Understanding Time Complexity: The Key to Efficient Algorithms

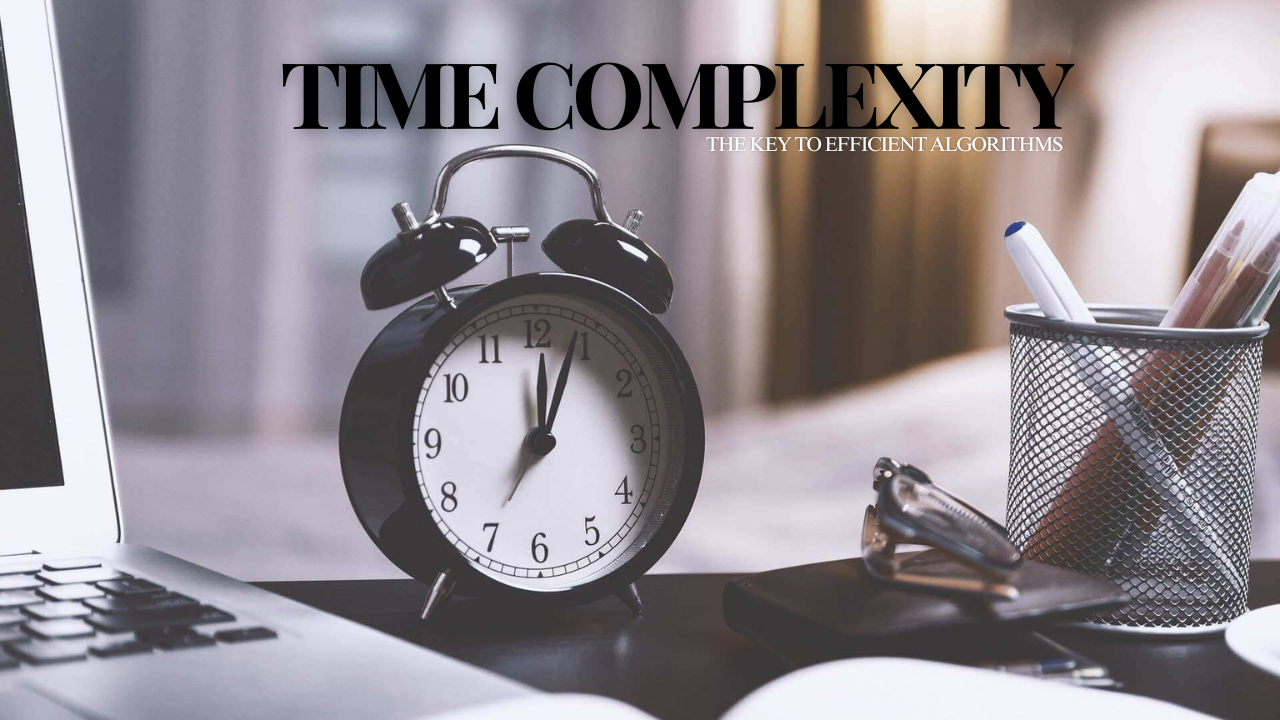
When learning Data Structures and Algorithms (DSA), one of the most critical concepts to master is time complexity. You might have heard terms like O(n) or O(log n), but what do they mean, and why do they matter? In this article, we'll break down the idea of time complexity in a way that's easy to grasp, so you can begin writing efficient algorithms from the start.
Time complexity is a way to describe how the runtime of an algorithm grows as the input size increases. Think of it like this: the larger the dataset or the more complex the problem, the longer it will take for an algorithm to complete its task. Time complexity gives us a way to predict that increase and determine how "fast" an algorithm really is, even as the problem size changes.
Understanding time complexity is essential because when you're dealing with large datasets (which is common in the real world), you want to ensure your code runs as efficiently as possible.
The Big O Notation
In DSA, time complexity is most commonly expressed using Big O Notation. It might seem intimidating at first, but it’s simply a mathematical way to express how the runtime of an algorithm behaves as the input size grows.
Here’s a basic breakdown of what the most common notations mean:
O(1): Constant Time
The algorithm takes the same amount of time, regardless of the input size.
Example: Accessing an element in an array by its index.
O(n): Linear Time
The runtime grows in direct proportion to the input size.
Example: Searching for a specific item in an unsorted list.
O(log n): Logarithmic Time
The runtime grows slowly, even as the input size increases dramatically. This is typical in algorithms that halve the input size with each step.
Example: Binary search in a sorted array.
O(n²): Quadratic Time
The runtime grows exponentially as the input size increases. This can be slow and inefficient for large datasets.
Example: A simple sorting algorithm like Bubble Sort.
How to Calculate Time Complexity
Now that we understand the notations, let’s dive into how we actually calculate time complexity. To do this, we need to examine the operations inside an algorithm and count how many times each operation is performed relative to the size of the input.
Here’s a step-by-step process to help you calculate time complexity:
Identify the Basic Operations:
Look at the code and identify the basic operations (e.g., arithmetic operations, comparisons, array accesses). These operations are typically what affect the runtime.
For example, in a loop, each iteration usually corresponds to a basic operation.
Determine the Input Size (n):
- The input size, often denoted as "n," refers to the number of elements in the input (such as the length of an array or the number of nodes in a tree).
Analyze Loops and Recursion:
For Loops: If you have a simple loop that runs
n
times, the time complexity is usually O(n). If there’s a nested loop, you multiply the loops, resulting in O(n²).Recursion: For recursive algorithms, the time complexity is often calculated using recurrence relations. A common example is binary search, where the input size is halved with each recursive call, leading to a time complexity of O(log n).
Focus on the Worst Case:
- Time complexity is generally expressed in terms of the worst-case scenario. For example, if you are searching for an item in an array, the worst-case scenario is that the item is not in the array, so you must search through all
n
elements.
- Time complexity is generally expressed in terms of the worst-case scenario. For example, if you are searching for an item in an array, the worst-case scenario is that the item is not in the array, so you must search through all
Ignore Constants:
- When calculating time complexity, constants are ignored. For example, O(2n) is simplified to O(n) because we’re interested in how the algorithm scales as the input size grows, and constants don’t affect scalability.
Example: Calculating Time Complexity for a Simple Algorithm
Let’s say you have the following code:
def sum_array(arr):
total = 0
for num in arr:
total += num
return total
Step 1: Basic Operation: The main operation here is adding each number to
total
.Step 2: Input Size: The input size
n
is the length of the arrayarr
.Step 3: Loop Analysis: The loop runs once for each element in
arr
, meaning it runsn
times.Step 4: Worst Case: In the worst case, the algorithm will have to iterate over every element in the array.
Step 5: Ignore Constants: There are no significant constants to account for here.
The time complexity of this algorithm is O(n) because the loop runs n
times.
Real-World Example: Linear Search vs. Binary Search
Let’s take two common search algorithms to see how time complexity plays a role.
Linear Search (O(n)): You have a list of unsorted numbers, and you need to find a specific one. A linear search would check each number one by one until it finds the right one. If you have 100 items, the worst-case scenario is that it checks all 100.
Binary Search (O(log n)): Now, if the list is sorted, you can use binary search. This algorithm cuts the list in half each time, significantly reducing the number of checks. In the worst case, with 100 items, it only needs about 7 comparisons to find the number.
Conclusion
Mastering time complexity is crucial for writing efficient algorithms, especially when you're working with large datasets. Understanding the basic principles of Big O Notation and knowing how to calculate time complexity will help you optimize your code and choose the best algorithms for the job.
As you continue your journey into Data Structures and Algorithms, keep time complexity in mind—it’s one of the keys to becoming an effective problem solver!
Subscribe to my newsletter
Read articles from Keerthi Ravilla Subramanyam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Keerthi Ravilla Subramanyam
Keerthi Ravilla Subramanyam
Hi, I'm Keerthi Ravilla Subramanyam, a passionate tech enthusiast with a Master's in Computer Science. I love diving deep into topics like Data Structures, Algorithms, and Machine Learning. With a background in cloud engineering and experience working with AWS and Python, I enjoy solving complex problems and sharing what I learn along the way. On this blog, you’ll find articles focused on breaking down DSA concepts, exploring AI, and practical coding tips for aspiring developers. I’m also on a journey to apply my skills in real-world projects like predictive maintenance and data analysis. Follow along for insightful discussions, tutorials, and code snippets to sharpen your technical skills.