Fetch API and AXIOS

Table of contents
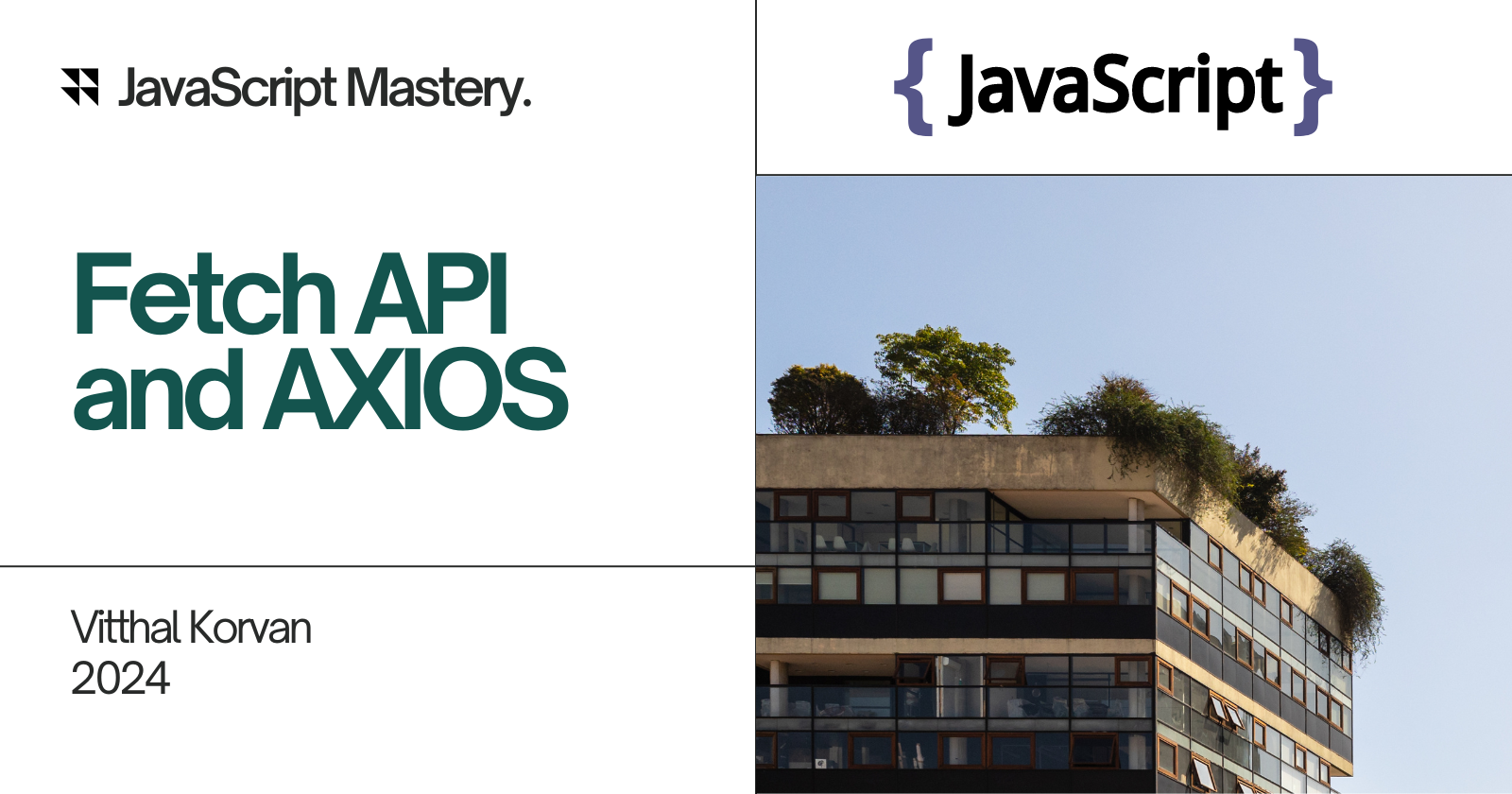
Fetch API
The Fetch API
is a modern, flexible, and powerful JavaScript interface for making HTTP requests to servers. It is a replacement for XMLHttpRequest
(XHR) and offers a simpler, cleaner syntax using promises, allowing developers to handle asynchronous requests more easily.
1. Basic Syntax
The fetch()
function takes in a resource (URL or request object) and returns a Promise that resolves to the Response
object, which represents the server's response.
Basic Example (GET request):
fetch('https://jsonplaceholder.typicode.com/posts')
.then(response => response.json()) // parsing the response to JSON
.then(data => console.log(data)) // handling the data
.catch(error => console.error('Error:', error)); // handling any errors
2. Promise-based
The fetch()
function is promise-based, which means:
Asynchronous: The code doesn't block further execution.
Chaining: Responses can be processed in chains of
.then()
calls, and errors can be handled in a.catch()
.
3. Response Handling
The response is not automatically parsed (like in XHR). The Response
object has methods to retrieve different types of body content:
response.json()
– parse the response as JSON.response.text()
– read the response as a string.response.blob()
– read the response as binary data (Blob).response.formData()
– read the response asFormData
.response.arrayBuffer()
– read the response as anArrayBuffer
.
Example:
fetch('https://api.example.com/data')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json(); // or response.text() or response.blob(), etc.
})
.then(data => console.log(data))
.catch(error =>
console.error('There was a problem with the fetch operation:', error));
4. HTTP Methods
The fetch()
API supports all standard HTTP methods (GET
, POST
, PUT
, DELETE
, etc.). For GET
, the default method is used, but for other methods like POST
, you must specify the method and options explicitly.
POST Request Example:
fetch('https://jsonplaceholder.typicode.com/posts', {
method: 'POST', // specifying the method
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
title: 'foo',
body: 'bar',
userId: 1,
}),
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
5. Headers
You can specify custom headers in the request, such as Content-Type
, Authorization
, or any other headers your API requires. You can do this using the headers
property in the options object.
Example with headers:
fetch('https://api.example.com/data', {
method: 'GET',
headers: {
'Authorization': 'Bearer someTokenValue',
'Content-Type': 'application/json',
},
})
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.error('Error:', error));
6. Handling Errors
The fetch()
function does not reject the promise on HTTP errors like 404 or 500. Instead, the promise is fulfilled, and you must check the response.ok
property to detect these types of errors.
Example:
fetch('https://api.example.com/unknown-endpoint')
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
.then(data => console.log(data))
.catch(error => console.error('Fetch error:', error));
7. Timeouts & Aborting Requests
The fetch()
API does not natively support request timeouts, but you can achieve this by using the AbortController
to abort a request manually.
Example of Timeout with AbortController
:
const controller = new AbortController();
const timeoutId = setTimeout(() => controller.abort(), 5000);
// Timeout after 5 seconds
fetch('https://api.example.com/data', { signal: controller.signal })
.then(response => response.json())
.then(data => console.log(data))
.catch(error => {
if (error.name === 'AbortError') {
console.error('Request aborted due to timeout');
} else {
console.error('Fetch error:', error);
}
});
8. Request and Response Objects
The fetch()
API allows you to create both Request and Response objects, which can give you more fine-grained control over the request and its configuration.
Request Example:
const request = new Request('https://api.example.com/data', {
method: 'POST',
headers: new Headers({
'Content-Type': 'application/json',
}),
body: JSON.stringify({ key: 'value' }),
});
fetch(request)
.then(response => response.json())
.then(data => console.log(data));
Using async/await
with Fetch
The fetch()
API works seamlessly with async/await
, which makes the code even cleaner and more readable than using .then()
chains.
Example with async/await
:
async function fetchData() {
try {
const response =
await fetch('https://jsonplaceholder.typicode.com/posts');
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Fetch error:', error);
}
}
fetchData();
AXIOS
Axios is a popular, promise-based HTTP client for the browser and Node.js. It is widely used in web development for making HTTP requests, such as sending data to a server or fetching data from a remote resource. Axios is often preferred over the Fetch API
or XMLHttpRequest
(XHR) because of its ease of use, comprehensive feature set, and better support for advanced use cases like intercepting requests, automatic JSON parsing, and canceling requests.
1. Installation
Axios can be used in both Node.js and the browser.
Browser: You can include it via CDN:
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"> </script>
Node.js: You can install it using
npm
oryarn
:npm install axios
2. Basic Usage
Axios provides methods for all the common HTTP verbs like GET
, POST
, PUT
, DELETE
, and others.
Example of a simple GET request:
axios.get('https://jsonplaceholder.typicode.com/posts')
.then(response => {
console.log(response.data); // Handle the response data
})
.catch(error => {
console.error('Error fetching data:', error); // Handle any errors
});
3. Axios Methods
Axios provides shorthand methods for all HTTP methods:
GET:
axios.get(url[, config])
POST:
axios.post
(url[, data[, config]])
PUT:
axios.put(url[, data[, config]])
DELETE:
axios.delete(url[, config])
PATCH:
axios.patch(url[, data[, config]])
Example for POST:
axios.post('https://jsonplaceholder.typicode.com/posts', {
title: 'foo',
body: 'bar',
userId: 1
})
.then(response => console.log(response.data))
.catch(error => console.error('Error:', error));
4. Promises and Async/Await
Like the Fetch API, Axios returns a promise, which can be handled with .then()
and .catch()
. Alternatively, you can use async/await
for cleaner, more synchronous-looking code.
Example with async/await
:
async function fetchData() {
try {
const response =
await axios.get('https://jsonplaceholder.typicode.com/posts');
console.log(response.data); // Process the response
} catch (error) {
console.error('Error fetching data:', error);
}
}
fetchData();
5. Request and Response Objects
Axios automatically transforms JSON data. It wraps requests and responses with convenient objects, making it easier to work with them.
Response Object
The response
object returned by Axios includes:
data
: The response body (already parsed as JSON if applicable).status
: HTTP status code of the response.statusText
: HTTP status message.headers
: Headers associated with the response.config
: The request configuration used for the request.request
: The underlying XMLHttpRequest or Request object.
axios.get('https://jsonplaceholder.typicode.com/posts')
.then(response => {
console.log('Data:', response.data);
console.log('Status:', response.status);
console.log('Headers:', response.headers);
});
Request Configuration
You can configure requests by passing a config
object. It supports many options like headers
, timeout
, params
, auth
, etc.
Example of a GET request with parameters:
axios.get('https://jsonplaceholder.typicode.com/posts', {
params: {
userId: 1
}
})
.then(response => console.log(response.data));
Fetch API vs Axios
Feature | Fetch API | Axios |
Built-in | Native to browsers, no installation needed | Third-party library (requires installation) |
Syntax Simplicity | Basic syntax, but requires more manual work (e.g., error handling, JSON parsing) | Simplified and more concise syntax for common tasks |
JSON Parsing | Requires manual parsing with response.json() | Automatically parses JSON response |
Error Handling | Manual check needed using response.ok | Built-in automatic error handling |
Timeout Handling | No built-in support, needs manual setup | Built-in support for request timeouts |
Interceptors | Not available | Supports request and response interceptors for preprocessing or logging |
Browser Support | Supported in all modern browsers | Works on browsers and Node.js environments |
Cancel Requests | Requires additional handling (AbortController) | Easy to cancel requests with built-in support |
File Uploads | Requires manual setup | Simplified syntax for file uploads |
Performance | Slightly faster due to being native | May have slightly more overhead due to being a library |
GitHub Link : GitHub - JavaScript Mastery
Subscribe to my newsletter
Read articles from Code Subtle directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Code Subtle
Code Subtle
At Code Subtle, we empower aspiring web developers through personalized mentorship and engaging learning resources. Our community bridges the gap between theory and practice, guiding students from basics to advanced concepts. We offer expert mentorship and write interactive, user-friendly articles on all aspects of web development. Join us to learn, grow, and build your future in tech!