Color Detector using Python and OpenCV
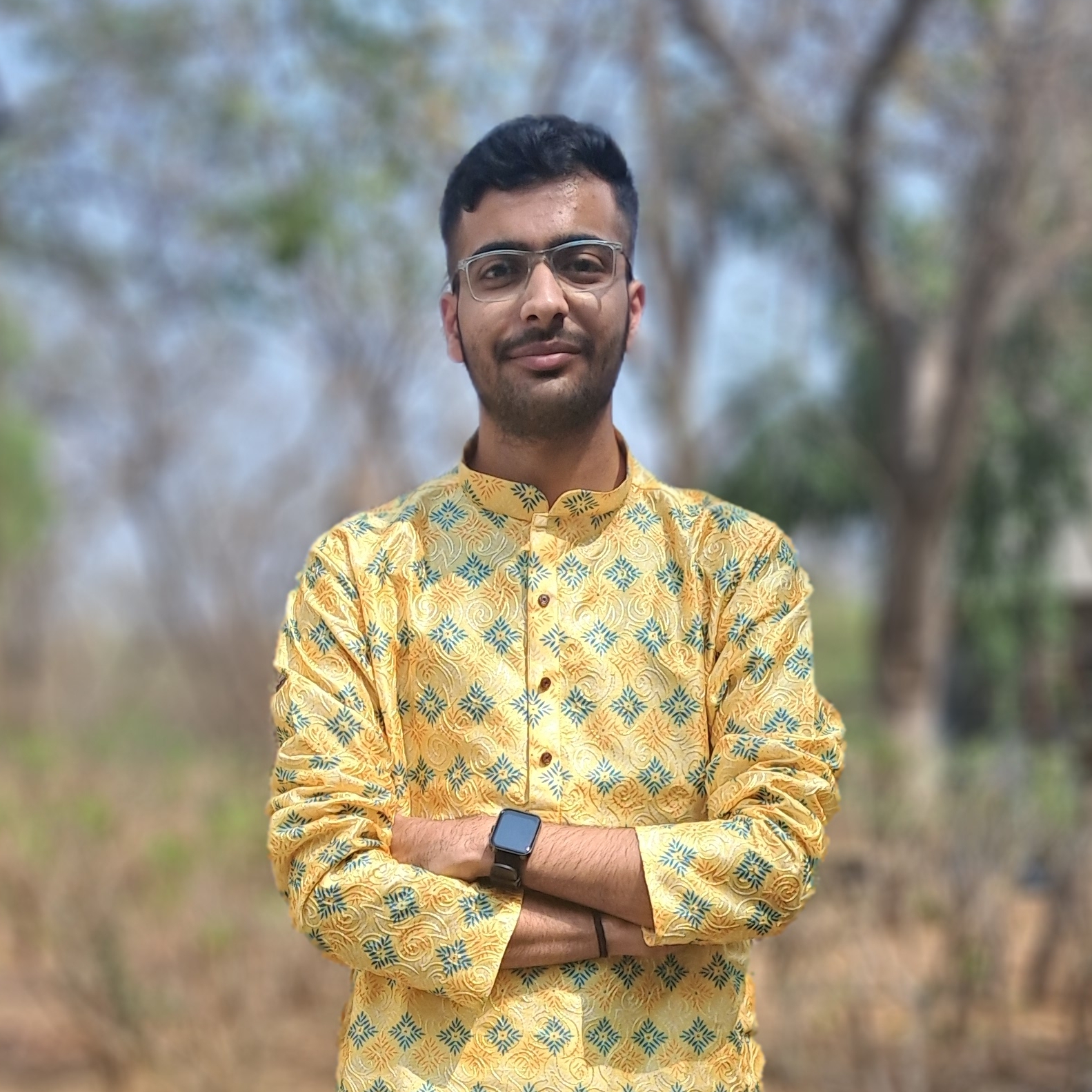
2 min read
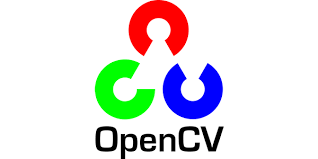
Here’s how you can make a color detector using Python and OpenCV :
Import all necessary libraries
import numpy as np import cv2 from PIL import Image
Select a color for detection
- For this project, we are detecting yellow color (yellow = [0,255,255])
Calculate the range of colors on the hue palette
def get_limits(color) : c = np.uint8([[color]]) hsvC = cv2.cvtColor(c, cv2.COLOR_BGR2HSV) lowerLimit = hsvC[0][0][0] - 10, 100, 100 upperLimit = hsvC[0][0][0] + 10, 255, 255 lowerLimit = np.array(lowerLimit, dtype=np.uint8) upperLimit = np.array(upperLimit, dtype=np.uint8) return lowerLimit, upperLimit
This function returns two values, the lowerLimit and upperLimit from the color palette, i.e. the range of the color.
Creating the structure of the project
yellow = [0, 255, 255]
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
hsvImage = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
lowerLimit, upperLimit = get_limits(color=yellow)
mask = cv2.inRange(hsvImage, lowerLimit, upperLimit)
mask_ = Image.fromarray(mask)
box = mask_.getbbox()
if box is not None:
x1, y1, x2, y2 = box
frame = cv2.rectangle(frame, (x1, y1), (x2, y2), (0,255,0), 2)
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
This code generates a window with real-time camera input and detects the specified color in real-time.
Complete code for the project :
import numpy as np
import cv2
from PIL import Image
def get_limits(color) :
c = np.uint8([[color]])
hsvC = cv2.cvtColor(c, cv2.COLOR_BGR2HSV)
lowerLimit = hsvC[0][0][0] - 10, 100, 100
upperLimit = hsvC[0][0][0] + 10, 255, 255
lowerLimit = np.array(lowerLimit, dtype=np.uint8)
upperLimit = np.array(upperLimit, dtype=np.uint8)
return lowerLimit, upperLimit
yellow = [0, 255, 255]
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
hsvImage = cv2.cvtColor(frame, cv2.COLOR_BGR2HSV)
lowerLimit, upperLimit = get_limits(color=yellow)
mask = cv2.inRange(hsvImage, lowerLimit, upperLimit)
mask_ = Image.fromarray(mask)
box = mask_.getbbox()
if box is not None:
x1, y1, x2, y2 = box
frame = cv2.rectangle(frame, (x1, y1), (x2, y2), (0,255,0), 2)
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
Peace ✌️
0
Subscribe to my newsletter
Read articles from Sanchit Pahurkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
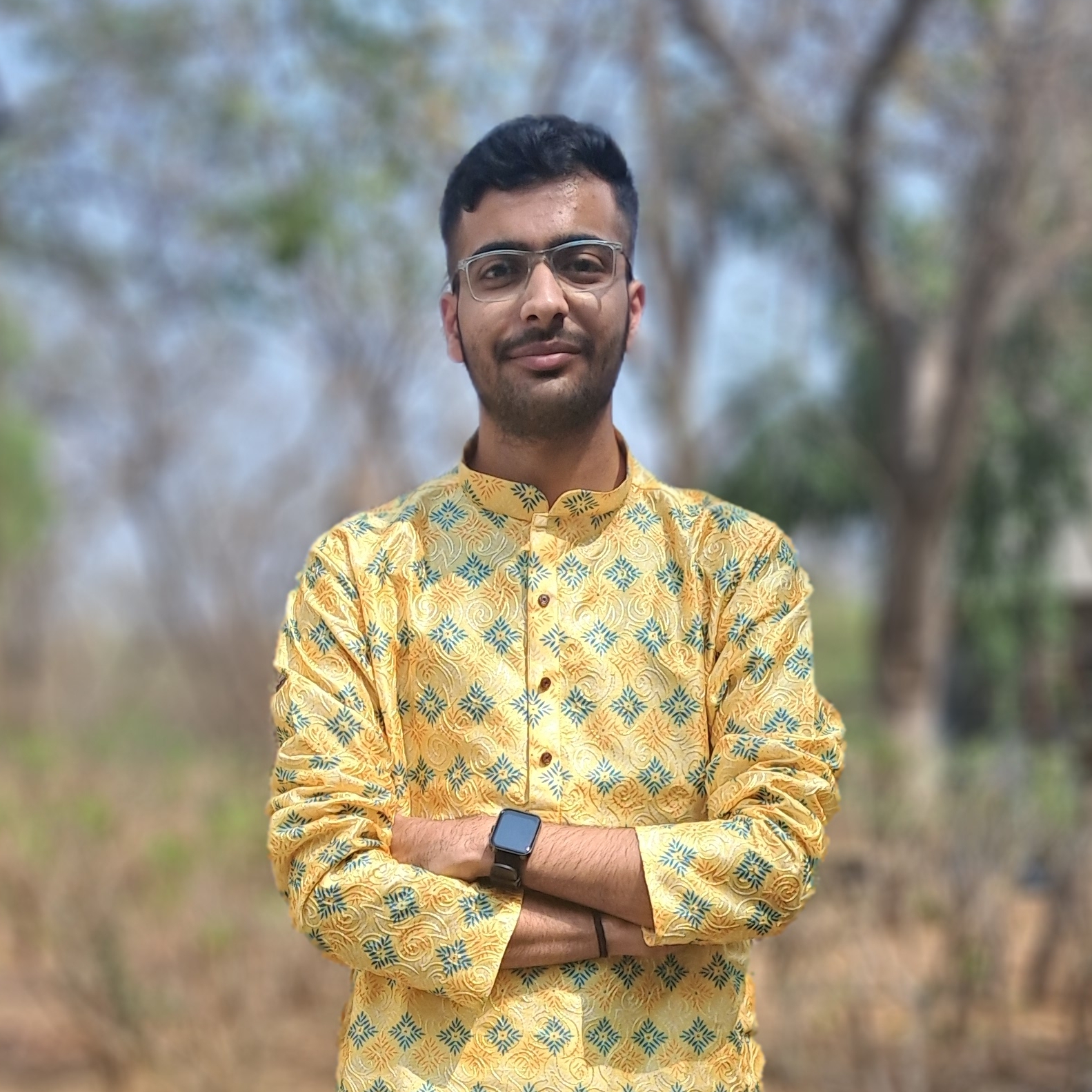