HashMap, Java

Table of contents
- Overview
- Hey there, let’s quickly go through Java HashMap, it is a powerful data structure that facilitates efficient storage and retrieval of key-value pairs. Java's HashMap is part of the java.util package and implements the Map interface. Unlike its predecessor Hashtable, HashMap is unsynchronized, allowing for faster performance in single-threaded scenarios.
- Characteristics
- Code Examples
- 1.Add an element
- 2. Modifying Elements
- 3. Removing Elements
- 4. Traversing HashMap
- Conclusion
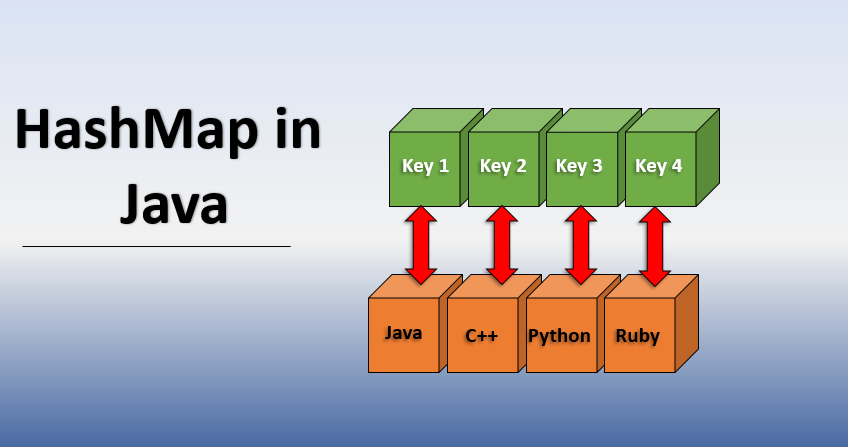
Overview
Hey there, let’s quickly go through Java HashMap, it is a powerful data structure that facilitates efficient storage and retrieval of key-value pairs. Java's HashMap
is part of the java.util
package and implements the Map
interface. Unlike its predecessor Hashtable
, HashMap
is unsynchronized, allowing for faster performance in single-threaded scenarios.
Characteristics
Key-Value Storage: Each entry in a
HashMap
consists of a unique key, which needs to be immutable, mapped to a specific value.Dynamic Resizing: When the number of entries exceeds the load factor and current capacity, the
HashMap
automatically resizes itself, typically doubling its capacity.Initial Size: Creates an empty map with an initial capacity of 16 and a load factor of 0.75.
Fast Access Time: The average time complexity for basic operations like insertion (
put()
) and retrieval (get()
) is O(1), assuming a well-distributed hash function.No Guaranteed Order: The order of entries is not guaranteed, which means that iterating over the keys or values may yield results in an unpredictable order.
Unsafe: HashMap is inherently not thread-safe, concurrent access by multiple threads can result in data inconsistencies. For multi-threaded environment, it is advisable to use
ConcurrentHashMap
, designed for concurrent access and provides better performance.
Code Examples
1.Add an element
import java.util.HashMap;
public class Example {
public static void main(String[] args) {
HashMap<Integer, String> map = new HashMap<>();
map.put(1, "One");
}
}
2. Modifying Elements
javamap.put(1, "Update One");
3. Removing Elements
javamap.remove(1);
4. Traversing HashMap
for (Map.Entry<Integer, String> entry : map.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
Conclusion
In summary, Java'sHashMap
provides a robust framework for managing collections of key-value pairs with high performance and flexibility. Its ability to handle null keys and values while maintaining fast access times makes it an essential tool in Java programming. However, developers should be mindful of its unsynchronized nature when working in multi-threaded environments.
Thanks there.
Subscribe to my newsletter
Read articles from Arpit Singh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Arpit Singh
Arpit Singh
AI engineer at Proplens AI, a final year student pursuing bachelor's in computer science and engineering.