The Power of TypeScript: Why JavaScript Developers Should Make the Switch
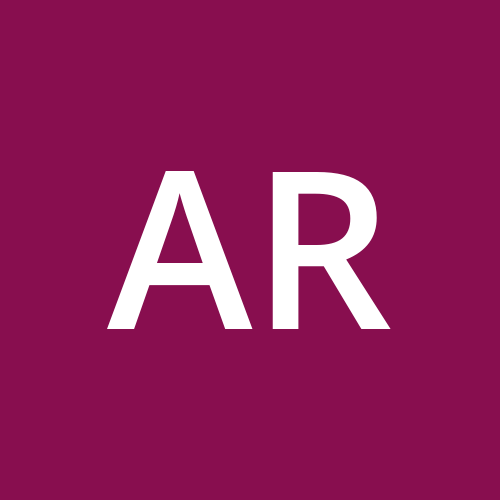
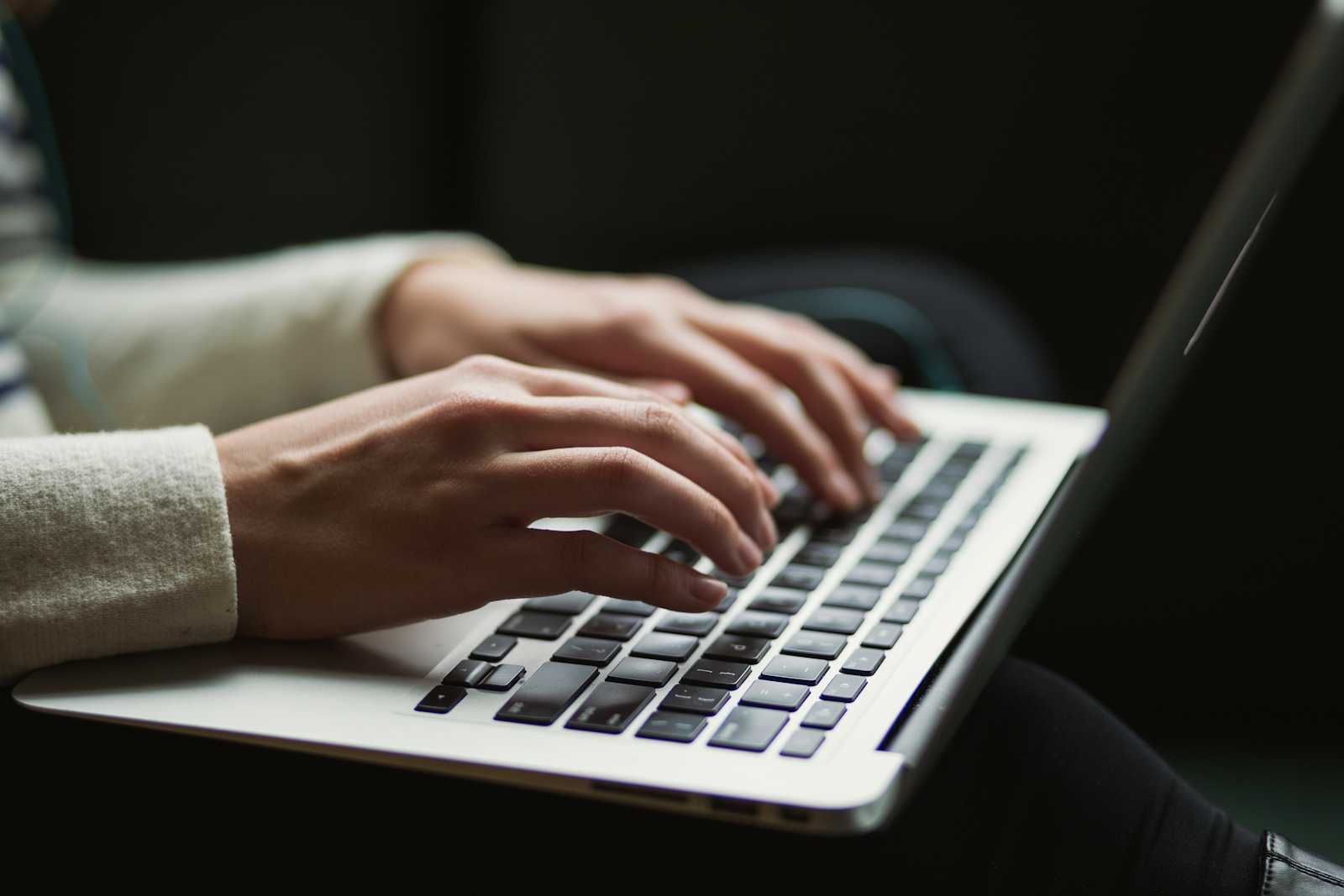
As JavaScript developers, we’re all familiar with the flexibility and simplicity of the language. It’s what makes JavaScript so versatile, powering both the front-end and back-end of web applications. However, as our projects grow larger and more complex, the language’s dynamic nature can start to work against us. We’ve all encountered those frustrating runtime errors or bugs caused by unexpected types, and without compile-time checks, these issues often slip through unnoticed.
Enter TypeScript: a strongly typed superset of JavaScript that addresses many of these pain points. With TypeScript, you can catch errors early, improve your code’s maintainability, and enjoy enhanced tooling and collaboration. If you’re still wondering whether to make the switch, here are some compelling reasons why TypeScript is worth considering.
Type Safety: Catch Errors Early
JavaScript is a dynamically typed language, meaning variables can be assigned any type of value at any point in your code. While this flexibility allows rapid prototyping, it also opens the door to runtime errors that can break your application. Imagine assigning a number to a variable that was supposed to hold a string, and not realizing it until that variable is used somewhere unexpected.
TypeScript introduces static types to JavaScript, allowing you to define the types of variables, function arguments, and return values upfront. This makes your code more predictable and easier to debug. For example:
// JavaScript function add(a, b) { return a + b; }
add(5, "10"); // Returns "510", an unintended result
With TypeScript, you’d catch this mistake early:
function add(a: number, b: number): number { return a + b; }
// Compilation error: Argument of type 'string' is not assignable to parameter of type 'number' add(5, "10");
In small projects, you might get away with a few unchecked type mismatches, but as your application grows, the risk increases. Type safety becomes crucial when maintaining large codebases, where changes made in one part of the application could have unintended consequences elsewhere. TypeScript ensures that as you scale your project, you retain consistency and reduce potential bugs.
What’s great about TypeScript is that it doesn’t force you to define types everywhere. Type inference automatically detects the types of variables when they are initialized, so you get the benefits of type safety without excessive overhead. For example:
let count = 5; // TypeScript infers that 'count' is a number.
count = "five"; // Error: Type 'string' is not assignable to type 'number'.
Improved Developer Experience
One of TypeScript’s biggest strengths is how it integrates seamlessly with modern editors and IDEs like Visual Studio Code. Thanks to type annotations and the type system, your editor can provide real-time autocompletion, inline documentation, and intelligent suggestions based on your code. These features make development faster, smoother, and less error-prone.
For example, consider the power of autocompletion in TypeScript:
interface User {
name: string;
age: number;
email: string;
}
const user: User = { name: "Alice", age: 25, email: "alice@example.com" };
// Autocomplete when accessing properties
console.log(user.nam); // TypeScript will suggest 'name' and flag 'nam' as incorrect.
When typing user.nam
, TypeScript immediately flags this as a typo and suggests the correct property name
. This immediate feedback saves time and reduces the risk of runtime errors caused by simple mistakes like typos.
Intelligent Refactoring: Refactoring is a common task in any development project, and TypeScript makes it significantly safer. If you rename a variable, function, or class in TypeScript, the IDE will automatically update all instances of that entity across your codebase. This prevents issues where a change in one part of your code could break other areas that depend on it, a common problem in JavaScript projects.
For example, if you refactor a function name from getUser
to fetchUser
, TypeScript and your IDE will update every reference to that function across the project, ensuring consistency.
Better Tooling and Debugging
JavaScript has grown immensely since its inception, and so has its ecosystem of tools. TypeScript leverages these tools, giving you all the power of modern JavaScript along with enhanced debugging capabilities. The TypeScript compiler flags potential errors before you even run the code, allowing you to focus on building instead of troubleshooting. It also works seamlessly with linters, build tools, and frameworks, adding an extra layer of reliability to your projects.
TypeScript also improves the debugging experience significantly. Since TypeScript is compiled to JavaScript, it generates more meaningful stack traces, allowing you to trace bugs back to the source more easily. Additionally, TypeScript’s static analysis narrows down potential issues by checking types ahead of time, so when you do encounter an error, there are fewer places to look for the root cause.
Enhanced Code Readability and Maintainability
When you’re working on a large project or collaborating with others, having clearly defined types can make all the difference. JavaScript’s dynamic typing can sometimes lead to ambiguity, where it’s unclear what kind of data is expected or returned by a function. TypeScript enforces structure by requiring you to explicitly define types for variables, function arguments, and return values. This not only reduces ambiguity but also ensures that your logic is more explicit, predictable, and easier to follow.
One of TypeScript’s most underrated benefits is that it makes your code self-documenting. Instead of relying on comments to explain what type of data a function expects or returns, you can use type annotations to clearly communicate intent:
// Without TypeScript (JavaScript)
function getUser(id) {
return fetch(`/api/user/${id}`)
.then(res => res.json())
.then(data => data);
}
// With TypeScript
function getUser(id: number): Promise<User> {
return fetch(`/api/user/${id}`)
.then(res => res.json())
.then((data: User) => data);
}
In the TypeScript version, it’s clear that id
is expected to be a number, and the function will return a Promise
that resolves to a User
object. This level of clarity is invaluable when collaborating with a team or revisiting the code later on.
Reducing Bugs and Ambiguity: In large codebases, it’s common to make incorrect assumptions about what types a function or variable will accept. This can lead to subtle bugs that are difficult to track down. TypeScript helps eliminate this ambiguity by enforcing type consistency across the entire project. If a function expects a certain type, you can be confident that it won’t receive anything unexpected. This proactive error prevention is particularly useful in complex applications with many interconnected components.
Compatibility with JavaScript Ecosystem
One of the best things about TypeScript is that it doesn’t require you to completely abandon JavaScript. Since TypeScript is a superset of JavaScript, any valid JavaScript code is also valid TypeScript code. This means you can introduce TypeScript incrementally, without having to rewrite your entire codebase. You can start by renaming .js
files to .ts
and adding type annotations to a few critical parts of your application, allowing you to ease into the TypeScript ecosystem gradually.
Gradual Adoption: TypeScript’s flexibility makes it easy to introduce on a project-by-project basis or even within specific parts of a large application. If you’re working in an existing JavaScript codebase, you can continue to write JavaScript in files that don’t require type checking, while leveraging TypeScript in more complex areas where type safety is crucial. Over time, as your team becomes more familiar with TypeScript, you can gradually introduce more types across your codebase without disrupting development workflows.
For example, you could start by simply adding TypeScript to a single module:
// Rename `file.js` to `file.ts`
function greet(name) {
return `Hello, ${name}!`;
}
From there, you can add types to improve safety and readability:
function greet(name: string): string {
return `Hello, ${name}!`;
}
Seamless Integration with Frameworks and Libraries: TypeScript works seamlessly with all major JavaScript libraries and frameworks, including React, Vue, Angular, Node.js, and more. Many popular libraries, such as React and Express, already provide built-in TypeScript type definitions, so you can start using TypeScript without any extra setup. This means that if you're already using JavaScript frameworks or third-party libraries, you don’t have to worry about compatibility issues—TypeScript fits in smoothly.
Future-Proof Your Code
JavaScript is evolving fast, and keeping up with the latest language features can be challenging, especially when different browsers or runtimes lag behind in supporting new syntax. TypeScript helps future-proof your code by allowing you to use cutting-edge JavaScript features before they are fully supported across all environments. By transpiling TypeScript into backward-compatible JavaScript, TypeScript ensures that your code remains compatible with a wide range of browsers and runtimes without sacrificing modern functionality.
TypeScript closely follows the ECMAScript standard (the specification for JavaScript), meaning you can take advantage of the latest language features as soon as they are proposed or finalized. For example, TypeScript introduced support for optional chaining (?.
) and nullish coalescing (??
) long before they were natively supported in most browsers. Here’s an example of how TypeScript lets you use these features safely, while ensuring compatibility:
const user = { name: "Alice", address: null };
// Optional chaining in TypeScript
console.log(user?.address?.city); // Avoids runtime errors if 'address' is null
// Nullish coalescing in TypeScript
const displayName = user.name ?? "Anonymous";
console.log(displayName); // Outputs 'Alice'
With TypeScript, you can use these advanced features now, and the TypeScript compiler will generate code that runs smoothly in environments that do not yet support them.
TypeScript transpiles your modern TypeScript code into widely compatible JavaScript that runs in older browsers or environments like Node.js. For instance, even if you use async/await or ES2021 modules in your TypeScript code, it will compile down to ES5 or ES6-compatible code, making sure that it works in older browsers or Node.js versions. This gives you the confidence to use the latest features without worrying about compatibility issues.
TypeScript allows you to try out new ECMAScript proposals even before they are officially part of the language. The TypeScript team works closely with the TC39 committee (the group responsible for evolving JavaScript), so developers can experiment with upcoming features and provide feedback to improve them. This proactive approach ensures that TypeScript is always one step ahead, giving developers early access to powerful new language features.
Conclusion
TypeScript offers a more robust and scalable solution to JavaScript’s dynamic nature. It helps developers catch errors early, improve productivity, and create maintainable, future-proof code. The best part? You don’t have to give up JavaScript to use it. If you’re a JavaScript developer looking to level up, TypeScript is worth the switch. Take the plunge and enjoy a more efficient, error-free coding experience!
If you found this post helpful, please give it a like and share it with others who might benefit from it. Happy coding!
Subscribe to my newsletter
Read articles from Artur directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
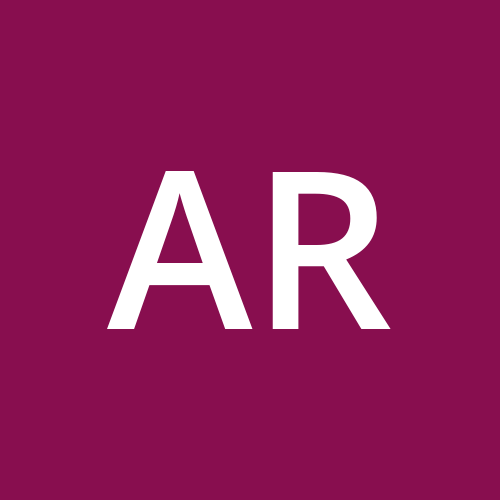