Python Control Statements Explained - Day 13 Learning

Table of contents
- Introduction :
- Control Statement :
- Example 1:
- Example 2 :
- Example 3 :
- Example 4 :
- Example 5:
- Example 6 :
- #wap to take a number is user input & count how many digits are available
- #wap to take a number is user input & count how many digits are available & from that digits display only even number.
- #wap to display first n number where n value given by user , from that number find sum of all even & product of all odd.
- #Wap to find all factors & count number fo factors and check that number is prime.
- #WAP to find factors, and count number of factors , sum of factors & display in desc order and check that numebr is prime or not.
- #WAP to Display all number 1 to 500 which is divided by both 3 & 5 not with 4 and from that list of numbers show all even numeber & find sum of all even number & find max even number
- #WAP to find count & display all prime aval in 1 to 500.
- Challenges :
- Resources :
- Goals for Tomorrow :
- Conclusion :
- Connect with me :
- Join the conversation :
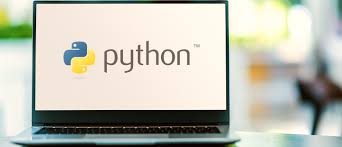
Introduction :
Welcome back to my Python journey! Yesterday, I laid the foundation with control structures in python.
Today, I dove into control statements, essential building blocks of any programming language. Let's explore what I learned!
Control Statement :
while Loop:
in c / c++ / java -
initilization;
while( condition )
{
body;
update value;
}
in Python:
initialization
while condition :
body
update value
Example 1:
Print python 5 Times using while loop.
from time import sleep
i = 1
while i <= 5 :
print ( "hello")
i += 1
sleep( 1) #in sec
Example 2 :
Print 1 to 10 in one Line.
i =1
while i <= 10:
print (i) #print (i,end = ' ')
i += 1
Example 3 :
To display the sum of first n numbers
n=int(input("Enter number:"))
sum=0
i=1
while i<=n:
sum=sum+i
i=i+1
print("The sum of first",n,"numbers is :",sum)
Example 4 :
write a program to prompt user to enter some name until entering jango
name=""
while name!="jango":
name=input("Enter Name:")
print("Thanks for confirmation")
Example 5:
Print multiplication table of a number
number = int(input('Enter a number'))
i =1
while i <= 10:
print ( i * number, end = " ")
i += 1
Example 6 :
Print Multiplication table of 1 to 10
from time import sleep
number = 1
while number <= 10:
i =1
while i <= 10:
print ( i * number, end = ' ')
i += 1
number +=1
print ()
sleep(1)
#wap to take a number is user input & count how many digits are available
x= int (input ("Enter a number"))
'''
count = 0
while x != 0 :
count +=1
x //= 10
print (count)
'''
print (len (str (x)))
#wap to take a number is user input & count how many digits are available & from that digits display only even number.
x= int (input ("Enter a number"))
count = 0
while x != 0 :
count+=1
n = x % 10 if n % 2 == 0 :
print ("even number=",n, end = " ")
x//=10
print ("\nnumber of digits=",count)
#wap to display first n number where n value given by user , from that number find sum of all even & product of all odd.
n= int (input ("Enter N value "))
i =1
sum = 0
odd= 1
while i <= n :
print (i)
if i % 2 == 0 :
sum = sum + i
else :
odd = odd * i
i+=1
print ("\nsum of all even=", sum)
print ("product of all odd =", odd)
#Wap to find all factors & count number fo factors and check that number is prime.
no = int (input ("Enter a number"))
i =1
list = []
while i <= no :
if no % i == 0 :
list.append (i)
i+=1
print (list, "number of factors", len (list))
print ("prime" if len (list) == 2 else "not prime")
#WAP to find factors, and count number of factors , sum of factors & display in desc order and check that numebr is prime or not.
no = int (input ("Enter a number"))
factor = []
i = 1
while i <= no :
if no % i == 0 :
factor. append (i)
i += 1
print("factor list=", factor, "\n", "number of factors=", len (factor) )
print ("sum of factors =", sum (factor), "\n", "desc sort =", sorted (factor, reverse = True ))
print ("primenumber" if len (factor)== 2 else "Not prime ")
#WAP to Display all number 1 to 500 which is divided by both 3 & 5 not with 4 and from that list of numbers show all even numeber & find sum of all even number & find max even number
l1 =[]
l2 =[]
i=1
while i <= 500 :
if i %3 ==0 and i % 5 == 0 and i % 4 != 0:
l1.append (i)
if i % 2 == 0 :
l2.append (i)
i += 1
print ("number which is divide by both 3 & 5 but not with 4=", l1)
print ("even list which is divide by both 3 & 5 but not with 4=", l2)
print ("sum of all even =", sum (l2), "\n","max even number=", max (l2))
#WAP to find count & display all prime aval in 1 to 500.
l =[]
i =1
while i <= 500:
j =1
nf = 0
while j <= i :
if i % j == 0 :
nf +=1
j +=1
if nf == 2 :
l.append (i)
i+=1
print ("prime list =", l, "number of prime =", len (l))
Challenges :
Understanding loop iteration.
Handling errors.
Resources :
Official Python Documentation: Control Structures
W3Schools' Python Tutorial: Control structures
Scaler's Python Course: Control Flow
Goals for Tomorrow :
Explore something more about control structure and statements.
Learn about control statements.
Conclusion :
Day 13’s a success!
What are your favorite Python resources? Share in the comments below.
Connect with me :
GitHub: [ https://github.com/p-archana1 ]
LinkedIn : [ https://www.linkedin.com/in/archana-prusty-4aa0b827a/ ]
Join the conversation :
Share your own learning experiences or ask questions in the comments.
HAPPY LEARNING!!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.