Integrating AWS X-Ray in Long-Running Processes: Avoiding Resource Exhaustion
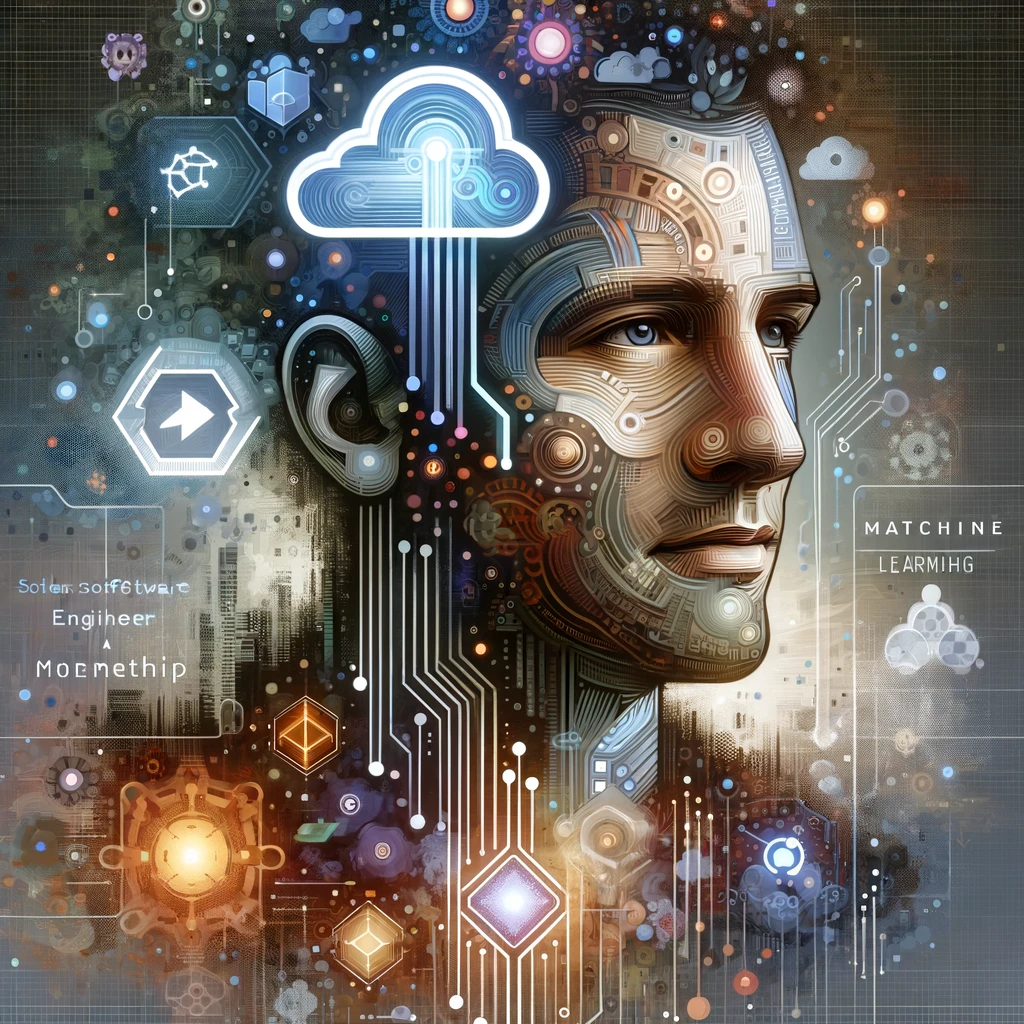
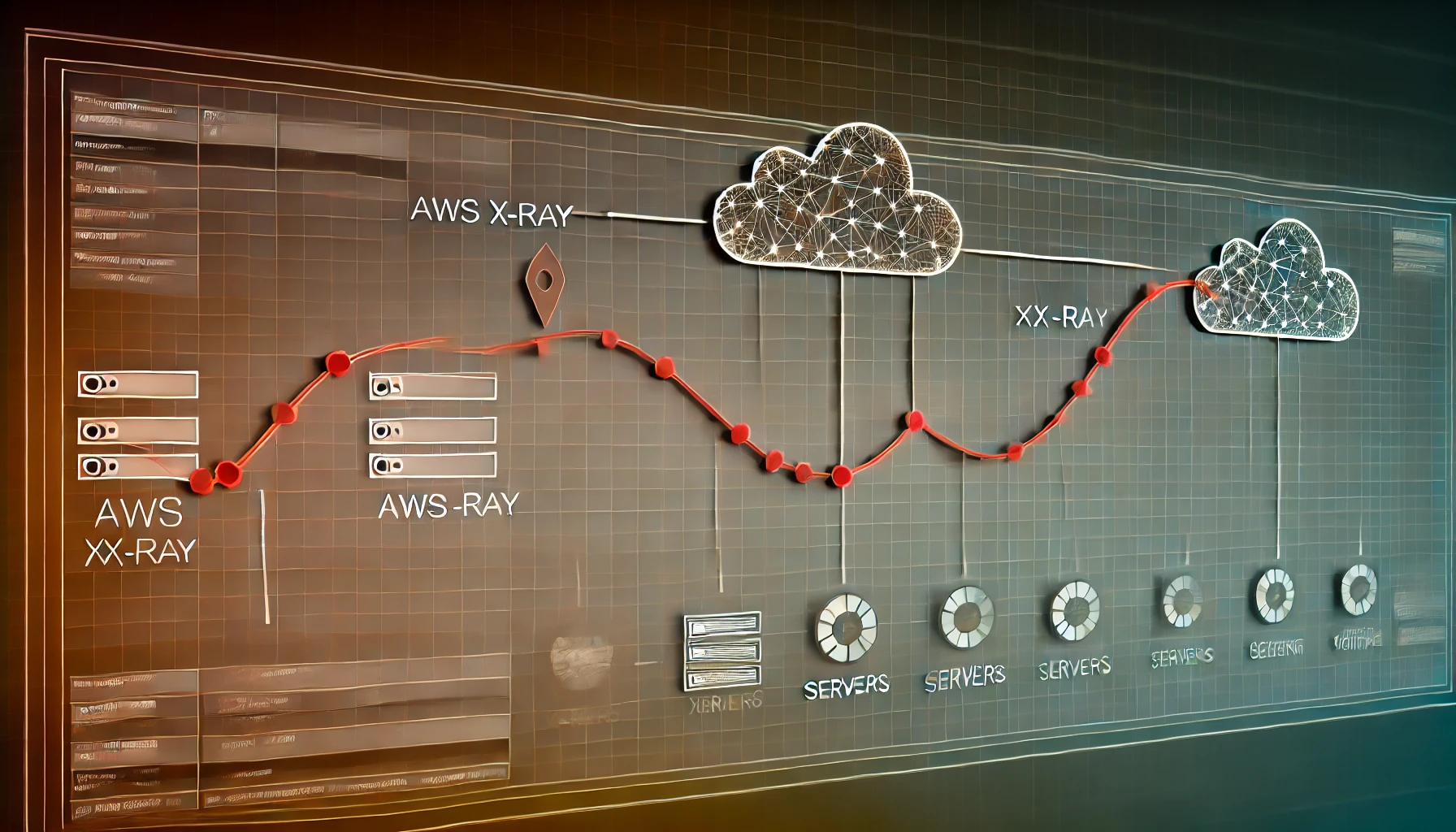
Introduction:
AWS X-Ray is a useful tool for gaining insights into distributed systems by tracing requests across different services. However, when integrating X-Ray into long-running processes, improper usage can lead to resource exhaustion. This article discusses how to efficiently integrate X-Ray with long-running processes, avoiding common pitfalls and managing system resources wisely.
Key Concepts of AWS X-Ray
Before diving into the specifics of long-running processes, it is essential to understand the basic components of AWS X-Ray:
Segments: A segment represents a unit of work, typically covering the work done by a service or application component.
Subsegments: Subsegments provide more detailed tracking within a segment. For example, a segment might represent the execution of a web service, while subsegments capture the individual calls made within that service (e.g., API calls or database queries).
Traces: A trace follows a request as it passes through various services, comprising multiple segments. Traces provide an end-to-end view of how a request flows through a system.
Annotations and Metadata: Annotations are indexed key-value pairs used for querying traces, while metadata provides additional, non-indexed information. Annotations are useful for filtering, while metadata provides context for troubleshooting.
Sampling: AWS X-Ray uses sampling to trace a subset of requests instead of capturing all of them. This is particularly useful for reducing overhead in high-traffic or long-running applications.
For more details, see the AWS X-Ray Documentation.
Problem with X-Ray in Long-Running Processes
When integrating X-Ray into long-running processes, one of the main challenges is how it handles subsegments. X-Ray does not commit subsegments until the parent segment is ended. If a segment stays open for an extended period, all the subsegments accumulate in memory. This leads to excessive use of CPU and memory resources as the process continues, ultimately causing resource exhaustion.
In a long-running task like an ETL job or background service, leaving a segment open for too long without committing subsegments will result in significant performance degradation. Over time, X-Ray will consume too many resources, slowing down or even crashing the system.
Basic Example of X-Ray Usage
Here is a generic example to illustrate how X-Ray is typically used to trace the flow of requests in an application:
pythonCopy codeimport aws_xray_sdk.core as xray
# Start a new segment
segment = xray.begin_segment('MyApplication')
try:
# Start subsegments to track specific tasks
xray.begin_subsegment('Task_A')
# Simulate task A
perform_task_a()
xray.end_subsegment()
xray.begin_subsegment('Task_B')
# Simulate task B
perform_task_b()
xray.end_subsegment()
finally:
# End the main segment
xray.end_segment()
This example demonstrates starting and ending segments and subsegments. The segment encompasses the entire process, while subsegments provide more granular insight into specific parts of the process. This basic flow helps in understanding the general usage of X-Ray for tracing.
Solutions to Avoid Resource Exhaustion
When integrating AWS X-Ray with long-running processes, several best practices can be employed to manage resource usage efficiently:
Apply Sampling Rules Sampling is a critical aspect of preventing X-Ray from overloading your system. Sampling allows you to trace a subset of requests, reducing the amount of data collected while still providing insights into system behavior.
Example: Configuring X-Ray Sampling Rules
pythonCopy codefrom aws_xray_sdk.core import xray_recorder # Custom sampling rule: Trace only 1% of requests, fixed target of 1 request per second sampling_rules = { "rules": [ { "description": "Sample 1% of requests", "http_method": "*", "url_path": "*", "fixed_target": 1, # Trace 1 request per second "rate": 0.01 # Trace 1% of additional requests } ], "default": { "fixed_target": 1, "rate": 0.01 } } # Set the sampling rules xray_recorder.configure(sampling_rules=sampling_rules)
Explanation of Sampling:
Fixed Target = 1: This means X-Ray will trace at least 1 request per second, regardless of the traffic volume.
Rate = 0.01: For every additional request beyond the fixed target, X-Ray will trace 1% of the traffic. So, if a service handles 1000 requests per second (TPS), X-Ray will trace 1 request per second due to the fixed target, and trace an additional 10 requests per second (1% of the remaining 999).
Scenario Example:
If a service processes 1000 TPS and runs for 10 seconds, the total number of requests during this time is 10,000. With a fixed target of 1 and a rate of 0.01, X-Ray would trace approximately 10 requests per second (1 fixed + 9 sampled), resulting in around 100 traces over the 10 seconds.
Break Down Long-Running Segments Instead of keeping a single segment open for the entire duration of a long-running process, break it into smaller units. Close the segments after a logical piece of work is done, such as completing a batch in an ETL process.
Example: Breaking Down Long-Running Segments
pythonCopy code# Start and close segments periodically within a long-running process for batch in data_batches: segment = xray.begin_segment(f'Batch_{batch.id}') try: # Process the batch process_batch(batch) finally: xray.end_segment() # Close the segment after processing each batch
This technique ensures that segments don't accumulate excessive amounts of data, keeping resource usage in check.
Reduce Subsegment Usage Limit the number of subsegments created in long-running processes. Focus on tracing only the most critical parts of the process, such as external service calls or database queries, rather than every function or action.
Optimize Annotations and Metadata Annotations, being indexed, can significantly increase resource usage if overused. Use annotations sparingly and rely on metadata for non-essential information that doesn't need to be indexed or queried frequently.
Conclusion
AWS X-Ray provides valuable insights into distributed systems, but integrating it into long-running processes requires careful planning to avoid resource exhaustion. By applying sampling rules, breaking down segments, and limiting subsegment usage, developers can optimize X-Ray for long-running tasks without overwhelming their systems. Proper management of X-Ray ensures that it remains a helpful tool for monitoring and performance tuning without causing unintended side effects.
For more details on X-Ray concepts and advanced configurations, visit the AWS X-Ray Documentation.
Subscribe to my newsletter
Read articles from Denny Wang directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
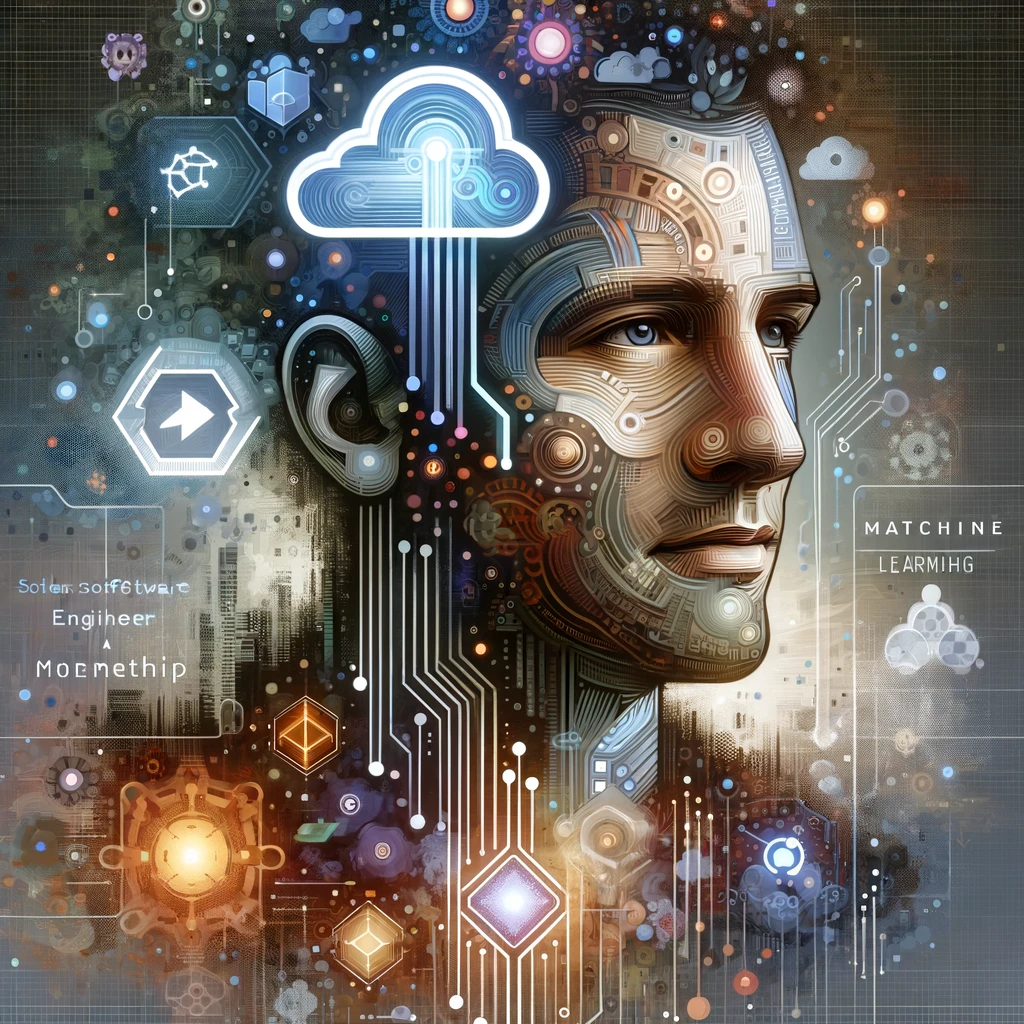
Denny Wang
Denny Wang
I'm Denny, a seasoned senior software engineer and AI enthusiast with a rich background in building robust backend systems and scalable solutions across accounts and regions at Amazon AWS. My professional journey, deeply rooted in the realms of cloud computing and machine learning, has fueled my passion for the transformative power of AI. Through this blog, I aim to share my insights, learnings, and the innovative spirit of AI and cloud engineering beyond the corporate horizon.