Arrays: The Ultimate Linear Data Structure
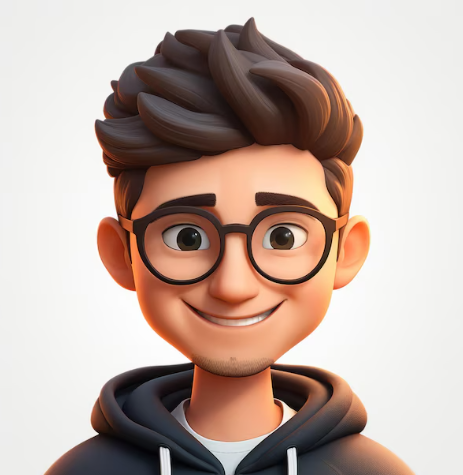
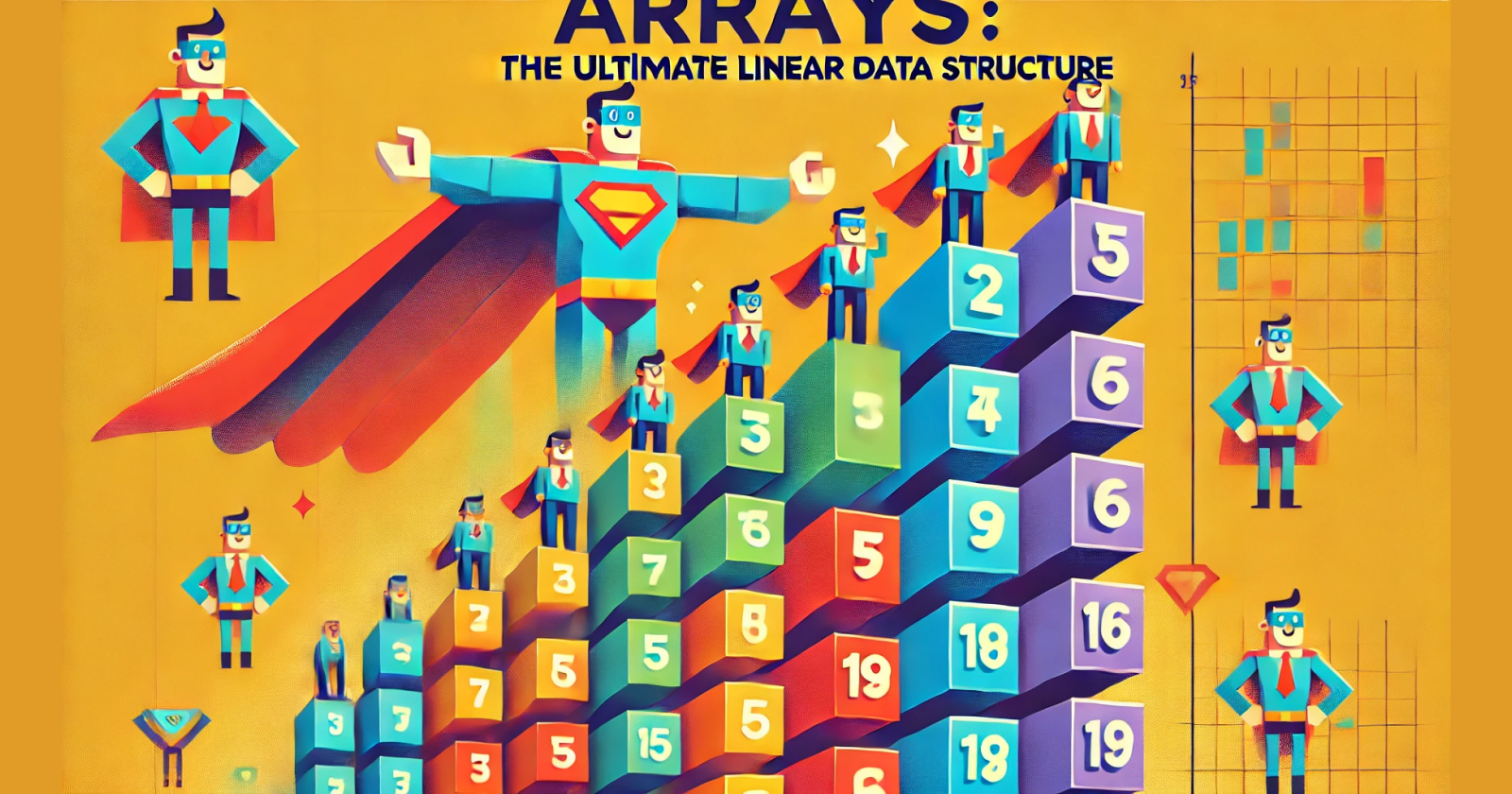
Arrays are the superheroes of the data structure world, providing a simple and efficient way to store elements in a linear format! Let’s dive into the fascinating world of arrays and see what makes them tick.
What Are Arrays?
At their core, arrays are just a collection of memory references, and guess what? They’re 0-indexed! That means the first element is accessed at index 0. In C++ and Java, you can create an array with the following syntax:
int a[] = {1, 2, 3};
Pretty straightforward, right? The variable a
holds the memory address of the first element (in this case, 1) stored at index 0. The next element is located at the address of the first element plus the size of the data type.
Accessing Elements
When you want to access an element in an array, you simply use the syntax a[i]
, where i
is the index. This expression translates to a specific memory address:
Address = Address of a + (i * (size of data type))
This is why arrays can only hold elements of a specific data type. When you access a[i]
, the program jumps directly to that calculated address, pulling out the value from the corresponding memory block.
For example:
Accessing the first element:
a[0]→Address of a + 0 × (size of data type) => starting index of arrayAccessing the third element:
a[2]→Address of a + 2 × (size of data type) => starting index of third element of array
Arrays in Python: A Whole New Game
Now, let’s talk about Python. Oh, how I love Python! Its flexibility and extensive library support make it a joy to work with. In Python, arrays are represented by lists, but they’re a bit different:
Mixed Data Types: Lists can store multiple data types in the same list.
Dynamic Sizing: The size of a list can change on the fly!
In Python, everything is an object. While data types like int
, float
, and bool
exist, they are all ultimately objects. This means that a list can store various object types seamlessly.
For even more insights into how memory works in Python, check out this blog: https://sailor.hashnode.dev/unlocking-the-magic-of-memory-in-python
The Magic of Dynamic Lists
One of the coolest features of Python lists is their ability to resize. When you append a new element, Python creates a new list with double the size of the old one, copying over all the elements and adding the new one. This process means that appending an element has a time complexity of O(n+1), where n is the size of the old list and 1 is for adding 1 new element
Want to Learn More?
If you're curious and want to dive deeper into the world of data structures and algorithms, check out my other blogs for more insights and fun examples!
Subscribe to my newsletter
Read articles from Sai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
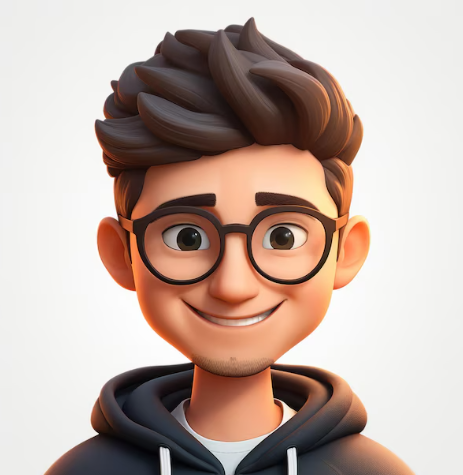
Sai
Sai
A graduate from a Tier 3 college, I transformed from someone who hated coding to a passionate enthusiast. Join me as I share my journey and insights into coding, DSA, and machine learning!