Recursion in React: A Fun Factory of Components ππ

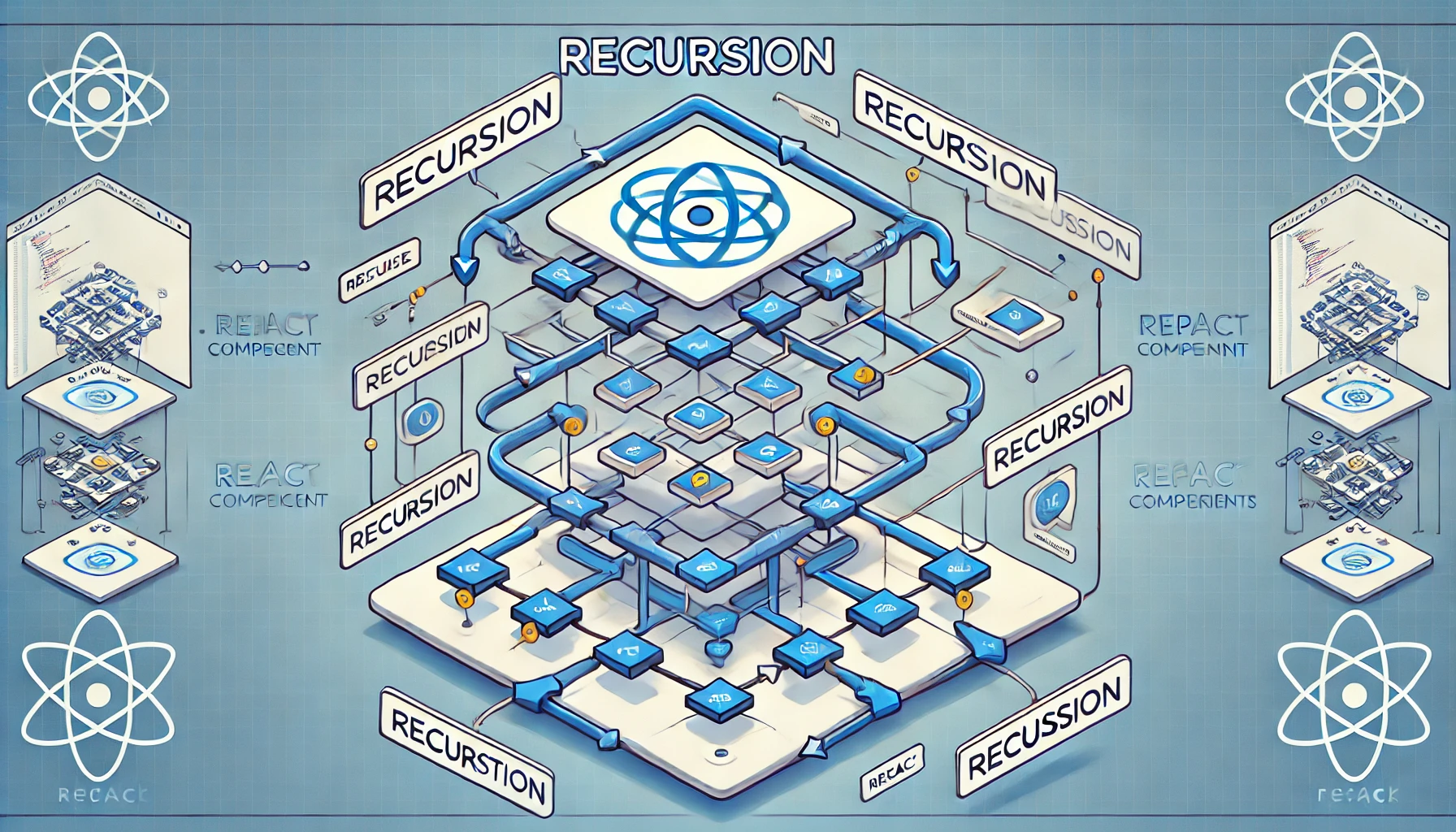
Recursion in React: A Fun Factory of Components
Hello, React fans! π Today, we're exploring the fascinating concept of recursion in Javascript. If that sounds a bit intimidating, don't worry β we'll simplify it and have some fun as we go!
What's Recursion, Anyway?
Imagine you're in a house of mirrors at a carnival. You see yourself reflected over and over, each time getting smaller. That's similar to recursion! In programming, it's when a function calls itself. In React, it's when a component renders itself. Pretty cool, right?
The RecursiveBox: Our Little Component Factory
Let's look at a fun example we call the RecursiveBox. Think of it as a magical box factory that can create boxes within boxes within boxes... you get the idea!
const RecursiveBox = ({ item }) => {
return (
<div style={{ /* some fancy styles */ }}>
<div>{item.containerId}</div>
<div>{item.elementDetails.name}</div>
<div>
{item.elementDetails.content.map((subItem) => (
<RecursiveBox item={subItem} />
))}
</div>
</div>
);
};
The RecursiveBox works like a skilled craftsman. It takes an item
and makes a box for it. The magic happens when the item has children (in item.elementDetails.content
); it calls itself to make boxes for those children too!
The Payload: Our Box-Making Instructions
Now, imagine you have a set of instructions for making boxes:
const payload = [
{
containerId: "__body",
elementDetails: {
content: [
{
containerId: "__body",
elementDetails: {
content: [],
id: 5434,
name: "<div>",
},
},
],
id: 1234,
name: "<div>",
},
},
];
This payload
is like a recipe for our box factory. It tells our RecursiveBox what boxes to make and what to put inside them.
The Magic Show: Putting It All Together
Now for the grand finale! We use our RecursiveBox to create a whole structure of boxes based on our payload:
const TestPage = () => {
return (
<div>
{payload.map((item) => (
<RecursiveBox item={item} />
))}
</div>
);
};
This TestPage is like the leader of our show. It takes our payload (the box-making instructions) and directs our RecursiveBox to begin creating!
Why Is This Cool?
It's Efficient: We write one component, and it can handle structures of any depth. It's like having one Swiss Army knife instead of a whole toolbox!
It's Flexible: Need to change how all boxes look? Just update the RecursiveBox component. One change affects everything!
It's Mind-Bending: It's a great way to visualize how complex UIs can be built from simple, repeating patterns.
Wrapping Up
Recursion in React is like having a magic wand that can build entire worlds with one spell. It might take some practice to get the hang of it, but once you do, you'll be making amazing, dynamic UIs in no time!
So go ahead, play around with the RecursiveBox. Change the styles, add new features, or create your own recursive components. The only limit is your imagination! Happy coding, and may your components always nest perfectly! ππβ¨
Connect with Me on Social Media π±
I love engaging with fellow developers and readers! If you have any questions, feedback, or just want to connect, feel free to reach out to me on social media. You can find me on Twitter and LinkedIn. I look forward to hearing from you and continuing the conversation! π
Subscribe to my newsletter
Read articles from Harsh Goswami directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harsh Goswami
Harsh Goswami
Harsh | Frontend Developer π¨βπ» Iβm a passionate frontend developer with 2 years of experience at Softcolon Pvt Ltd. I specialize in creating responsive, user-centric web applications using modern frameworks like React.js, Next.js, and Remix. Currently, Iβm focused on enhancing my skills in web development and exploring new technologies. When I'm not coding, youβll find me collaborating with developers worldwide, participating in hackathons, or working on my portfolio website. Iβm also considering starting a blog to share insights and connect with the frontend community. Letβs build amazing things together!