Easy Routing in React: A React-Toolkit Tutorial

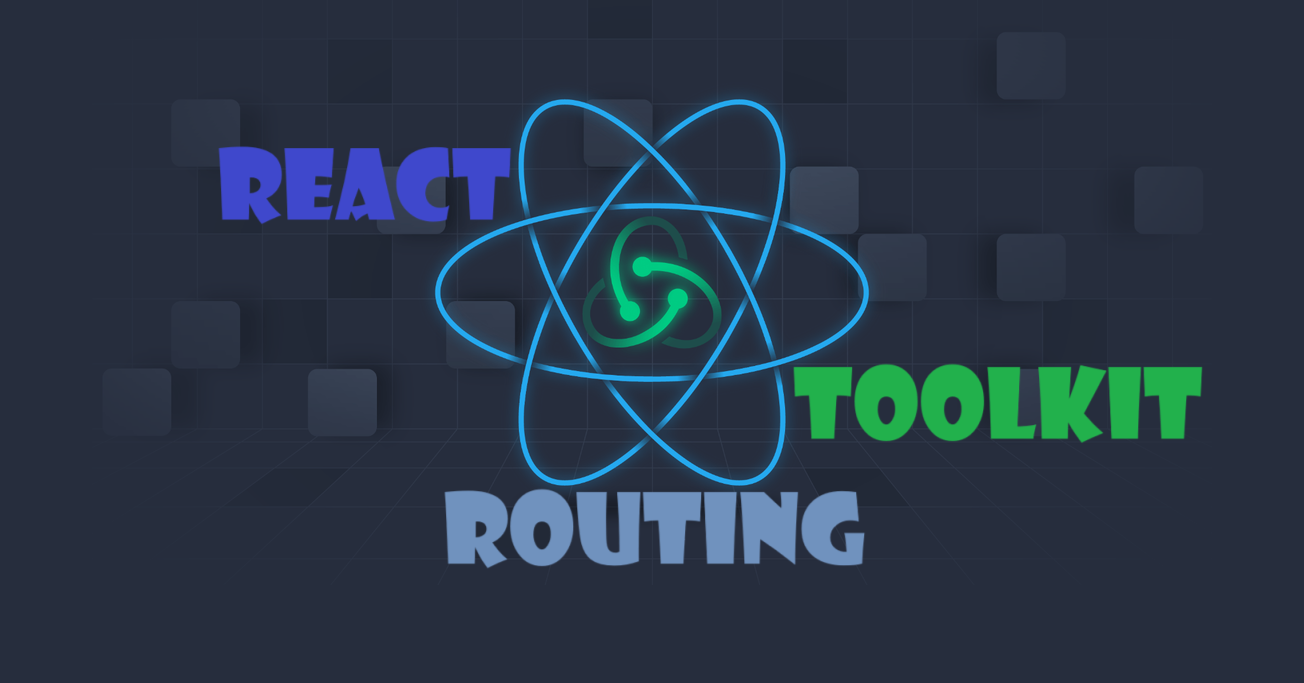
First Create a Folder with named anything. Open that Folder using VS Code. In vs code open terminal and write these command to create a React Application first. Here we are going to create react application using vite. Because of it is light weight.
npm create vite@latest my-app-name
After this, Vite create a React Apllication in your Folder. Now install other Dependencies. Like- React-router-dom, React-redux, @reduxjs/toolkit
npm i react-router-dom react-redux @reduxjs/toolkit
Now run your website to check weather it running properly or not using this command.
npm run dev
After Successfully Running, It look’s like.
Open main.jsx and import the following dependencies to Browse Route and Create Routes.
import { BrowserRouter } from 'react-router-dom'
And Wrap the <App/> components with <BrowserRouter><BrowserRouter/> Components that we just import. now your main.jsx page looks like this
import { StrictMode } from 'react'
import { createRoot } from 'react-dom/client'
import App from './App.jsx'
import './index.css'
import { BrowserRouter } from 'react-router-dom'
createRoot(document.getElementById('root')).render(
<StrictMode>
<BrowserRouter>
<App />
</BrowserRouter>
</StrictMode>,
)
Now open App.jsx and remove all the lines froms the return block. and Import these dependencies.
import { Route, Routes } from 'react-router-dom'
Make sure that, every Route you declear is inside the Routes.
Create a folder name it - pages inside the src folder. And in the pages folder create three pages. One is Login.jsx and another is Register.jsx and last is Home.jsx.
In all pages write the react functional component with default export.
Open Login.jsx page and write a h1 that hold “This is Login Page.“ Same for Register page that hold “This is Register Page“ and same for Home Page.
After this, Open App.jsx and inside the return Bring Routes Component from 'react-router-dom' that we just import.
Now inside <Routes><Routes/> write your Route Like this.
The Routes wraps all of your Route and this line <Route path='/' element={<Home/>}/> is the parent of the Login and Register. here we declear Home page as parent and Login and Register are the children pages.
<Routes>
<Route path='/' element={<Home/>}/>
<Route path='login' element={<Login/>}/>
<Route path='register' element={<Register/>}/>
</Route>
</Routes>
if you want to define another route that is not the children of Home. You can write like this.
<Routes>
<Route path='/' element={<Home/>}/>
<Route path='login' element={<Login/>}/>
<Route path='register' element={<Register/>}/>
</Route>
<Route path='your-path' element={<your-component/>}/>
</Routes>
make sure your all Route is under Routes, and if you declear any children then the children must be inside the <Route><Route/>
Now run your application using the npm run dev
and write url.
http://localhost:5173/ - this url serve home page content. And if you want to access childrens the just write your pathhttp://localhost:5173/login
http://localhost:5173/register
You get your content of that components
That’s it.
Happy Coding. 😊😊
Subscribe to my newsletter
Read articles from RIMANSHU SINGH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

RIMANSHU SINGH
RIMANSHU SINGH
I'm a passionate frontend developer with a knack for crafting immersive user experiences. Over the years, I've honed my skills in ReactJS, MongoDB, Redux, React-Toolkit, and SQL, leveraging these tools to deliver robust and dynamic web applications. I am passionate about explore and learning new things and willing to work beyond my capacity to administer projects. 💼 Currently, Diving deep into MEAN stack