Understanding Concurrency vs. Parallelization
Table of contents
- Introduction
- Defining the Concepts
- Core Differences Between Concurrency and Parallelization
- Real-World Use Cases
- Mnemonics to Remember the Differences
- Visualizations of Concurrency and Parallelization
- Practical Implications for Developers
- Common Pitfalls and Challenges
- Conclusion
- Further Reading and Resources
- Frequently Asked Questions (FAQs)
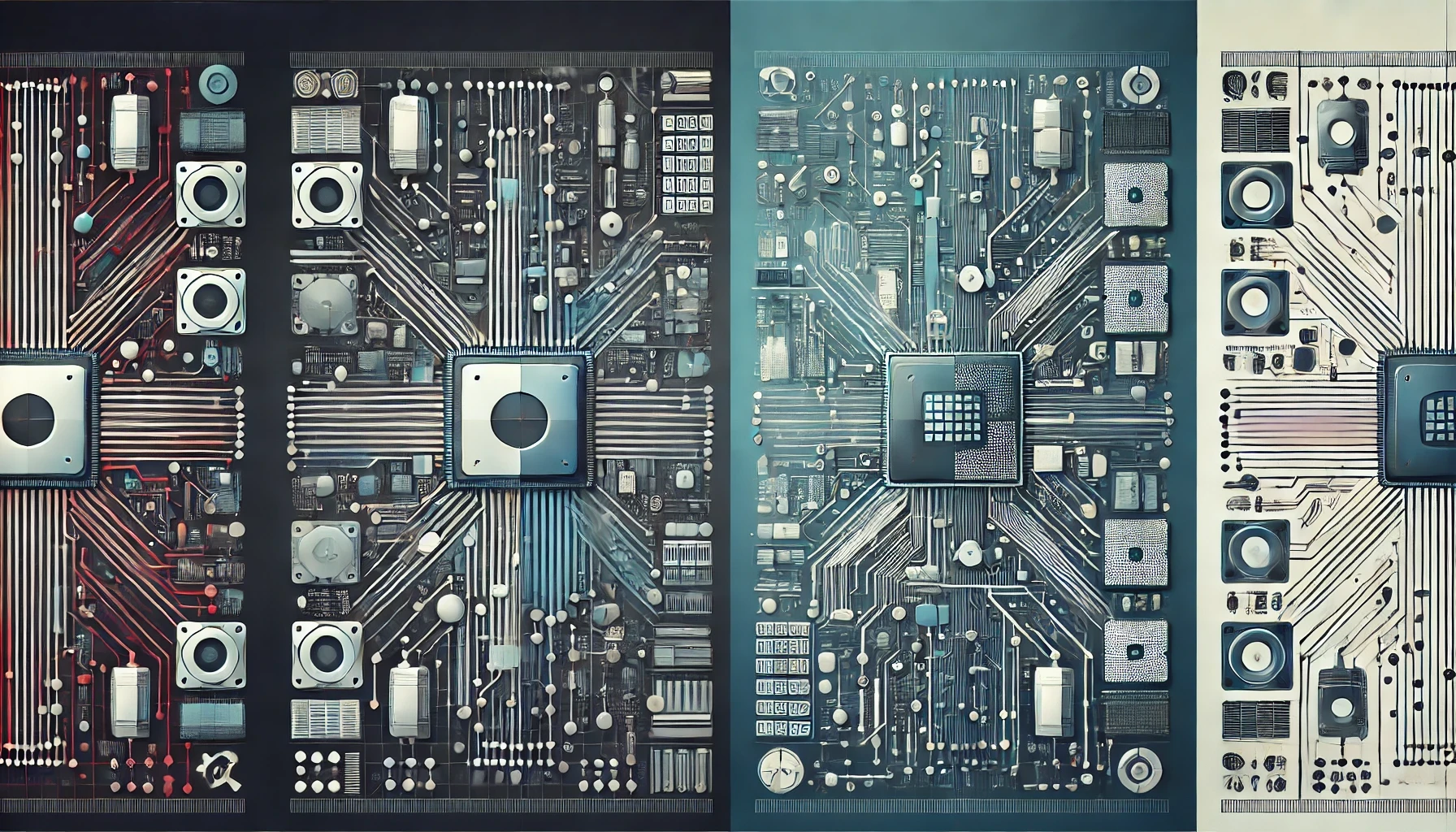
Introduction
Imagine you're in a busy kitchen during dinner rush hour. As the head chef, you're managing multiple dishes at once—checking the oven, chopping vegetables, and stirring a sauce. You aren't doing these tasks simultaneously, but you're switching between them quickly to keep everything moving. This is concurrency—handling many tasks by switching between them, making sure nothing burns while you're multitasking.
Now picture that same kitchen, but this time you're not alone. You have a team of chefs, each working on a different dish at the same time. One chef chops the vegetables, another stirs the sauce, and someone else checks the oven. Together, you're speeding up the entire process by working simultaneously. This is parallelization—dividing tasks across multiple workers to get things done faster.
In software development, concurrency and parallelization are two key concepts that define how tasks are handled and executed. Both are vital to improving efficiency and performance, but they work in different ways. Whether you're writing code for a responsive web server or speeding up computations in scientific applications, understanding the differences between these two approaches can help you optimize your projects.
Defining the Concepts
What is Concurrency?
Concurrency is about managing multiple tasks at the same time but not necessarily executing them simultaneously. Think of it as time-sharing or task-switching—each task gets a slice of time to make progress, and the system switches between them efficiently. The main goal of concurrency is to ensure that a system can handle multiple operations without slowing down, maintaining responsiveness even when handling many tasks.
Key characteristics of concurrency:
Task interleaving: Tasks appear to run in parallel, but they’re actually being processed one after another in short bursts.
Responsiveness: Concurrency helps systems remain responsive to new inputs, even when they’re already busy with other tasks.
Single or multiple cores: Concurrency can work on a single processor core by switching between tasks, but it can also leverage multiple cores to improve efficiency.
What is Parallelization?
Parallelization, on the other hand, involves breaking down a task into smaller parts that can be executed simultaneously on multiple processors or cores. The primary goal of parallelization is to increase speed and performance by doing more in less time. This approach is common in tasks that require heavy computation, such as processing large datasets, rendering graphics, or training machine learning models.
Key characteristics of parallelization:
Simultaneous execution: Tasks are divided and executed at the same time, utilizing multiple cores or processors.
Performance boost: Parallelization is used to reduce execution time by distributing the workload across multiple workers.
Requires multiple cores: Unlike concurrency, parallelization requires more than one core or processor to truly execute tasks simultaneously.
Core Differences Between Concurrency and Parallelization
While concurrency and parallelization are often discussed together, they operate in fundamentally different ways. Here's a side-by-side comparison of their key characteristics:
Aspect | Concurrency | Parallelization |
Execution Model | Task switching and interleaving, not simultaneous | Simultaneous execution of multiple tasks across multiple processors/cores |
Objective | Efficient task management and maintaining responsiveness | Speeding up execution by performing tasks at the same time |
Resource Utilization | Can operate on a single core or processor | Requires multiple cores or processors |
Speedup Potential | May not always result in faster execution, focuses on responsiveness | Designed to reduce execution time and improve performance |
Use Case Focus | Ideal for handling many tasks without requiring simultaneous execution | Best for heavy computation tasks needing faster completion times |
Real-World Use Cases
Concurrency and parallelization are applied in different scenarios depending on the goals of the system—whether it’s managing multiple tasks or speeding up performance. Here are some practical examples of both in action:
Concurrency Use Cases:
Web Servers: Modern web servers handle multiple client requests concurrently. Even if a single server has only one core, it can still manage thousands of connections by quickly switching between them, ensuring no request is left unattended.
User Interface (UI) Applications: In apps like video editors or web browsers, concurrency ensures the interface remains responsive. While a file is being loaded or an image is being processed in the background, the user can still scroll, click, and interact with the UI.
Operating Systems: Operating systems manage multiple processes at the same time, allocating CPU time to each one in quick bursts. This makes it possible to run multiple applications simultaneously without noticeable slowdowns.
Parallelization Use Cases:
Scientific Computing: Tasks like weather forecasting, protein folding simulations, or astrophysics modeling involve enormous amounts of data. Parallelization divides these computations across multiple cores or machines, drastically speeding up the time required to get results.
Video and Image Processing: Rendering a 3D scene or encoding large videos can be computationally intensive. Parallelization allows different parts of the rendering or encoding process to be handled at the same time, shortening the time required for the task.
Machine Learning: Training deep learning models on massive datasets can take days or even weeks. Parallelizing the computation across GPUs or clusters of machines reduces training time by processing multiple parts of the dataset simultaneously.
Hybrid Use Cases:
- Database Systems: Databases use concurrency to handle multiple queries at once, ensuring responsiveness, but they also parallelize large, complex queries to speed up the data retrieval and processing.
Mnemonics to Remember the Differences
To make the concepts of concurrency and parallelization easier to remember, here are some clever mnemonics:
Concurrency: "One Chef, Many Dishes"
- Imagine a single chef managing several dishes. They chop vegetables, stir a sauce, and check the oven, but they only work on one task at a time, constantly switching between them to keep everything on track. This is concurrency—managing multiple tasks by switching between them efficiently, without doing them all at once.
Parallelization: "A Team of Chefs"
- Now, imagine a kitchen with multiple chefs. Each chef is working on a different dish simultaneously—one chops vegetables, another stirs the sauce, and someone else handles the oven. This is parallelization—dividing the work across multiple workers (or processors) so tasks get done at the same time, speeding up the entire process.
These mnemonics should help you remember that concurrency is about juggling tasks, while parallelization is about doing tasks simultaneously.
Visualizations of Concurrency and Parallelization
Concurrency on a Single-Core CPU
Time
│
v
___ ___ ___ ___
|th1| ---> |th2| ---> |th3| ---> |th4| ---> (Single-core CPU switching between threads)
| | | | | | | |
|___| |___| |___| |___|
- Explanation: In concurrency, a single-core CPU manages multiple tasks (threads), but only one task is running at any given moment. It switches between tasks rapidly, making progress on all tasks, but never executing them at the same time.
Parallelization on a Multi-Core CPU
Time
│
v
___ ___ ___ ___ ___ ___ ___ ___
|th1|th2| |th3|th4| |th5|th6| |th7|th8| ---> (Multi-core CPU executing threads in parallel)
| | | | | | | | | | | |
|___|___| |___|___| |___|___| |___|___|
- Explanation: In parallelization, multiple cores execute different tasks at the same time. Each thread (task) runs on its own core, allowing the system to perform multiple operations simultaneously, thus improving performance and reducing execution time.
You’re welcome! Let's complete the rest of the article, keeping the quality and clarity high. Here’s the final portion:
Practical Implications for Developers
Understanding when to apply concurrency versus parallelization can help developers build more efficient and responsive applications. Here are some guidelines on when and how to use these techniques:
When to Use Concurrency:
Responsive Applications: If you’re building a web server or a user interface that must handle multiple tasks or requests simultaneously, concurrency helps manage those tasks efficiently without overwhelming the system.
I/O Bound Tasks: For tasks that spend a lot of time waiting on external resources (e.g., network requests, file reading), concurrency is beneficial. Instead of idly waiting for one task to finish, the system can switch to another task, improving overall responsiveness.
When to Use Parallelization:
Performance-Intensive Applications: If your application requires a lot of computation—such as processing large datasets, performing heavy calculations, or rendering graphics—parallelization will improve performance by splitting the workload across multiple cores or processors.
CPU Bound Tasks: When tasks are constrained by the CPU’s processing power (e.g., machine learning model training, simulations), parallelization will help by utilizing the full capacity of the hardware.
Best Practices:
Concurrency: Make use of asynchronous programming models (like async/await) to handle I/O-bound tasks. Ensure that you manage resources (such as threads or file handles) efficiently to avoid bottlenecks.
Parallelization: Use tools like OpenMP for parallel processing in C/C++ or joblib and multiprocessing in Python to take advantage of multi-core systems. Be mindful of synchronization to avoid race conditions.
Common Pitfalls and Challenges
Both concurrency and parallelization come with their own set of challenges. Understanding these pitfalls can help you avoid performance bottlenecks and bugs in your applications.
Concurrency Challenges:
Race Conditions: When two or more tasks access shared resources simultaneously, without proper synchronization, it can lead to inconsistent results.
Deadlocks: Occurs when two tasks are waiting for each other to release resources, causing both tasks to stall indefinitely.
Task Management Complexity: Managing multiple concurrent tasks can become complex as the number of tasks grows, leading to bugs that are difficult to reproduce and debug.
Parallelization Challenges:
Diminishing Returns: According to Amdahl’s Law, as you increase the number of parallel tasks, the time spent on the sequential portion of the task becomes the bottleneck, limiting the potential speedup.
Synchronization Overhead: Parallel tasks often need to synchronize to share data or complete a collective operation, and this can introduce delays, negating the performance benefits.
Hardware Limitations: Adding more cores or processors does not always guarantee better performance, especially if tasks require frequent communication or memory access, which can become the bottleneck.
Solutions:
Concurrency: Use proper locking mechanisms (mutexes, semaphores) to manage access to shared resources. Design your application to avoid circular dependencies, which can lead to deadlocks.
Parallelization: Break down tasks into as many independent sub-tasks as possible, minimizing the need for synchronization. Profile your application to understand where bottlenecks occur before adding more threads or cores.
Conclusion
Concurrency and parallelization are fundamental concepts that can drastically improve the performance and responsiveness of software applications, but they are not interchangeable. Concurrency helps manage multiple tasks by switching between them, making systems more responsive, while parallelization boosts performance by executing tasks simultaneously.
As a developer, it’s important to understand the nature of the tasks you are working with. Are you managing a lot of I/O-bound tasks? Concurrency is your friend. Are you trying to reduce the time spent on CPU-heavy computations? Parallelization will provide the speedup you need.
By understanding the differences, real-world applications, and potential pitfalls of concurrency and parallelization, you can make informed decisions that lead to better-designed systems.
Further Reading and Resources
For readers interested in diving deeper into these topics, here are some great resources:
Online Articles:
Tools and Libraries:
Python:
asyncio
,multiprocessing
,concurrent.futures
Java:
java.util.concurrent
packageC/C++: OpenMP, POSIX threads
Frequently Asked Questions (FAQs)
Q: Is concurrency always faster than sequential execution?
A: Not necessarily. Concurrency focuses on responsiveness and task management, not speed. It allows multiple tasks to make progress without waiting, but it doesn’t always lead to faster execution times, especially for CPU-bound tasks.
Q: Can I use concurrency and parallelization together?
A: Yes! Many systems use concurrency to handle multiple tasks and parallelization to speed up specific subtasks. For example, a web server might use concurrency to manage requests and parallelization to perform computations on those requests.
Q: What’s the main advantage of parallelization?
A: Parallelization shines when you need to improve performance by reducing the time it takes to complete a task. By dividing a task into smaller parts and running them simultaneously across multiple cores, you can significantly shorten execution time.
Q: How do I avoid deadlocks in concurrent programming?
A: Deadlocks occur when tasks are waiting on each other to release resources. To avoid deadlocks, ensure that tasks acquire resources in a consistent order, and try to use non-blocking synchronization mechanisms like locks with timeouts.
Q: Can concurrency and parallelization be used in everyday applications?
A: Absolutely. Many everyday applications, like web browsers and media players, use concurrency to manage multiple tasks (e.g., playing a video while loading a webpage) and parallelization to improve performance (e.g., decoding a video using multiple cores).
Subscribe to my newsletter
Read articles from Sean Coughlin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Sean Coughlin
Sean Coughlin
Software Engineer