Defeating Python: Phase 3 Complete

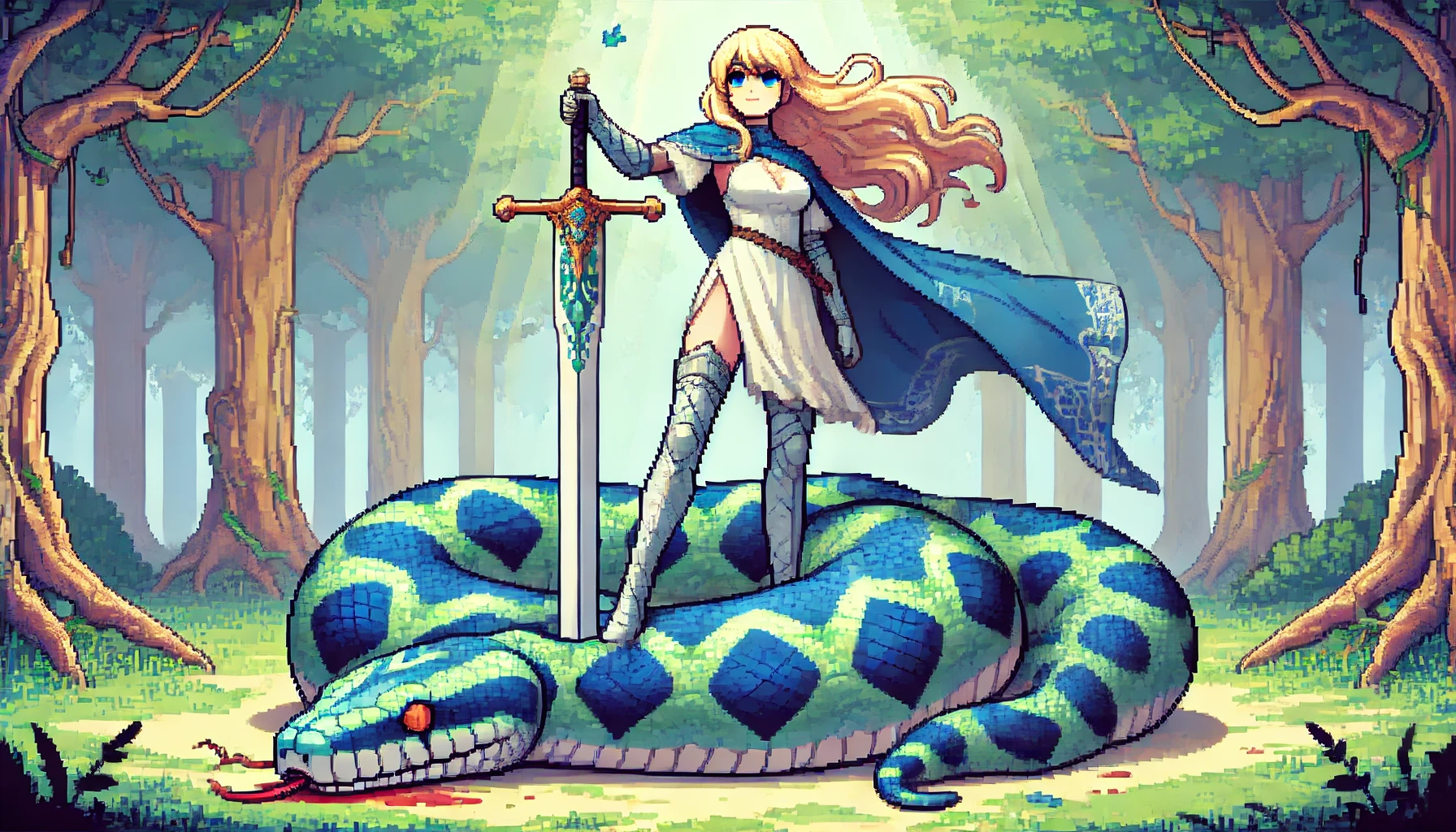
Hey everyone, Phase 3 is officially complete, and I’m thrilled to share my progress with you! I had a much smoother experience with Python compared to my time with React. For this phase's project, I chose to create an adventure choice-based game, which was an exciting challenge. The most time-consuming part of the project was crafting the story. I loved developing it for the user and ended up leaving it on a cliffhanger for now, but I plan to expand it in the future. Even with the cliffhanger, the project feels complete and fully functional.
The game begins with the player waking up in a forest. Armed with only 100 gold coins, 100 health points, a stick, and the memory of their name (which they input into the console), they embark on their journey. The player first encounters a large dung beetle, then a mysterious vampire alchemist deep in the woods, and eventually makes their way to the city of Constania. Here, they meet Dylan, the blacksmith merchant, who provides them with the weapon they'll need to face the High Wizard. The story pauses just as they leave Constania, heading towards Cerulean City, where the High Wizard awaits.
As the player navigates the journey, they might gain new weapons, lose or gain gold and HP, acquire healing items, and uncover the lore of this world! If you're curious about the game’s mechanics, check out the README in the repository linked below, where I've included AI-generated visuals to enhance the gameplay experience.
A Look At The Code: Setting Up The Player:
One of the most interesting parts of the game was setting up the player’s initial stats and making sure the choices they make affect these stats dynamically throughout the game. Here’s an example of how I initialized the player:
def main():
global player
print("\n -------Welcome to The Journey Never Ends Adventure Game!-------")
print("\n ------------------------------")
print("""\n You have just awoken in a foggy, mystical forest. You see that your health is at 100, you have 100 gold coins in your pocket,
and a sturdy-looking stick beside you that would make a pitiful, but semi-useful weapon. You must have hit your head because
you cannot remember much. But you do remember your name. """)
player_name = input("What is your name?: ")
player = Character(player_name)
player.save()
class Character():
def __init__(self, name, health_points = 100, gold_coins = 100, id=None):
self.id = id
self.name = name
self.health_points = health_points
self.gold_coins = gold_coins
self.inventory = []
self.current_weapon = Item("Stick", "Weapon", 5)
This code sets up the player with an initial 100 gold coins, 100 health points, a basic weapon (a stick), and an empty inventory. As the player progresses through the game, they have the opportunity to gain new items, find new weapons, and either lose or earn more gold and health depending on the choices they make.
The Impact of Player Choices
Player choices dynamically affect their journey. For example, here’s how I handle a fight where the player can gain or lose health:
def continue_story():
global story_progress
if story_progress == 0:
print(f"""\n Well, {player.name}, you are in for a bit of a surprise.
There is a human-sized dung beetle heading right towards you.
What do you do?""")
print("1. Fight")
print("2. Flee")
print("3. Menu")
choice = input("> ")
if choice == "1":
story_progress = 2
print("""\n You grab your sturdy stick and take a fighting stance against the beetle.
It stops and observes you...
It starts barreling forward, but you dodge.
You turn and start beating the dung beetle to a pulp.
It collapses to the ground, dead.""")
elif choice == "2":
story_progress = 1
print("""\n You gather yourself and run away.
You trip and hit your head, losing 10 HP.
The dung beetle is closing in.""")
player.lose_health(10)
player.save()
elif choice == "3":
menu()
return
else: print("Invalid Choice")
This code snippet shows how the player's choices lead to different outcomes. If they choose to fight, they earn a new weapon but lose 10 gold. If they flee, they lose health. This decision-making mechanic is key to making the game feel interactive and engaging.
I began this phase feeling like I was battling Python, but now I can confidently say I’ve conquered (or at least learned) Python! In the next phase, we dive into SQLAlchemy, an open-source Python library that provides an SQL toolkit and Object Relational Mapper (ORM) for interacting with databases. SQLAlchemy allows developers to work with databases using Python objects, enabling efficient and flexible database access. I’m looking forward to this new challenge and continuing to grow my software development skills.
If you're interested in checking out my project or giving the game a try, you can find my project repository on GitHub with a detailed README [HERE].
Thanks for following along on this journey—more to come!
xoxo,
Bre <3
Subscribe to my newsletter
Read articles from Breanna Humphres directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Breanna Humphres
Breanna Humphres
Hi, I'm Bre! I'm a software engineering student at Flatiron School. I graduate in November of 2024 and look forward to joining the gaming development or tech world afterward! I'm very passionate about gaming, and have a big goal of working in the gaming development world.