Hash Maps in DSA
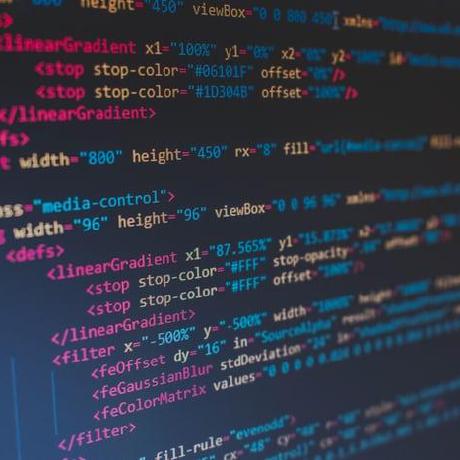
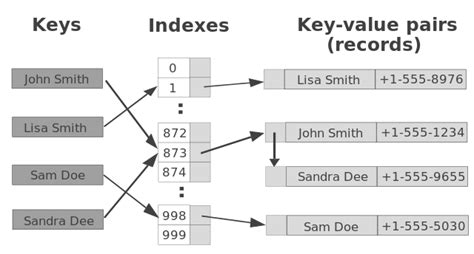
What are Hash Maps
Hash maps are data structures that store key-value pairs, allowing for efficient data retrieval. They function similarly to a dictionary, where a unique key corresponds to a specific value. For instance, consider a scenario where you want to store the names of popular Nigerian dishes and their corresponding recipes. In this case, the dish names serve as keys, and the recipes are the values. When you want to retrieve a recipe, you simply use the dish name as the key, making it quick and straightforward.
Types of Hash Maps
There are two primary types of hash maps:
Open Addressing: In this method, if a particular index in the hash map is occupied, the algorithm searches for the next available index. This is akin to looking for an empty seat in a crowded Nigerian bus; if your preferred seat is taken, you simply move to the next one.
Chaining: This approach involves storing multiple values at a single index using a linked list or another data structure. If two keys hash to the same index, they are stored in a list at that index. This can be compared to having multiple people sharing a table at a local eatery when all chairs are occupied.
Operations of Hash Maps and Their Time Complexities
Hash maps are known for their efficiency in performing various operations. Here are some common operations along with their average time complexities:
Insert (put): Adding a new key-value pair typically takes O(1) time on average. For example, inserting the dish "Jollof Rice" with its recipe into the hash map can be done quickly.
Search (get): Retrieving a value using its key also takes about O(1) time on average. If you want to find the recipe for "Egusi Soup," you can do so almost instantly.
Delete (remove): Removing an entry from the hash map generally takes around O(1) time on average as well.
However, in cases of collisions—when multiple keys hash to the same index—the time complexity can degrade to O(n) in the worst case.
Uses of Hash Maps
Hash maps have numerous practical applications across various domains:
Storing User Information: Websites and applications often use hash maps to store user profiles, enabling quick access to user data based on unique identifiers like usernames or email addresses.
Counting Frequencies: In data analysis, hash maps can efficiently count occurrences of items. For example, if you were analyzing sales data for different types of Nigerian snacks, you could use a hash map to tally how many times each snack was sold.
Caching Results: Hash maps are commonly used in caching mechanisms to store previously computed results or the results of requests made regularly for quick retrieval. This is similar to how local restaurants keep popular dishes on standby during busy hours.
Implementation of Hash Maps in Multiple Languages
Here’s how hash maps can be implemented in various programming languages:
TypeScript
There is no actual implementation of hash maps in JavaScript however, the concept of it is similar to a map in JavaScript.
let hashMap = new Map<number, string>();
hashMap.set(1, "Jollof Rice Recipe");
hashMap.set(2, "Egusi Soup Recipe");
C
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int key;
char* value;
} HashMapEntry;
typedef struct {
HashMapEntry* entries;
int size;
} HashMap;
C++
#include <unordered_map>
#include <string>
std::unordered_map<int, std::string> hashMap;
hashMap[1] = "Jollof Rice Recipe";
hashMap[2] = "Egusi Soup Recipe";
Python
hash_map = {}
hash_map["Jollof Rice"] = "Recipe for Jollof Rice"
hash_map["Egusi Soup"] = "Recipe for Egusi Soup"
Java
import java.util.HashMap;
HashMap<String, String> recipes = new HashMap<>();
recipes.put("Jollof Rice", "Recipe for Jollof Rice");
recipes.put("Egusi Soup", "Recipe for Egusi Soup");
Advantages of Hash Maps (and Over Other Data Structures)
Hash maps offer several advantages:
Fast Access: They provide rapid access to values based on keys, making them more efficient than arrays or lists when it comes to searching for items.
Flexible Keys: Unlike arrays that require integer indices, hash maps allow for keys of various types—strings, numbers, or even objects—making them versatile.
Ease of Use: Most programming languages provide built-in support for hash maps with simple methods for adding and retrieving items.
Compared to other data structures such as arrays or linked lists, hash maps excel in scenarios where speed and flexibility are crucial.
Disadvantages of Hash Maps
Despite their advantages, hash maps also have some drawbacks:
Unordered Storage: The elements stored in a hash map do not maintain any specific order. If you need your items sorted—like organizing your favorite Nigerian dishes by preparation time—you may need additional steps.
Collisions: When multiple keys hash to the same index, it can lead to performance issues. This is similar to having too many people trying to enter a popular market at once; it can slow down access significantly.
Memory Consumption: Hash maps may consume more memory than necessary due to their underlying structure designed to minimize collisions and maintain efficiency.
Conclusion
In summary, hash maps are powerful tools that facilitate efficient storage and retrieval of data through key-value pairs. Their applications range from web development to data analysis and beyond. While they offer significant advantages over other data structures, understanding their limitations is essential for effective implementation in programming tasks.
Subscribe to my newsletter
Read articles from Kingsley Ihemelandu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
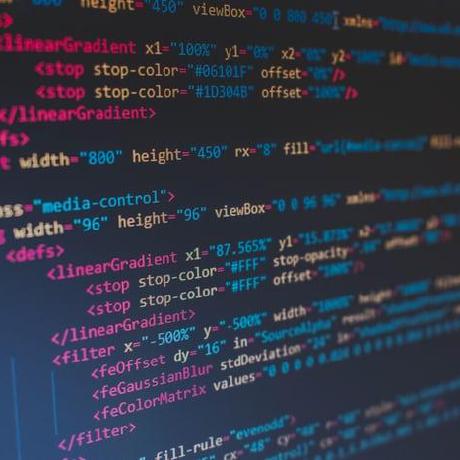