Setup a pre-commit hook with EsLint and prettier with husky in Javascript/Typescript

Table of contents
- Prerequisites
- Step1 - Install Essential Dependencies
- Step2 - Initialize Husky for Git Hooks
- Step3 - Set Up a Pre-commit Hook with Husky
- Step 4: Configure lint-staged
- Step 5: Ensure Husky Hooks are Executable
- Step 6: Create ESLint and Prettier Configuration Files
- Step 7: Test Your Setup for Smooth Operation
- Conclusion: Maintain Code Quality with Automated Linting and Formatting
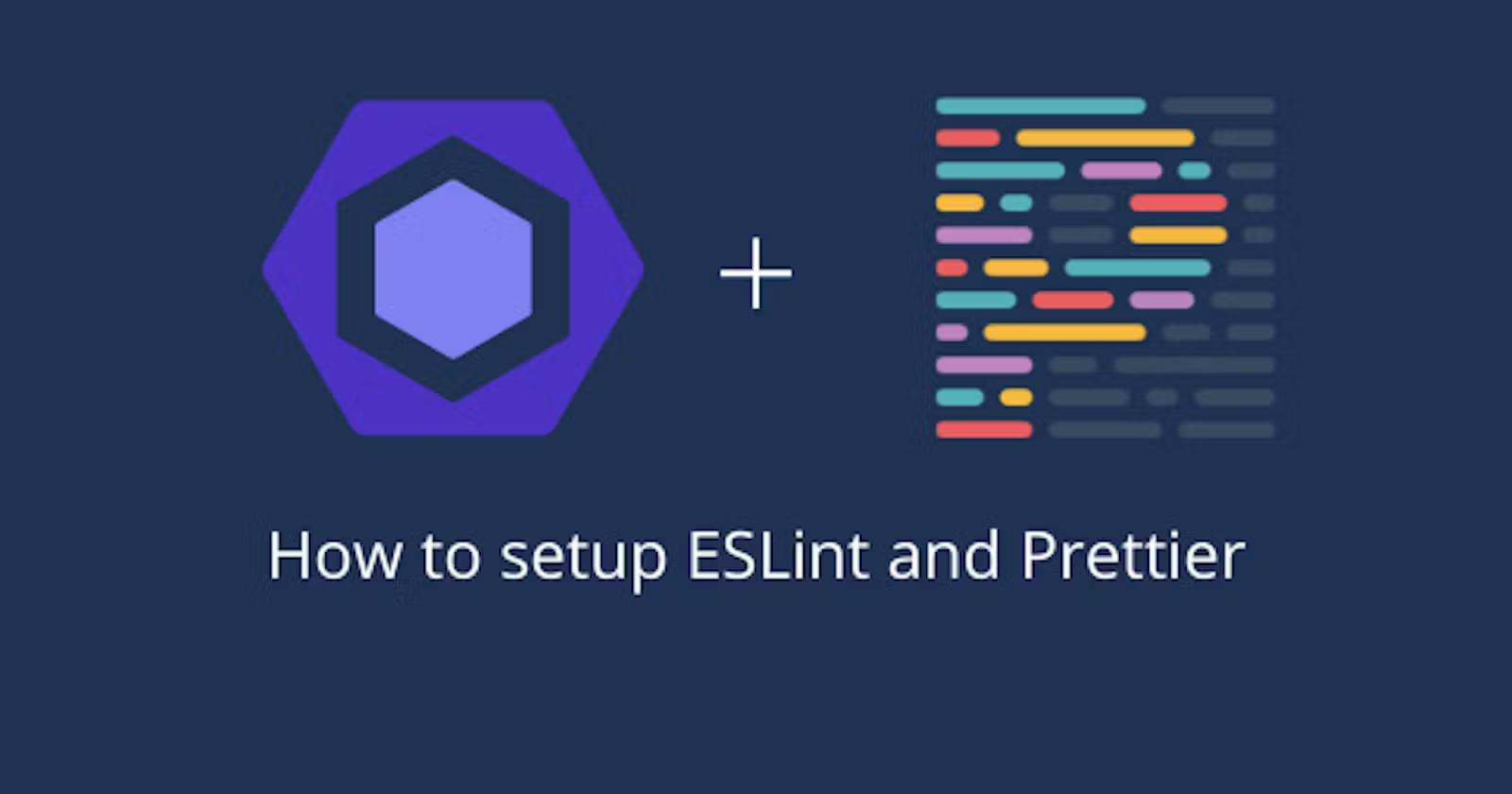
Eslint is a tool for identifying and fixing bugs in javascript code. When working in a team, it ensures that all team member adhere to set of rules or guidelines, catching errors before code is committed. For example, you can define rule for single quote
only, disallowing unused vars
, preferring ===
over ==
.
Prettier is a tool to make your code look pretty and maintains a consistent style. It formats your code to make it clean and readable. For example, it can add semicolons
, enforce single quotes
, and set tabWidth
.
Let’s setup ESLint and Prettier in your codebase with husky to automate code formating before each git commit.
Prerequisites
Make sure you have Node.js installed on your machine. If you haven’t already, you can download Node.js from nodejs.org
Step1 - Install Essential Dependencies
npm install --save-dev husky lint-staged prettier eslint eslint-config-prettier eslint-plugin-prettier babel-eslint @babel/eslint-parser
Step2 - Initialize Husky for Git Hooks
npx husky-init && npm install
This will setup Husky in your project and installs the required dependencies.
Step3 - Set Up a Pre-commit Hook with Husky
npx husky add .husky/pre-commit "npx lint-staged"
This will create .husky/pre-commit
file that will run lint-staged before each commit and verify its content and should match with.
#!/usr/bin/env sh
. "$(dirname -- "$0")/_/husky.sh"
npx lint-staged --allow-empty
Step 4: Configure lint-staged
In package.json, add below configuration
"scripts": {
"prepare": "husky && husky install",
"lint": "eslint src/**/*.{ts,tsx}"
},
"husky": {
"hooks": {
"pre-commit": "lint-staged"
}
},
"lint-staged": {
"src/**/*.{ts,tsx}": [
"prettier --write",
"eslint --fix"
]
},
So, once you do git commit then npx lint-stage
will trigger from .husky/pre-commit
configuration. Configuration under lint-staged will tell to run eslint and prettier for src folder files with extension .ts and .tsx only, other files and folder will get ignored.
Step 5: Ensure Husky Hooks are Executable
chmod +x .husky/pre-commit
chmod +x .husky/_/husky.sh
Step 6: Create ESLint and Prettier Configuration Files
.prettierrc.json
{
"semi": true,
"singleQuote": true,
"printWidth": 100,
"tabWidth": 2,
"useTabs": false
}
.eslintrc.cjs
module.exports = {
"env": {
"browser": true,
"es6": true,
"node": true,
},
"parserOptions": {
"sourceType": "module"
},
"ignorePatterns": [
"node_modules/*",
"dist/*"
],
"extends": [
'eslint:recommended',
'plugin:prettier/recommended',
"plugin:@typescript-eslint/recommended"
],
"plugins": ['prettier'],
"rules": {
'prettier/prettier': 'error',
"quotes": ['error', 'single'],
'no-unused-vars': 'off',
'@typescript-eslint/no-unused-vars': ['error']
}
}
Now run npm run prepare
command to create .husky/_/
husky.sh
file
Step 7: Test Your Setup for Smooth Operation
Now make some changes in file, do git add
and then commit
your changes, now Husky
and lint-staged
should automatically run Prettier
and ESLint
to format and lint your code before the commit is finalised. Alternatively, you can run npm run lint
to see the lint issue.
Conclusion: Maintain Code Quality with Automated Linting and Formatting
By integrating ESLint and Prettier with Husky in your JavaScript or TypeScript project, you ensure that your codebase remains clean, consistent, and error-free. This setup automates the process of code formatting and linting before each commit, enhancing team collaboration and maintaining code quality. With these tools in place, you can focus more on writing great code and less on manual code reviews and formatting issues.
Subscribe to my newsletter
Read articles from Parveen Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Parveen Kumar
Parveen Kumar
I am a Full-stack MERN engineer with a product centric approach, specializing in the design and development of scalable, reliable, production ready web application, I bring expertise in JavaScript, React, and Node Js. Get in touch! paulparveen01@gmail.com