Inheritance
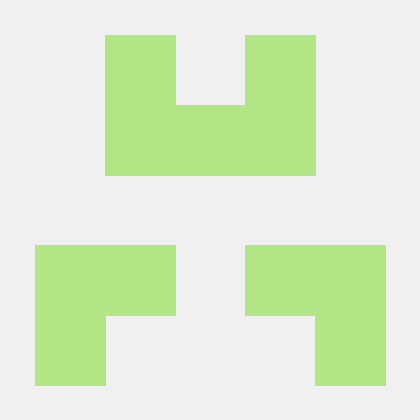
Inheritance is the mechanism by which one class acquires the properties and features of another class. The class that inherits the properties is called a sub-class (child class), while the class from which the properties are inherited is called a super-class (parent class). In Java, the keyword extends
is used to inherit properties from the parent class to the child class.
Example:
class Parent {
void display() {
System.out.println("This is the Parent class");
}
}
class Child extends Parent {
void show() {
System.out.println("This is the Child class");
}
}
public class InheritanceExample {
public static void main(String[] args) {
Child c = new Child();
c.display(); // Inherited method from Parent class
c.show(); // Method from Child class
}
}
Single Level Inheritance
Single level inheritance occurs when a class inherits from only one superclass. This is the most basic form of inheritance.
Example:
class Animal {
void eat() {
System.out.println("Animal is eating");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Dog is barking");
}
}
public class SingleLevelInheritance {
public static void main(String[] args) {
Dog d = new Dog();
d.eat(); // Inherited method
d.bark(); // Method from Dog class
}
}
Multi-level Inheritance
In multi-level inheritance, a class can inherit from a class that is already a subclass of another class.
Example:
class Animal {
void eat() {
System.out.println("Animal is eating");
}
}
class Mammal extends Animal {
void walk() {
System.out.println("Mammal is walking");
}
}
class Dog extends Mammal {
void bark() {
System.out.println("Dog is barking");
}
}
public class MultiLevelInheritance {
public static void main(String[] args) {
Dog d = new Dog();
d.eat(); // Inherited from Animal
d.walk(); // Inherited from Mammal
d.bark(); // Method from Dog class
}
}
Hierarchical Inheritance
Hierarchical inheritance occurs when multiple classes inherit from a single superclass.
Example:
class Animal {
void eat() {
System.out.println("Animal is eating");
}
}
class Dog extends Animal {
void bark() {
System.out.println("Dog is barking");
}
}
class Cat extends Animal {
void meow() {
System.out.println("Cat is meowing");
}
}
public class HierarchicalInheritance {
public static void main(String[] args) {
Dog d = new Dog();
Cat c = new Cat();
d.eat(); // Inherited from Animal
d.bark(); // Method from Dog class
c.eat(); // Inherited from Animal
c.meow(); // Method from Cat class
}
}
Multiple Inheritance (Not supported directly in Java)
In Java, multiple inheritance is not supported through classes to avoid complexity and ambiguity. However, multiple inheritance is possible using interfaces.
Hybrid Inheritance (Combination of two or more types of inheritance)
Hybrid inheritance is a combination of more than one type of inheritance. Since Java does not support multiple inheritance directly through classes, hybrid inheritance is also handled through interfaces.
We Will learn more about multiple and hybrid inheritance in Abstraction topic.
Subscribe to my newsletter
Read articles from Kandadi Manasa directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
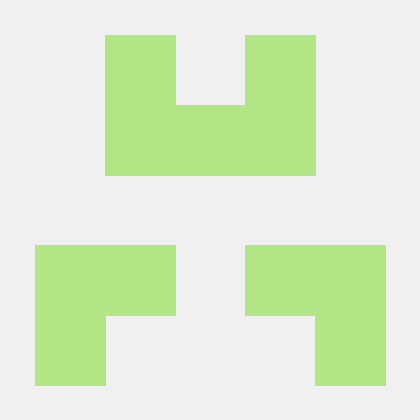