Understanding Variadic Functions vs. Slice Parameters in Go
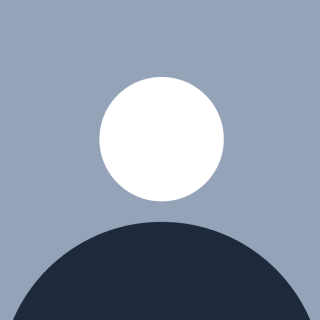
When writing functions in Go, you may encounter situations where you need to accept multiple arguments of the same type. Go provides two primary ways to handle this:
- Variadic Functions using the spread operator (
...
). - Functions that accept a slice parameter (
[]type
).
While both approaches allow you to work with multiple values, they differ in how you define the function and how you call it. In this blog post, we'll explore these differences in detail to help you choose the right approach for your needs.
Variadic Functions with the Spread Operator (...)
Defining Variadic Functions
Variadic functions allow you to accept a variable number of arguments. You define a variadic parameter using the spread operator (...
) before the type.
func myVariadicFunc(values ...int) {
// values is of type []int inside the function
}
Key Points:
- The variadic parameter must be the last parameter in the function signature.
- Inside the function, the variadic parameter is treated as a slice of the specified type (
[]int
in this case).
Calling Variadic Functions
You can call variadic functions in two ways:
- Passing individual arguments:
myVariadicFunc(1, 2, 3, 4, 5)
- Passing a slice with the spread operator:
nums := []int{1, 2, 3, 4, 5}
myVariadicFunc(nums...) // Note the '...'
Key Points:
- When passing a slice to a variadic function, you must expand it using the spread operator (
...
). - You can mix fixed parameters with variadic parameters:
func printNumbers(prefix string, nums ...int) {
fmt.Println(prefix, nums)
}
Functions with Slice Parameters
Defining Functions with Slice Parameters
Functions can also accept a slice as a parameter:
func mySliceFunc(values []int) {
// values is of type []int
}
Key Points:
- The function expects a single argument: a slice of the specified type.
- There are no restrictions on the position of the slice parameter in the function signature.
Calling Functions with Slice Parameters
You must pass a slice when calling the function:
nums := []int{1, 2, 3, 4, 5}
mySliceFunc(nums)
Key Points:
- You cannot pass individual arguments directly.
mySliceFunc(1, 2, 3, 4, 5) // This will result in a compilation error
Key Differences Between Variadic Functions and Slice Parameters
Function Calls
- Variadic Functions (
...
):- Can be called with zero or more individual arguments.
- Can accept a slice if expanded with the spread operator.
myVariadicFunc(1, 2, 3)
myVariadicFunc(nums...) // nums is a slice
- Slice Parameter Functions (
[]type
):- Must be called with a slice.
mySliceFunc(nums) // nums is a slice
Flexibility
Variadic Functions:
- More flexible in accepting arguments.
- Useful when the number of arguments is not fixed.
- Allows for cleaner and more intuitive function calls.
Slice Parameter Functions:
- Enforces that the caller provides a slice.
- Useful when the function logically operates on a collection.
Code Clarity and Intent
Variadic Functions:
- Indicates that the function is designed to handle a variable number of arguments.
- Ideal for functions like
fmt.Println
that need to accept any number of arguments.
Slice Parameter Functions:
- Indicates that the function operates on a pre-existing collection.
- Emphasizes that the data should be prepared before function invocation.
Practical Examples
Variadic Function Example
package main
import "fmt"
func sumNumbers(nums ...int) int {
total := 0
for _, num := range nums {
total += num
}
return total
}
func main() {
// Calling with individual arguments
fmt.Println(sumNumbers(1, 2, 3, 4, 5)) // Output: 15
// Calling with a slice
numbers := []int{6, 7, 8, 9, 10}
fmt.Println(sumNumbers(numbers...)) // Output: 40
}
Slice Parameter Function Example
package main
import "fmt"
func sumSlice(nums []int) int {
total := 0
for _, num := range nums {
total += num
}
return total
}
func main() {
numbers := []int{1, 2, 3, 4, 5}
fmt.Println(sumSlice(numbers)) // Output: 15
// Error: Cannot pass individual arguments
// fmt.Println(sumSlice(1, 2, 3, 4, 5)) // This will not compile
}
Conclusion
Variadic functions offer flexibility and cleaner syntax when dealing with a variable number of arguments, while slice parameter functions provide control and clarity when operating on collections.
Key Takeaways:
- Variadic Functions (
...
): Accept zero or more individual arguments and treat them as a slice within the function. - Slice Parameter Functions (
[]type
): Require a slice to be passed as a single argument, emphasizing the function's dependence on a collection.
Happy Coding!
Subscribe to my newsletter
Read articles from Manoj Parvathaneni directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by