How to Fetch and Display Random User Data Using JavaScript: A Beginner's Guide

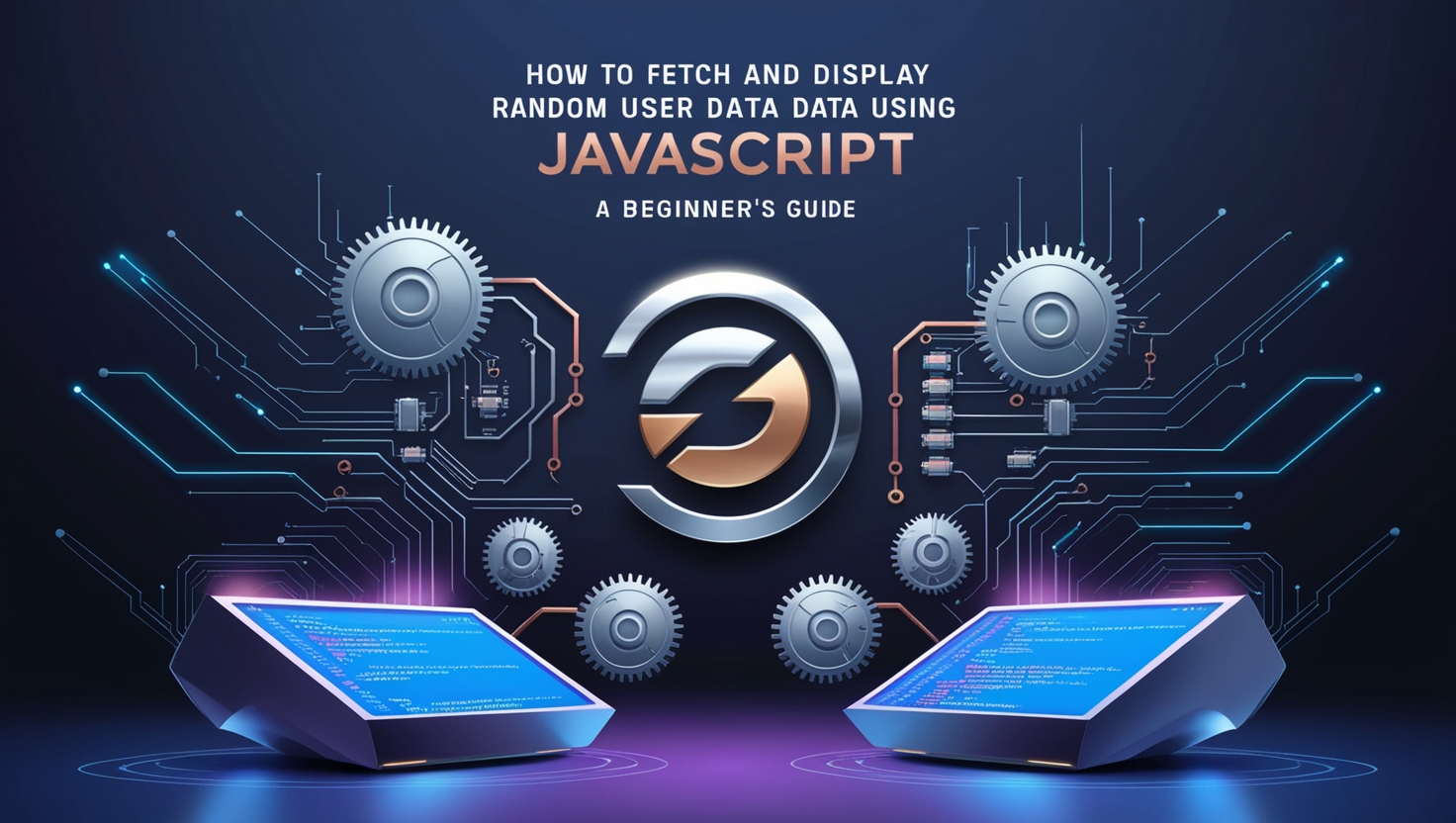
Introduction
In this tutorial, we'll build a simple Random User Data Generator using JavaScript. This project will teach you how to:
Fetch data from an external API using
fetch()
Display the fetched data on a webpage dynamically
Implement error handling to gracefully manage any issues that arise when making API requests.
We'll use the randomuser.me
API to retrieve random user data such as names, emails, and profile pictures.
Table of Contents:
Setting Up the Project
Fetching Data from an API
Displaying API Data in the DOM
Handling Errors Gracefully
Testing the Error Handling
Conclusion
Setting Up the Project
Step 1: Create the following files:
index.html
style.css
index.js
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Random User Generator</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div class="dataContainer">
<h1>Random Data Generator</h1>
<button id="genButton">Generate Fake Data</button>
<div id="apiData"></div>
</div>
<script src="app.js"></script>
</body>
</html>
.dataContainer{
height: 20vh;
display: grid;
align-items: center;
justify-content: center;
}
#genButton{
border: 0;
outline: 0;
background: #019f55;
color: #fff;
font-size: 22px;
font-weight: 300;
display: flex;
align-items:center;
justify-content: center;
padding: 16px 26px;
border-radius: 5px;
cursor: pointer;
}
.dataContainer h1{
color: #fff;
}
#apiData{
color: #fff;
}
Fetching Data from an API
We'll use the fetch
API to get random user data. The randomuser.me
API is free and returns random user details in JSON format
let genButton = document.getElementById("genButton");
async function getFakeData() {
try {
const resp = await fetch("https://randomuser.me/api/");
const data = await resp.json();
const results = data.results[0];
const fullName = `${results.name.title} ${results.name.first} ${results.name.last}`;
const age = results.dob.age;
const email = results.email;
const picture = results.picture.large;
let dataContainer = document.getElementById("apiData");
dataContainer.innerHTML = `
<p><strong>Name:</strong> ${fullName}</p>
<p><strong>Age:</strong> ${age} years</p>
<p><strong>Email:</strong> ${email}</p>
<img src="${picture}" alt="User Picture" />
`;
} catch (error) {
let dataContainerError = document.getElementById("apiData");
dataContainerError.innerHTML=`<p style="color:red;">An error occurred : Try Agian !</p>`;
}
}
genButton.addEventListener("click", getFakeData);
Explanation:
We attach an event listener to the "Generate Fake Data" button.
The
getFakeData()
function is responsible for making thefetch
request.If the response is successful, we extract the user's name, email, and profile picture and insert it into the DOM.
In case of any error, we log it to the console for debugging purposes
Testing the Error Handling
You can test the catch
block in different ways:
Simulate a network error by going offline in your browser’s DevTools (Chrome: Network tab → Offline).
Change the API URL to an incorrect one like
https://randomuser.me/api-invalid/
to force a 404 error.Manually throw an error in the
try
block to verify how the error handling works.
Conclusion
In this project, we successfully built a random user data generator that:
Fetches data from an external API using
fetch()
Dynamically displays the data on a webpage
Handles errors gracefully by notifying the user when something goes wrong
This simple yet powerful example helps you understand how to work with APIs in JavaScript and improve the robustness of your applications by handling errors effectively. You can extend this project by fetching more data or styling the output to look better.
End Result
sample work : https://rakesh709.github.io/most-weird-website/
Thank you for reading 😊
Don't copy and paste; try it on your own
Rakesh Kumar
https://www.linkedin.com/in/rakesh-kumar07/
Subscribe to my newsletter
Read articles from Rakesh kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
