What is a JIT Compiler?

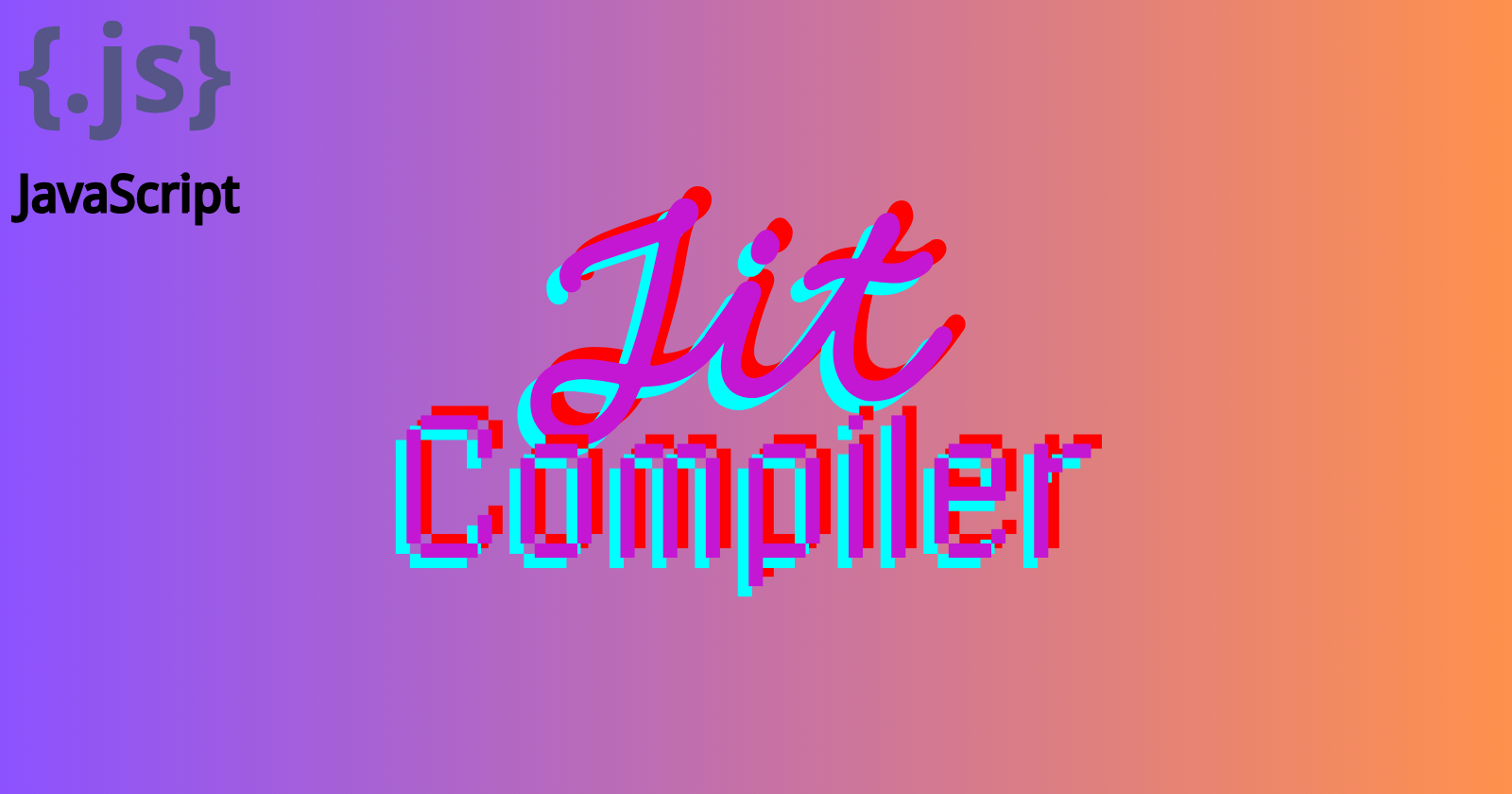
A JIT (Just-In-Time) compiler is a part of the runtime environment that improves the performance of applications by converting bytecode or intermediate code into native machine code just before execution. This process is done on-the-fly, during the execution of the program, rather than beforehand. The JIT compiler is used to optimize the code for the specific hardware it is running on, resulting in faster execution.
In the context of JavaScript, the JIT compiler is integrated into JavaScript engines like V8 (used by Node.js and Google Chrome) to dynamically compile JavaScript code into optimized machine code. This compilation happens in real-time as the code is being run, enabling significant performance improvements.
How Does the JIT Compiler Work?
The JIT compiler operates in several steps:
Parsing and Tokenizing: The JavaScript code is parsed and tokenized into an Abstract Syntax Tree (AST), representing the syntactic structure of the code.
Bytecode Generation: The AST is then converted into an intermediate representation known as bytecode. Bytecode is a low-level, platform-independent representation of the code, which is easier to execute than high-level code.
Dynamic Analysis and Optimization: The JIT compiler monitors the execution of the bytecode, collecting data on the types of variables, frequently executed paths, and other runtime information. Based on this analysis, the JIT compiler can optimize the code. For example, if a variable is consistently used as an integer, the JIT compiler may optimize operations on that variable for integer use.
Machine Code Generation: Once optimizations are identified, the JIT compiler translates the bytecode into machine code, which is the native code specific to the host CPU architecture. This machine code can be executed directly by the processor.
Execution and Optimization Feedback: The generated machine code is executed. The JIT compiler continues to monitor and may further optimize the code based on new runtime information, continuously improving performance.
Types of JIT Compilation
Baseline JIT Compilation: Compiles code quickly with minimal optimizations. It focuses on reducing the time from code parsing to execution.
Optimizing JIT Compilation: Once baseline JIT has collected enough profiling data, the optimizing JIT compiler kicks in, applying more advanced optimizations like inlining functions, loop unrolling, and more.
JIT Compiler's Usefulness in Node.js
The JIT compiler plays a critical role in enhancing the performance of Node.js applications by optimizing JavaScript code during execution. Here's how it benefits Node.js:
Improved Performance: JIT compilation allows JavaScript code to run almost as fast as native machine code. By converting frequently executed code paths into optimized machine code, the JIT compiler reduces execution time, leading to faster application performance.
Dynamic Optimization: Since Node.js applications are often long-running (e.g., web servers), they can benefit significantly from the JIT compiler’s ability to optimize based on real-time execution patterns. The JIT compiler can make the code more efficient based on how it is used, improving the performance of the server over time.
Efficient Use of Resources: By dynamically optimizing code, JIT compilation can reduce CPU usage, which is crucial for server environments. This efficiency helps in handling more requests and scaling applications effectively.
Support for Modern JavaScript Features: JIT compilers are designed to support the latest JavaScript features and standards. This ensures that Node.js can run modern JavaScript code efficiently, taking advantage of the latest language improvements.
Adaptive Execution: Node.js applications can handle a wide range of workloads. The JIT compiler adapts to the nature of the workload, optimizing the code paths that are used the most and minimizing the overhead for rarely executed code.
Example: How JIT Compilation Works in V8 for Node.js
When a Node.js application is executed, the V8 engine initially parses the JavaScript code and generates bytecode. As the application runs, the V8 JIT compiler monitors the code's execution, looking for "hot spots" or frequently executed code paths. Once these hot spots are identified, the JIT compiler generates optimized machine code for these paths.
For example, if a Node.js web server frequently handles a specific type of request, the JIT compiler will optimize the request-handling code. Subsequent requests of the same type will be processed faster because they will be executed using the optimized machine code, rather than interpreting the bytecode each time.
Conclusion
The JIT compiler is a cornerstone of modern JavaScript engines, including V8, and plays a vital role in the performance of Node.js applications. By dynamically compiling and optimizing JavaScript code into machine code, the JIT compiler ensures that Node.js can handle high-performance, scalable applications efficiently. This capability makes Node.js a powerful choice for building fast, responsive, and scalable server-side applications.
Subscribe to my newsletter
Read articles from SANKALP HARITASH directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

SANKALP HARITASH
SANKALP HARITASH
Hey 👋🏻, I am , a Software Engineer from India. I am interested in, write about, and develop (open source) software solutions for and with JavaScript, ReactJs. 📬 Get in touch Twitter: https://x.com/SankalpHaritash Blog: https://sankalp-haritash.hashnode.dev/ LinkedIn: https://www.linkedin.com/in/sankalp-haritash/ GitHub: https://github.com/SankalpHaritash21 📧 Sign up for my newsletter: https://sankalp-haritash.hashnode.dev/newsletter