Tutoriel : Comment créer et déployer votre propre collection NFT sur Morph L2 (Ethers.js v6 et Hardhat)

Table of contents
- Aperçu :
- Prérequis :
- Étape 1 : Configurer votre environnement de développement
- Étape 2 : Installer les contrats OpenZeppelin
- Étape 3 : Écrire le contrat intelligent NFT
- Étape 4 : Déployer le contrat intelligent NFT sur Morph L2
- Étape 5 : Frappez votre premier NFT
- Étape 6 : Créer des métadonnées pour vos NFTs
- Étape 7 : Construire un frontend simple pour afficher vos NFTs
- Conclusion
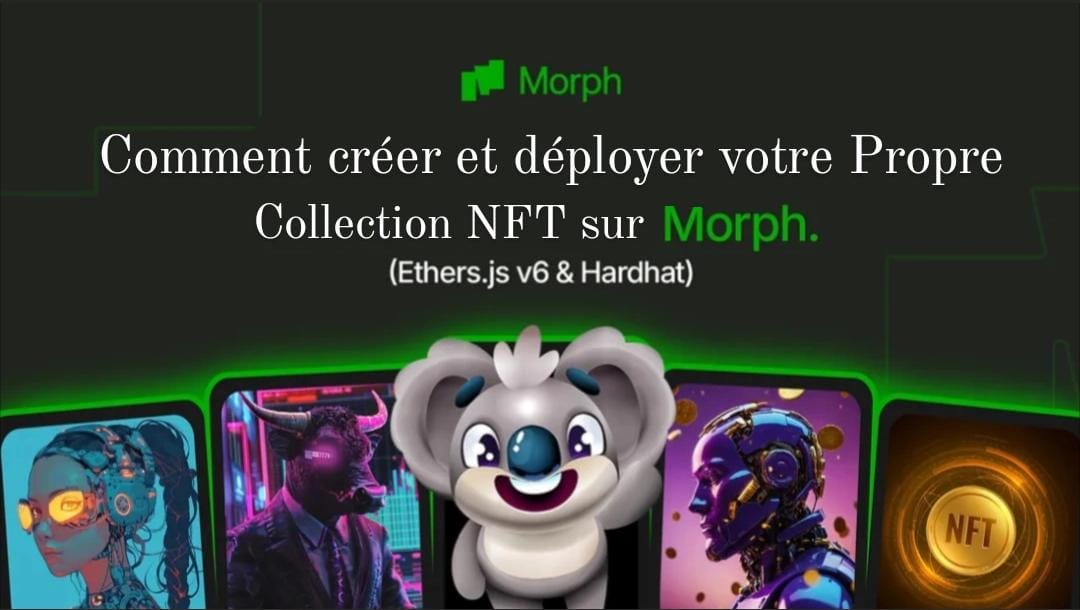
Aperçu :
Dans ce tutoriel, nous allons créer étape par étape et déployer votre propre collection NFT sur Morph L2, en utilisant les dernières versions d'Ethers.js v6 et Hardhat. Déployer des NFTs sur Layer 2 réduit les frais de gaz et améliore la vitesse des transactions, ce qui est idéal pour les projets NFT.
Prérequis :
Compréhension de base de la blockchain, des NFTs et de Solidity.
Environnement de développement configuré avec Node.js, Hardhat et Ethers.js v6.
Un portefeuille (par exemple, MetaMask) connecté au réseau Morph L2.
Accès au testnet de Morph L2.
Étape 1 : Configurer votre environnement de développement
Installez Node.js si ce n'est pas déjà fait. Téléchargez Node.js.
Créez un nouveau projet Hardhat et installez les dépendances nécessaires.
mkdir morph-nft cd morph-nft npm init -y npm install --save-dev hardhat
Initialisez Hardhat :
npx hardhat
Suivez les instructions pour créer un « projet d'exemple de base ». Cela configurera la structure de votre projet Hardhat.
Étape 2 : Installer les contrats OpenZeppelin
Nous utiliserons les contrats OpenZeppelin pour implémenter la norme ERC721 pour les NFTs.
npm install @openzeppelin/contracts
Étape 3 : Écrire le contrat intelligent NFT
Créez un nouveau fichier nommé MorphNFT.sol
dans le dossier des contrats et écrivez votre contrat intelligent NFT.
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.20;
import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
import "@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol";
import "@openzeppelin/contracts/access/Ownable.sol";
contract MorphNFT is ERC721URIStorage, Ownable {
uint256 public tokenCounter;
constructor() ERC721("MorphNFT", "MNFT") {
tokenCounter = 0;
}
function createNFT(string memory tokenURI) public onlyOwner returns (uint256) {
uint256 newItemId = tokenCounter;
_safeMint(msg.sender, newItemId);
_setTokenURI(newItemId, tokenURI);
tokenCounter += 1;
return newItemId;
}
}
Explication :
ERC721 : Contrat standard pour les NFTs.
ERC721URIStorage : Ajoute un support pour le stockage des URIs de tokens (métadonnées et images).
Ownable : Assure que seul le propriétaire du contrat peut frapper de nouveaux NFTs.
Étape 4 : Déployer le contrat intelligent NFT sur Morph L2
Configurez Hardhat pour le réseau Morph L2 :
Modifiez le fichierhardhat.config.js
pour inclure vos détails de réseau :require("@nomicfoundation/hardhat-toolbox"); require('dotenv').config(); module.exports = { solidity: "0.8.20", networks: { morphL2: { url: "https://rpc-quicknode-holesky.morphl2.io", accounts: [process.env.PRIVATE_KEY] // Store your private key in .env file for security } } };
Créez un script de déploiement :
Créez un fichier nommédeploy.js
dans le dossier desscripts
.const { ethers } = require("hardhat"); async function main() { const [deployer] = await ethers.getSigners(); console.log("Deploying contracts with the account:", deployer.address); // Get the contract factory const NFTContract = await ethers.getContractFactory("MorphNFT"); // Deploy the contract const nft = await NFTContract.deploy(); // Log the contract address console.log("NFT Contract deployed to:", nft.target); // Use .target to get the deployed address in Ethers.js v6 } main().catch((error) => { console.error(error); process.exitCode = 1; });
Déployez le contrat sur Morph L2 en exécutant le script :
npx hardhat run scripts/deploy.js --network morphL2
Une fois déployé, vous verrez l'adresse du contrat affichée dans votre console.
Étape 5 : Frappez votre premier NFT
Maintenant que le contrat est déployé, nous pouvons frapper notre premier NFT. Créez un nouveau fichier nommé mint.js
dans le dossier des scripts
.
const { ethers } = require("hardhat");
async function main() {
const [deployer] = await ethers.getSigners();
const nftAddress = "YOUR_DEPLOYED_NFT_ADDRESS"; // Replace with the deployed contract address
const NFTContract = await ethers.getContractAt("MorphNFT", nftAddress);
const tokenURI = "https://your-nft-metadata-uri.com/metadata.json"; // Replace with your metadata URI
const tx = await NFTContract.createNFT(tokenURI);
await tx.wait();
console.log("NFT minted with URI:", tokenURI);
}
main().catch((error) => {
console.error(error);
process.exitCode = 1;
});
Exécutez le script de minting :
npx hardhat run scripts/mint.js --network morphL2
Étape 6 : Créer des métadonnées pour vos NFTs
Les métadonnées des NFTs sont stockées hors chaîne, généralement en utilisant IPFS. Voici un exemple de fichier de métadonnées :
{
"name": "Morph NFT #1",
"description": "This is the first NFT in the Morph L2 collection.",
"image": "https://ipfs.io/ipfs/Qm.../nft1.png",
"attributes": [
{
"trait_type": "Background",
"value": "Blue"
},
{
"trait_type": "Eyes",
"value": "Green"
}
]
}
Utilisez des services comme Infura pour télécharger vos métadonnées sur IPFS, et utilisez l'URL résultante comme tokenURI
lors de la frappe des NFTs.
Étape 7 : Construire un frontend simple pour afficher vos NFTs
Vous pouvez créer un frontend basique pour permettre aux utilisateurs d'interagir avec vos NFTs. Voici un exemple simple utilisant React et Ethers.js v6.
Configurez une application React :
npx create-react-app morph-nft-frontend cd morph-nft-frontend npm install ethers
Modifiez App.js :
import React, { useState } from 'react'; import { ethers } from 'ethers'; import NFTContractABI from './NFTContractABI.json'; // Make sure to export the ABI from Hardhat const contractAddress = "YOUR_DEPLOYED_NFT_ADDRESS"; // Replace with your contract's address function App() { const [nftURI, setNFTURI] = useState(""); const getNFTData = async () => { const provider = new ethers.BrowserProvider(window.ethereum); const signer = await provider.getSigner(); const contract = new ethers.Contract(contractAddress, NFTContractABI, signer); const tokenURI = await contract.tokenURI(0); // Fetch the URI of token 0 setNFTURI(tokenURI); }; return ( <div> <h1>My Morph L2 NFT Collection</h1> <button onClick={getNFTData}>Fetch NFT Data</button> {nftURI && <p>NFT URI: {nftURI}</p>} </div> ); } export default App;
Exécutez le frontend :
npm start
Ce frontend simple permet aux utilisateurs de récupérer et d'afficher les métadonnées du premier NFT frappé depuis votre contrat.
Conclusion
Vous avez réussi à créer et déployer votre propre collection NFT sur Morph L2 en utilisant les dernières versions d'Ethers.js v6 et Hardhat ! Vous avez appris comment :
Configurer un contrat intelligent en utilisant la norme ERC-721 d'OpenZeppelin.
Le déployer sur un réseau Layer 2.
Frappes un NFT et construisez un frontend qui interagit avec votre contrat.
N'hésitez pas à étendre ce tutoriel en ajoutant des fonctionnalités comme le minting par lot, l'intégration des normes de redevance ou en construisant un marché NFT complet !
Vous pouvez trouver le code exemple pour ce tutoriel ici : Github Repos
Subscribe to my newsletter
Read articles from Daniel Kambale directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Daniel Kambale
Daniel Kambale
Hello! I’m Daniel, a Web3 developer specializing in Solidity and smart contract development. My journey in the blockchain space is driven by a vision of a fully decentralized world, where technology empowers individuals and transforms industries. As a Web3 ambassador , I’m committed to fostering growth and innovation in this space, helping to shape a future that values transparency and security. Fluent in both English and French, I enjoy connecting with diverse communities and sharing my insights across languages. This is why you’ll find some of my articles in French, while others are in Swahili, as I believe knowledge should be accessible to all. I use my Hashnode blog to document my learning process, explore decentralized solutions, and share practical tutorials on Web3 development. Whether it's diving deep into Solidity, discussing the latest in blockchain, or exploring new tools, I’m passionate about contributing to a decentralized future and connecting with others who share this vision. Let’s build the future together!