Blog Post: Integrating Paystack in React Native and Storing Paystack Response in a MySQL Database
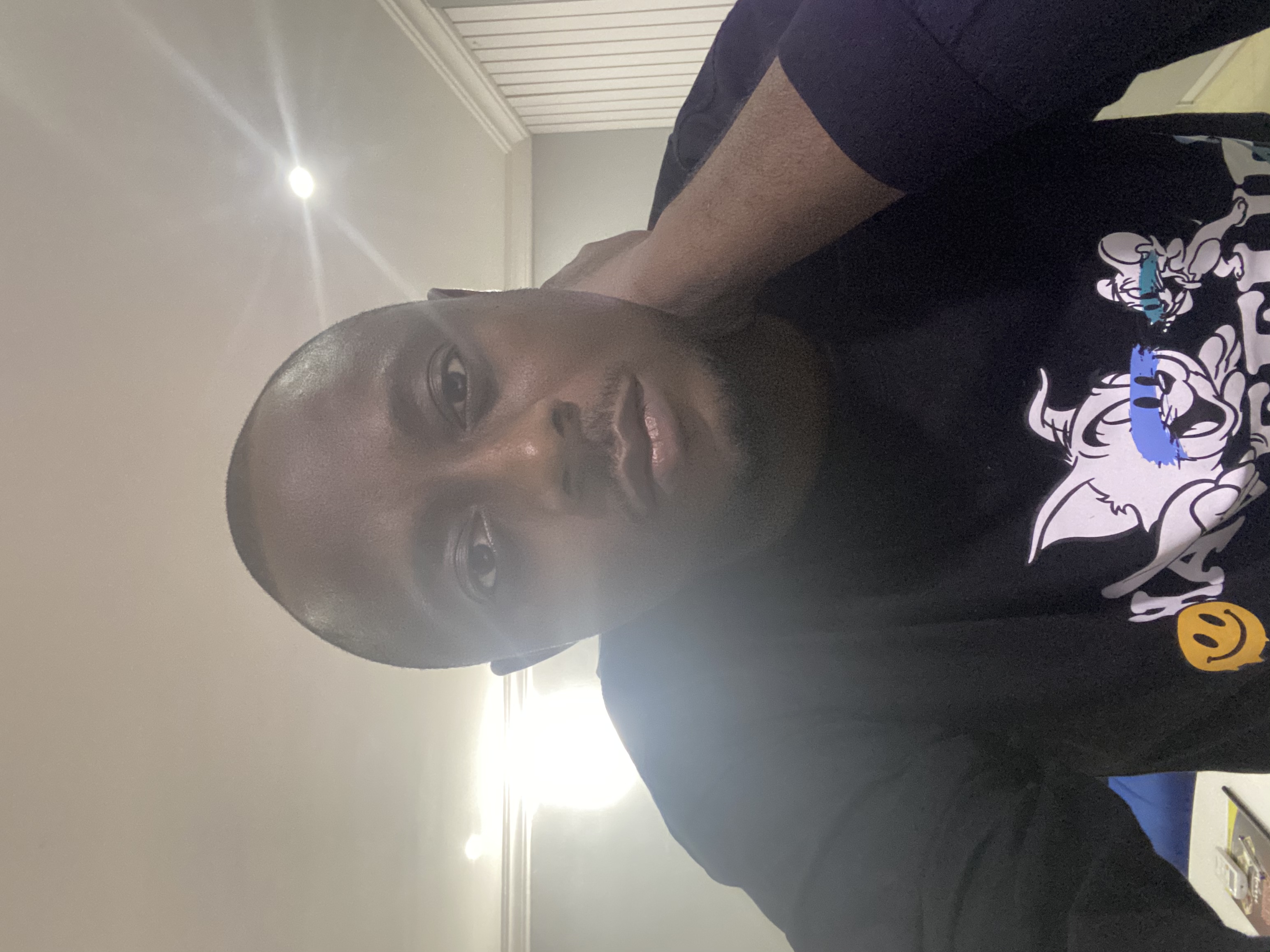
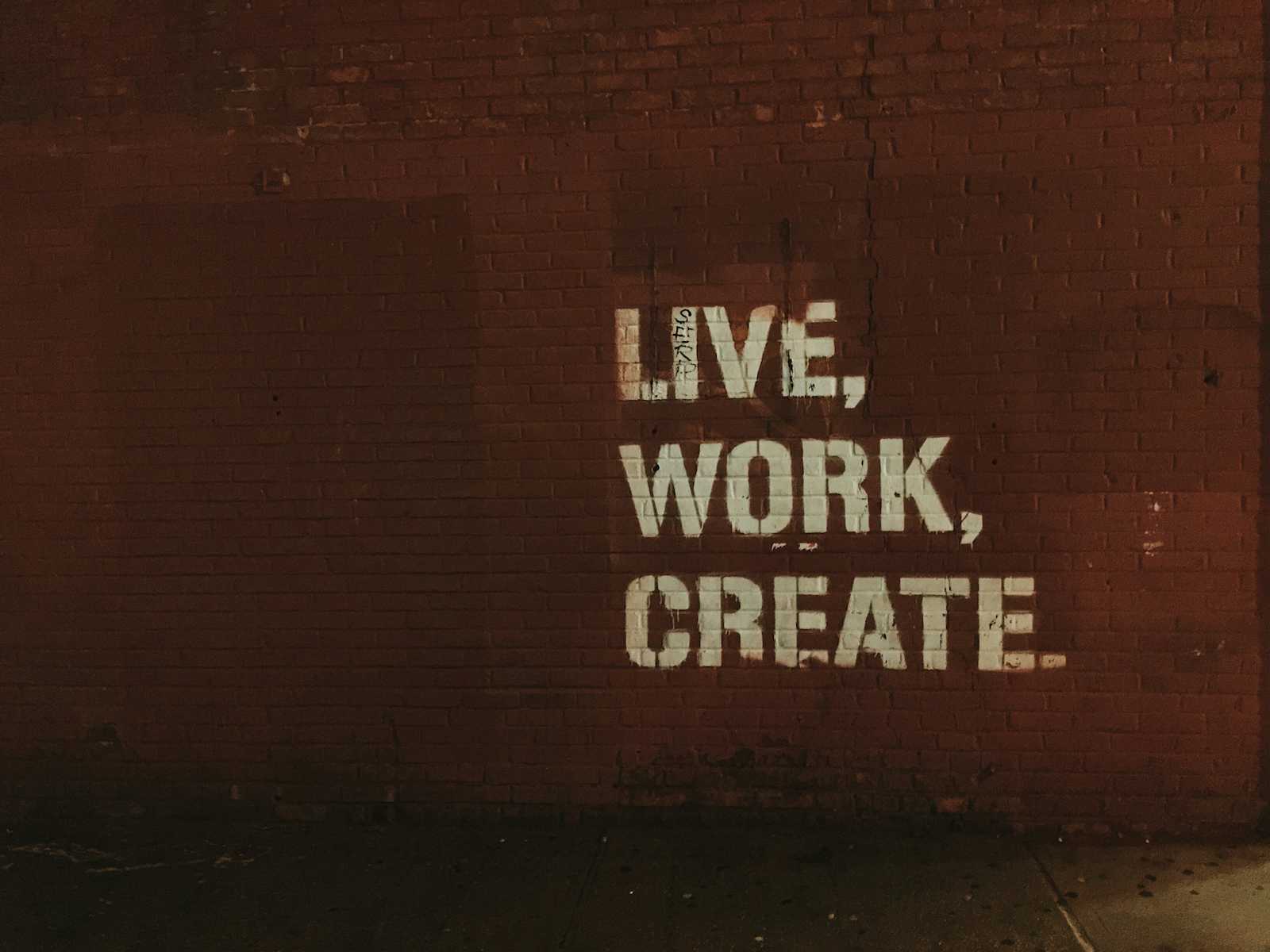
In this blog post, I’ll walk you through how to integrate Paystack for payments in a React Native application and store the payment response in a MySQL database using PHP.
Step 1: Install Paystack in React Native
You need to install the Paystack library for React Native. You can do this by running:
Install the react-native-paystack-webview
package
npm install react-native-paystack-webview
Alternatively, if you are using Yarn:
yarn add react-native-paystack-webview
This library allows you to handle payments via Paystack directly from your React Native application using a webview.
Install the react-native-webview
package
To ensure the WebView component works in React Native, install the react-native-webview
dependency as well.
npm install react-native-webview
After installation, always run npm install
(or yarn
) to sync up your dependencies.
Step 2: React Native Code
Below is an example of how to set up Paystack in your React Native app.
import React, { useState, useRef } from 'react';
import { ScrollView, TouchableOpacity, Text, View, StyleSheet } from 'react-native';
import { Paystack, paystackProps } from 'react-native-paystack-webview';
const DriverScreen = () => {
const [pay, setPay] = useState(1); // Payment status
const paystackWebViewRef = useRef(paystackProps.PayStackRef); // Ref for the Paystack WebView
const email = "user@example.com"; // User email
const payment = 5000; // Payment amount
const currencyCode = "NGN"; // Currency code
return (
<ScrollView style={styles.container}>
<Text style={styles.title}>Pending Payment</Text>
<Text style={styles.amount}>Amount Due: {currencyCode} {payment}</Text>
<View>
<TouchableOpacity
style={styles.button}
onPress={() => {
// Start the transaction
paystackWebViewRef.current.startTransaction();
}}>
<Text style={styles.buttonText}>Pay Now</Text>
</TouchableOpacity>
<View style={{ flex: 1 }}>
<Paystack
paystackKey="pk_live_XXXXXXXXXXXXXX" // Your live Paystack public key
billingEmail={email}
amount={payment}
onCancel={(e) => {
console.log('Transaction canceled:', e);
}}
onSuccess={(res) => {
const { data } = res;
const transactionRef = data.transactionRef.reference;
const transactionStatus = data.transactionRef.status;
const amountPaid = payment;
const emailUsed = email;
// Post data to the backend
fetch('https://yourwebsite.com/drivercallback.php', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
transactionRef,
transactionStatus,
amountPaid,
emailUsed,
}),
})
.then((response) => response.json())
.then((data) => {
console.log('Backend response:', data);
// If the transaction is successful, update the payment status
if (transactionStatus === 'success') {
setPay(0);
}
})
.catch((error) => console.error('Error posting to backend:', error));
}}
ref={paystackWebViewRef}
channels={['card', 'ussd', 'qr', 'bank_transfer']} // Supported payment channels
/>
</View>
</View>
</ScrollView>
);
};
const styles = StyleSheet.create({
container: { flex: 1, padding: 20 },
title: { fontSize: 24, fontWeight: 'bold' },
amount: { fontSize: 18, marginVertical: 10 },
button: { backgroundColor: 'blue', padding: 15, borderRadius: 5 },
buttonText: { color: 'white', textAlign: 'center' },
});
export default DriverScreen;
Step 3: Backend PHP Code
After a successful transaction, we need to store the Paystack response and update the user's payment status in the MySQL database.
The PHP code below handles the Paystack transaction response and updates the driver
table:
<?php
include("config.php"); // Database connection
// Process incoming data
if ($_SERVER["REQUEST_METHOD"] === "POST") {
$data = json_decode(file_get_contents("php://input"), true);
// Get the data from the request
$email = $data["emailUsed"];
$transactionRef = $data["transactionRef"];
$transactionStatus = $data["transactionStatus"];
$amountPaid = $data["amountPaid"];
$pay = 0; // Payment status after success
$typer = 'Taxi Payment'; // Type of payment
// Insert the transaction details into the database
$sql = "INSERT INTO `paystacktransaction` (`email`, `reference`, `status`, `amount`, `typer`)
VALUES ('$email', '$transactionRef', '$transactionStatus', '$amountPaid', '$typer')";
mysqli_query($link, $sql);
// Update the driver's payment status
$stmt = $link->prepare("UPDATE driver SET pay = ?, payment = payment - ? WHERE email = ?");
$stmt->bind_param('iis', $pay, $amountPaid, $email);
// Execute the statement
if ($stmt->execute()) {
$response = array(
"status" => "success",
"message" => "Status Updated"
);
} else {
$response = array(
"status" => "error",
"message" => "Failed to update status"
);
}
// Close the statement
$stmt->close();
// Send the response as JSON
header("Content-Type: application/json");
echo json_encode($response);
}
?>
Step 4: Testing the Integration
Start by initiating a payment in your React Native app.
Once the payment is successful, the Paystack WebView will call the
onSuccess
handler.The transaction data will be sent to your PHP backend, which updates the database and sends back a success message.
Conclusion
By following these steps, you can integrate Paystack into your React Native app to accept payments and store the transaction details in a MySQL database. You can further customize this integration to fit your business needs by adding more checks and validations.
Subscribe to my newsletter
Read articles from Ogunuyo Ogheneruemu B directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
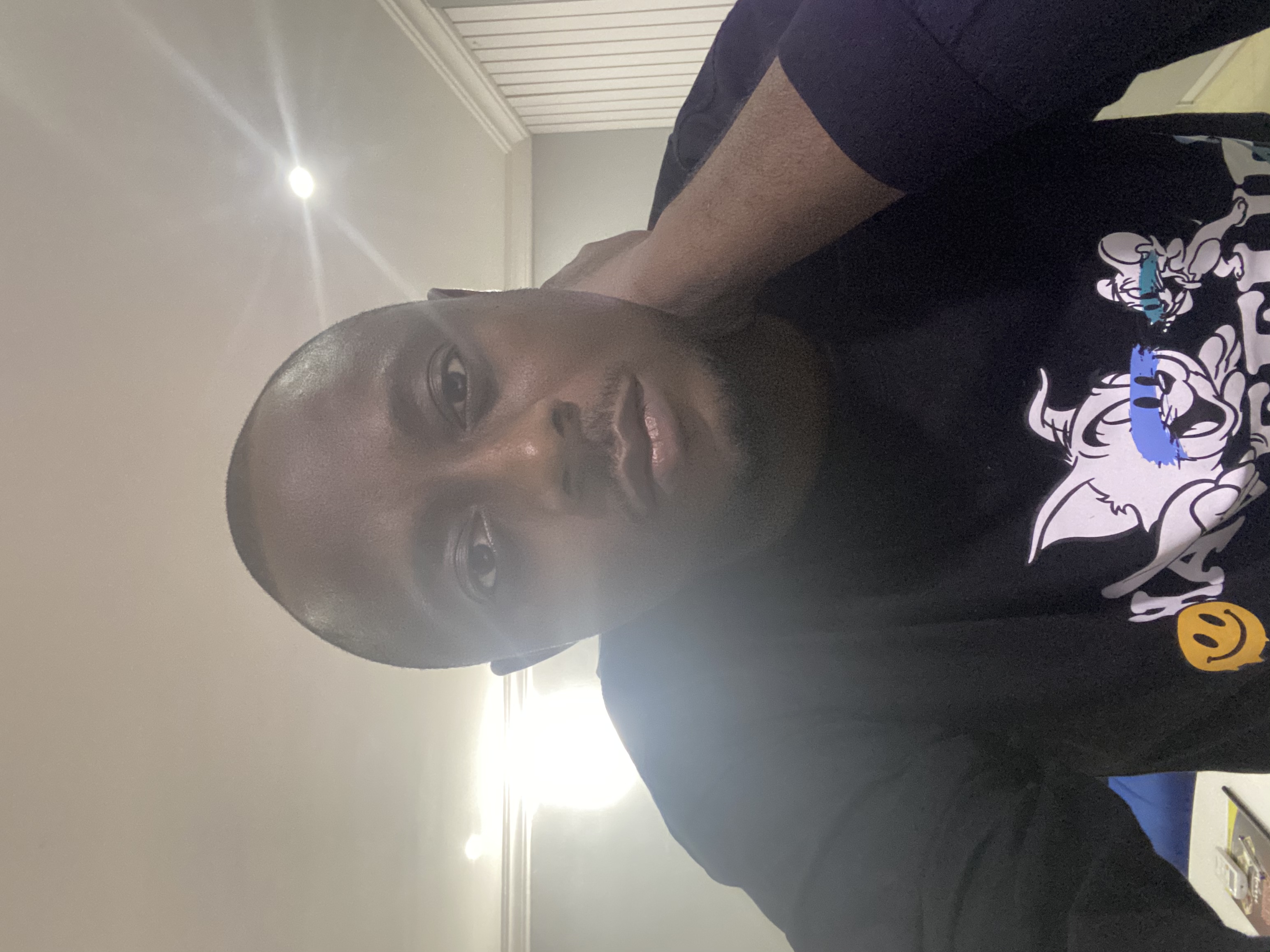
Ogunuyo Ogheneruemu B
Ogunuyo Ogheneruemu B
I'm Ogunuyo Ogheneruemu Brown, a senior software developer. I specialize in DApp apps, fintech solutions, nursing web apps, fitness platforms, and e-commerce systems. Throughout my career, I've delivered successful projects, showcasing strong technical skills and problem-solving abilities. I create secure and user-friendly fintech innovations. Outside work, I enjoy coding, swimming, and playing football. I'm an avid reader and fitness enthusiast. Music inspires me. I'm committed to continuous growth and creating impactful software solutions. Let's connect and collaborate to make a lasting impact in software development.