Java Comments
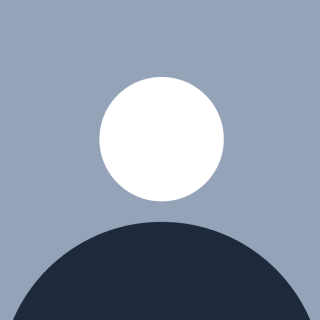
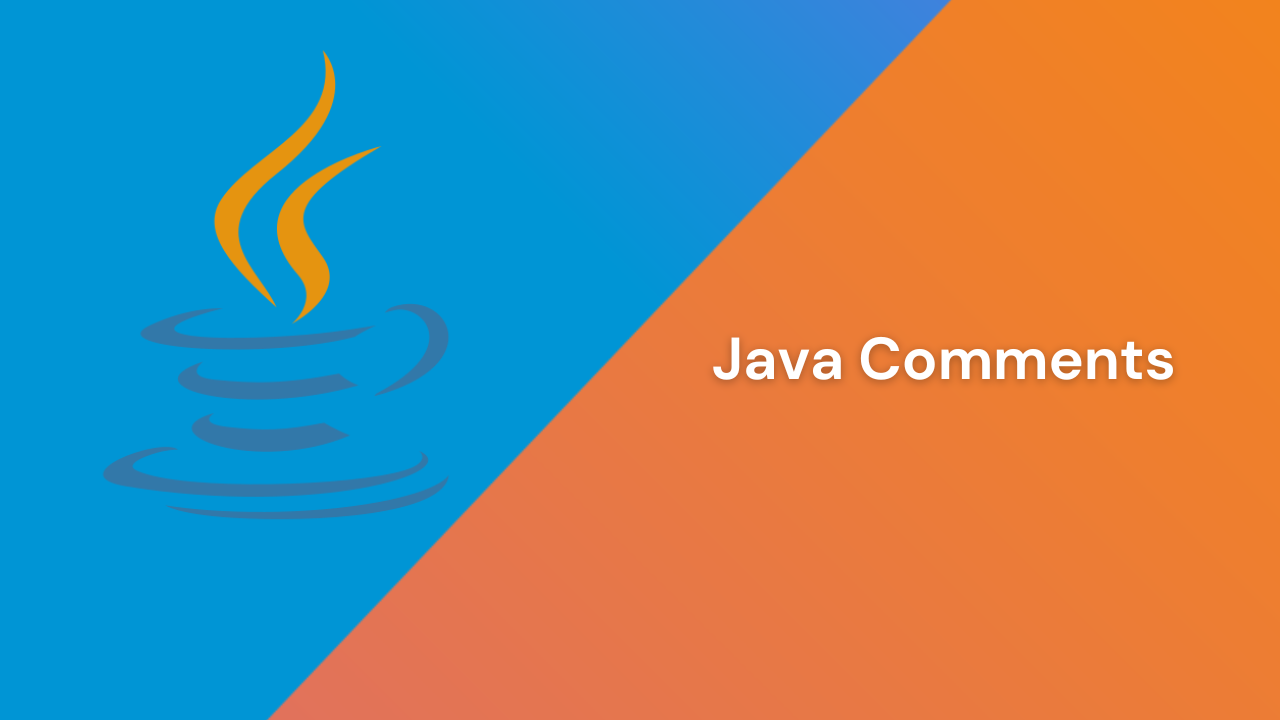
In Java, comments are used for code readability and documentation, ignored by the compiler. There are three types: single-line (//
), multi-line (/* ... */
), and documentation comments (/** ... */
). Documentation comments are processed by Javadoc to create HTML documentation. Comments are removed during the initial compilation phase and do not affect program execution.
Introduction
Comments in Java are purely for human readability and documentation purposes. They are ignored by the Java compiler during the compilation process, which means they do not translate into bytecode and have no impact on the program's execution.
Preprocessing: During the initial phase of compilation, known as preprocessing, the compiler scans the source code to identify and remove comments. This process is part of the lexical analysis stage, which is the first step in the compilation process. Although Java does not have a separate preprocessing phase like C/C++, the initial scanning of the source code can be considered a form of preprocessing. During this phase, the compiler reads through the entire source code to identify and handle comments, which are then removed before further processing.
Lexical Analysis: This stage involves breaking down the source code into smaller, manageable pieces called tokens. Tokens are the basic building blocks of the code, such as keywords, operators, identifiers, and literals. Comments are not part of the program's logic and are therefore not converted into tokens. Instead, they are discarded during this stage, as they are meant for human readability and documentation purposes only.
The source code, including comments, is typically written in a text format that uses a character encoding like ASCII or UTF-8. Character encoding is a system that maps characters to numbers, allowing the computer to understand and process text. This encoding is essential for the compiler to read and interpret the source code correctly. However, the encoding process itself is not specific to comments; it applies to the entire source code.
Comments serve to explain the code, provide context, and document the functionality of classes and methods. They are essential for code maintainability and collaboration among developers.
While regular comments are ignored by the compiler, Javadoc comments are processed by the Javadoc tool to generate HTML documentation. This tool extracts the comments and associated tags to create structured documentation, but this process is separate from the compilation of the code itself.
Types of Java Comments
There are three types of comments in Java:
Single-line Comments
Multi-line Comments
Documentation Comments
1. Single-line Comments
These comments are used for brief explanations or notes within the code. They begin with two forward slashes (//
). Everything following these slashes on that line is considered a comment and is ignored by the compiler.
Example:
int sum = a + b; // This line calculates the sum of a and b
2. Multi-line Comments
These comments are used for longer explanations that span multiple lines. They start with a forward slash followed by an asterisk (/*
) and end with an asterisk followed by a forward slash (*/
). Everything between these symbols is considered a comment.
Example:
/*
* This function calculates the factorial of a number.
* It uses a recursive approach to compute the result.
*/
public int factorial(int n) {
if (n <= 1) return 1;
else return n * factorial(n - 1);
}
3. Documentation Comments
These comments are used to generate external documentation and are written using a special format. They start with a forward slash followed by two asterisks (/**
) and end with an asterisk followed by a forward slash (*/
). These comments can include tags to describe classes, methods, parameters, and other elements of the code. Tools like Javadoc can process them to create HTML documentation.
Example:
/**
* Calculates the sum of two integers.
*
* @param a the first integer
* @param b the second integer
* @return the sum of a and b
*/
public int add(int a, int b) {
return a + b;
}
Documentation comments in Java, also known as Javadoc comments, are a special type of comment used to describe the functionality of classes, methods, and other elements in the code. These comments are processed by the Javadoc tool to generate HTML documentation, making it easier for developers to understand and use the code.
Javadoc Tags
Javadoc comments can include various tags to provide structured information about the code. Here are some commonly used Javadoc tags:
Tag | Syntax | Description |
{@docRoot} | {@docRoot} | Depicts the relative path to the root directory of the generated document from any page. |
@author | @author name | Adds the author of the class. |
{@code} | {@code text} | Displays text in code font without interpreting it as HTML markup or a nested Javadoc tag. |
@version | @version version-text | Specifies the "Version" subheading and version-text when the -version option is used. |
@since | @since release | Adds a "Since" heading with the specified text to the generated documentation. |
@param | @param parameter-name description | Adds a parameter with the given name and description to the 'Parameters' section. |
@return | @return description | Required for every method that returns something (except void). |
@throws | @throws exception-name description | Describes an exception that a method can throw. |
@see | @see reference | Provides a reference to another topic or element, creating a hyperlink in the documentation. |
@deprecated | @deprecated description | Marks a method, class, or field as deprecated, indicating it should no longer be used. |
@link | {@link reference} | Inserts an inline link to another element in the documentation. |
@inheritDoc | @inheritDoc | Inherits documentation from the nearest superclass or interface. |
Example using the Javadoc tags:
/**
* The Calculator class provides methods to perform basic arithmetic operations.
* <p>
* This class includes methods for addition, subtraction, multiplication, and division.
* </p>
*
* {@docRoot}
*
* @author John Doe
* @version 1.0
* @since 2024
*/
public class Calculator {
/**
* Adds two integers and returns the result.
*
* @param a the first integer
* @param b the second integer
* @return the sum of a and b
* @see #subtract(int, int)
*/
public int add(int a, int b) {
return a + b;
}
/**
* Subtracts the second integer from the first and returns the result.
*
* @param a the first integer
* @param b the second integer
* @return the result of a minus b
* @see #add(int, int)
*/
public int subtract(int a, int b) {
return a - b;
}
/**
* Multiplies two integers and returns the result.
*
* @param a the first integer
* @param b the second integer
* @return the product of a and b
* @throws ArithmeticException if an overflow occurs
*/
public int multiply(int a, int b) throws ArithmeticException {
return Math.multiplyExact(a, b);
}
/**
* Divides the first integer by the second and returns the result.
*
* @param a the dividend
* @param b the divisor
* @return the quotient of a divided by b
* @throws ArithmeticException if b is zero
* @deprecated Use {@link #safeDivide(int, int)} instead to handle division by zero.
*/
@Deprecated
public int divide(int a, int b) throws ArithmeticException {
return a / b;
}
/**
* Safely divides the first integer by the second and returns the result.
*
* @param a the dividend
* @param b the divisor
* @return the quotient of a divided by b, or 0 if b is zero
* @since 1.1
*/
public int safeDivide(int a, int b) {
if (b == 0) {
return 0; // Avoid division by zero
}
return a / b;
}
}
The Calculator
class in Java provides methods for basic arithmetic operations like addition, subtraction, multiplication, and division. It uses Javadoc comments to document each method, specifying parameters, return values, exceptions, and related methods. The divide
method is marked as deprecated, suggesting the use of safeDivide
to handle division by zero safely.
Are Java comments executable?
Ans: Java comments are not executed by the compiler or interpreter. However, before the compiler processes the code, the contents are encoded into ASCII to simplify processing.
Conclusion
In conclusion, comments in Java play a significant role in enhancing code readability and documentation. They are a valuable tool for developers to explain the logic, provide context, and document the functionality of the code. While comments are ignored by the compiler and do not affect program execution, they contribute to code maintainability and collaboration among developers. Understanding the different types of comments—single-line, multi-line, and documentation comments—enables developers to effectively document their code. Additionally, utilizing Javadoc comments and tags allows for the generation of comprehensive HTML documentation, further aiding in the understanding and usage of the code by others.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer