Learn Data Structures and Algorithms: A Beginner's Introduction

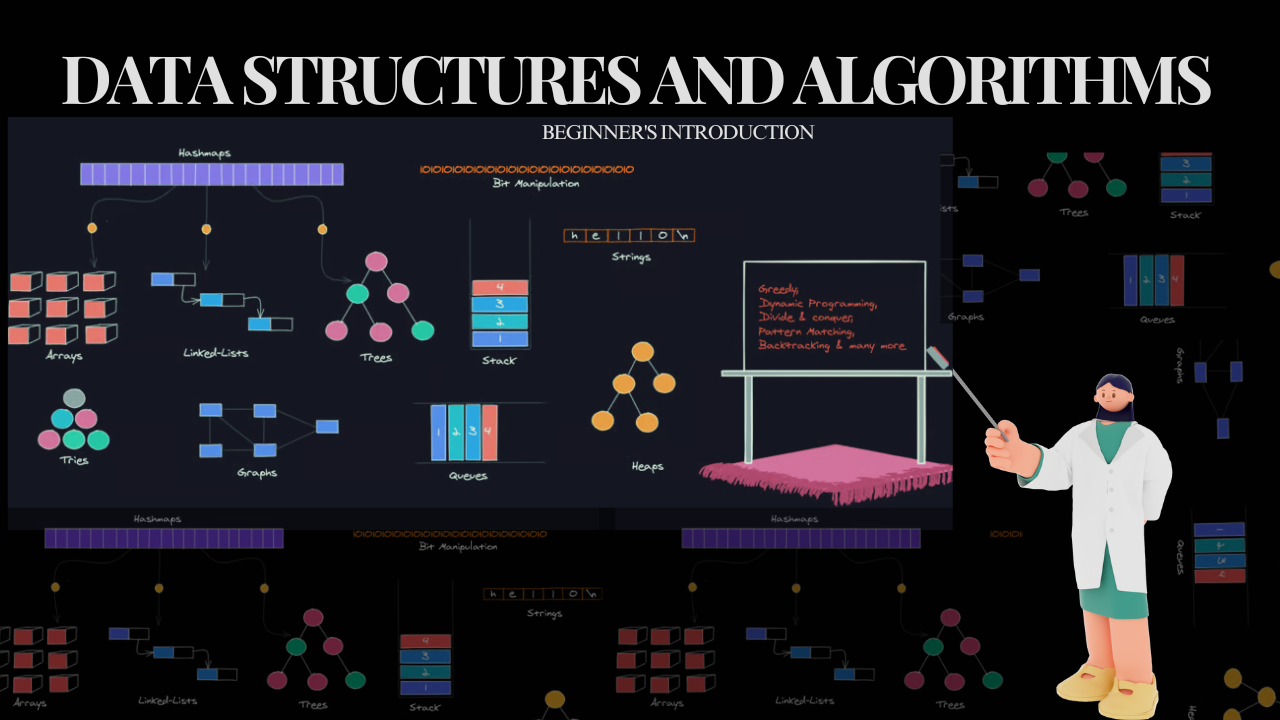
When you start your coding journey, one term that keeps popping up is "DSA" – Data Structures and Algorithms. At first, it may seem like an abstract, intimidating concept, but in reality, DSA is the heart of computer science and a key skill for every programmer.
What Exactly is DSA?
Data Structures refer to the way we organize and store data, while Algorithms are the step-by-step procedures to perform a task or solve a problem. Think of data structures as containers that hold your data in specific ways, and algorithms as the recipes or instructions to manipulate that data efficiently.
In simpler terms:
Data Structures: How we store data to make it easier to access and manipulate.
Algorithms: The techniques we use to operate on that stored data, whether it's sorting, searching, or optimizing.
Why is DSA Important?
Every program you write involves data in one form or another. How you handle that data can make or break the efficiency of your code. This is where DSA comes in – it allows you to choose the right method to store and manipulate data based on the problem you're solving.
Here’s why mastering DSA matters:
Efficiency: Proper data structures and algorithms help your programs run faster and use less memory.
Problem Solving: DSA enhances your ability to break down problems and find optimal solutions.
Interview Prep: For most tech jobs, especially at top companies like Google, DSA skills are a must-have for coding interviews.
Real-World Applications: Whether you’re building a search engine, a recommendation system, or simply sorting user data, you'll rely on DSA techniques to get the job done.
Key Data Structures and Algorithms
Some common data structures you’ll encounter include:
Arrays: A fundamental data structure that stores a fixed-size sequence of elements in contiguous memory locations. Arrays enable efficient index-based access, making them ideal for scenarios where quick retrieval is necessary.
Linked Lists: A dynamic data structure consisting of a sequence of elements, or nodes, where each node contains a reference (or pointer) to the next node in the sequence. Linked lists are particularly useful for applications that require frequent insertion and deletion of elements, as they can easily adapt to changes in size.
Stacks and Queues: Abstract data types that organize data based on specific access rules.
Stacks operate on a Last-In-First-Out (LIFO) principle, where the most recently added element is the first to be removed. They are commonly used in function calls and undo mechanisms.
Queues follow a First-In-First-Out (FIFO) principle, where the first element added is the first to be removed, making them suitable for task scheduling and resource management.
Trees: A hierarchical data structure composed of nodes, with a single root node and potentially many levels of additional nodes (children). Trees are often employed in decision-making systems, database indexing, and representing hierarchical relationships in data.
Graphs: Data structures that consist of nodes (vertices) connected by edges, used to represent relationships between objects. Graphs are versatile and can model various real-world scenarios, such as social networks, transportation systems, and web page link structures.
In addition to data structures, understanding algorithms is equally important. Key categories of algorithms include:
Sorting Algorithms: Techniques for arranging data efficiently in a specified order. Common sorting algorithms include:
Bubble Sort: A straightforward algorithm that repeatedly steps through the list, comparing adjacent elements and swapping them if they are in the wrong order.
Merge Sort: A more efficient algorithm that employs a divide-and-conquer strategy, recursively dividing the dataset into smaller subarrays, sorting them, and merging them back together.
Quick Sort: An efficient sorting algorithm that selects a pivot element to partition the array into smaller segments, leading to improved average-case performance.
Searching Algorithms: Methods for retrieving specific data from a structure. Notable searching algorithms include:
Binary Search: An efficient algorithm that operates on sorted arrays by repeatedly dividing the search interval in half, achieving a time complexity of O(log n).
Linear Search: A simple approach that examines each element sequentially, suitable for unsorted data with a time complexity of O(n).
Dynamic Programming: A powerful optimization technique that is used to solve complex problems by breaking them down into simpler overlapping subproblems. By storing the results of subproblems, dynamic programming reduces redundant calculations, enhancing efficiency. This technique is widely applied in scenarios such as the Fibonacci sequence, the Knapsack problem, and the Longest Common Subsequence problem.
Conclusion
Mastering DSA is crucial for becoming an efficient and well-rounded programmer. It may seem daunting at first, but with consistent practice, you’ll begin to see how these concepts apply to real-world problems. In the following posts, we’ll dive deeper into individual data structures and algorithms, breaking them down so they’re easier to understand and apply.
Stay tuned, and let’s start this exciting journey into the world of Data Structures and Algorithms!
Subscribe to my newsletter
Read articles from Keerthi Ravilla Subramanyam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Keerthi Ravilla Subramanyam
Keerthi Ravilla Subramanyam
Hi, I'm Keerthi Ravilla Subramanyam, a passionate tech enthusiast with a Master's in Computer Science. I love diving deep into topics like Data Structures, Algorithms, and Machine Learning. With a background in cloud engineering and experience working with AWS and Python, I enjoy solving complex problems and sharing what I learn along the way. On this blog, you’ll find articles focused on breaking down DSA concepts, exploring AI, and practical coding tips for aspiring developers. I’m also on a journey to apply my skills in real-world projects like predictive maintenance and data analysis. Follow along for insightful discussions, tutorials, and code snippets to sharpen your technical skills.