Understanding JavaScript Closures: A Deep Dive
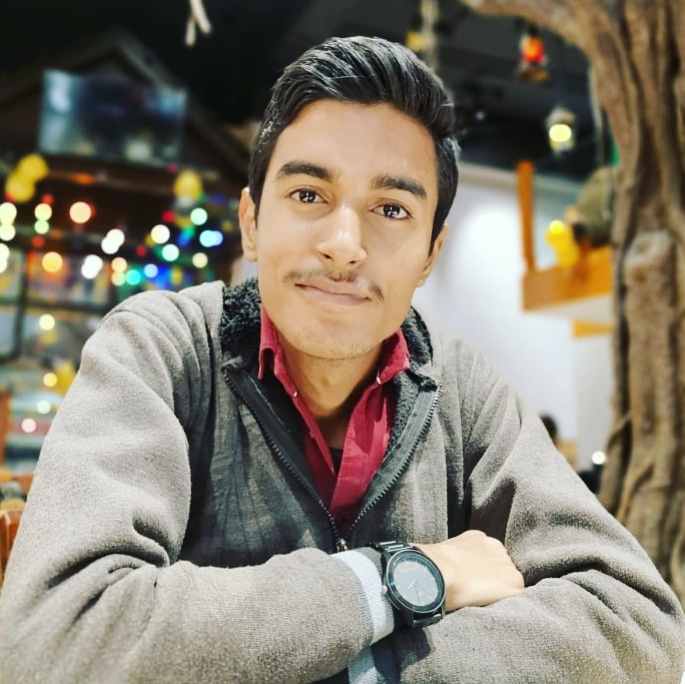
Table of contents
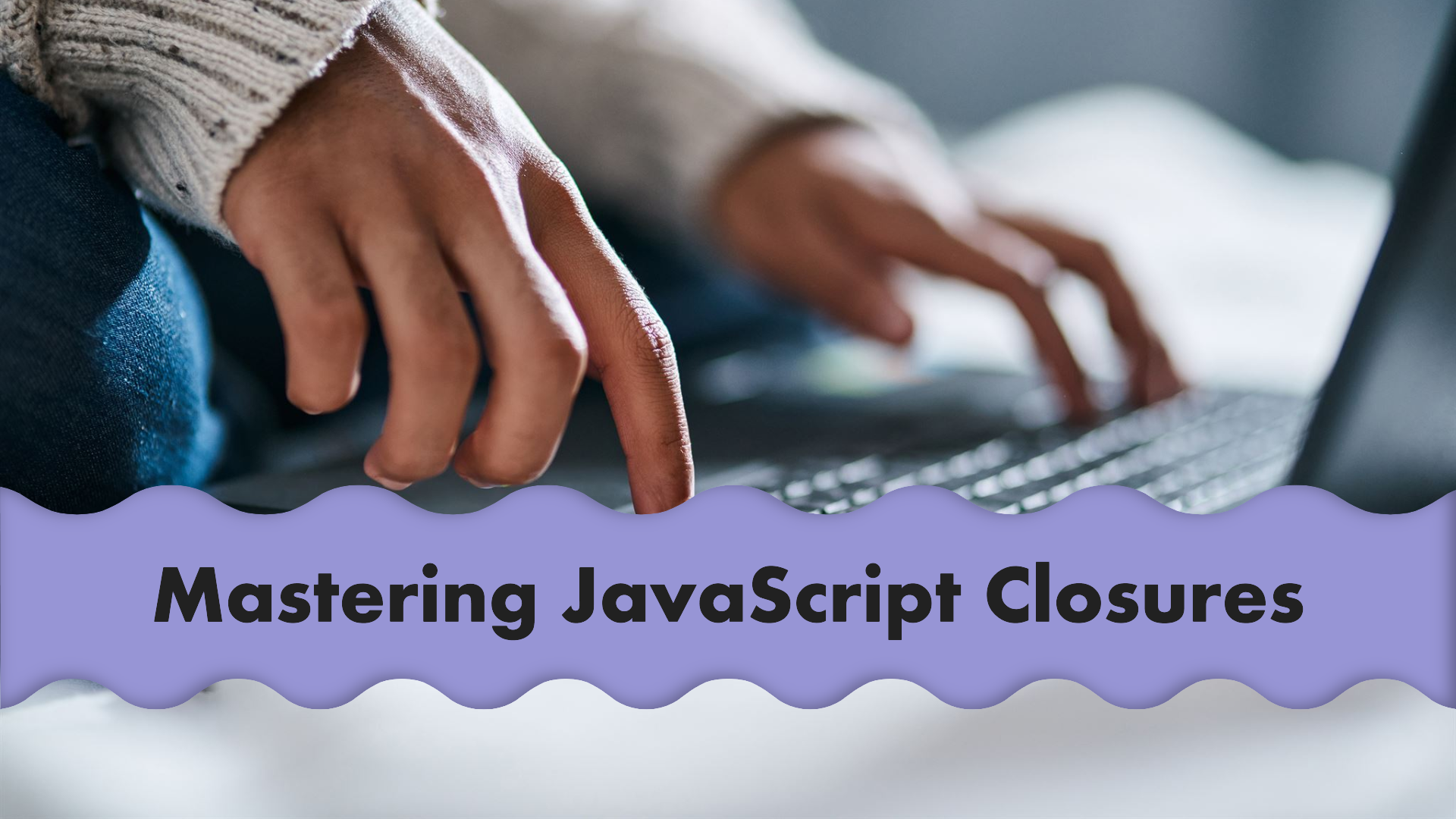
A closure in JavaScript is a function that has access to variables in its outer (lexical) scope, even after the outer function has returned. This concept is fundamental to understanding how JavaScript functions work and is often used to create private variables or implement design patterns like modules.
How Closures Work
When a function is defined, it creates a scope chain. This chain includes the function's own scope (local variables) and the scopes of its outer functions. When the function is executed, it looks for variables in its own scope first. If not found, it searches up the scope chain until it finds the variable or reaches the global scope.
A closure is created when a function is returned or passed as a reference while still having access to variables from its outer scope. The inner function becomes a closure, and its scope chain is preserved, allowing it to access the outer variables even after the outer function has finished executing.
Example of a Closure
JavaScript
function outerFunction() {
let x = 5;
function innerFunction() {
console.log(x); // Accesses the outer variable x
}
return innerFunction;
}
let closureFunction = outerFunction();
closureFunction(); // Output: 5
Use code with caution.
In this example, innerFunction
is a closure because it has access to the variable x
from the outer function outerFunction
. Even after outerFunction
returns, closureFunction
can still call innerFunction
, and it will have access to the original value of x
.
Use Cases of Closures
Creating Private Variables: Closures can be used to create private variables that are accessible only within the function and its inner functions. This helps in encapsulation and prevents accidental modification of variables.
Implementing Modules: Closures are often used to implement modules in JavaScript, providing a way to organize code into reusable components with private variables and methods.
Creating Closures with Arguments: Closures can also be created with arguments passed to the outer function. These arguments become part of the closure's scope and can be accessed by the inner function.
Important Considerations
Memory Management: Closures can lead to memory leaks if not used carefully. If a closure is created and stored in a variable, the outer function's variables will also be kept in memory, even if the outer function is no longer needed.
Performance: While closures are powerful, they can have a slight performance impact due to the additional memory and scope chain management.
Conclusion
Understanding closures is essential for mastering JavaScript. By understanding how closures work and their use cases, you can write more efficient and organized code. Remember to use closures judiciously to avoid potential memory leaks and performance issues.
Subscribe to my newsletter
Read articles from Abhishek Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
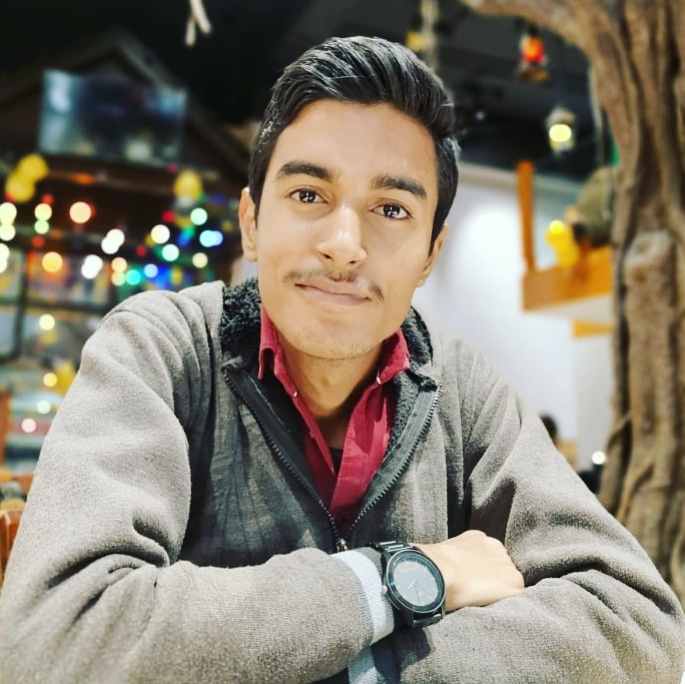
Abhishek Sharma
Abhishek Sharma
Abhishek is a designer, developer, and gaming enthusiast! He love creating things, whether it's building websites, designing interfaces, or conquering virtual worlds. With a passion for technology and its impact on the future, He is curious about how AI can be used to make work better and play even more fun.