Understanding SQL Joins and Aggregate Functions

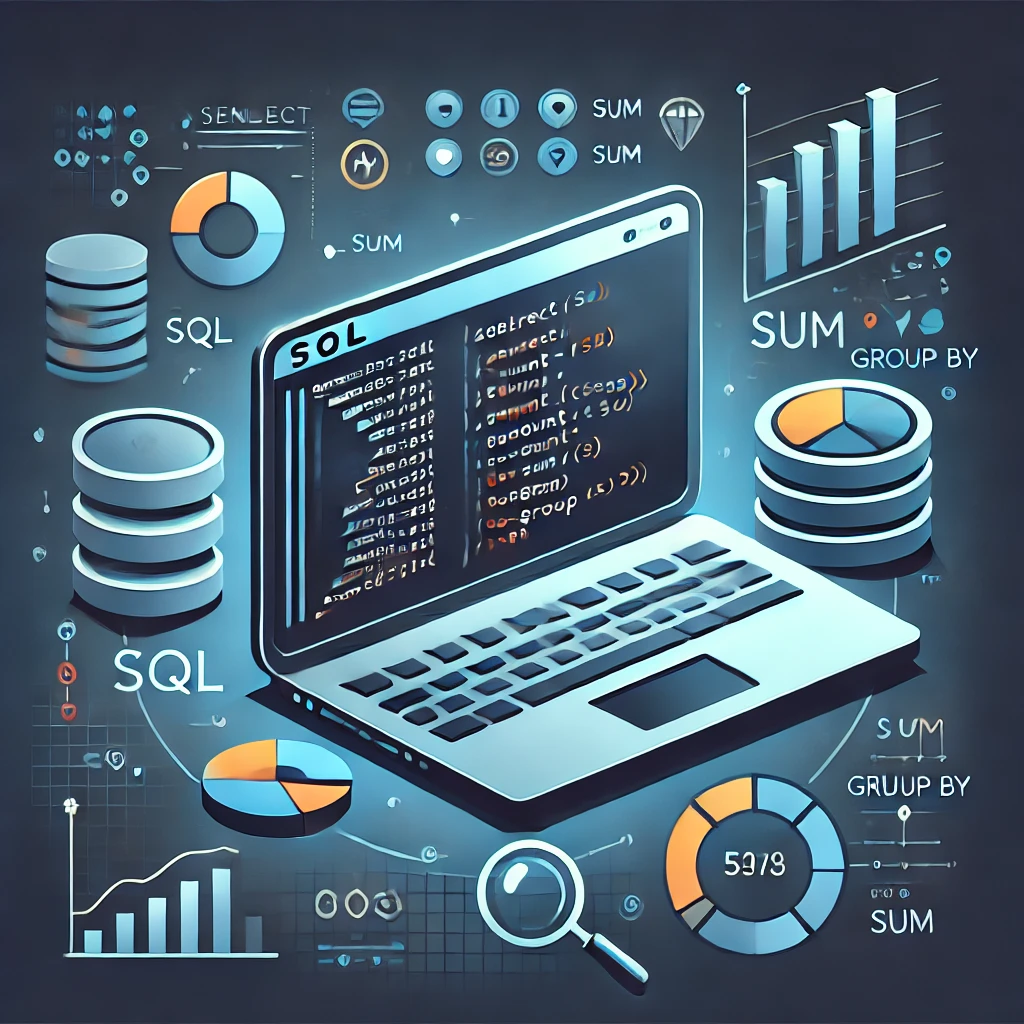
The Role of SQL:
Structured Query Language (SQL) is the backbone of any data-driven application. It allows us to interact with databases to retrieve, insert, update, and delete data. However, when dealing with relational databases, data is often spread across multiple tables. To retrieve meaningful insights, we need to combine these tables. That's where SQL joins and aggregate functions come in. In this blog, we will explore how SQL joins allow us to combine data from different tables, and how aggregate functions help in summarizing the data for analysis.
What are SQL Joins?
SQL joins are used to combine rows from two or more tables based on a related column between them. A typical relational database consists of multiple tables that store data in a structured format. Often, these tables are related to each other through a foreign key.
By using SQL joins, we can fetch data from multiple tables in a single query, enabling us to create more complex and meaningful reports. Without joins, we would have to run multiple queries and manually combine the results, which would be inefficient and prone to error.
Why are Joins Important?
Data Unification: In a normalized database, related data is often split across different tables to avoid redundancy. Joins allow us to reunite this data.
Efficient Queries: Instead of running multiple queries to gather data from different tables, joins enable us to retrieve all the relevant data in a single query.
Complex Reporting: Joins are essential for generating complex reports that require combining data from multiple sources, such as sales figures combined with customer information.
Types of SQL Joins
Will take the sales data as an example for the SQL Joins here
Let's assume we have two tables in our database:
Sales: Contains details of each sale made.
- Columns:
sale_id
,product_id
,quantity
,sale_date
Products: Contains information about the products sold.
- Columns:
product_id
,product_name
,price
Now, we'll explore how different types of joins work using these tables.
1. INNER JOIN
The INNER JOIN
returns only the rows where there is a match between the Sales
and Products
tables. If a product does not have any sales, or a sale references a non-existent product, it will not be included in the result.
Example: Get a list of all sales, showing the product name and the quantity sold.
SELECT Sales.sale_id, Products.product_name, Sales.quantity
FROM Sales
INNER JOIN Products
ON Sales.product_id = Products.product_id;
Result: This query will return only the sales where the product exists in both tables, showing the sale ID, product name, and the quantity sold.
2. LEFT JOIN (LEFT OUTER JOIN)
The LEFT JOIN
returns all records from the left table (Sales
), and the matched records from the right table (Products
). If a sale was made for a product that no longer exists in the products table, the result will still include that sale, but the product_name
will show as NULL
.
Example: Get a list of all sales, including those for products that might not exist in the Products
table anymore.
SELECT Sales.sale_id, Products.product_name, Sales.quantity
FROM Sales
LEFT JOIN Products
ON Sales.product_id = Products.product_id;
Result: This query will return all sales, even if a product is no longer in the Products
table. For such sales, the product name will be NULL
.
3. RIGHT JOIN (RIGHT OUTER JOIN)
The RIGHT JOIN
returns all records from the right table (Products
), and the matched records from the left table (Sales
). This is useful if you want to see all products, even those that haven’t had any sales yet.
Example: Get a list of all products and the quantity sold, if any.
SELECT Products.product_name, Sales.quantity
FROM Sales
RIGHT JOIN Products
ON Sales.product_id = Products.product_id;
4. FULL JOIN (FULL OUTER JOIN)
The FULL JOIN
returns all records when there is a match in either the Sales
or the Products
table. It combines the result of both LEFT JOIN
and RIGHT JOIN
.
Example: Get a list of all sales and products, including unmatched records from both tables.
SELECT Sales.sale_id, Products.product_name, Sales.quantity
FROM Sales
FULL OUTER JOIN Products
ON Sales.product_id = Products.product_id;
Result: This query will return all sales and all products. If there are products without sales, the sale details will be NULL
, and if there are sales for products that are no longer in the product list, the product name will be NULL
.
5. CROSS JOIN
The CROSS JOIN
returns the Cartesian product of the two tables, meaning it will pair each sale with every product in the Products
table. This is rarely used in real-world applications because it produces a large result set.
Example: Get a Cartesian product of sales and products
SELECT Sales.sale_id, Products.product_name
FROM Sales
CROSS JOIN Products;
Result: This query will return every possible combination of sales and products, which can be useful in specific scenarios, such as generating comparisons for analysis but is generally impractical for large datasets.
6. SELF JOIN
A Self Join is a type of join where a table is joined with itself. This is useful when we need to compare rows within the same table or find relationships within the same set of data. We essentially treat the table as two separate entities by giving it aliases.
SELECT s1.sale_id AS Current_Sale, s1.product_id AS Current_Product,
s2.sale_id AS Referred_Sale, s2.product_id AS Referred_Product
FROM Sales s1
LEFT JOIN Sales s2
ON s1.referred_sale_id = s2.sale_id;
s1
is the alias for the current sale.s2
is the alias for the referred sale (using thereferred_sale_id
to match it to thesale_id
of the previous sale).The
LEFT JOIN
ensures that we include sales that were not referred by any previous sale (those will haveNULL
for the referred sale).
Result:
Current_Sale | Current_Product | Referred_Sale | Referred_Product |
101 | 201 | 99 | 150 |
102 | 202 | NULL | NULL |
103 | 203 | 101 | 201 |
104 | 204 | NULL | NULL |
So here in this example:
Sale 101 was referred by Sale 99.
Sale 102 was not referred by any previous sale (so its
Referred_Sale
isNULL
).Sale 103 was referred by Sale 101.
Aggregate Functions in SQL:
Aggregate functions in SQL allow you to perform calculations on a set of values and return a single result. These functions are useful when we need to summarize or analyze large amounts of data, especially when we're dealing with grouped records. Aggregate functions work by taking multiple values from a column and returning a computed value based on that set, such as a sum, average, count, etc.
These functions are often used alongside the GROUP BY
clause to group rows that share a common field and apply the function to each group.
Common Aggregate Functions in SQL
COUNT() – Counts the number of rows or non-null values in a set.
SUM() – Adds up all values in a column.
AVG() – Calculates the average value in a column.
MAX() – Returns the maximum value in a column.
MIN() – Returns the minimum value in a column.
1. COUNT() – Counting the Number of Sales
The COUNT()
function is used to count the number of rows that match a specified condition or to count non-null values in a column.
Example:
Suppose we want to count the total number of sales in the Sales
table.
SELECT COUNT(*) AS total_sales
FROM Sales;
Result: This query returns the total number of sales, including all rows in the table.
2. SUM() – Calculating Total Sales Volume
The SUM()
function adds up all the values in a column. It’s especially useful when calculating totals, such as total revenue or total quantities sold.
Example:
To calculate the total quantity of products sold:
SELECT SUM(quantity) AS total_quantity_sold
FROM Sales;
Result: The query returns the total number of units sold across all sales.
3. AVG() – Calculating the Average Quantity Sold
The AVG()
function calculates the average of all the values in a column. This can help us determine trends, such as the average number of products sold per sale.
Example:
To find the average number of units sold in each sale:
SELECT AVG(quantity) AS avg_quantity_per_sale
FROM Sales;
Result: The query returns the average quantity sold per sale.
4. MAX() and MIN() – Finding the Maximum and Minimum Quantities Sold
The MAX()
function returns the highest value in a column, while MIN()
returns the lowest value. These are helpful when analyzing ranges, such as the highest or lowest quantities sold in a single sale.
Example:
To find the highest and lowest number of units sold in any sale:
SELECT MAX(quantity) AS max_quantity_sold, MIN(quantity) AS min_quantity_sold
FROM Sales;
Result: The query returns the maximum and minimum quantity sold in a single sale.
5. Using Aggregate Functions with GROUP BY
We can combine aggregate functions with the GROUP BY
clause to group rows that share a common attribute and apply the function to each group. For example, we can calculate total sales per product.
Example:
To calculate the total quantity sold for each product:
SELECT product_id, SUM(quantity) AS total_quantity_sold
FROM Sales
GROUP BY product_id;
This query groups the rows by product_id
, so it calculates the total quantity sold for each product.
Result: The output will show the total number of units sold for each product.
product_id | total_quantity_sold |
101 | 500 |
102 | 300 |
103 | 700 |
In conclusion, understanding SQL joins and aggregate functions is crucial for effective data analysis, enabling professionals to derive meaningful insights from complex datasets. I encourage all to apply these techniques to your own data to enhance your analytical capabilities and improve reporting accuracy.
If you found this blog informative, please consider sharing it within your network, and feel free to leave any questions or comments below; your engagement is greatly appreciated. Stay tuned for future posts. Thank you for reading!
Subscribe to my newsletter
Read articles from Sai Chandu Manchala directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sai Chandu Manchala
Sai Chandu Manchala
Driven software developer with a focus on building scalable applications and solving complex problems. I write to share my knowledge, experiences, and insights in software development.