Annotations in Java
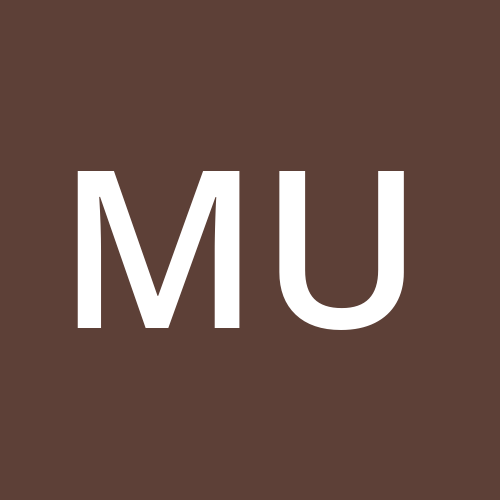
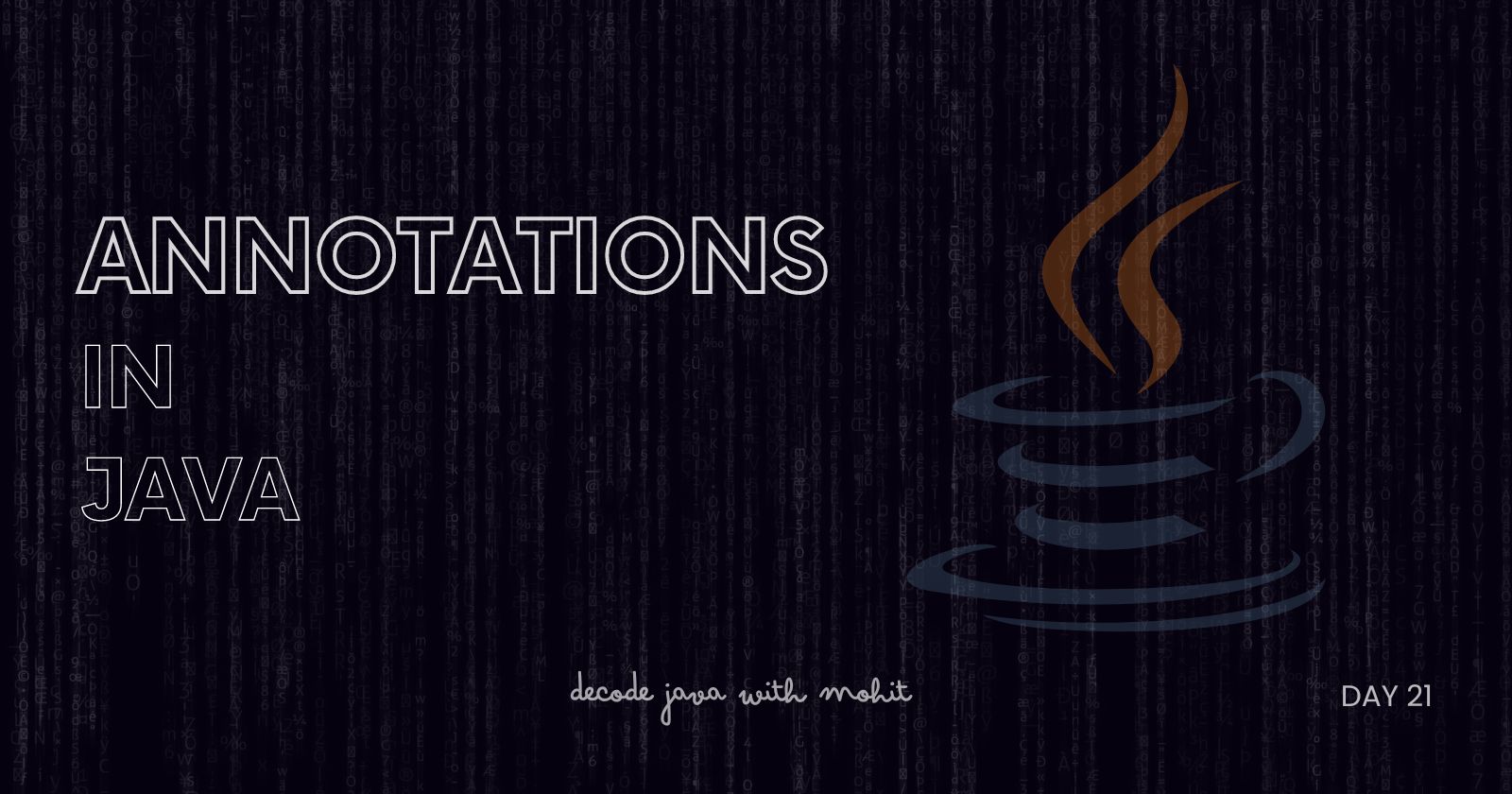
On Day 21, the focus is on Annotations in Java. Annotations are a form of metadata that provide data about a program but do not have direct influence on the program's logic. They are widely used to provide additional information for compilers, development tools, and frameworks, enhancing the behavior or handling of specific elements in Java code.
In this article, we will cover built-in annotations, creating custom annotations, and discuss common use cases and best practices for working with annotations.
1. What are Annotations?
Annotations are a special type of syntactic metadata that can be added to Java code. They are prefixed by the @
symbol and can be applied to:
Classes
Methods
Variables
Parameters
Constructors
Annotations can have elements (key-value pairs) and do not affect the program execution directly but can be used by other tools or frameworks to process the code.
2. Built-in Annotations in Java
Java comes with several built-in annotations that are frequently used for various purposes, including overriding methods, suppressing warnings, and indicating deprecated code. Here are some of the most common ones:
A. @Override
The @Override
annotation indicates that a method overrides a method in its superclass. This helps developers catch errors, such as mis-typed method names or incorrect method signatures.
class Parent {
void show() {
System.out.println("Parent show()");
}
}
class Child extends Parent {
@Override
void show() {
System.out.println("Child show()");
}
}
B. @Deprecated
The @Deprecated
annotation marks a method, class, or field as deprecated, signaling that it should no longer be used because it might be removed in future versions. Tools and IDEs will issue warnings when using deprecated elements.
@Deprecated
void oldMethod() {
System.out.println("This method is deprecated.");
}
C. @SuppressWarnings
The @SuppressWarnings
annotation tells the compiler to suppress specific warnings that it would otherwise issue. For example, warnings related to unchecked operations or unused variables can be suppressed.
@SuppressWarnings("unchecked")
void myMethod() {
List myList = new ArrayList(); // unchecked warning suppressed
}
D. @FunctionalInterface
This annotation indicates that an interface is a Functional Interface, which means it has only one abstract method. It is used to facilitate the use of lambda expressions.
@FunctionalInterface
interface MyFunction {
void execute();
}
3. Creating Custom Annotations
You can create custom annotations in Java to provide additional metadata for your programs or frameworks. Custom annotations can have their own elements (parameters) that act like key-value pairs and are defined using the @interface
keyword.
A. Defining a Custom Annotation
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
// Define a custom annotation
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
public @interface MyCustomAnnotation {
String author();
String date();
int version() default 1;
}
B. Using Custom Annotations
public class MyClass {
@MyCustomAnnotation(author = "John Doe", date = "2024-09-13", version = 2)
public void myMethod() {
System.out.println("This is a custom annotated method.");
}
}
@Target
: Specifies where the annotation can be applied (e.g., methods, fields, classes).@Retention
: Specifies when the annotation is available (e.g., source, class, runtime). If marked asRetentionPolicy.RUNTIME
, the annotation is available at runtime and can be accessed via reflection.
C. Accessing Annotations via Reflection
Annotations can be accessed at runtime using Java's reflection API:
import java.lang.reflect.Method;
public class AnnotationProcessor {
public static void main(String[] args) throws Exception {
Method method = MyClass.class.getMethod("myMethod");
if (method.isAnnotationPresent(MyCustomAnnotation.class)) {
MyCustomAnnotation annotation = method.getAnnotation(MyCustomAnnotation.class);
System.out.println("Author: " + annotation.author());
System.out.println("Date: " + annotation.date());
System.out.println("Version: " + annotation.version());
}
}
}
4. Use Cases of Annotations
Annotations are widely used in Java for various purposes, including:
A. Frameworks (Spring, Hibernate)
Many frameworks like Spring and Hibernate heavily rely on annotations to inject dependencies, configure components, map classes to databases, and more.
For example:
@Autowired
: Used in Spring for dependency injection.@Entity
: Used in Hibernate to mark a class as an entity mapped to a database table.
@Entity
class User {
@Id
private int id;
private String name;
// Getters and setters
}
B. Unit Testing (JUnit)
Annotations are extensively used in testing frameworks such as JUnit to mark test methods and setup/teardown methods.
@Test
: Marks a method as a test case.@BeforeEach
: Marks a method to be executed before each test case.
public class MyTest {
@BeforeEach
public void setup() {
System.out.println("Setting up before test.");
}
@Test
public void testMethod() {
System.out.println("Test executed.");
}
}
C. Documentation
Java provides the @Documented
annotation, which is used to include annotations in the generated documentation (JavaDocs). It's a helpful way to make sure that the presence of an annotation is visible to users when they read the documentation.
5. Best Practices for Using Annotations
To use annotations effectively, consider the following best practices:
A. Use Built-in Annotations Whenever Possible
Before creating custom annotations, check if Java already provides one that fits your needs. Using standard annotations increases the readability and maintainability of your code.
B. Keep Annotations Simple
Annotations should be as simple as possible. Avoid complex logic inside annotations, as their primary purpose is to provide metadata, not perform complex operations.
C. Use Annotations Consistently
When you create custom annotations, ensure they are used consistently throughout your codebase. This improves code organization and reduces confusion.
D. Combine Annotations with Reflection Judiciously
Annotations can be powerful when combined with reflection, but reflection is slow compared to other operations. Use it judiciously, particularly in performance-critical areas.
E. Document Custom Annotations
When creating custom annotations, provide clear documentation explaining their purpose, use cases, and how they should be applied. This ensures that other developers understand how and when to use them.
Summary
Annotations are a powerful feature in Java that enable developers to provide additional metadata to the code, which can then be used by compilers, tools, and frameworks. From the built-in annotations such as @Override
and @Deprecated
to creating custom annotations for specialized use cases, annotations make the code more expressive and easier to integrate with frameworks and libraries. Understanding how to use annotations effectively will help you write more modular, readable, and maintainable code in Java. Stay tuned! for further updates.
Subscribe to my newsletter
Read articles from Mohit Upadhyay directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
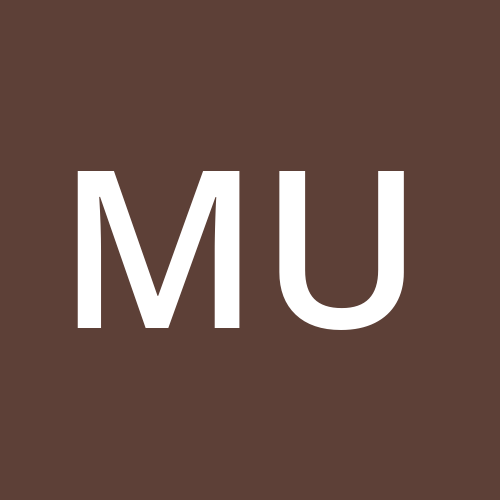