20 Seaborn concepts with Before-and-After Examples
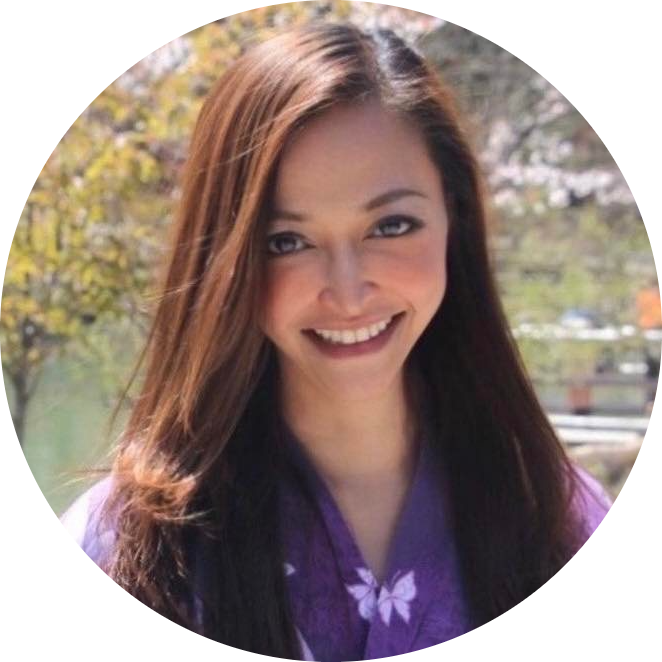
Table of contents
- 1. Line Plot (seaborn.lineplot) ๐
- 2. Bar Plot (seaborn.barplot) ๐
- 3. Count Plot (seaborn.countplot) ๐
- 4. Histogram (seaborn.histplot) ๐
- 5. Box Plot (seaborn.boxplot) ๐ฆ
- 6. Violin Plot (seaborn.violinplot) ๐ป
- 7. Scatter Plot (seaborn.scatterplot) ๐ต
- 8. Pair Plot (seaborn.pairplot) ๐
- 9. Heatmap (seaborn.heatmap) ๐ฅ
- 10. Joint Plot (seaborn.jointplot) ๐
- 11. Regression Plot (seaborn.regplot) ๐
- 12. KDE Plot (seaborn.kdeplot) ๐
- 13. Facet Grid (seaborn.FacetGrid) ๐
- 14. Swarm Plot (seaborn.swarmplot) ๐
- 15. Point Plot (seaborn.pointplot) ๐
- 16. LM Plot (seaborn.lmplot) ๐งฎ
- 17. Strip Plot (seaborn.stripplot) ๐ก
- 18. PairGrid (seaborn.PairGrid) ๐
- 19. Joint Grid (seaborn.JointGrid) โ๏ธ
- 20. Heatmap with Annotations (seaborn.heatmap) ๐ข
1. Line Plot (seaborn.lineplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a line plot to display trends over time or ordered data. ๐
Goal: Visualize continuous data over a range or time. ๐ฏ
Sample Code:
# Example data
data = [1, 2, 3, 4, 5]
sns.lineplot(x=[1, 2, 3, 4, 5], y=[2, 4, 6, 8, 10])
Before Example: You have a sequence of data points but no clear trend visualization. ๐ค
Data: x = [1, 2, 3, 4, 5], y = [2, 4, 6, 8, 10]
After Example: With lineplot, the trend of the data is clear! ๐
Output: A line plot connecting the points.
Challenge: ๐ Try adding markers with markers=True
to make each data point stand out.
2. Bar Plot (seaborn.barplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a bar plot to compare categorical data. ๐
Goal: Compare means or other summary statistics across categories. ๐ฏ
Sample Code:
# Example data
sns.barplot(x=['A', 'B', 'C'], y=[10, 20, 30])
Before Example: You have categories and values but no visual way to compare them. ๐ค
Data: x = ['A', 'B', 'C'], y = [10, 20, 30]
After Example: With barplot, you can compare the values across categories! ๐
Output: A bar chart showing categorical comparisons.
Challenge: ๐ Try adding error bars using the ci
parameter to represent uncertainty.
3. Count Plot (seaborn.countplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a count plot to show the count of observations in each category. ๐
Goal: Visualize the frequency of each category. ๐ฏ
Sample Code:
# Example data
sns.countplot(x=['cat', 'dog', 'dog', 'cat', 'bird'])
Before Example: You have categorical data but no way to visualize how often each category appears. ๐ค
Data: ['cat', 'dog', 'dog', 'cat', 'bird']
After Example: With countplot, you see how frequently each category appears! ๐
Output: A bar plot showing the count of each category.
Challenge: ๐ Try using hue
to split the counts by an additional categorical variable.
4. Histogram (seaborn.histplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a histogram to visualize the distribution of numerical data. ๐
Goal: Display the frequency of data points within bins. ๐ฏ
Sample Code:
# Example data
sns.histplot(data=[1, 2, 2, 3, 3, 4, 5], bins=5)
Before Example: You have numerical data but no way to visualize its distribution. ๐ค
Data: [1, 2, 2, 3, 3, 4, 5]
After Example: With histplot, the data distribution becomes clear! ๐
Output: A histogram showing data frequencies.
Challenge: ๐ Try changing the number of bins to see how it affects the histogram.
5. Box Plot (seaborn.boxplot) ๐ฆ
Boilerplate Code:
import seaborn as sns
Use Case: Create a box plot to show the distribution of data and detect outliers. ๐ฆ
Goal: Summarize the data distribution using quartiles and outliers. ๐ฏ
Sample Code:
# Example data
sns.boxplot(data=[1, 2, 3, 4, 5, 6, 7, 8, 9])
Before Example: You have numerical data but no way to visualize its range, quartiles, and outliers. ๐ค
Data: [1, 2, 3, 4, 5, 6, 7, 8, 9]
After Example: With boxplot, you can see the distribution and detect any outliers! ๐ฆ
Output: A box plot summarizing the data.
Challenge: ๐ Try splitting the box plots by categories using x
and y
arguments.
6. Violin Plot (seaborn.violinplot) ๐ป
Boilerplate Code:
import seaborn as sns
Use Case: Create a violin plot to show the distribution of data and its probability density. ๐ป
Goal: Combine the benefits of box plots and density plots. ๐ฏ
Sample Code:
# Example data
sns.violinplot(data=[1, 2, 3, 4, 5, 6, 7, 8, 9])
Before Example: You have numerical data but want a more detailed look at its distribution than just a box plot. ๐ค
Data: [1, 2, 3, 4, 5, 6, 7, 8, 9]
After Example: With violinplot, you visualize both the distribution and probability density! ๐ป
Output: A violin plot showing data density and distribution.
Challenge: ๐ Try splitting violins by categories to compare distributions between groups.
7. Scatter Plot (seaborn.scatterplot) ๐ต
Boilerplate Code:
import seaborn as sns
Use Case: Create a scatter plot to show the relationship between two numerical variables. ๐ต
Goal: Visualize individual data points to detect patterns or correlations. ๐ฏ
Sample Code:
# Example data
sns.scatterplot(x=[1, 2, 3, 4], y=[10, 11, 12, 13])
Before Example: You have two variables but no way to visualize their relationship. ๐ค
Data: x = [1, 2, 3, 4], y = [10, 11, 12, 13]
After Example: With scatterplot, the relationship between the variables is visualized! ๐ต
Output: A scatter plot showing individual data points.
Challenge: ๐ Try adding a third dimension by mapping a categorical variable to hue
.
8. Pair Plot (seaborn.pairplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a pair plot to visualize relationships between multiple pairs of variables. ๐
Goal: Show scatter plots for each pair of variables in a dataset. ๐ฏ
Sample Code:
# Example data
sns.pairplot(sns.load_dataset("iris"))
Before Example: You have multiple variables but no way to visualize their relationships in one view. ๐ค
Data: Iris dataset (sepal length, sepal width, petal length, petal width)
After Example: With pairplot, you can view scatter plots for each pair of variables! ๐
Output: A grid of scatter plots for each pair of variables.
Challenge: ๐ Try using hue
to differentiate categories in the dataset.
9. Heatmap (seaborn.heatmap) ๐ฅ
Boilerplate Code:
import seaborn as sns
Use Case: Create a heatmap to visualize data intensity in a matrix. ๐ฅ
Goal: Represent numerical data as color-coded intensity. ๐ฏ
Sample Code:
# Example data
import numpy as np
data = np.array([[1, 2], [3, 4]])
sns.heatmap(data)
Before Example: You have a matrix of numerical data but no clear way to visualize the intensity. ๐ค
Data: [[1, 2], [3, 4]]
After Example: With heatmap, you visualize the data intensity using colors! ๐ฅ
Output: A heatmap representing the matrix.
Challenge: ๐ Try using annot=True
to show the data values inside the heatmap cells.
10. Joint Plot (seaborn.jointplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a joint plot to visualize both the distribution and relationship of two variables. ๐
Goal: Combine scatter plots and distribution plots into one view. ๐ฏ
Sample Code:
# Example data
sns.jointplot(x=[1, 2, 3], y=[4, 5, 6])
Before Example: You have two variables but no way to visualize both their relationship and distribution. ๐ค
Data: x = [1, 2, 3], y = [4, 5, 6]
After Example: With jointplot, you can visualize both the scatter plot and histograms in one! ๐
Output: A combined scatter and histogram plot.
Challenge: ๐ Try using different kind
options like reg
for regression or hex
for hexbin plots.
11. Regression Plot (seaborn.regplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a regression plot to display a linear regression line with data points. ๐
Goal: Visualize the relationship between two variables with a regression line. ๐ฏ
Sample Code:
# Example data
sns.regplot(x=[1, 2, 3, 4], y=[5, 6, 7, 8])
Before Example: You have two variables but no way to represent their linear relationship. ๐ค
Data: x = [1, 2, 3, 4], y = [5, 6, 7, 8]
After Example: With regplot, you visualize the relationship with a linear regression line! ๐
Output: A scatter plot with a regression line.
Challenge: ๐ Try adding ci=None
to remove the confidence interval or change the order
for polynomial regression.
12. KDE Plot (seaborn.kdeplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a Kernel Density Estimate (KDE) plot to visualize the distribution of data. ๐
Goal: Show the probability density function of a variable. ๐ฏ
Sample Code:
# Example data
sns.kdeplot(data=[1, 2, 2, 3, 4, 5])
Before Example: You have numerical data but no smooth representation of its distribution. ๐ค
Data: [1, 2, 2, 3, 4, 5]
After Example: With kdeplot, you see a smooth curve representing the data's distribution! ๐
Output: A KDE plot showing the probability density.
Challenge: ๐ Try combining kdeplot
with histplot
for a detailed view of the distribution.
13. Facet Grid (seaborn
.FacetGrid) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a facet grid to plot multiple plots based on subsets of data. ๐
Goal: Display the relationship between variables across different subsets of data. ๐ฏ
Sample Code:
# Load example data
df = sns.load_dataset("tips")
# Create FacetGrid
g = sns.FacetGrid(df, col="sex", row="time")
g.map(sns.scatterplot, "total_bill", "tip")
Before Example: You have data split by categories but no way to visualize each subset clearly. ๐ค
Data: Tips dataset with sex and time categories.
After Example: With FacetGrid, you get multiple plots for each subset of the data! ๐
Output: A grid of scatter plots showing tips by total bill.
Challenge: ๐ Try using hue
to add another categorical dimension to the grid.
14. Swarm Plot (seaborn.swarmplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a swarm plot to display categorical scatter plots with non-overlapping points. ๐
Goal: Show the distribution of data points within categories without overlapping. ๐ฏ
Sample Code:
# Example data
sns.swarmplot(x=["cat", "cat", "dog", "dog"], y=[2, 3, 4, 5])
Before Example: You have categorical data but no way to show individual data points clearly. ๐ค
Data: Categories: ["cat", "dog"], Values: [2, 3, 4, 5]
After Example: With swarmplot, you display individual data points without overlap! ๐
Output: A swarm plot showing the distribution of points.
Challenge: ๐ Try using hue
to color-code points based on an additional variable.
15. Point Plot (seaborn.pointplot) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a point plot to visualize the relationship between categorical data and numerical data. ๐
Goal: Show mean values of categories with confidence intervals. ๐ฏ
Sample Code:
# Example data
sns.pointplot(x=["A", "B", "C"], y=[4, 5, 6])
Before Example: You have categorical data but no way to visualize the mean values across categories. ๐ค
Data: Categories: ["A", "B", "C"], Values: [4, 5, 6]
After Example: With pointplot, the mean values are visualized with error bars! ๐
Output: A point plot showing means with error bars.
Challenge: ๐ Try adding hue
to compare means across different categories.
16. LM Plot (seaborn.lmplot) ๐งฎ
Boilerplate Code:
import seaborn as sns
Use Case: Create an lmplot to visualize linear relationships with multiple facets. ๐งฎ
Goal: Combine regression lines and scatter plots with facet grids. ๐ฏ
Sample Code:
# Load example data
df = sns.load_dataset("tips")
# Create lmplot
sns.lmplot(x="total_bill", y="tip", hue="sex", data=df)
Before Example: You want to visualize both scatter plots and linear regression with multiple subsets of data. ๐ค
Data: Tips dataset with total bill and tip variables.
After Example: With lmplot, the linear regression lines and scatter plots are displayed! ๐งฎ
Output: A scatter plot with regression lines based on gender.
Challenge: ๐ Try using col
to split the plot by time of day.
17. Strip Plot (seaborn.stripplot) ๐ก
Boilerplate Code:
import seaborn as sns
Use Case: Create a strip plot to display individual data points with some jitter. ๐ก
Goal: Show the distribution of data points along a categorical axis with slight jitter. ๐ฏ
Sample Code:
# Example data
sns.stripplot(x=["cat", "dog", "dog", "cat"], y=[2, 3, 4, 5])
Before Example: You have categorical data and want to display individual points, but they overlap. ๐ค
Data: Categories: ["cat", "dog"], Values: [2, 3, 4, 5]
After Example: With stripplot, you can add jitter to make individual points clearer! ๐ก
Output: A strip plot with jittered data points.
Challenge: ๐ Try using hue
to differentiate points based on another category.
18. PairGrid (seaborn.PairGrid) ๐
Boilerplate Code:
import seaborn as sns
Use Case: Create a pair grid to visualize pairwise relationships between variables using different plots. ๐
Goal: Combine multiple plot types (scatter, histograms, etc.) into a grid for deeper analysis. ๐ฏ
Sample Code:
# Load example data
df = sns.load_dataset("iris")
# Create PairGrid
g = sns.PairGrid(df)
g.map_diag(sns.histplot)
g.map_offdiag(sns.scatterplot)
Before Example: You have multiple variables and want to use different plot types to explore their relationships. ๐ค
Data: Iris dataset with multiple numerical variables.
After Example: With PairGrid, you combine scatter plots, histograms, and more in one view! ๐
Output: A grid of plots for each pair of variables.
Challenge: ๐ Try mapping different plot types to diagonal and off-diagonal elements for a more detailed analysis.
19. Joint Grid (seaborn.JointGrid) โ๏ธ
Boilerplate Code:
import seaborn as sns
Use Case: Create a joint grid to visualize the relationship between two variables using multiple plots. โ๏ธ
Goal: Create a custom plot that combines scatter plots, KDE, or histograms. ๐ฏ
Sample Code:
# Example data
g = sns.JointGrid(x=[1, 2, 3], y=[4, 5, 6])
g.plot(sns
.scatterplot, sns.histplot)
Before Example: You want to customize the combination of scatter and distribution plots. ๐ค
Data: x = [1, 2, 3], y = [4, 5, 6]
After Example: With JointGrid, you customize how different plots are displayed together! โ๏ธ
Output: A combined scatter plot and histogram.
Challenge: ๐ Try using plot_kws
to add custom styles to the plots.
20. Heatmap with Annotations (seaborn.heatmap) ๐ข
Boilerplate Code:
import seaborn as sns
Use Case: Create a heatmap with annotations to visualize a matrix of numbers with text annotations. ๐ข
Goal: Display numerical data as color-coded intensity and show the exact values. ๐ฏ
Sample Code:
# Example data
import numpy as np
data = np.array([[1, 2], [3, 4]])
sns.heatmap(data, annot=True)
Before Example: You have a matrix of numbers but want both a color map and the exact values. ๐ค
Data: [[1, 2], [3, 4]]
After Example: With heatmap and annotations, the data is color-coded and labeled! ๐ข
Output: A heatmap showing data values with annotations.
Challenge: ๐ Try changing the fmt
argument to display different formats for annotations.
Seaborn documentation
Subscribe to my newsletter
Read articles from Anix Lynch directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
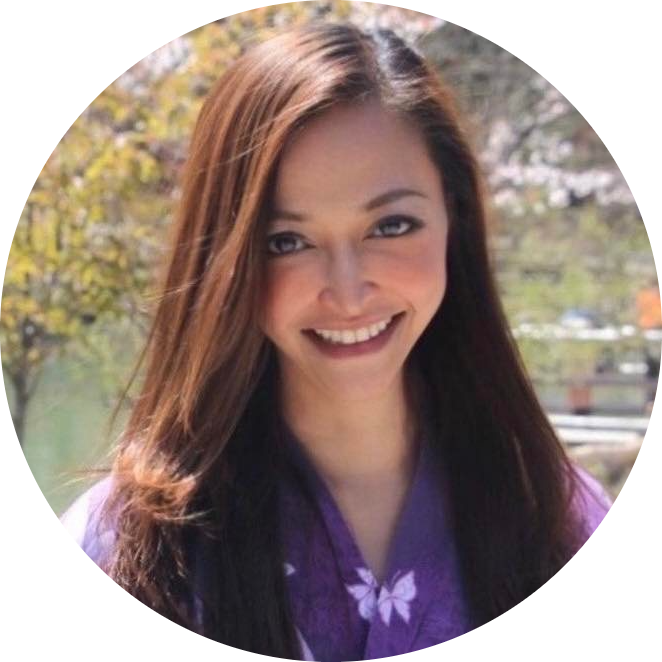