How to Handle Events in NestJS with the Event Emitter
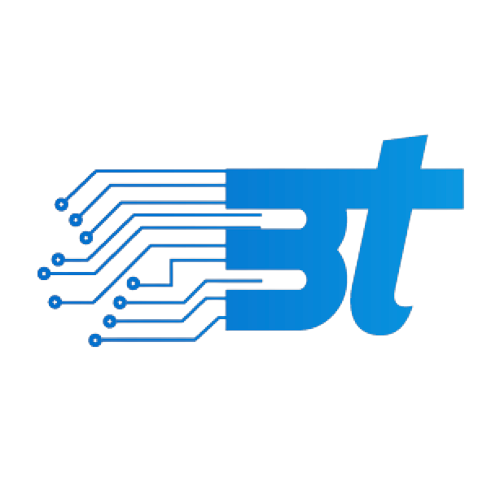
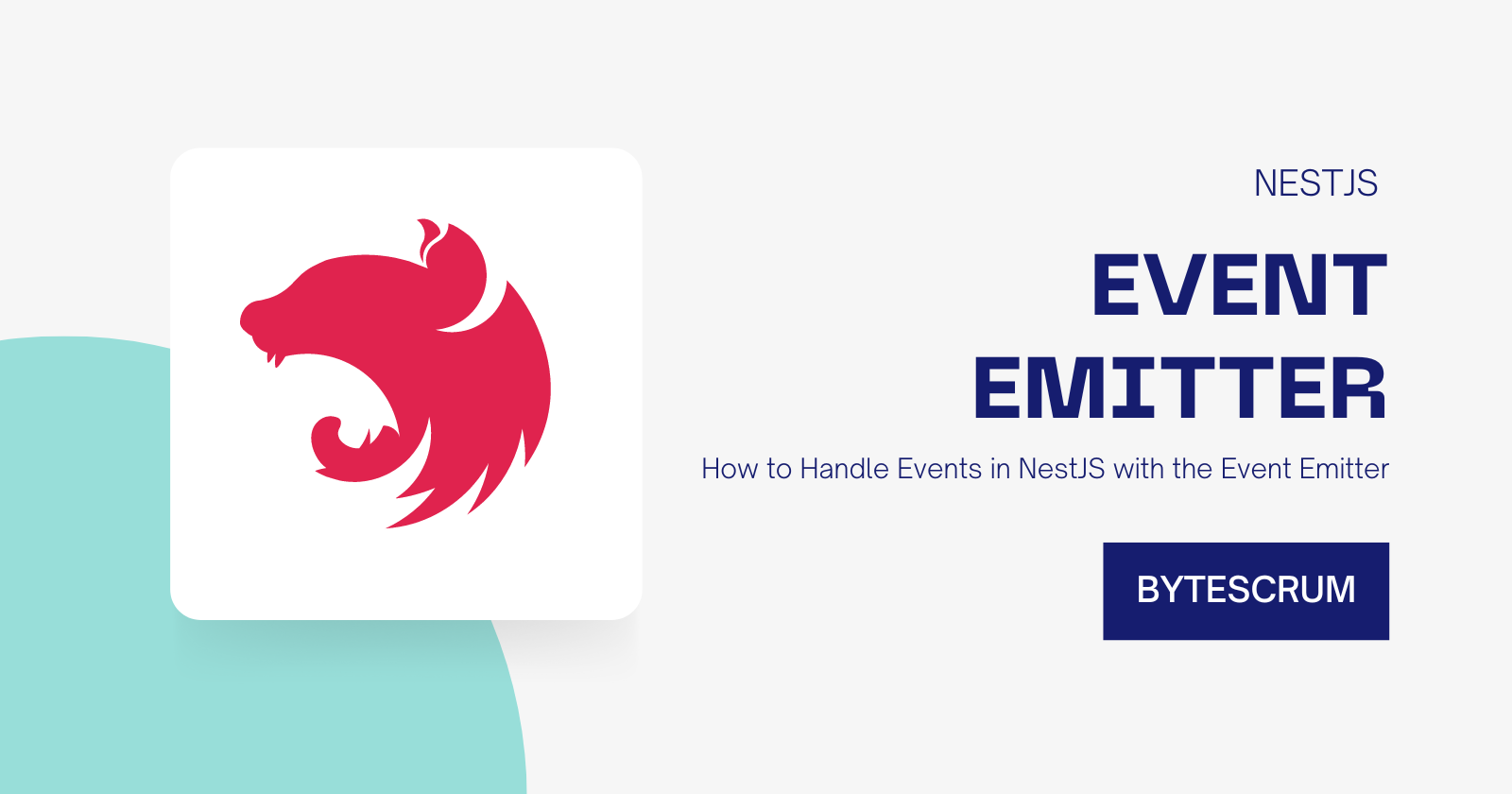
NestJS is a progressive Node.js framework that provides an out-of-the-box application architecture, making it ideal for building highly scalable, maintainable, and testable applications. One of its lesser-discussed but highly useful features is the Event Emitter—a mechanism that allows you to decouple different parts of your application by letting them communicate through events.
In this blog, we will explore how to use the Event Emitter in NestJS, why it's beneficial, and provide an example of a real-world use case. Let's dive in!
What is Event Emitter?
An Event Emitter is a pattern that allows different parts of your application to communicate asynchronously by emitting and listening to events. It's particularly useful for decoupling modules and handling tasks like logging, notifications, and any form of side effects. This is achieved by separating the logic that triggers an event from the logic that handles it.
NestJS provides an easy-to-use, built-in solution for event-driven development using the @nestjs/event-emitter
package.
Why Use Event Emitters in NestJS?
Here are some of the primary advantages of using the Event Emitter pattern in NestJS:
Decoupling: By emitting events, you allow different services or modules to subscribe to these events and respond accordingly without tight coupling. This results in a cleaner, more modular design.
Asynchronous Task Handling: If you need to execute tasks that are not directly related to the main flow of your application, like sending emails or notifications, using event emitters can handle such tasks asynchronously.
Scalability: Event emitters provide a scalable way to manage side effects or background tasks as your application grows, without cluttering the main business logic.
Setting Up Event Emitter in NestJS
Let's start by adding the Event Emitter package to our NestJS project. You'll need to install the required dependency and set it up within your application.
Installation
To install the Event Emitter package in NestJS, run the following command:
npm install @nestjs/event-emitter
Configuration
Once the package is installed, you need to import the EventEmitterModule
in the root module (AppModule
) of your NestJS application.
import { Module } from '@nestjs/common';
import { EventEmitterModule } from '@nestjs/event-emitter';
@Module({
imports: [
EventEmitterModule.forRoot(), // Register Event Emitter globally
],
})
export class AppModule {}
Here, the EventEmitterModule.forRoot()
method initializes the event emitter system globally, making it available for your entire application.
How to Use Event Emitter in NestJS
Step 1: Define an Event
Events in NestJS can be any custom event that suits your application's needs. Typically, you create a TypeScript interface to define the structure of the event.
Here’s an example of an event called UserCreatedEvent
that is triggered when a new user is created:
export class UserCreatedEvent {
constructor(
public readonly userId: string,
public readonly email: string,
) {}
}
This event holds information about the newly created user, such as their ID and email.
Step 2: Emitting Events
To emit an event, inject the EventEmitter2
service into your class and call the emit()
method, passing the event name and the event data.
In the following example, we'll emit the UserCreatedEvent
inside a service when a user is created:
import { Injectable } from '@nestjs/common';
import { EventEmitter2 } from '@nestjs/event-emitter';
import { UserCreatedEvent } from './events/user-created.event';
@Injectable()
export class UserService {
constructor(private eventEmitter: EventEmitter2) {}
createUser(userId: string, email: string) {
// Business logic to create the user
console.log(`User created: ${userId}, ${email}`);
// Emit the event after the user is created
const userCreatedEvent = new UserCreatedEvent(userId, email);
this.eventEmitter.emit('user.created', userCreatedEvent);
}
}
In the createUser()
method, after creating the user, we emit the user.created
event along with the event data (i.e., UserCreatedEvent
).
Step 3: Listening to Events
Now that we've emitted an event, we need a way to listen for it. In NestJS, we can easily create event listeners by using the @OnEvent()
decorator provided by the @nestjs/event-emitter
package.
Here’s how we can listen to the user.created
event and perform some actions, such as sending a welcome email:
import { Injectable } from '@nestjs/common';
import { OnEvent } from '@nestjs/event-emitter';
import { UserCreatedEvent } from './events/user-created.event';
@Injectable()
export class NotificationService {
@OnEvent('user.created')
handleUserCreatedEvent(event: UserCreatedEvent) {
// Handle the event, e.g., send a welcome email
console.log(`Sending welcome email to ${event.email}`);
}
}
In the above example, the handleUserCreatedEvent()
method listens for the user.created
event. When the event is triggered, it logs a message indicating that a welcome email is being sent to the user.
Real-World Use Case: Sending Notifications on User Actions
Let’s consider a real-world use case where an event-driven system can be very effective. Suppose you have a social media platform, and whenever a user creates a post, you want to notify their followers asynchronously.
Here’s how you can set this up with NestJS Event Emitters:
Define the Event: You’ll need an event that holds information about the post that was created:
export class PostCreatedEvent { constructor( public readonly postId: string, public readonly authorId: string, ) {} }
Emit the Event: When a user creates a new post, emit the
PostCreatedEvent
:import { Injectable } from '@nestjs/common'; import { EventEmitter2 } from '@nestjs/event-emitter'; import { PostCreatedEvent } from './events/post-created.event'; @Injectable() export class PostService { constructor(private eventEmitter: EventEmitter2) {} createPost(postId: string, authorId: string) { // Business logic for creating the post // Emit the post.created event const event = new PostCreatedEvent(postId, authorId); this.eventEmitter.emit('post.created', event); } }
Listen to the Event: Now, you can have a notification service that listens for the event and notifies the followers:
import { Injectable } from '@nestjs/common'; import { OnEvent } from '@nestjs/event-emitter'; import { PostCreatedEvent } from './events/post-created.event'; @Injectable() export class NotificationService { @OnEvent('post.created') handlePostCreatedEvent(event: PostCreatedEvent) { // Business logic to notify followers of the author console.log(`Notifying followers of author ${event.authorId} about the new post ${event.postId}`); } }
In this use case, the event-driven approach ensures that the post creation logic remains clean and focused on its task, while the notification logic is handled independently by another module.
Error Handling with Event Emitters
Error handling is crucial when working with event emitters, as different parts of your application might fail, and you need to ensure that these failures are managed gracefully. NestJS allows you to catch errors during event emission and processing.
Here's how you can handle errors effectively:
1. Wrapping Event Emitters in Try-Catch Blocks
When emitting an event, wrap the emission in a try-catch
block to handle any unexpected errors:
try {
this.eventEmitter.emit('user.created', userCreatedEvent);
} catch (error) {
console.error('Error emitting user.created event:', error);
}
This ensures that if an error occurs during the event emission, it doesn’t crash your entire application.
2. Handling Errors in Event Listeners
Similarly, it’s a good idea to handle potential errors in the event listener as well. You can do this by adding a try-catch
block inside the listener method:
@OnEvent('user.created')
handleUserCreatedEvent(event: UserCreatedEvent) {
try {
// Process event
console.log(`Processing user created event for ${event.email}`);
} catch (error) {
console.error('Error handling user.created event:', error);
}
}
This makes your application more resilient to failures during event processing.
Advanced Features
NestJS Event Emitter offers some advanced features to further enhance the event-driven architecture. Let’s explore them briefly:
1. Event Prioritization
By default, event listeners are invoked in the order they are registered. However, NestJS allows you to prioritize certain listeners over others. This can be useful when you want a specific listener to execute before others.
To prioritize an event listener, pass a second argument to the @OnEvent()
decorator:
@OnEvent('user.created', { priority: 1 }) // Higher priority
handleCriticalEvent(event: UserCreatedEvent) {
console.log('Critical event listener executed first');
}
Listeners with a higher priority value will be invoked first.
2. Wildcard Event Listeners
NestJS also supports wildcard event listeners, allowing you to listen for multiple events with a single handler. This is useful when you want to react to a group of related events.
Here’s an example:
@OnEvent('user.*')
handleUserEvents(event: any) {
console.log('A user-related event occurred:', event);
}
In this case, the handleUserEvents
method will respond to any event that starts with user.
, such as user.created
, user.updated
, etc.
3. Event Payload Transformation
You can also transform event payloads before processing them in listeners. This can be useful if you want to modify the data passed to a listener without affecting the original event emission logic.
Simply transform the event data inside your listener:
@OnEvent('user.created')
handleUserCreatedEvent(event: UserCreatedEvent) {
const transformedData = {
...event,
timestamp: new Date().toISOString(),
};
console.log('User created with additional timestamp:', transformedData);
}
Best Practices for Using Event Emitters
To get the most out of the Event Emitter pattern in NestJS, it’s important to follow best practices:
Decouple Modules: Use event emitters to decouple business logic from secondary processes like logging, sending notifications, or auditing. This separation makes your code cleaner and easier to maintain.
Avoid Excessive Event Emission: While events are useful, emitting too many events can clutter your code and make it harder to follow. Use event emitters for critical processes and avoid unnecessary emissions.
Handle Errors Gracefully: Always wrap event emission and listener logic in
try-catch
blocks to handle errors gracefully. This prevents a single failure from crashing your entire application.Use Event Prioritization: If certain listeners are more critical than others, take advantage of the
priority
option in@OnEvent()
to ensure that they are executed first.
Event-driven architectures offer a powerful way to build scalable and maintainable applications by decoupling different components. NestJS's Event Emitter provides a simple yet flexible way to implement such patterns. By emitting and listening to events, you can handle side effects like notifications, logging, and background tasks asynchronously, keeping your main application logic clean and modular.
Whether you're handling user actions, notifications, or complex workflows, the Event Emitter in NestJS can enhance your application's architecture, making it more maintainable and scalable.
If you haven’t already, consider integrating the Event Emitter pattern into your next NestJS project. It will make your code more modular, and your app will be better equipped to handle background processes, all while remaining clean and maintainable. Happy coding!
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
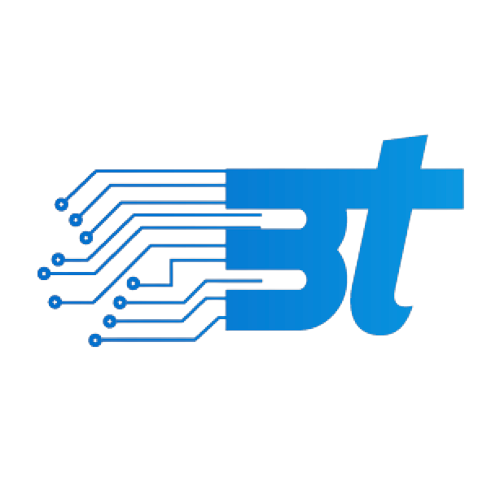
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.