20 Xgboost concepts with Before-and-After Examples
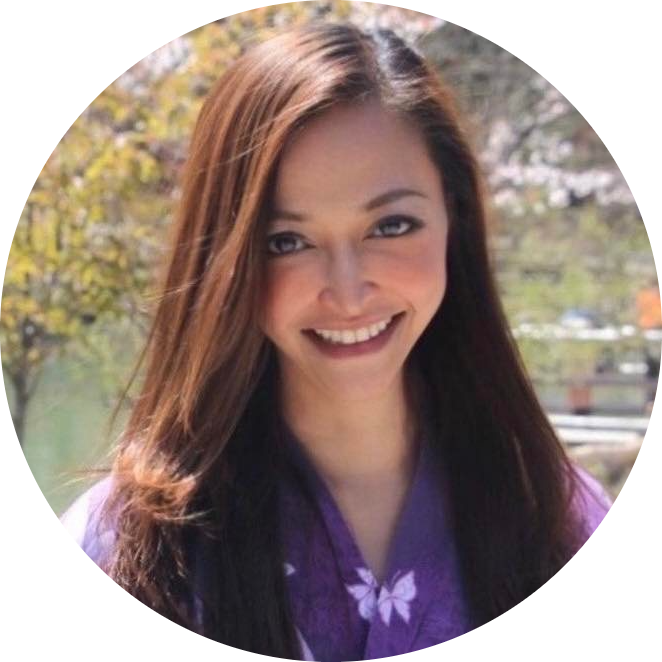
Table of contents
- 1. DMatrix (Efficient Data Structure) ๐
- 2. Training a Model (xgb.train) ๐๏ธ
- 3. Predicting with a Model (model.predict) ๐ฎ
- 4. Cross-Validation (xgb.cv) ๐
- 5. Evaluating a Model (evals_result) ๐
- 6. Early Stopping (Stopping when performance stagnates) ๐
- 7. Feature Importance (model.get_score) ๐
- 8. Parameter Tuning (Grid Search) ๐ง
- 9. Learning Rate Schedule (Decay) ๐
- 10. Handling Imbalanced Data (scale_pos_weight) โ๏ธ
- 11. Saving and Loading Models (model.save_model / xgb.Booster.load_model) ๐พ
- 12. Feature Selection (xgb.feature_importances_) ๐
- 13. Handling Missing Data (DMatrix missing parameter) ๐ซ
- 14. Regularization (Lambda and Alpha) ๐ก๏ธ
- 15. Custom Loss Functions (Objective) ๐ฏ
- 16. Multiclass Classification (Objective) ๐จ
- 17. F1 Score (Evaluation Metric) ๐
- 18. GPU Acceleration (tree_method) โก
- 19. Shrinking Trees (eta) โฌ
- 20. Verbose Logging (verbosity) ๐ฃ๏ธ
1. DMatrix (Efficient Data Structure) ๐
Think of DMatrix
like a well-organized filing system ๐ for XGBoost. Imagine youโre running a race ๐โโ๏ธ, and you have all the tools you need, but theyโre scattered everywhere. You waste time searching for the right shoes, your water bottle, or your stopwatch โฑ๏ธ.
Now, if you organize everything neatlyโshoes ready to wear, water on hand, and stopwatch setโyou're prepared for peak performance. Thatโs what DMatrix
does: it organizes and optimizes your data so XGBoost can work at top speed ๐ without wasting time on inefficient structures.
Boilerplate Code:
import xgboost as xgb
dtrain = xgb.DMatrix(data, label=labels)
Use Case: Create an efficient data structure that XGBoost can work with for training and testing. ๐
Goal: Prepare data in the most optimized format for XGBoost. ๐ฏ
Before Example: has raw data but itโs not in the optimized format for XGBoost. ๐ค
Data: raw format [X, Y]
After Example: With DMatrix, the data is ready for high-performance training! ๐
DMatrix: optimized data for XGBoost.
Challenge: ๐ Try converting your data from different sources like NumPy arrays or Pandas DataFrames into DMatrix format.
2. Training a Model (xgb.train) ๐๏ธ
Training an XGBoost model is like preparing for a competition ๐๏ธ. Imagine youโre coaching someone for a big event. You set specific rules or strategies for trainingโlike focusing on endurance, strength, or agility (similar to setting hyperparameters like max_depth
, learning_rate
) ๐ฏ. Each training session, or "boosting round" ๐ช, builds on the previous one, gradually improving performance. After enough rounds (like 100 training sessions), your trainee is stronger and faster, ready for the big competition (your trained model is now optimized for prediction) ๐!
Use Case: Train a model using the XGBoost framework. ๐๏ธ
Goal: Build and train a model using boosting iterations and hyperparameters. ๐ฏ
Sample Code:
# Train the XGBoost model
params = {"objective": "reg:squarederror", "max_depth": 3}
model = xgb.train(params, dtrain, num_boost_round=100)
Before Example: has data but no trained model. ๐ค
Data: [X, Y]
After Example: With xgb.train(), now has a trained XGBoost model! ๐๏ธ
Model: trained with 100 boosting rounds.
Challenge: ๐ Try changing the num_boost_round
and tuning other hyperparameters like learning_rate
or gamma
.
3. Predicting with a Model (model.predict) ๐ฎ
Use Case: Use a trained model to make predictions on new data. ๐ฎ
Goal: Generate predictions from the trained XGBoost model. ๐ฏ
Sample Code:
# Predict with the trained model
predictions = model.predict(dtest)
Before Example: has a trained model but no predictions yet. ๐ค
Model: trained but no predictions made.
After Example: With model.predict(), predictions are generated! ๐ฎ
Predictions: [Y1, Y2, Y3...]
Challenge: ๐ Try using the model to predict on different test sets and evaluate the results.
4. Cross-Validation (xgb.cv) ๐
Cross-validation is like testing a new car ๐ on different roads before launching it to the market. Imagine youโve built a car (your model), but you want to be sure it performs well in various conditionsโsmooth highways, bumpy roads, or winding mountain paths (different data splits) ๐. By running cross-validation, you drive the car on 5 different tracks (5-fold CV), seeing how it handles each. After testing on all tracks, you have a better idea of how it will perform in the real world ๐, ensuring the model is robust and not just trained for one specific condition.
Use Case: Perform cross-validation to evaluate the modelโs performance on different splits of the data. ๐
Goal: Test your modelโs performance across multiple folds of data to ensure robustness. ๐ฏ
Sample Code:
# Perform cross-validation
cv_results = xgb.cv(params, dtrain, nfold=5, num_boost_round=100)
Before Example: we train the model but doesnโt know how well it generalizes across different data splits. ๐ค
Model: trained, but performance on various splits unknown.
After Example: With xgb.cv(), we get cross-validation results for different folds! ๐
Cross-Validation: results for 5 different folds.
Challenge: ๐ Try changing the number of folds (nfold
) and experiment with more advanced cross-validation strategies.
5. Evaluating a Model (evals_result) ๐
Evaluating a model with evals_result
is like checking your fitness tracker ๐๏ธโโ๏ธ during each workout session. Imagine youโre working out but want to know how well youโre doing as you go alongโtracking your heart rate, calories burned, or distance covered ๐. Without it, youโre in the dark about your progress. With evals_result
, itโs like having that tracker on your wrist, giving you detailed stats for every rep (boosting round). You can see if youโre improving, plateauing, or overdoing it (overfitting) and adjust accordingly to stay on track ๐!
Use Case: Monitor the evaluation metrics during training to track the modelโs performance. ๐
Goal: Keep an eye on training metrics to prevent overfitting or underfitting. ๐ฏ
Sample Code:
# Track evaluation results
evals_result = {}
model = xgb.train(params, dtrain, evals=[(dtrain, 'train')], evals_result=evals_result)
# Check evaluation results
print(evals_result)
Before Example: Train the model but has no insight into how well itโs performing during training. ๐ค
Model: training without evaluation tracking.
After Example: With evals_result, the intern gets metrics for every boosting round! ๐
Evaluation: detailed training metrics at every step.
Challenge: ๐ Try adding validation sets to track metrics for both training and validation data.
6. Early Stopping (Stopping when performance stagnates) ๐
Use Case: Implement early stopping to stop training when the model performance plateaus. ๐
Goal: Prevent overfitting by halting training once the validation performance stops improving. ๐ฏ
Sample Code:
# Implement early stopping
model = xgb.train(params, dtrain, num_boost_round=1000, early_stopping_rounds=10, evals=[(dval, 'validation')])
Before Example: The intern continues training even after the model stops improving, wasting resources. ๐ฌ
Training: no stopping even when performance stagnates.
After Example: With early stopping, training halts as soon as the performance plateaus! ๐
Training stopped after no improvement for 10 rounds.
Challenge: ๐ Try adjusting the early_stopping_rounds
parameter and see how it affects the final model.
7. Feature Importance (model.get_score) ๐
Use Case: Check the importance of each feature to understand which features have the most impact on the model. ๐
Goal: Identify the most significant features contributing to the modelโs predictions. ๐ฏ
Sample Code:
# Get feature importance
importance = model.get_score(importance_type='weight')
# Print feature importance
print(importance)
Before Example: The intern has trained the model but doesnโt know which features are most impactful. ๐ค
Model: feature importance unknown.
After Example: With feature importance, the intern now knows which features matter the most! ๐
Feature Importance: [feature1: 0.4, feature2: 0.3...]
Challenge: ๐ Try plotting the feature importance using xgb.plot_importance()
.
8. Parameter Tuning (Grid Search) ๐ง
Boilerplate Code:
from sklearn.model_selection import GridSearchCV
Use Case: Perform hyperparameter tuning to find the best combination of parameters for your model. ๐ง
Goal: Improve model performance by optimizing hyperparameters. ๐ฏ
Sample Code:
# Define parameter grid
param_grid = {
'max_depth': [3, 5, 7],
'learning_rate': [0.01, 0.1, 0.2]
}
# Perform Grid Search
grid_search = GridSearchCV(estimator=xgb.XGBRegressor(), param_grid=param_grid, cv=3)
grid_search.fit(X, y)
Before Example: We use default parameters, but the modelโs performance is suboptimal. ๐ค
Model: default hyperparameters.
After Example: With Grid Search, we find the best parameters for optimal performance! ๐ง
Tuned Parameters: max_depth=5, learning_rate=0.1.
Challenge: ๐ Try using RandomizedSearchCV for faster tuning with larger parameter grids.
9. Learning Rate Schedule (Decay) ๐
Learning rate decay is like training for a marathon ๐โโ๏ธ. At the start, you go hard, putting in a lot of effort (high learning rate) to build up stamina quickly. But as you get closer to the race, you start slowing down your training intensity (lowering the learning rate) to avoid burning out and make sure your body recovers and adapts. By tapering off, you allow yourself to fine-tune your performance without risking injury (instability in training), making sure youโre fully prepared by race day (smooth model convergence) ๐.
Use Case: Use a learning rate schedule to gradually reduce the learning rate during training. ๐
Goal: Help the model converge more smoothly by lowering the learning rate over time. ๐ฏ
Sample Code:
# Set learning rate decay
params = {'learning_rate': 0.1}
params['lr_decay'] = 0.99 # Apply decay factor per round
Before Example: We use a constant learning rate, which can lead to instability in training. ๐ฌ
Learning Rate: constant at 0.1.
After Example: With learning rate decay, the learning rate decreases gradually! ๐
Learning Rate: starts at 0.1, decays over time.
Challenge: ๐ Try adjusting the decay factor and observe how it affects the modelโs convergence.
10. Handling Imbalanced Data (scale_pos_weight) โ๏ธ
Handling imbalanced data with scale_pos_weight
is like adding extra workers to a small team in a big project ๐๏ธ. Imagine you have two teams: one large and one small. The big team (majority class) easily handles their workload, while the small team (minority class) struggles to keep up. By adding more workers (adjusting scale_pos_weight
), you give the small team extra help, so they can complete their tasks just as efficiently. This balances the workload between the two teams, ensuring the project (model performance) runs smoothly on both fronts โ๏ธ.
Use Case: Adjust for imbalanced datasets where one class is much larger than the other. โ๏ธ
Goal: Balance the modelโs predictions when one class is more frequent than the other. ๐ฏ
Sample Code:
# Set scale_pos_weight for handling class imbalance
params = {'scale_pos_weight': 10} # Higher for imbalanced class
Before Example: data is imbalanced, and the model is biased toward the larger class. ๐ฌ
Data: class imbalance, poor performance on minority class.
After Example: With scale_pos_weight, the model correctly adjusts for the imbalance! โ๏ธ
Balanced Model: better performance on minority class.
Challenge: ๐ Try experimenting with different scale_pos_weight
values to see how they affect the modelโs performance.
11. Saving and Loading Models (model.save_model / xgb.Booster.load_model) ๐พ
Saving a model is like saving your game progress in a video game ๐ฎ. Imagine you've played through several levels (trained the model), and you donโt want to start from scratch every time you power off the console (restart the environment) ๐ฌ. By saving the game (using model.save
_model()
), you can return right where you left off without replaying all the levels. When you load your saved file (use load_model()
), youโre back in the action instantly, ready to continue without wasting time on previous stages ๐พ!
Use Case: Save a trained model to disk and load it later for inference or further use. ๐พ
Goal: Store models for future use without retraining. ๐ฏ
Sample Code:
# Save the model
model.save_model('xgb_model.json')
# Load the model
loaded_model = xgb.Booster()
loaded_model.load_model('xgb_model.json')
Before Example: We train a model but needs to retrain it every time they restart the environment. ๐ค
Trained model: not saved, retraining required.
After Example: With model.save_model(), the trained model is saved and can be reloaded anytime! ๐พ
Saved model: 'xgb_model.json', loaded for future use.
Challenge: ๐ Try saving the model in different formats like .bin
and test loading it.
12. Feature Selection (xgb.feature_importances_) ๐
Feature selection with xgb.feature_importances_
is like packing for a trip with limited luggage space ๐งณ. Imagine you have a lot of items (features), but not all of them are equally important for your journey. You need to figure out which ones are essential (most impactful) and which ones you can leave behind (less important) ๐ค. By checking feature importance, itโs like weighing each item to see how much value it adds to your trip. Now, you can pack only the things that really matter, ensuring a smooth and efficient journey (model performance) ๐ฏ!
Use Case: Perform feature selection by checking the importance of each feature based on gain or split. ๐
Goal: Identify which features contribute the most to model predictions. ๐ฏ
Sample Code:
# Get feature importance based on gain
feature_importance = model.get_score(importance_type='gain')
# Print feature importance
print(feature_importance)
Gain: It measures how much each feature improves the modelโs performance during the splitting process in decision trees. A feature with high gain contributes significantly to better splits, meaning it provides more predictive power.
Before Example: has many features but doesnโt know which ones matter most. ๐ค
Data: many features, no ranking of importance.
After Example: With feature importance, we can now rank features by their contribution! ๐
Feature Importance: ranked based on gain.
Challenge: ๐ Try visualizing feature importance with xgb.plot_importance()
.
13. Handling Missing Data (DMatrix missing parameter) ๐ซ
Use Case: Efficiently handle missing values in the dataset. ๐ซ
Goal: Automatically manage missing data without having to manually fill or drop them. ๐ฏ
Sample Code:
# Handle missing values in the data
dtrain = xgb.DMatrix(data, label=labels, missing=np.nan)
Before Example: has missing values in the dataset and manually handles them. ๐ฌ
Data: missing values not efficiently handled.
After Example: With missing parameter, XGBoost automatically manages missing data! ๐ซ
Missing values: efficiently handled with np.nan.
Challenge: ๐ Try experimenting with missing values in different datasets and see how the model adjusts.
14. Regularization (Lambda and Alpha) ๐ก๏ธ
Boilerplate Code:
params = {'lambda': 1.0, 'alpha': 0.5}
Use Case: Apply L2 (lambda) and L1 (alpha) regularization to avoid overfitting. ๐ก๏ธ
Goal: Prevent the model from becoming too complex and overfitting the training data. ๐ฏ
Sample Code:
# Apply regularization
params = {'lambda': 1.0, 'alpha': 0.5}
model = xgb.train(params, dtrain, num_boost_round=100)
Before Example: The model overfits the training data by being too complex. ๐ฌ
Model: overfitting, poor generalization.
After Example: With regularization, the model is now less prone to overfitting! ๐ก๏ธ
Regularized Model: improved generalization.
Challenge: ๐ Try experimenting with different lambda
and alpha
values to find the best balance between complexity and performance.
15. Custom Loss Functions (Objective) ๐ฏ
Boilerplate Code:
params = {'objective': custom_loss}
Use Case: Define a custom loss function to optimize the model for specific use cases. ๐ฏ
Goal: Tailor the loss function to fit the needs of your specific problem. ๐ฏ
Sample Code:
# Define a custom loss function
def custom_loss(preds, dtrain):
labels = dtrain.get_label()
diff = preds - labels
return 'custom_loss', np.sum(diff**2)
# Apply custom loss function
params = {'objective': custom_loss}
model = xgb.train(params, dtrain, num_boost_round=100)
Before Example: we are restricted by the default loss functions, which donโt quite fit their problem. ๐ค
Loss function: limited to defaults.
After Example: With custom loss, the model is optimized for a more specific use case! ๐ฏ
Custom Loss: tailored to the problem.
Challenge: ๐ Try experimenting with custom loss functions for different types of regression or classification problems.
16. Multiclass Classification (Objective) ๐จ
Multiclass classification is like sorting items into multiple bins ๐๏ธ instead of just two. Imagine you're running a library ๐, and before, you only had two shelves: one for fiction and one for non-fiction (binary classification). Now, the library is growing, and you need to organize books into more specific categories like fiction, history, and science (multiclass classification) ๐จ. With XGBoost's multiclass classification, you can predict which "shelf" each book belongs to, ensuring every book is placed correctly based on its type. This way, you're no longer limited to just two choices; you have multiple categories to work with ๐!
Boilerplate Code:
params = {'objective': 'multi:softmax', 'num_class': 3}
Use Case: Perform multiclass classification using XGBoost, predicting more than two classes. ๐จ
Goal: Build a model that predicts multiple categories instead of just binary outcomes. ๐ฏ
Sample Code:
# Set up multiclass classification
params = {'objective': 'multi:softmax', 'num_class': 3}
model = xgb.train(params, dtrain, num_boost_round=100)
Before Example: trying to predict multiple categories but the model is only set up for binary classification. ๐ฌ
Model: binary classification only.
After Example: With multiclass classification, the model can predict multiple categories! ๐จ
Multiclass Model: predicts 3 classes.
Challenge: ๐ Try using multi:softprob
to get probability estimates for each class instead of just class labels.
17. F1 Score (Evaluation Metric) ๐
Adding F1 score as an evaluation metric is like grading a student not just on their final exam score (accuracy) but also on how well they performed in different areas like homework (precision) and participation (recall) ๐. Relying only on the final exam can be misleading if they excel in certain areas but struggle in others (imbalanced data). By considering the F1 score, youโre looking at the overall balance between their strengths and weaknesses, ensuring a fairer assessment of their performance ๐ . Similarly, F1 score helps balance precision and recall, giving you a more complete view of your modelโs ability to handle imbalanced datasets.
Use Case: Add F1 score as an evaluation metric to better assess model performance. ๐
Goal: Track the balance between precision and recall with F1 score. ๐ฏ
Sample Code:
# Set evaluation metric to F1 score
params = {'eval_metric': 'mlogloss', 'eval_metric': 'merror', 'eval_metric': 'f1'}
Before Example: only tracking accuracy, which can be misleading for imbalanced datasets. ๐ค
Evaluation: accuracy-only.
After Example: With F1 score, can better evaluate performance on imbalanced data! ๐
Evaluation: accuracy + F1 score.
Challenge: ๐ Try tracking multiple evaluation metrics (e.g., precision, recall, F1 score) at the same time.
18. GPU Acceleration (tree_method) โก
Boilerplate Code:
params = {'tree_method': 'gpu_hist'}
Use Case: Speed up training with GPU acceleration, especially on large datasets. โก
Goal: Leverage the power of GPUs to drastically reduce training time. ๐ฏ
Sample Code:
# Use GPU for training
params = {'tree_method': 'gpu_hist'}
model = xgb.train(params, dtrain, num_boost_round=100)
Before Example: The model trains too slowly on large datasets using CPU. ๐ข
Training: slow on large dataset.
After Example: With GPU acceleration, training time is drastically reduced! โก
Training: lightning-fast with GPU.
Challenge: ๐ Try comparing the training speed with and without GPU acceleration.
19. Shrinking Trees (eta) โฌ
Adjusting eta
in XGBoost is like turning down the volume on a speaker ๐๏ธ. Imagine youโre listening to music, but the volume is too high (high learning rate), and itโs overwhelming (overfitting). By turning down the volume (lowering eta
), you can still enjoy the music ๐ถ, but now itโs more balanced and easier on the ears (reduces overfitting). In the boosting process, lowering eta
reduces the impact of each tree, allowing the model to gradually learn from the data without over-amplifying mistakes ๐!
Boilerplate Code:
params = {'eta': 0.1}
Use Case: Use shrinkage by adjusting eta (learning rate) to reduce overfitting. โฌ
Goal: Control the impact of each individual tree in the boosting process. ๐ฏ
Sample Code:
# Set eta for shrinkage
params = {'eta': 0.1}
model = xgb.train(params, dtrain, num_boost_round=100)
Before Example: The model overfits because each tree has too much influence. ๐ฌ
Model: overfitting due to high learning rate.
After Example: With eta, each treeโs contribution is reduced, preventing overfitting! โฌ
Shrunk Model: reduced overfitting with lower eta.
Challenge: ๐ Try experimenting with very low eta
values (e.g., eta=0.01
) and increase the number of boosting rounds.
20. Verbose Logging (verbosity) ๐ฃ๏ธ
"Verbosity" means the quality of using more words than necessary or providing excessive detail. In simpler terms, it refers to how wordy or detailed something is. For example, a "verbose" explanation might be long-winded or overly detailed, while a "non-verbose" one would be short and to the point.
In programming, "verbosity" controls how much information (or logs) is printed during a process. A higher verbosity level means more detailed logs, while a lower verbosity level shows fewer details.
Setting verbosity
in XGBoost is like adjusting the commentary during a sports game ๐๏ธ. Imagine you're watching a match, and the commentator either talks nonstop (too verbose) or is completely silent (too quiet) ๐ฌ. If thereโs too much talking, you get overwhelmed, but if thereโs no commentary, you miss important updates. By setting the verbosity level (like lowering the volume of commentary), you get just the right amount of information, hearing key highlights without being overwhelmed. Similarly, adjusting verbosity
lets you see enough training details without drowning in logs or missing critical info ๐!
Boilerplate Code:
params = {'verbosity': 2}
Use Case: Adjust the verbosity level of training logs to get more or less detailed information. ๐ฃ๏ธ
Goal: Control how much logging information is shown during training. ๐ฏ
Sample Code:
# Set verbosity to a moderate level
params = {'verbosity': 2}
Before Example: The log is either too verbose or too quiet, making it hard to track progress. ๐ค
Log: too much/too little information.
After Example: With verbosity, the intern gets just the right amount of information! ๐ฃ๏ธ
Log: moderate level of detail, easy to follow.
Challenge: ๐ Try setting different verbosity levels (0
= silent, 1
= warning, 2
= info, 3
= debug) to control the output.
Subscribe to my newsletter
Read articles from Anix Lynch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
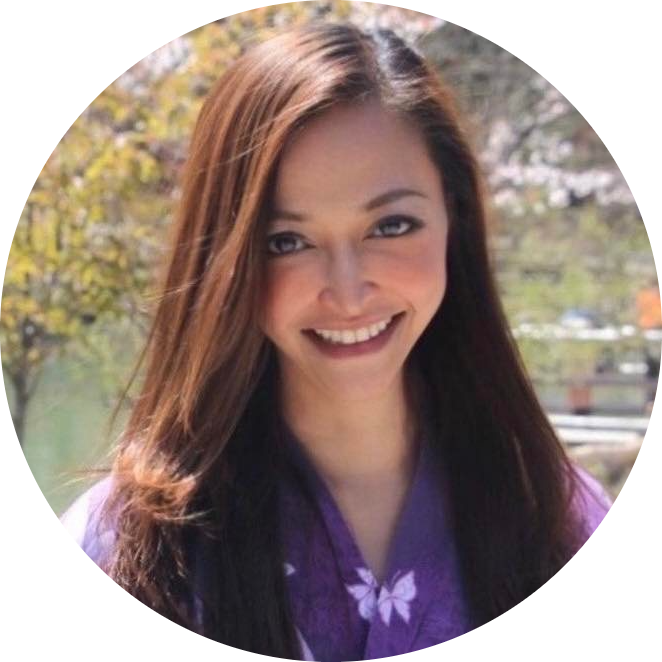