Decoding 'this' keyword in JavaScript

Table of contents
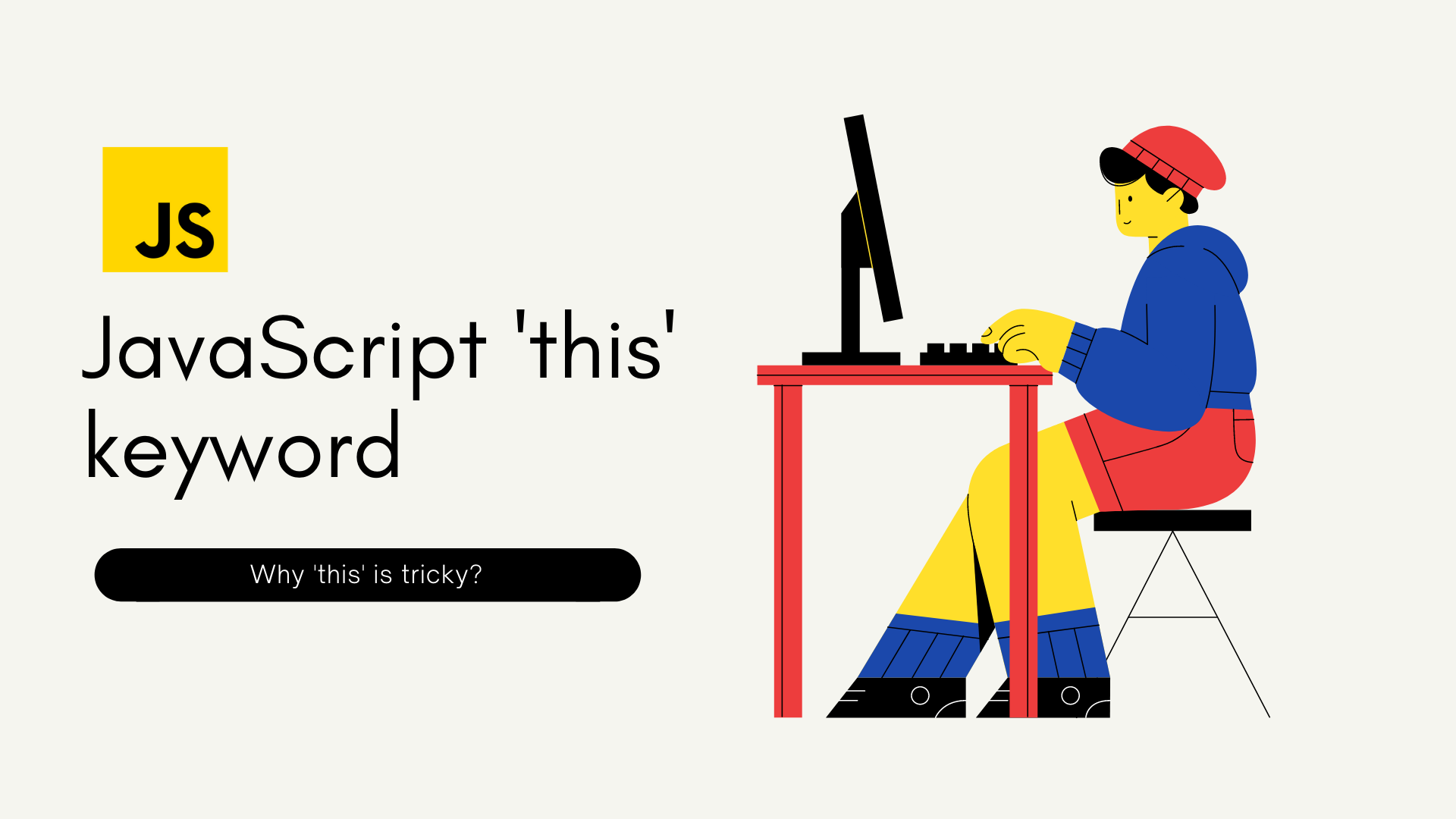
If you are new to the world of JavaScript, then you may find 'this' keyword a little bit tricky. In this blog, I will discuss some basics of 'this' keyword, how we can use and its problems.
What is 'this'?
Generally, this keyword refers to the object it belongs to or the parent object. Now, it also varies on which context we use it. Like, in a method, 'this' refers to its parent object. Alone, 'this' refers to the global object. In a function, 'this' refers to the global object. But in a function with 'strict' mode, 'this' refers to undefined. In an event, 'this' refers to the element that received the event. Ex:-
var person = {
fname: "Robert",
printName: function() {
console.log(this.fname);
}
}
person.printName(); // Robert
But sometimes it creates a lot of confusion. Ex:-
var person = {
id: 1,
greet: function() {
setTimeout(function() {
console.log(this.id);
}, 1000)
}
}
person.greet(); // undefined
When we are using 'this' in the setTimeout it creates its context for 'this'. So it isn't able to access 'this' of the outer scope and bound to its scope. As 'this' is not defined within the function, the output is undefined. There are two ways to resolve this problem.
Using another variable: We can create another variable inside the function and use that to access id.
var person = { id: 1, greet: function() { var self = this; setTimeout(function() { console.log(self.id); }, 1000) } } person.greet(); // 1
Using the arrow function: We can also use the arrow function to resolve this problem. As the arrow function doesn't create its own context of 'this', we can access the 'this' of its parent.
var person = { id: 1, greet: function() { setTimeout(() => console.log(this.id), 1000) } } person.greet(); // 1
Summary
this
keyword is tricky and sometimes feels complicated if you don’t understand the internal workings of it. But it surely gives your project many benefits if you use it properly. If you like my blogs and want to learn more about Software Engineering and Frontend Development follow me on hashnode.
Subscribe to my newsletter
Read articles from Debajit Mallick directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Debajit Mallick
Debajit Mallick
I am a Software Engineer with 3+ years of experience, currently at P360. I have a passion for creating intuitive web interfaces and actively contribute to tech communities as the Organizer of GDG Siliguri, ex-Microsoft Learn Student Ambassador, and former Hack Club Lead. As a tech speaker, I’ve presented at events like FrontendDays Siliguri, GDG Bhopal, and Azure DevDay. I’m also passionate about hackathons and open-source: Smart India Hackathon 2020 winner, HacktoberFest contributor, and mentor to the winning team of SIH 2022.