The Ultimate Beginner's Guide To Python Frameworks

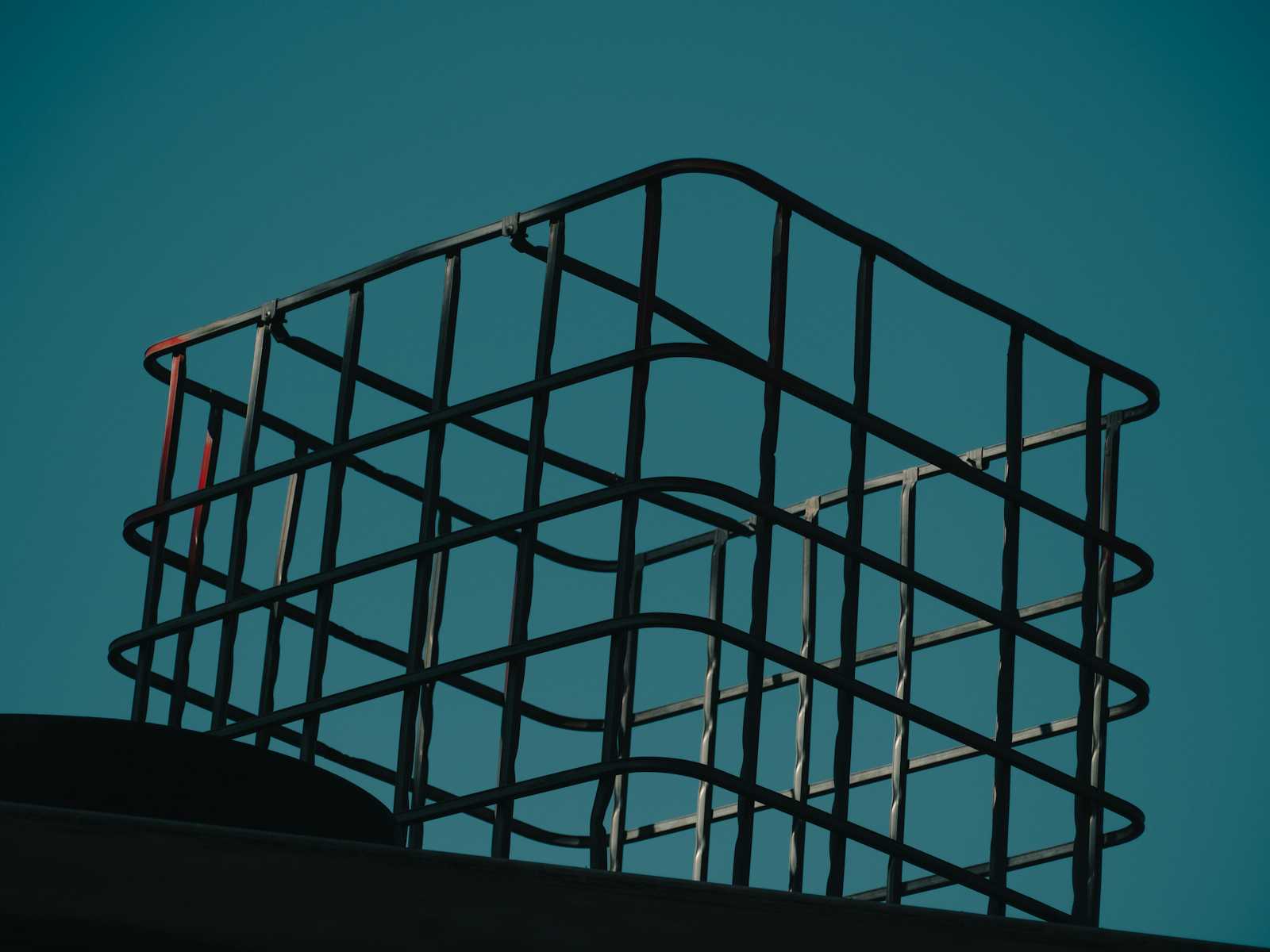
Before diving into frameworks, let us understand basic definitions of modules, packages, libraries, and frameworks in Python.
Modules are the basic building blocks or individual files containing Python code that define functions, classes, or variables
Packages are the collections of related modules organized in directories.
Libraries are collections of modules and packages.
Frameworks provide a structured approach using modules, packages, and libraries
Modules
math: A built-in module providing mathematical functions like sqrt, sin, cos, etc.
random: A built-in module for generating random numbers.
os: A built-in module for interacting with the operating system.
time: A built-in module for working with time-related operations.
Packages
requests: A package for making HTTP requests.
NumPy: A package for numerical computing.
pandas: A package for data analysis and manipulation.
Django: It is a web framework. It includes packages like django.contrib.auth for authentication. It also has django.contrib.admin for admin interfaces.
Libraries
NumPy: A library for numerical computing, combining modules and packages.
SciPy: A library for scientific and technical computing, built on top of NumPy.
Matplotlib: A library for making visualizations. It's often used with NumPy and SciPy.
TensorFlow: A library for machine learning, utilizing modules and packages for deep learning.
Frameworks
Django: A web framework for building complex web applications.
Flask: A lightweight web framework for smaller-scale applications.
FastAPI: A modern web framework for building APIs.
Keras: A high-level API for building and training neural networks, often used with TensorFlow.
The terms "library" and "package" are often interchangeable, especially for large or complex collections of modules. The key distinction lies in their purpose: libraries provide tools for a specific domain, while packages are general-purpose collections of modules.
Frameworks aim to simplify development. They provide guidelines for building software and handle some complex, repetitive tasks.
Example: Handling HTTP requests, most web apps must handle this type of request. So, developers use existing frameworks to facilitate this. They avoid writing from scratch or reusing the same code in different projects.
Types of Python Frameworks
1. Full-Stack Frameworks
These frameworks provide all you need to build a web app. They include tools for both the front end and back end.
Django: A popular choice with many built-in features for easy development.
TurboGears: Combines the best parts of different frameworks for quick app development.
2. Microframeworks
Microframeworks are lightweight and focus on simplicity. They provide only the essential tools, giving you more flexibility.
Flask: Easy to use and great for small to medium applications.
Bottle: Very simple and ideal for small projects or prototypes.
3. Asynchronous Frameworks
These frameworks handle many connections at once, making them perfect for real-time applications.
FastAPI: Fast and efficient for building APIs with support for asynchronous programming.
Tornado: It manages thousands of connections. It's often used in chat apps and real-time services.
Key Features of Python Frameworks
Routing:
It maps URLs to functions. This makes it easy to define how users access different parts of the app.
Example: Flask uses decorators to link URLs to specific functions.
Templating:
Separates HTML from Python code, allowing for dynamic content generation.
Example: Django uses a templating engine to render HTML with data from the backend.
Object-Relational Mapping (ORM):
Allows developers to interact with databases using Python objects instead of SQL queries.
Example: Django’s ORM simplifies database operations with a high-level API.
Middleware Support:
Processes requests globally, enabling functionalities like authentication and logging.
Example: Flask allows middleware functions to modify requests and responses.
Form Handling:
Manages user input with validation and error handling.
Example: Django provides tools for creating and validating forms easily.
Popular Python Frameworks
Django
Key Features: It uses MVT architecture, has an admin interface, and strong security.
Use Cases: Ideal for large applications like e-commerce sites and social networks.
Flask
Flexibility: A minimalistic framework that allows developers to add only what they need.
Simplicity: Great for small projects or APIs where quick development is important.
Use Cases: Best for lightweight applications or when more control is needed.
FastAPI
Strengths: Built for fast API development with automatic documentation generation.
Performance: Supports asynchronous programming for handling many requests at once.
Use Cases: Suitable for high-performance applications, like real-time data processing.
Real-world Applications of Python Frameworks
Django:
- Instagram: It uses Django for its scalability and features. They help it manage millions of users efficiently.
Flask:
- Pinterest: Chooses Flask for its simplicity. It allows rapid development and testing of new features.
FastAPI:
- Netflix utilizes FastAPI for building microservices that need high performance and quick responses.
- If you found this article helpful, drop a ❤️ below.
Subscribe to my newsletter
Read articles from Vijayendra Prasad directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vijayendra Prasad
Vijayendra Prasad
B.Tech graduate with a deep love for content creation.