Data Structure Again

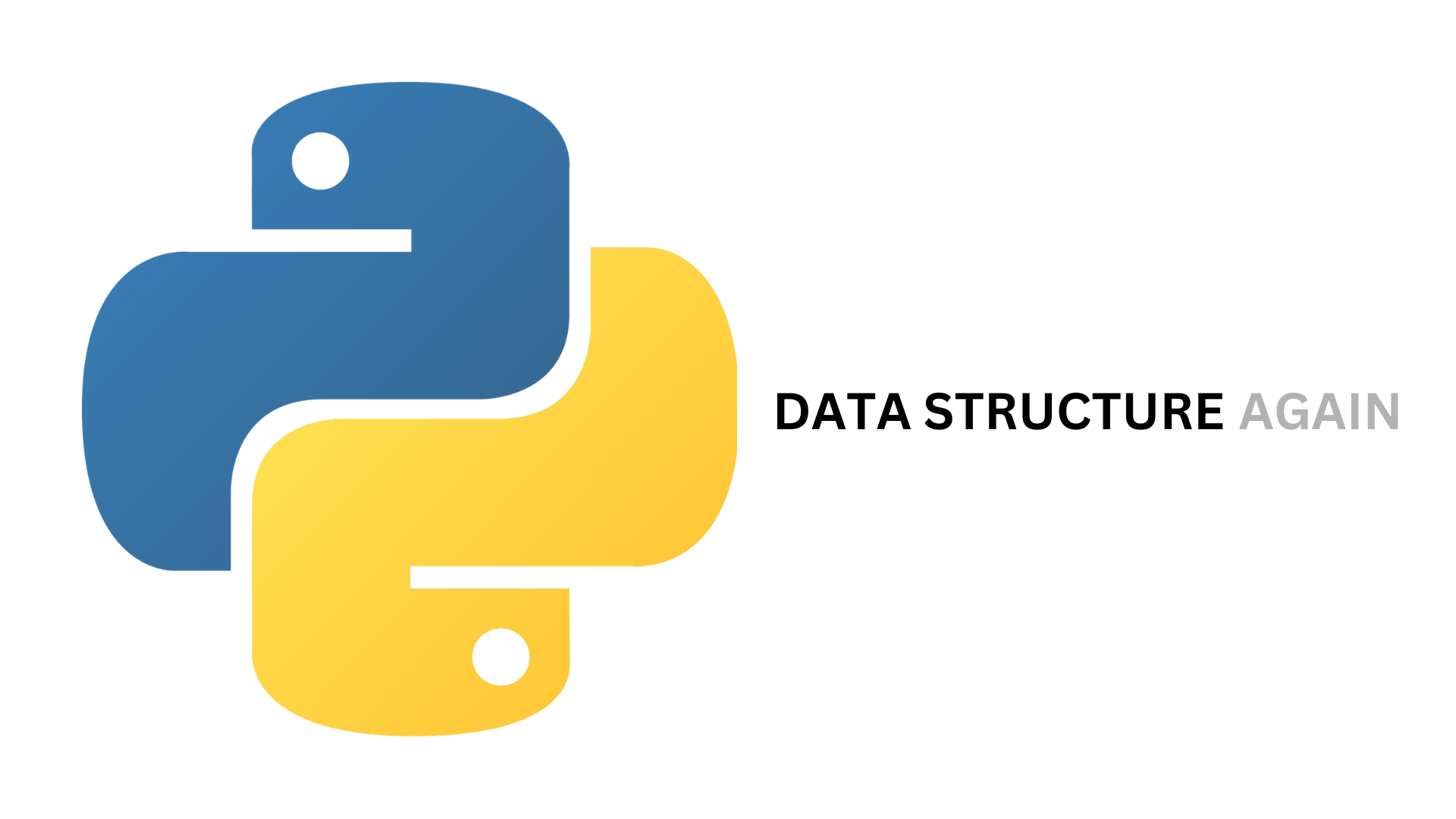
Again
A data structure in programming is a specialized format for organizing, processing, and storing data. Data structures enable efficient data management and retrieval, impacting performance and scalability.
My Research about Data Structure on Hashnode:
https://walterific.hashnode.dev/research-definitionprogramming-and-data-structures
Learning Targets:
Define and Identify List, Object, and List of Objects
List - a list is a fundamental data structure that represents an ordered collection of elements. Lists can store multiple items in a single variable, allowing for efficient data management and manipulation.
employer_list = ['Inductivo, Walter Mark', 'Idanan, Rodney', 'Cano, Roerenz', 'Toribio, Fiercival', 'Taay, James Kent']
The elements in employer_list
maintain their order. For example, 'Inductivo, Walter Mark' is at index 0, 'Idanan, Rodney' is at index 1, and so on.
You can change the contents of employer_list
after its creation. You can add, remove, or modify elements.
Although in this example all elements are strings, lists can contain mixed data types (e.g., numbers and strings together).
Object - An object allows for the storage of key-value pairs, where each piece of information (such as an employee's name, department, etc.) is linked to a specific key.
Here are individual employee objects:
employee_1 = {
"name": "Walter Mark Inductivo",
"department": "IT",
"id": 1,
"email": "walter@gmail.com",
"age": 22
}
employee_2 = {
"name": "Rodney Idanan",
"department": "IT",
"id": 2,
"email": "rodney@gmail.com",
"age": 22
}
employee_3 = {
"name": "Roerenz Cano",
"department": "IT",
"id": 3,
"email": "roerenz@gmail.com",
"age": 21
}
employee_4 = {
"name": "Fiercival Toribio",
"department": "IT",
"id": 4,
"email": "fiercival@gmail.com",
"age": 21
}
employee_5 = {
"name": "James Kent Taay",
"department": "IT",
"id": 5,
"email": "james@gmail.com",
"age": 21
}
Objects/Dictionaries/HashMaps are ideal for holding related information about a single entity, such as an employee's details. You can access data using the key, for instance, employee_1["name"]
retrieves the employee's name.
List of Objects - which allows you to group multiple related objects into a single collection. This is particularly useful for managing data that shares common characteristics, such as a list of employees or students. Each object in the list can be a dictionary, a custom object, or any other data structure.
Here’s an example demonstrating how to create a list of employee objects using dictionaries:
employees = [
{
"name": "Walter Mark Inductivo",
"department": "IT",
"id": 1,
"email": "walter@gmail.com",
"age": 22
},
{
"name": "Rodney Idanan",
"department": "IT",
"id": 2,
"email": "rodney@gmail.com",
"age": 22
},
{
"name": "Roerenz Cano",
"department": "IT",
"id": 3,
"email": "roerenz@gmail.com",
"age": 21
},
{
"name": "Fiercival Toribio",
"department": "IT",
"id": 4,
"email": "fiercival@gmail.com",
"age": 21
},
{
"name": "James Kent Taay",
"department": "IT",
"id": 5,
"email": "james@gmail.com",
"age": 21
}
]
Apply the concepts of list, object, and list of objects
1. Product Table
ID | Name | Category | Price | Stock | Supplier Email |
1 | Laptop | Electronics | 750 | 50 | supplier1@gmail.com |
2 | Desk Chair | Furniture | 100 | 200 | supplier2@gmail.com |
3 | Smartwatch | Electronics | 200 | 150 | supplier3@gmail.com |
4 | Notebook | Stationery | 5 | 500 | supplier4@gmail.com |
5 | Running Shoes | Apparel | 80 | 100 | supplier5@gmail.com |
List
product_name_list = ["Laptop", "Desk Chair", "Smartwatch", "Notebook", "Running Shoes"]
product_category_list = ["Electronics", "Furniture", "Electronics", "Stationery", "Apparel"]
product_id_list = [1, 2, 3, 4, 5]
product_price_list = [750, 100, 200, 5, 80]
product_stock_list = [50, 200, 150, 500, 100]
product_supplier_email_list = ["supplier1@gmail.com", "supplier2@gmail.com", "supplier3@gmail.com", "supplier4@gmail.com", "supplier5@gmail.com"]
Object
product_object1 = {
"name": "Laptop",
"category": "Electronics",
"id": 1,
"price": 750,
"stock": 50,
"supplier_email": "supplier1@gmail.com"
}
product_object2 = {
"name": "Desk Chair",
"category": "Furniture",
"id": 2,
"price": 100,
"stock": 200,
"supplier_email": "supplier2@gmail.com"
}
product_object3 = {
"name": "Smartwatch",
"category": "Electronics",
"id": 3,
"price": 200,
"stock": 150,
"supplier_email": "supplier3@gmail.com"
}
product_object4 = {
"name": "Notebook",
"category": "Stationery",
"id": 4,
"price": 5,
"stock": 500,
"supplier_email": "supplier4@gmail.com"
}
product_object5 = {
"name": "Running Shoes",
"category": "Apparel",
"id": 5,
"price": 80,
"stock": 100,
"supplier_email": "supplier5@gmail.com"
}
List of Objects
products = [
{
"id": 1,
"name": "Laptop",
"category": "Electronics",
"price": 750,
"stock": 50,
"supplier_email": "supplier1@gmail.com"
},
{
"id": 2,
"name": "Desk Chair",
"category": "Furniture",
"price": 100,
"stock": 200,
"supplier_email": "supplier2@gmail.com"
},
{
"id": 3,
"name": "Smartwatch",
"category": "Electronics",
"price": 200,
"stock": 150,
"supplier_email": "supplier3@gmail.com"
},
{
"id": 4,
"name": "Notebook",
"category": "Stationery",
"price": 5,
"stock": 500,
"supplier_email": "supplier4@gmail.com"
},
{
"id": 5,
"name": "Running Shoes",
"category": "Apparel",
"price": 80,
"stock": 100,
"supplier_email": "supplier5@gmail.com"
}
]
2. Employee Table
ID | Name | Department | Age | |
1 | John Doe | Sales | 30 | john.doe@company.com |
2 | Jane Smith | Human Resources | 25 | jane.smith@company.com |
3 | Mark Johnson | IT | 40 | mark.johnson@company.com |
4 | Lisa Wong | Marketing | 28 | lisa.wong@company.com |
5 | Paul McDonald | Finance | 35 | paul.mcdonald@company.com |
List
employee_name_list = ["John Doe", "Jane Smith", "Mark Johnson", "Lisa Wong", "Paul McDonald"]
employee_department_list = ["Sales", "Human Resources", "IT", "Marketing", "Finance"]
employee_id_list = [1, 2, 3, 4, 5]
employee_age_list = [30, 25, 40, 28, 35]
employee_email_list = ["john.doe@company.com", "jane.smith@company.com", "mark.johnson@company.com", "lisa.wong@company.com", "paul.mcdonald@company.com"]
Object
employee_object1 = {
"id": 1,
"name": "John Doe",
"department": "Sales",
"age": 30,
"email": "john.doe@company.com"
}
employee_object2 = {
"id": 2,
"name": "Jane Smith",
"department": "Human Resources",
"age": 25,
"email": "jane.smith@company.com"
}
employee_object3 = {
"id": 3,
"name": "Mark Johnson",
"department": "IT",
"age": 40,
"email": "mark.johnson@company.com"
}
employee_object4 = {
"id": 4,
"name": "Lisa Wong",
"department": "Marketing",
"age": 28,
"email": "lisa.wong@company.com"
}
employee_object5 = {
"id": 5,
"name": "Paul McDonald",
"department": "Finance",
"age": 35,
"email": "paul.mcdonald@company.com"
}
List of Objects
employees = [
{
"id": 1,
"name": "John Doe",
"department": "Sales",
"age": 30,
"email": "john.doe@company.com"
},
{
"id": 2,
"name": "Jane Smith",
"department": "Human Resources",
"age": 25,
"email": "jane.smith@company.com"
},
{
"id": 3,
"name": "Mark Johnson",
"department": "IT",
"age": 40,
"email": "mark.johnson@company.com"
},
{
"id": 4,
"name": "Lisa Wong",
"department": "Marketing",
"age": 28,
"email": "lisa.wong@company.com"
},
{
"id": 5,
"name": "Paul McDonald",
"department": "Finance",
"age": 35,
"email": "paul.mcdonald@company.com"
}
]
3. Books Table
ID | Title | Author | Genre | Published Year | ISBN | Stock | Price |
1 | The Great Gatsby | F. Scott Fitzgerald | Fiction | 1925 | 978-0743273565 | 20 | 15.99 |
2 | To Kill a Mockingbird | Harper Lee | Fiction | 1960 | 978-0060935467 | 35 | 10.99 |
3 | 1984 | George Orwell | Dystopian | 1949 | 978-0451524935 | 40 | 9.99 |
4 | The Catcher in the Rye | J.D. Salinger | Fiction | 1951 | 978-0316769488 | 25 | 8.99 |
5 | A Brief History of Time | Stephen Hawking | Non-fiction | 1988 | 978-0553380163 | 10 | 18.99 |
List
book_id_list = [1, 2, 3, 4, 5]
book_title_list = ["The Great Gatsby", "To Kill a Mockingbird", "1984", "The Catcher in the Rye", "A Brief History of Time"]
book_author_list = ["F. Scott Fitzgerald", "Harper Lee", "George Orwell", "J.D. Salinger", "Stephen Hawking"]
book_genre_list = ["Fiction", "Fiction", "Dystopian", "Fiction", "Non-fiction"]
book_published_year_list = [1925, 1960, 1949, 1951, 1988]
book_isbn_list = ["978-0743273565", "978-0060935467", "978-0451524935", "978-0316769488", "978-0553380163"]
book_stock_list = [20, 35, 40, 25, 10]
book_price_list = [15.99, 10.99, 9.99, 8.99, 18.99]
Object
book1 = {
"id": 1,
"title": "The Great Gatsby",
"author": "F. Scott Fitzgerald",
"genre": "Fiction",
"published_year": 1925,
"isbn": "978-0743273565",
"stock": 20,
"price": 15.99
}
book2 = {
"id": 2,
"title": "To Kill a Mockingbird",
"author": "Harper Lee",
"genre": "Fiction",
"published_year": 1960,
"isbn": "978-0060935467",
"stock": 35,
"price": 10.99
}
book3 = {
"id": 3,
"title": "1984",
"author": "George Orwell",
"genre": "Dystopian",
"published_year": 1949,
"isbn": "978-0451524935",
"stock": 40,
"price": 9.99
}
book4 = {
"id": 4,
"title": "The Catcher in the Rye",
"author": "J.D. Salinger",
"genre": "Fiction",
"published_year": 1951,
"isbn": "978-0316769488",
"stock": 25,
"price": 8.99
}
book5 = {
"id": 5,
"title": "A Brief History of Time",
"author": "Stephen Hawking",
"genre": "Non-fiction",
"published_year": 1988,
"isbn": "978-0553380163",
"stock": 10,
"price": 18.99
}
List of Objects
books = [
{
"id": 1,
"title": "The Great Gatsby",
"author": "F. Scott Fitzgerald",
"genre": "Fiction",
"published_year": 1925,
"isbn": "978-0743273565",
"stock": 20,
"price": 15.99
},
{
"id": 2,
"title": "To Kill a Mockingbird",
"author": "Harper Lee",
"genre": "Fiction",
"published_year": 1960,
"isbn": "978-0060935467",
"stock": 35,
"price": 10.99
},
{
"id": 3,
"title": "1984",
"author": "George Orwell",
"genre": "Dystopian",
"published_year": 1949,
"isbn": "978-0451524935",
"stock": 40,
"price": 9.99
},
{
"id": 4,
"title": "The Catcher in the Rye",
"author": "J.D. Salinger",
"genre": "Fiction",
"published_year": 1951,
"isbn": "978-0316769488",
"stock": 25,
"price": 8.99
},
{
"id": 5,
"title": "A Brief History of Time",
"author": "Stephen Hawking",
"genre": "Non-fiction",
"published_year": 1988,
"isbn": "978-0553380163",
"stock": 10,
"price": 18.99
}
]
4. University Table
ID | Name | Location | Established Year | Type | Website |
1 | University of the Philippines | Quezon City | 1908 | Public | www.up.edu.ph |
2 | Ateneo de Manila University | Quezon City | 1859 | Private | www.ateneo.edu |
3 | De La Salle University | Manila | 1911 | Private | www.dlsu.edu.ph |
4 | University of Santo Tomas | Manila | 1611 | Private | www.ust.edu.ph |
5 | Polytechnic University of the Philippines | Manila | 1904 | Public | www.pup.edu.ph |
List
university_id_list = [1, 2, 3, 4, 5]
university_name_list = ["University of the Philippines", "Ateneo de Manila University", "De La Salle University", "University of Santo Tomas", "Polytechnic University of the Philippines"]
university_location_list = ["Quezon City", "Quezon City", "Manila", "Manila", "Manila"]
university_established_year_list = [1908, 1859, 1911, 1611, 1904]
university_type_list = ["Public", "Private", "Private", "Private", "Public"]
university_website_list = ["www.up.edu.ph", "www.ateneo.edu", "www.dlsu.edu.ph", "www.ust.edu.ph", "www.pup.edu.ph"]
Object
university1 = {
"id": 1,
"name": "University of the Philippines",
"location": "Quezon City",
"established_year": 1908,
"type": "Public",
"website": "www.up.edu.ph"
}
university2 = {
"id": 2,
"name": "Ateneo de Manila University",
"location": "Quezon City",
"established_year": 1859,
"type": "Private",
"website": "www.ateneo.edu"
}
university3 = {
"id": 3,
"name": "De La Salle University",
"location": "Manila",
"established_year": 1911,
"type": "Private",
"website": "www.dlsu.edu.ph"
}
university4 = {
"id": 4,
"name": "University of Santo Tomas",
"location": "Manila",
"established_year": 1611,
"type": "Private",
"website": "www.ust.edu.ph"
}
university5 = {
"id": 5,
"name": "Polytechnic University of the Philippines",
"location": "Manila",
"established_year": 1904,
"type": "Public",
"website": "www.pup.edu.ph"
}
List of Objects
universities = [
{
"id": 1,
"name": "University of the Philippines",
"location": "Quezon City",
"established_year": 1908,
"type": "Public",
"website": "www.up.edu.ph"
},
{
"id": 2,
"name": "Ateneo de Manila University",
"location": "Quezon City",
"established_year": 1859,
"type": "Private",
"website": "www.ateneo.edu"
},
{
"id": 3,
"name": "De La Salle University",
"location": "Manila",
"established_year": 1911,
"type": "Private",
"website": "www.dlsu.edu.ph"
},
{
"id": 4,
"name": "University of Santo Tomas",
"location": "Manila",
"established_year": 1611,
"type": "Private",
"website": "www.ust.edu.ph"
},
{
"id": 5,
"name": "Polytechnic University of the Philippines",
"location": "Manila",
"established_year": 1904,
"type": "Public",
"website": "www.pup.edu.ph"
}
]
ID | Name | Location | Cuisine Type | Established Year | Website or Contact |
1 | Vikings Luxury Buffet | Pasay City | Buffet | 2011 | www.vikings.ph |
2 | Antonio's Restaurant | Tagaytay | Fine Dining | 2002 | www.antoniosrestaurant.ph |
3 | Mesa Filipino Moderne | Makati City | Filipino | 2009 | www.mesa.ph |
4 | Manam Comfort Filipino | Quezon City | Filipino | 2013 | www.manam.ph |
5 | Ramen Nagi | Various Locations | Japanese | 2013 | www.ramennagi.com.ph |
List
restaurant_id_list = [1, 2, 3, 4, 5]
restaurant_name_list = ["Vikings Luxury Buffet", "Antonio's Restaurant", "Mesa Filipino Moderne", "Manam Comfort Filipino", "Ramen Nagi"]
restaurant_location_list = ["Pasay City", "Tagaytay", "Makati City", "Quezon City", "Various Locations"]
restaurant_cuisine_type_list = ["Buffet", "Fine Dining", "Filipino", "Filipino", "Japanese"]
restaurant_established_year_list = [2011, 2002, 2009, 2013, 2013]
restaurant_website_list = ["www.vikings.ph", "www.antoniosrestaurant.ph", "www.mesa.ph", "www.manam.ph", "www.ramennagi.com.ph"]
Object
restaurant1 = {
"id": 1,
"name": "Vikings Luxury Buffet",
"location": "Pasay City",
"cuisine_type": "Buffet",
"established_year": 2011,
"website": "www.vikings.ph"
}
restaurant2 = {
"id": 2,
"name": "Antonio's Restaurant",
"location": "Tagaytay",
"cuisine_type": "Fine Dining",
"established_year": 2002,
"website": "www.antoniosrestaurant.ph"
}
restaurant3 = {
"id": 3,
"name": "Mesa Filipino Moderne",
"location": "Makati City",
"cuisine_type": "Filipino",
"established_year": 2009,
"website": "www.mesa.ph"
}
restaurant4 = {
"id": 4,
"name": "Manam Comfort Filipino",
"location": "Quezon City",
"cuisine_type": "Filipino",
"established_year": 2013,
"website": "www.manam.ph"
}
restaurant5 = {
"id": 5,
"name": "Ramen Nagi",
"location": "Various Locations",
"cuisine_type": "Japanese",
"established_year": 2013,
"website": "www.ramennagi.com.ph"
}
List of Objects
restaurants = [
{
"id": 1,
"name": "Vikings Luxury Buffet",
"location": "Pasay City",
"cuisine_type": "Buffet",
"established_year": 2011,
"website": "www.vikings.ph"
},
{
"id": 2,
"name": "Antonio's Restaurant",
"location": "Tagaytay",
"cuisine_type": "Fine Dining",
"established_year": 2002,
"website": "www.antoniosrestaurant.ph"
},
{
"id": 3,
"name": "Mesa Filipino Moderne",
"location": "Makati City",
"cuisine_type": "Filipino",
"established_year": 2009,
"website": "www.mesa.ph"
},
{
"id": 4,
"name": "Manam Comfort Filipino",
"location": "Quezon City",
"cuisine_type": "Filipino",
"established_year": 2013,
"website": "www.manam.ph"
},
{
"id": 5,
"name": "Ramen Nagi",
"location": "Various Locations",
"cuisine_type": "Japanese",
"established_year": 2013,
"website": "www.ramennagi.com.ph"
}
]
Why is data structure important again?
Understanding how to organize data with lists and objects is essential for creating more complicated data structures and algorithms in programming. Efficient data organization improves the speed of accessing, retrieving, and modifying data, which is critical for software development.
Frontend and Backend Development Context
Frontend: You may need to dynamically show student or product information on a website.
Backend: Data may need to be stored, processed, or manipulated before it can be sent to a frontend application or saved in a database.
Additional information and this is based on Chat GPT might be helpful for more resources.
Data structures are fundamental to computer science and programming, as they provide a means to organize, manage, and store data efficiently. Here are some key reasons highlighting their importance:
Efficiency: Different data structures provide different efficiencies for various operations (e.g., searching, insertion, deletion). Choosing the right data structure can significantly improve the performance of algorithms.
Organization: Data structures allow for the organized storage of data, making it easier to access and manipulate. This organization is crucial for handling large amounts of data.
Code Maintenance: Well-chosen data structures make code easier to read, understand, and maintain. They can encapsulate data and functionality, promoting cleaner code and reducing bugs.
Memory Management: Data structures enable efficient use of memory, allowing programmers to allocate and deallocate memory dynamically. This is particularly important for applications with varying data sizes.
Scalability: Efficient data structures are essential for building scalable applications. As the size of the data grows, the choice of data structure impacts the application’s ability to handle that growth.
Algorithm Design: Many algorithms are built around specific data structures (e.g., trees, graphs). Understanding data structures helps in designing algorithms that can solve problems effectively.
Real-world Modeling: Data structures can represent real-world entities and relationships, making them invaluable in simulations, databases, and complex systems.
Performance Optimization: Knowledge of data structures allows developers to optimize performance for specific applications, leading to faster and more responsive systems.
Complexity Management: They help manage the complexity of data handling, enabling developers to focus on higher-level programming without getting bogged down by implementation details.
For further reading, you might find these resources helpful:
- Data Structures and Algorithm Specialization - Coursera: https://www.coursera.org/specializations/data-structures-algorithms
Subscribe to my newsletter
Read articles from Walter Mark B. Inductivo Jr. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Walter Mark B. Inductivo Jr.
Walter Mark B. Inductivo Jr.
Watch Me Whip 👊, Watch Me Nae-Nae 🖐️