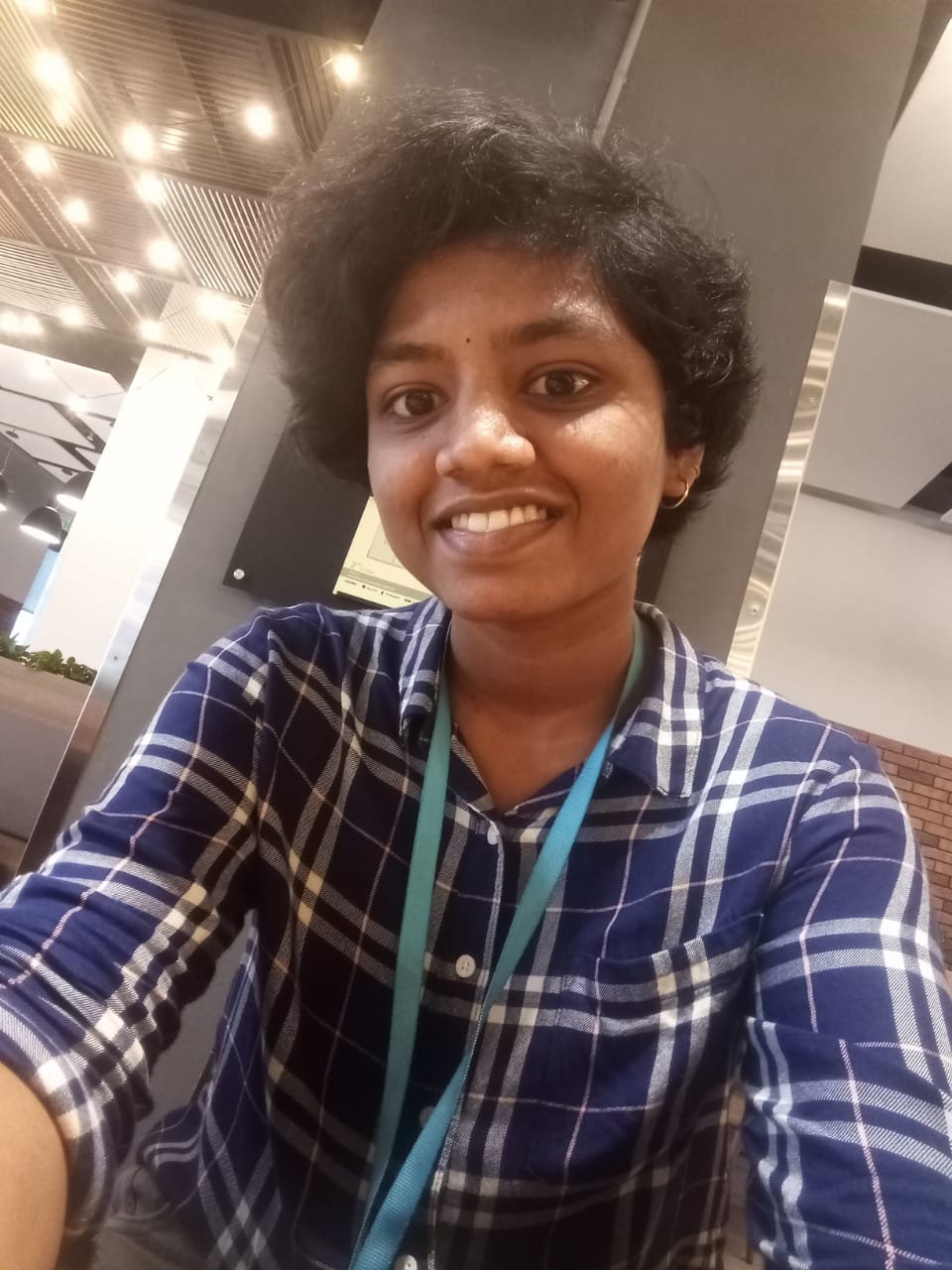
Shamitha Reddy Regenti
Shamitha Reddy Regenti
Hey everyone, I'm Shamitha, working as a programmer analyst at amazon. and also i teach DSA and AWS in a practical way. look me up at teacheron!