GraphQL: Why and How to Use It
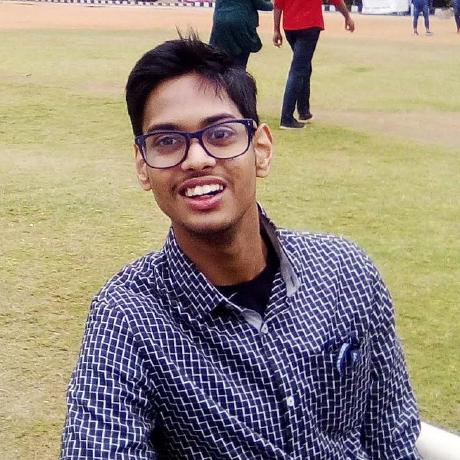
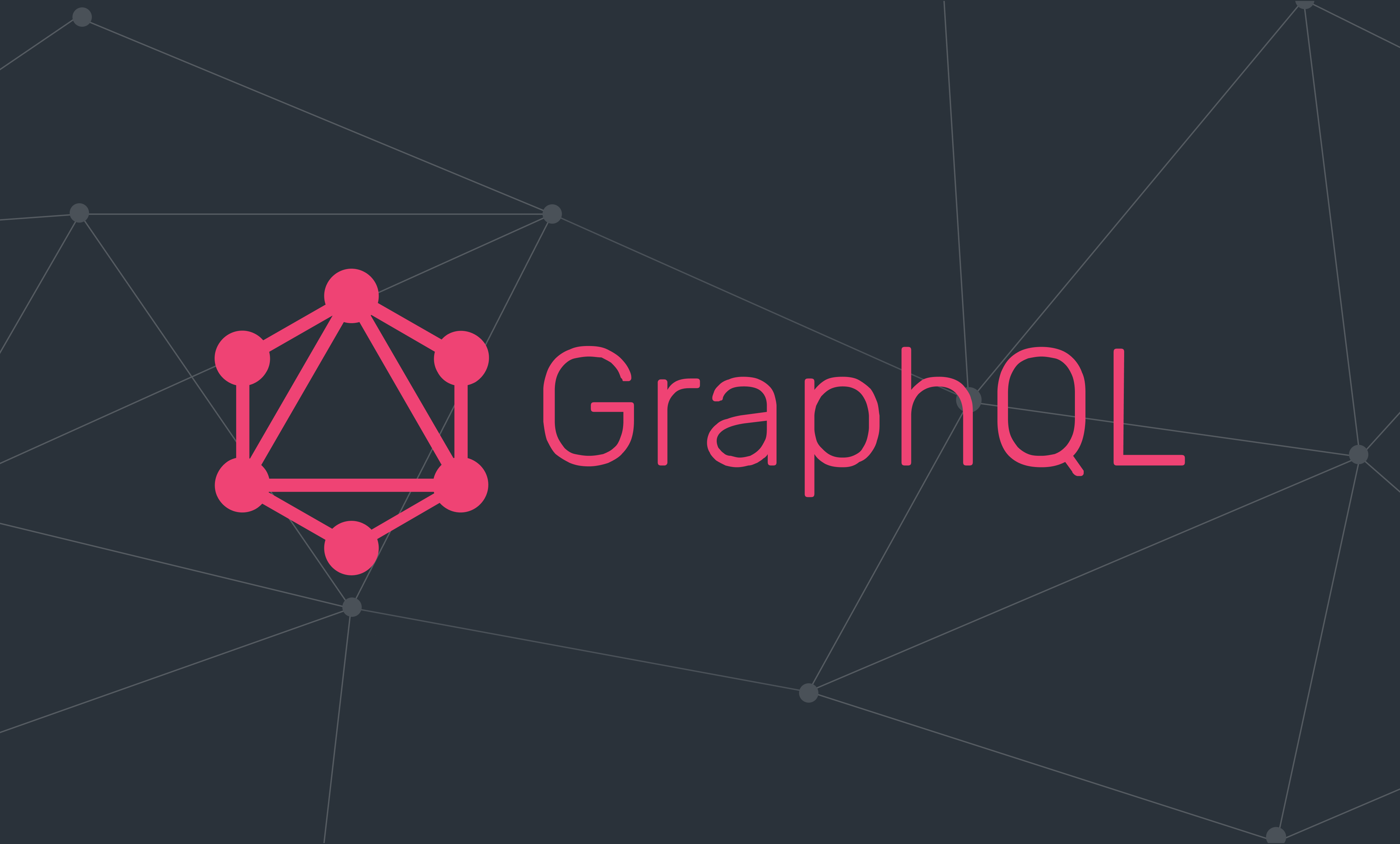
In recent years, GraphQL has become a buzzword in web development, offering an alternative to the traditional REST API. Developed by Facebook in 2012 and open-sourced in 2015, GraphQL is now widely adopted by tech giants and small startups alike. But what exactly is GraphQL, and why has it garnered so much attention? Let’s dive into the core concepts and understand why and how to use GraphQL in modern application development.
What is GraphQL?
GraphQL is a query language for APIs and a runtime for executing those queries. Unlike REST, which exposes multiple endpoints for each type of resource (e.g., /users
, /posts
), GraphQL exposes a single endpoint through which clients can request specific data by defining the structure of their query.
This makes it flexible, efficient, and declarative. Instead of getting predefined sets of data, clients can specify exactly what they need, making over-fetching and under-fetching of data a thing of the past.
Why Use GraphQL?
1. Flexibility and Efficiency
In REST APIs, you may need to make multiple API calls to get related data from different endpoints. This can lead to over-fetching, where unnecessary data is retrieved, or under-fetching, where additional requests are needed.
With GraphQL, you can request exactly the data you need in a single query. For example, if you only want a user’s name and email, you can query just those fields instead of fetching the entire user object. This reduces network overhead and improves the efficiency of data transfer.
2. Single Endpoint
GraphQL operates through a single endpoint (/graphql
), whereas REST uses multiple endpoints for different resources. This simplifies the API architecture and reduces the complexity of routing. You can query for multiple resources in one call, as opposed to making multiple requests to different endpoints.
3. Real-Time Updates with Subscriptions
For apps that require real-time updates (like live chat or real-time notifications), GraphQL supports subscriptions, allowing clients to receive updates over WebSockets. This makes it an ideal choice for applications that need live data streams.
4. Self-Documenting
GraphQL APIs are self-documenting. The schema, which defines the types of queries, mutations, and objects, serves as a source of truth for the API. Developers can easily explore the available operations and data types using tools like GraphiQL or Apollo Studio, reducing the need for separate documentation.
5. Strongly Typed Schema
GraphQL uses a strongly typed schema that defines what data can be queried, along with types and relationships. This makes the API more predictable, and ensures that both frontend and backend developers have a clear understanding of what data is available.
Here is a simple example of the GraphQL server using NodeJS and ExpressJS.
const express = require('express');
const { graphqlHTTP } = require('express-graphql');
const { buildSchema } = require('graphql');
const app = express();
const schema = buildSchema(`
type Query {
hello: String
}
`);
hello: () => 'Hello, GraphQL!'
};
app.use('/graphql', graphqlHTTP({
schema: schema,
rootValue: root,
graphiql: true,
}));
app.listen(4000, () => console.log('GraphQL server running on http://localhost:4000/graphql'));
The schema in GraphQL defines what types of queries clients can perform and what types of data the server returns. A typical GraphQL schema consists of:
Queries for reading data
Mutations for creating, updating, or deleting data
Subscriptions for real-time data updates
type User { id: ID! name: String! email: String! } type Query { user(id: ID!): User users: [User] } type Mutation { createUser(name: String!, email: String!): User }
Making Queries
Once the GraphQL server is running, clients can send queries using fetch, axios
, or a client library like Apollo Client. Here’s an example query to fetch a list of users:
query {
users {
id
name
email
}
}
Conclusion
GraphQL’s flexibility, real-time capabilities, and self-documenting nature make it a powerful tool for modern APIs. GraphQL improves performance, reduces network requests, and streamlines development by allowing clients to request exactly the data they need. Whether you're building a small project or a large-scale application, GraphQL is worth considering for your API needs.
Embrace the shift from REST to GraphQL and enjoy a more efficient and structured way of managing your data!
Subscribe to my newsletter
Read articles from Anish Agrawal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
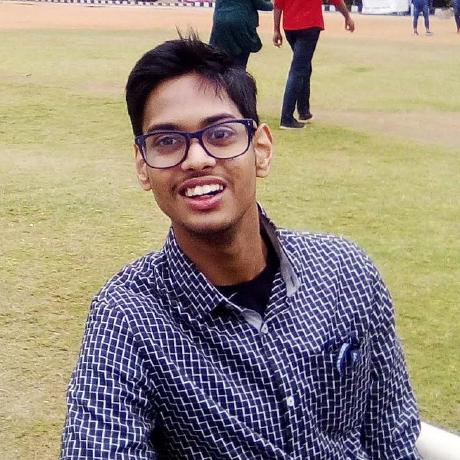