25 Essential Coding Questions to Build a Strong Foundation (With Explanations)
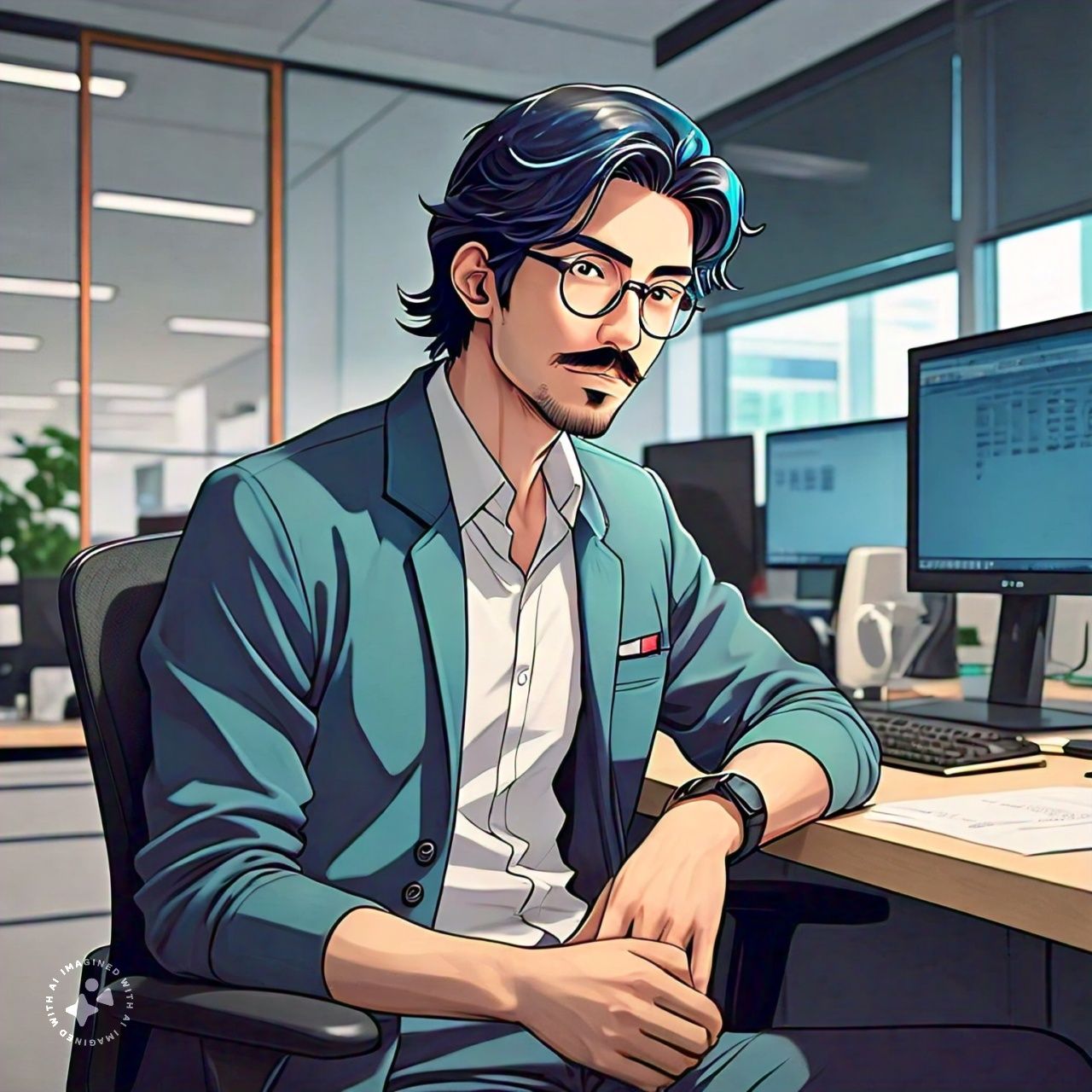
Learning a new programming language can be a challenging yet rewarding experience. To build a strong foundation, practicing key coding problems is essential. This article presents 25 essential coding questions, divided into five categories: Numbers, Recursion, Arrays, Strings, and Matrices. Working through these will strengthen your understanding and help prepare you for coding interviews and more advanced topics.
Table of Contents
Numbers
Recursion
Arrays
Strings
Matrices
Numbers: Coding Problems and Solutions
Definition: Numbers are fundamental data types in programming used for arithmetic operations and comparisons. They can be integers, floating-point, or complex numbers, and are key to solving a wide range of mathematical and logical problems.
Check if a Number is Positive
Explanation: This function checks whether a number is positive. This is a basic coding task that introduces conditional logic.
def is_positive(number):
return number > 0
print(is_positive(10)) # True
print(is_positive(-5)) # False
Sum of first N natural Numbers
Explanation: This Problem help you practice basic arithmetic. It calculates the sum of first N natural numbers using the formula:
n * (n + 1) // 2.
def sum_natural(n):
total = 0
for i in range(1, n + 1):
total += i
return total
print(sum_natural(5)) # 15
print(sum_natural(10)) # 55
Reverse a Number
Explanation: This function takes an integer and reverses its digits. This introduces you to string manipulation and type conversion.
def reverse_number(number):
return int(str(number)[::-1])
print(reverse_number(12345)) # 54321
Factorial of a Number
Explanation: This computes the factorial of a number, a foundational concept in mathematics and combinatorics.
def factorial(number):
if number == 0:
return 1
return number * factorial(number - 1)
print(factorial(5)) # 120
Check if a Number is Armstrong
Explanation: This checks if a number is an Armstrong number (where the sum of its digits raised to the power of the number of digits equals the number itself).
def is_armstrong(number):
num_str = str(number)
power = len(num_str)
return sum(int(digit) ** power for digit in num_str) == number
print(is_armstrong(153)) # True
print(is_armstrong(123)) # False
Recursion: Common Coding Challenges
Definition: Recursion is a programming technique where a function calls itself to break down complex problems into smaller, manageable subproblems. It's often used in algorithms like searching, sorting, and tree traversal.
HCF of Two Numbers
Explanation: This function uses recursion to find the highest common factor (HCF) of two numbers using the Euclidean algorithm.
pythonCopy codedef hcf(a, b):
if b == 0:
return a
return hcf(b, a % b)
print(hcf(54, 24)) # 6
Factorial Using Recursion
Explanation: The same factorial problem can be solved using recursive function calls.
pythonCopy codedef factorial_recursive(number):
if number == 0:
return 1
return number * factorial_recursive(number - 1)
print(factorial_recursive(5)) # 120
Reverse a Number Using Recursion
Explanation: This function recursively reverses the digits of a number.
pythonCopy codedef reverse_number_recursive(number, reversed_number=0):
if number == 0:
return reversed_number
return reverse_number_recursive(number // 10, reversed_number * 10 + number % 10)
print(reverse_number_recursive(12345)) # 54321
Length of a String Using Recursion
Explanation: This recursive function calculates the length of a string.
pythonCopy codedef length_of_string(s):
if not s:
return 0
return 1 + length_of_string(s[1:])
print(length_of_string("hello")) # 5
Print All Permutations of a String
Explanation: This recursive function generates all permutations of a given string.
pythonCopy codedef permutations(s, chosen=""):
if not s:
print(chosen)
return
for i in range(len(s)):
permutations(s[:i] + s[i+1:], chosen + s[i])
permutations("abc")
Arrays: Key Problems to Master
Definition: Arrays are data structures that store multiple elements of the same type in a contiguous memory location. They allow efficient data access and manipulation, making them essential for sorting, searching, and dynamic programming.
Sum of Elements in the Array
Explanation: This function calculates the sum of elements in an array.
pythonCopy codedef sum_of_array(arr):
return sum(arr)
print(sum_of_array([1, 2, 3, 4, 5])) # 15
Find the Smallest Element in the Array
Explanation: This function finds the smallest element in an array using the min() function.
pythonCopy codedef smallest_element(arr):
return min(arr)
print(smallest_element([3, 1, 4, 1, 5, 9])) # 1
Reverse an Array
Explanation: This function reverses the array using slicing.
pythonCopy codedef reverse_array(arr):
return arr[::-1]
print(reverse_array([1, 2, 3, 4, 5])) # [5, 4, 3, 2, 1]
Check if the Array is Palindrome
Explanation: This function checks if an array is a palindrome (same forwards and backwards).
pythonCopy codedef is_palindrome(arr):
return arr == arr[::-1]
print(is_palindrome([1, 2, 3, 2, 1])) # True
Find Repeating Elements in an Array
Explanation: This function identifies the elements that appear more than once in the array.
pythonCopy codedef find_repeating_elements(arr):
counts = {}
for element in arr:
counts[element] = counts.get(element, 0) + 1
return [element for element, count in counts.items() if count > 1]
print(find_repeating_elements([1, 2, 3, 2, 1])) # [1, 2]
Strings: Essential Coding Questions
Definition: Strings are sequences of characters used to store and manipulate text. They are crucial for tasks like parsing, searching, and text processing, and offer a variety of methods for transformations and analysis.
Count the Number of Vowels in a String
Explanation: This function counts the vowels in a string, both uppercase and lowercase.
pythonCopy codedef count_vowels(s):
vowels = 'aeiouAEIOU'
return sum(1 for char in s if char in vowels)
print(count_vowels("hello world")) # 3
Remove All Spaces from a String
Explanation: This function removes all spaces from the string using the replace() method.
pythonCopy codedef remove_spaces(s):
return s.replace(" ", "")
print(remove_spaces("hello world")) # "helloworld"
Frequency of Characters in a String
Explanation: This function counts the frequency of each character in the string.
pythonCopy codedef frequency_of_characters(s):
frequency = {}
for char in s:
frequency[char] = frequency.get(char, 0) + 1
return frequency
print(frequency_of_characters("hello world")) # {'h': 1, 'e': 1, 'l': 3, 'o': 2, 'w': 1, 'r': 1, 'd': 1}
Replace a Substring in a String
Explanation: This function replaces a substring within the string using replace().
pythonCopy codedef replace_substring(s, old, new):
return s.replace(old, new)
print(replace_substring("hello world", "world", "there")) # "hello there"
Check if Two Strings are Anagrams
Explanation: This function checks if two strings are anagrams (rearrangements of the same characters).
pythonCopy codedef is_anagram(s1, s2):
return sorted(s1) == sorted(s2)
print(is_anagram("listen", "silent")) # True
Matrix: Key Programming Challenges
In this section, we focus on matrix-related problems, which are essential for handling two-dimensional arrays. Matrices are widely used in various domains such as image processing, graph algorithms, and scientific computations.
Search for an Element in a Matrix
Explanation: This function searches for a target element within a matrix. It iterates through each row to see if the target exists.
pythonCopy codedef search_matrix(matrix, target):
for row in matrix:
if target in row:
return True
return False
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
print(search_matrix(matrix, 5)) # True
print(search_matrix(matrix, 10)) # False
Print Matrix Elements in Sorted Order
Explanation: This function flattens a matrix and sorts its elements in ascending order. It’s useful for data normalization or sorting operations.
pythonCopy codedef sort_matrix(matrix):
flattened = [item for row in matrix for item in row]
return sorted(flattened)
matrix = [
[9, 8, 7],
[6, 5, 4],
[3, 2, 1]
]
print(sort_matrix(matrix)) # [1, 2, 3, 4, 5, 6, 7, 8, 9]
Rotate Matrix by 90 Degrees
Explanation: This function rotates the matrix 90 degrees clockwise using list comprehension and Python’s built-in
zip()
function. This is common in image rotations or geometric transformations.
pythonCopy codedef rotate_matrix(matrix):
return [list(row) for row in zip(*matrix[::-1])]
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
print(rotate_matrix(matrix)) # [[7, 4, 1], [8, 5, 2], [9, 6, 3]]
Find the Nth Smallest Element in a Row-Column Wise Sorted Matrix
Explanation: This function finds the nth smallest element in a row-column sorted matrix. This type of matrix is sorted both row-wise and column-wise. The solution uses a min-heap to efficiently extract the smallest elements.
pythonCopy codeimport heapq
def nth_smallest(matrix, n):
min_heap = []
for row in matrix:
for element in row:
heapq.heappush(min_heap, element)
return heapq.nsmallest(n, min_heap)[-1]
matrix = [
[1, 5, 9],
[10, 11, 13],
[12, 13, 15]
]
print(nth_smallest(matrix, 5)) # 11
Spiral Traversal of a Matrix
Explanation: This function prints the matrix in a spiral order, which is a common problem in matrix manipulation. It starts from the top-left corner and moves around the matrix in a spiral, printing elements.
pythonCopy codedef spiral_traversal(matrix):
result = []
while matrix:
result += matrix.pop(0) # Take the first row
if matrix and matrix[0]: # Take the last column
for row in matrix:
result.append(row.pop())
if matrix: # Take the last row in reverse
result += matrix.pop()[::-1]
if matrix and matrix[0]: # Take the first column in reverse
for row in matrix[::-1]:
result.append(row.pop(0))
return result
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
print(spiral_traversal(matrix)) # [1, 2, 3, 6, 9, 8, 7, 4, 5]
Conclusion
These 25 coding questions are essential for building a strong programming foundation. By solving these, you'll improve your ability to tackle more advanced coding challenges, preparing you for coding interviews and real-world problem-solving. Practice regularly, and soon you'll master these essential programming concepts.
Subscribe to my newsletter
Read articles from Mayank Tomar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
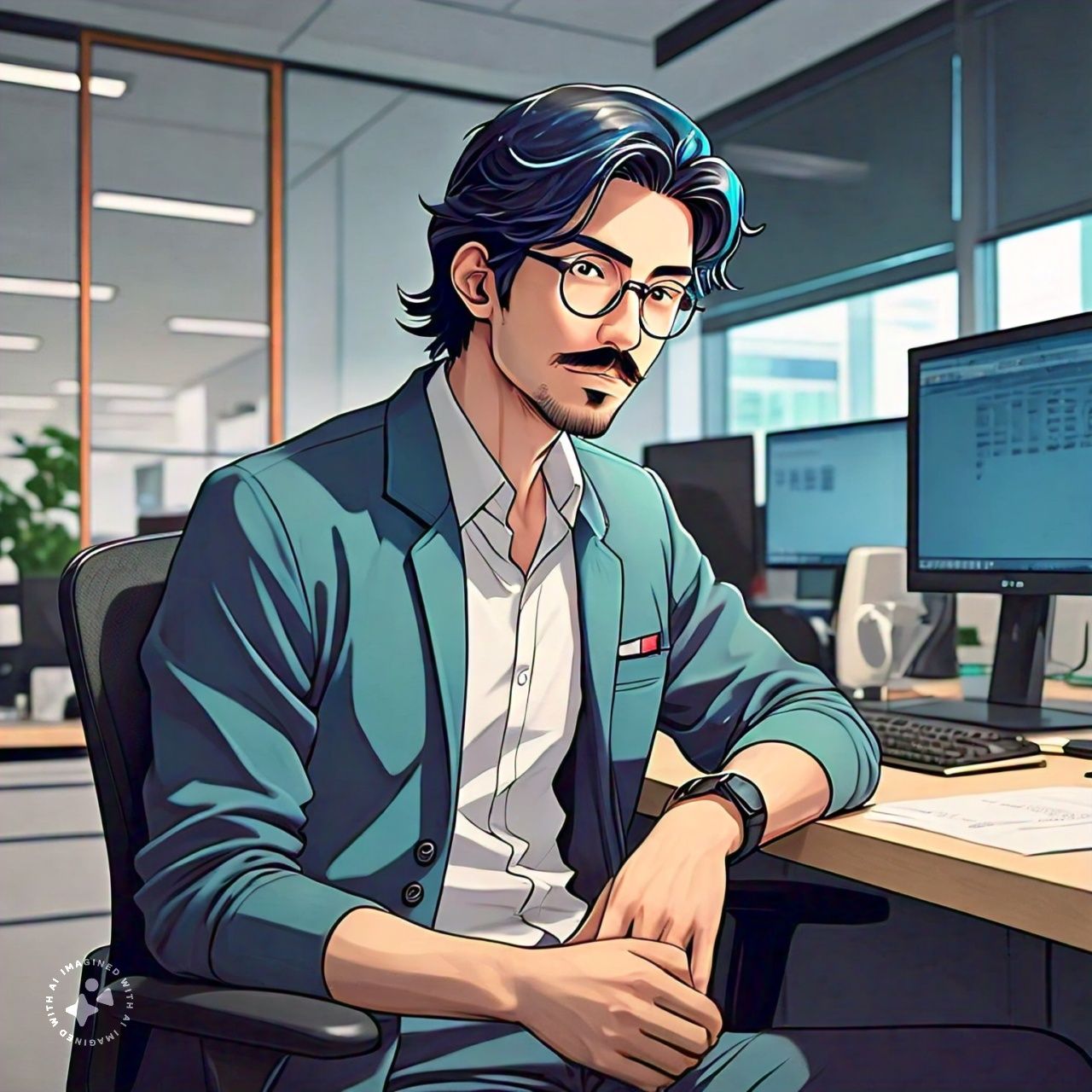
Mayank Tomar
Mayank Tomar
Hey! I am a software engineering student, Building the way to learn coding easily.