How to Set Up a React Development Environment: A Beginner's Guide
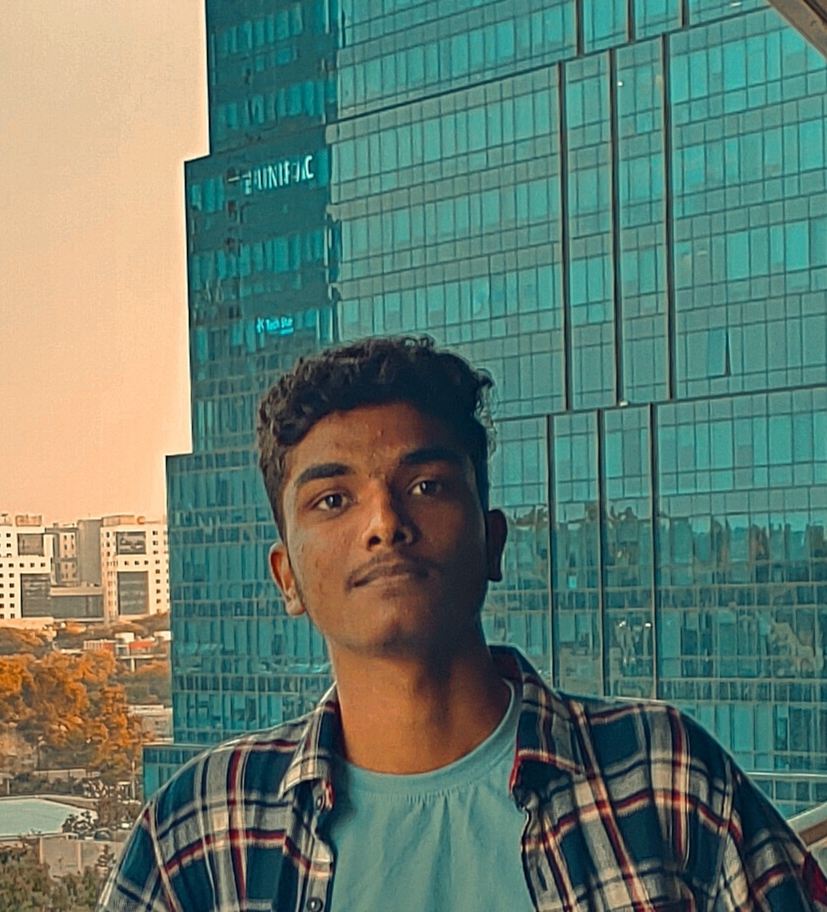
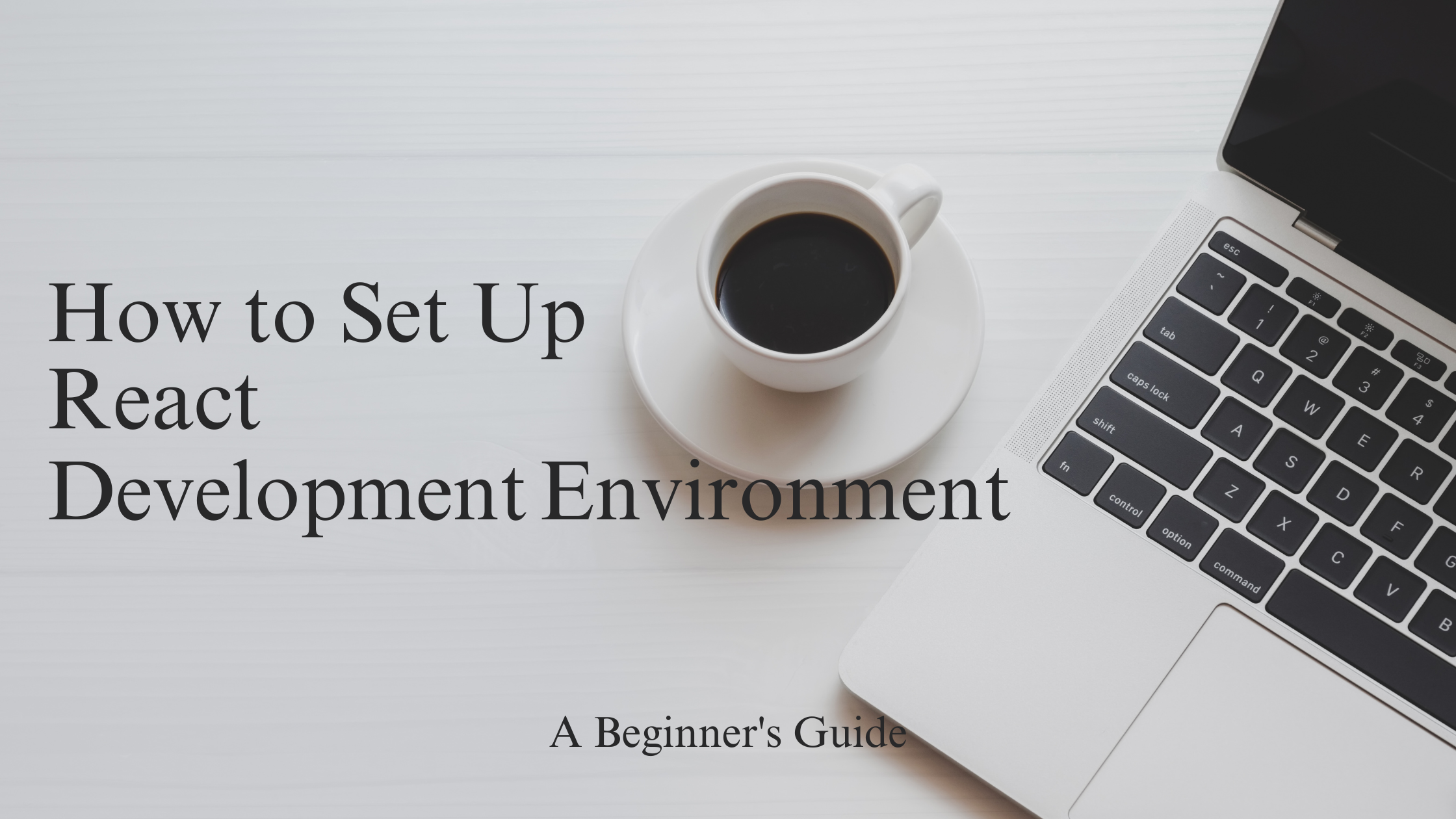
Tools and Libraries You’ll Need
To build a React application, there are a few core tools and libraries that are recommended:
1. Node.js and npm
Node.js is a JavaScript runtime that allows you to run JavaScript code on your machine outside the browser. Along with Node.js comes npm (Node Package Manager), which helps you install and manage external libraries and packages in your project.
Installation:
Download and install Node.js from the official website.
After installation check if Node.js and npm are properly installed by running the following commands in your terminal:
node -v npm -v
2. Create React App
The easiest way to get started with React is by using Create React App, a CLI (Command-Line Interface) tool that sets up a new React project with no configuration required. It includes everything you need to start building a React application right away, including a development server, Webpack.
To create a new React app:
Open your terminal and run the following command:
npx create-react-app my-app
Replace
my-app
with the name of your project.
Once the setup is complete, you can navigate to your project folder and start the development server by running:
cd my-app
npm start
3. Code Editor (VS Code)
While other code editor can be used to write React code, Visual Studio Code (VS Code) is highly recommended due to its lightweight nature, robust extensions, and customization options for JavaScript and React development.
VS Code Features:
IntelliSense for auto-completion and smart suggestions.
Extensions like ESLint, Prettier, and React snippets make development easier and more efficient.
Built-in terminal for running commands directly within the editor.
You can download VS Code from the official site.
4. Browser Developer Tools
For any web development, browser developer tools are essential for inspecting and debugging your application in real-time. Both Chrome and Firefox come with built-in developer tools that allow you to monitor network activity, view elements, debug JavaScript, and more.
Additionally, the React Developer Tools browser extension helps you inspect React components, view their props and state, and debug your React application more effectively.
You can install React Developer Tools for:
Key Libraries for React Development
Besides the basic setup, there are several key libraries that enhance your React development experience:
1. React Router
React Router is a powerful library for creating navigational components in a single-page application. It allows you to define multiple routes in your app and render different components based on the URL.
To install React Router, run:
npm install react-router-dom
2. Axios
If your application needs to interact with APIs, Axios is a popular library for making HTTP requests. It simplifies fetching data and comes with built-in features for handling errors and making asynchronous requests.
To install Axios, run:
npm install axios
Best Practices for Setting Up a React Project
While setting up your environment, it’s important to keep the following best practices:
Folder Structure: Organize your project’s folder structure in a way that’s scalable as the app grows. A common structure looks like this:
my-app/ ├── public/ ├── src/ │ ├── components/ │ ├── assets/ │ ├── App.js │ └── index.js └── package.json
Use Version Control (Git): Always use Git or another version control system to manage your project. This ensures you can track changes, collaborate with others, and roll back to previous versions if necessary.
To initialize a Git repository:
git init
Install ESLint and Prettier(Optional): To maintain clean and consistent code, you can set up ESLint for linting and Prettier for automatic formatting. These tools ensure your codebase follows best practices and maintains readability.
To install ESLint and Prettier:
npm install eslint prettier
Conclusion
Setting up your React environment properly is the first step to a smooth and successful development experience. With tools like Create React App, VS Code, and browser developer tools, you’ll be well-equipped to build your applications efficiently. Additionally, using essential libraries like React Router and Axios will provide you with the functionality needed to build robust and dynamic web applications. Once your environment is ready, you're all set to start developing with React!
Subscribe to my newsletter
Read articles from Gondi Bharadhwaj Reddy directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
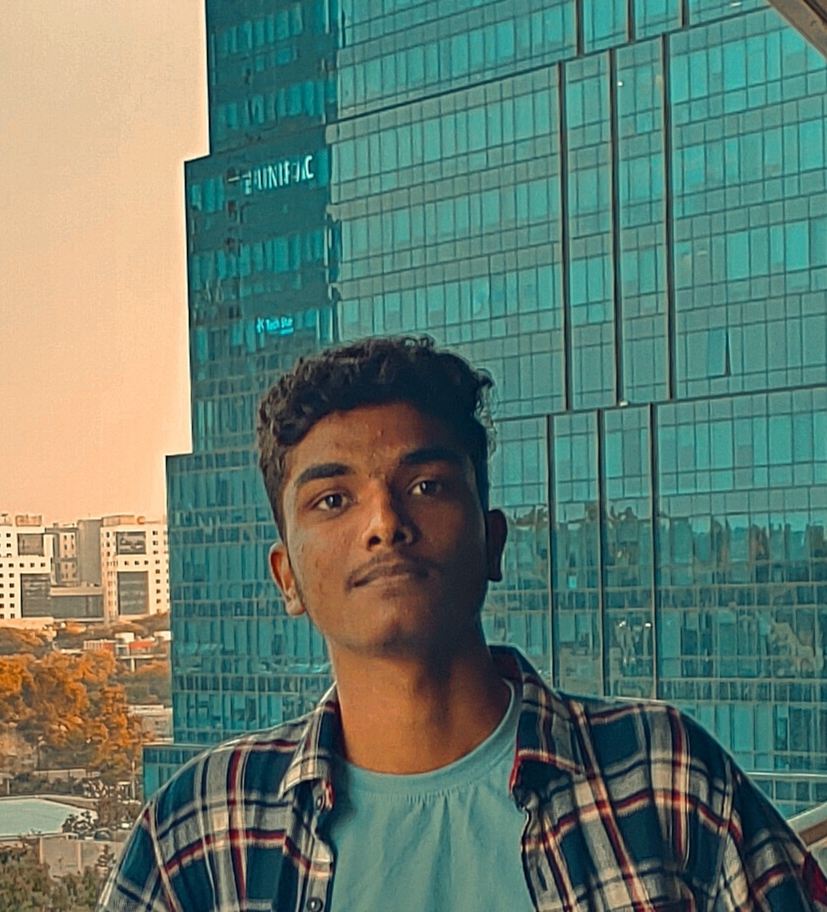
Gondi Bharadhwaj Reddy
Gondi Bharadhwaj Reddy
I am a dedicated undergraduate student passionate about exploring and learning new things. My interests span across various fields, from technology and web development. Currently focused on projects that enhance learning experiences and make meaningful contributions to communities.