Java Packages
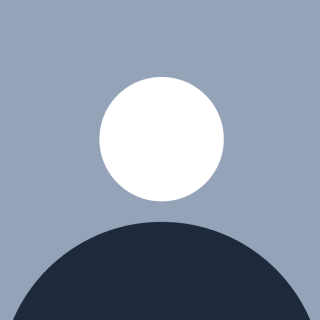
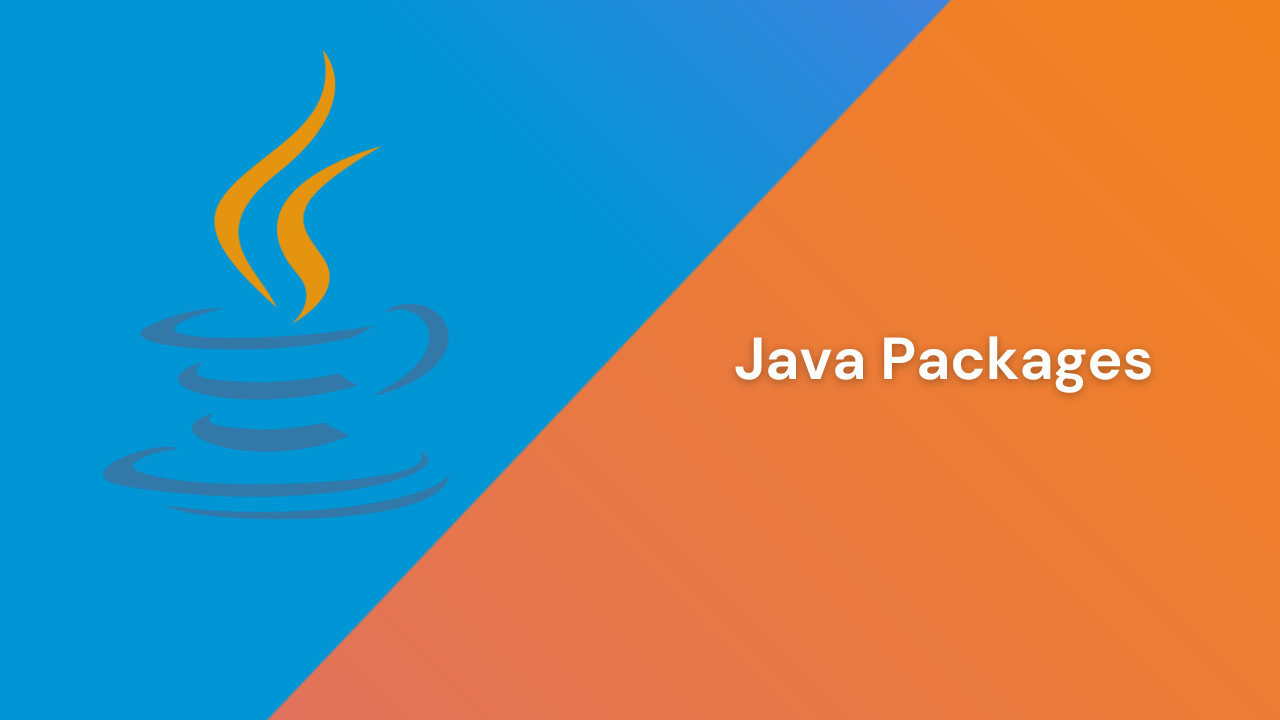
Java packages are essential for organizing and managing code in Java projects. They group related classes and interfaces, helping to avoid name conflicts, control access, and create a hierarchical structure for easier code management. Packages also enhance reusability across projects. To create a package, use the package
keyword, and to use classes from other packages, use the import
statement. It's best practice to define packages, even though Java allows a default package. Naming conventions include using reverse domain names and lowercase letters. Access modifiers like public, protected, default, and private determine accessibility within and across packages. Understanding packages is crucial for building scalable and maintainable Java applications.
What are Java Packages?
Java packages are similar to folders on your computer. Just as you organize files into folders, you organize Java classes and interfaces into packages. This helps in avoiding name conflicts and makes the code more modular and easier to manage.
Why Use Packages?
Namespace Management: Packages help in avoiding name conflicts. For example, you can have two classes with the same name in different packages.
Access Control: Packages provide a way to control access with the help of access modifiers. Classes in the same package can access each other's package-private members.
Organizational Structure: They help in organizing classes into a hierarchical structure, making it easier to locate and manage them.
Reusability: Packages allow you to reuse classes and interfaces across different projects.
Creating a Package
To create a package, you use the package
keyword at the top of your Java file. For example:
package com.example.tools;
public class Calculator {
// Class implementation
}
In this example, com.example.tools
is the package name. The directory structure should match the package name, with folders com
, example
, and tools
.
Importing Packages
To use a class from a different package, you need to import it. This is done using the import
statement:
import com.example.tools.Calculator;
public class Main {
public static void main(String[] args) {
Calculator calc = new Calculator();
// Use the calculator
}
}
You can also use a wildcard to import all classes from a package:
import com.example.tools.*;
Default Package
If you don't specify a package, the class goes into the default package. However, it's a good practice to always define a package, especially for larger projects.
Package Naming Conventions
Reverse Domain Name:It's common to use the reverse of your domain name as the package name to ensure uniqueness. For example, if your domain is
example.com
, your package might becom.example
.Lowercase Letters: Package names are typically written in all lowercase to avoid conflicts with class names.
Access Modifiers and Packages
Java provides four access levels: public
, protected
, default
(no modifier), and private
. Packages determine the accessibility of classes and their members.:
Public: Accessible from any other class.
Protected: Accessible within the same package and subclasses.
Default: Accessible only within the same package.
Private: Accessible only within the same class.
Conclusion
Java packages are essential for organizing code, managing namespaces, and controlling access. They help in building scalable and maintainable applications by providing a structured way to group related classes and interfaces.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer