Hot Cup Of JavaScript
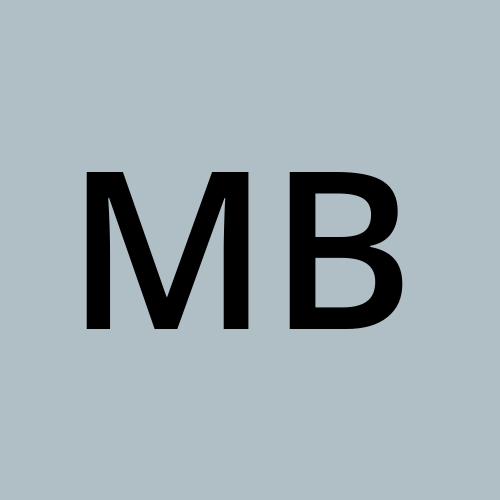
Table of contents
- Into
- History
- The Origins: JavaScript in 10 Days (1995)
- Browser Wars: Chaos and Confusion (1996-1999)
- Standardization and ECMAScript: The Early Days (1997-1999)
- The JavaScript Dark Ages (2000-2005)
- The Rise of AJAX and Web 2.0 (2005-2010)
- V8 Engine and Node.js: JavaScript Grows Up (2008-2009)
- ECMAScript 5: The Renaissance (2009)
- ES6 and Beyond: The Modern Era (2015-present)
- JavaScript Today: Ubiquitous and Unstoppable
- Wrapping It UP
- Before We Dive Deep
- Syntax
- 1. Variables
- 2. Data Types
- 3. Operators
- 4. Functions
- What are Functions?
- Declaring a Function
- Calling a Function
- Function Declaration:
- Calling the Function:
- Parameters and Arguments
- Return Statement
- Types of Functions
- Function Scope
- Best Practice
- 5. Conditionals
- if Statement
- else Statement
- else if Statement
- switch Statement
- Ternary Operator
- 6. Loops - Proceed With Coition
- Why Loops Are Important:
- Why Loops Can Be Dangerous:
- Common Causes of Infinite Loops:
- How to Prevent Infinite Loops:
- Example Problem:
- 7. Objects
- What are Objects?
- Why Do We Use Objects?
- Creating an Object
- How to Access Information in an Object?
- How to Add or Change Information in an Object? Modifying Objects
- Adding New Properties
- Deleting Properties
- Methods in Objects
- Calling Object Methods
- Nested Objects
- Looping Through an Object
- Checking for Property Existence
- Objects vs. Arrays
- Object.keys() and Object.values()
- Best Practices
- Summary of JavaScript Objects:
- 8. Arrays
- Why Do We Use Arrays?
- How Do We Create an Array?
- Accessing Items in an Array
- Changing or Adding Items
- Array Length
- Looping Through an Array
- Adding and Removing Items
- What Can Arrays Store?
- Why Arrays Are Useful
- In Summary:
- 9. Events
- Why Are Events Important?
- Common Types of Events
- How Do We Use Events?
- Adding an Event Listener
- Event Handler Functions
- Removing Event Listeners
- Event Object
- Event Propagation
- Why Events Are Useful
- In Summary:
- 10. Comments
- Why Comments Are So Important in JavaScript
- Summary of JavaScript Syntax Rules:
- Make Your Code Easy to Understand
- Help You Collaborate with Others
- Help You Learn
- Make Debugging Easier
- Document Complex Logic
- Types of Comments in JavaScript
- Avoid Over-commenting
- Self-documenting Code
- In Summary:
- Conclusion
- Thoughts Feelings and Storytime
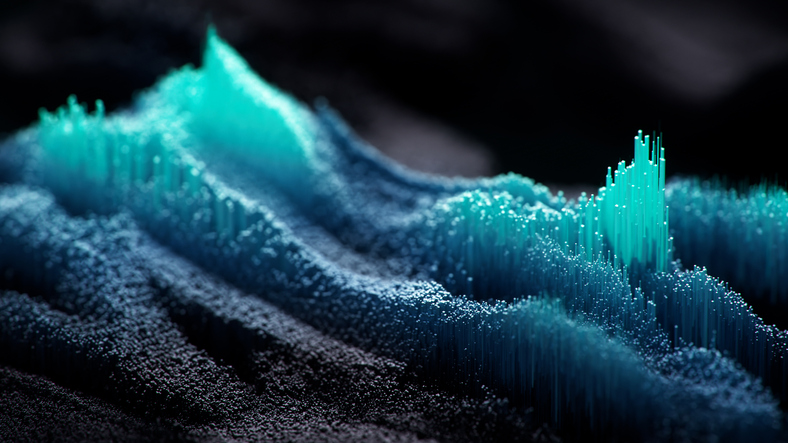
Into
Let me take a slightly different approach this time: a quick dive into the quirky, chaotic, and often misunderstood history of JavaScript. Why? Because this language is as versatile as it is polarizing. Whether you love it, tolerate it, or occasionally curse it, understanding how JavaScript evolved into the powerhouse it is today sheds light on the web’s transformation itself.
History
The Origins: JavaScript in 10 Days (1995)
In 1995, Brendan Eich created JavaScript in just 10 whirlwind days while working at Netscape Communications. Originally dubbed Mocha (and later LiveScript), it was rebranded to JavaScript in a clever marketing ploy to ride on the coattails of Java’s then-rising popularity. The goal? A lightweight, dynamic scripting language that would allow non-programmers to add interactivity to web pages, a revolutionary concept at the time.
Browser Wars: Chaos and Confusion (1996-1999)
As Netscape and Microsoft battled for browser supremacy, things got messy. Microsoft developed its own version of JavaScript, calling it JScript, which led to web developers pulling their hair out as they wrestled with cross-browser incompatibilities. In an effort to bring order to the madness, JavaScript was standardized by ECMA International, resulting in the creation of ECMAScript—the official specification for what we now call JavaScript.
Standardization and ECMAScript: The Early Days (1997-1999)
The late 90s saw the release of ECMAScript 1 (1997), ECMAScript 2 (1998), and ECMAScript 3 (1999). ECMAScript 3 was a game-changer, introducing features like exception handling and regular expressions, which made JavaScript significantly more capable, even though many still viewed it as a toy language for web animations and form validation.
The JavaScript Dark Ages (2000-2005)
The early 2000s were not kind to JavaScript. Browsers were inconsistent, and developers often turned to plugins like Flash for anything beyond basic web interactions. JavaScript wasn’t dead, but it certainly wasn’t thriving. However, behind the scenes, developers were beginning to use asynchronous programming techniques, laying the groundwork for the next phase of its evolution.
The Rise of AJAX and Web 2.0 (2005-2010)
Enter the AJAX revolution in 2005. Suddenly, web pages could update dynamically without reloading, giving birth to the era of Web 2.0. JavaScript was now at the forefront, driving interactive and dynamic web apps like Google Maps and Gmail. Around this time, JavaScript libraries like jQuery emerged, smoothing over browser inconsistencies and making the language far more accessible.
V8 Engine and Node.js: JavaScript Grows Up (2008-2009)
In 2008, Google introduced the V8 engine, a high-performance JavaScript engine that significantly sped up the execution of JavaScript code. Then, in 2009, Node.js came along, thanks to Ryan Dahl. With Node.js, JavaScript was no longer confined to the browser—it could now be used server-side, making it a truly full-stack language and opening up a world of possibilities.
ECMAScript 5: The Renaissance (2009)
ECMAScript 5 arrived in 2009, bringing with it improvements like strict mode, native JSON support, and more robust array methods. These updates weren’t revolutionary, but they paved the way for JavaScript’s future by cleaning up its quirks and making it more reliable for large-scale applications.
ES6 and Beyond: The Modern Era (2015-present)
ECMAScript 6 (ES6), released in 2015, was a seismic shift. The introduction of modern features like arrow functions, classes, modules, let/const for variable scoping, and Promises for handling asynchronous code turned JavaScript into a much more sophisticated and scalable language. This update fundamentally altered the way developers wrote JavaScript, making it feel less like a toy and more like a mature, powerful tool.
Subsequent annual updates—ES7, ES8, and beyond—added even more powerful features, such as async/await, object spread/rest, and dynamic imports, solidifying JavaScript’s place as the backbone of modern web development.
JavaScript Today: Ubiquitous and Unstoppable
Today, JavaScript is everywhere: in browsers, on servers (thanks to Node.js), in mobile apps (with frameworks like React Native), and even in IoT devices. With a vibrant ecosystem of frameworks like React, Vue.js, and Angular, JavaScript isn’t just surviving—it’s thriving. Tools like Webpack, Babel, and npm have become essential for managing JavaScript projects and keeping pace with its rapid evolution.
Wrapping It UP
JavaScript’s journey from a hastily-written browser toy to the cornerstone of the web is a testament to its adaptability. It may have started with humble beginnings, but today, JavaScript is the indispensable glue holding together the modern web. So, whether you embrace it or grumble while using it, understanding how JavaScript became what it is today will make you appreciate its quirks and, perhaps, even its genius.
Before We Dive Deep
Let me start with a quick disclaimer: I’m not sure how deeply I can guide you toward a full grasp of everything with just text alone. But, I will provide as much sample code as I can, because when it comes to learning programming, code examples are often worth more than a thousand words. A well-placed example can make a concept crystal clear in ways that paragraphs of explanation can’t.
With JavaScript especially, practice is everything. It's a language that’s deceptively simple on the surface, but to truly grasp the fundamentals and get comfortable with it, you’ll need to dive in and start experimenting. The good news is, it’s relatively easy to pick up with dedication, time, and hands-on experience.
The best advice I can offer: get comfortable by playing around with code in a live editor. Tinker with it, break it, fix it, and break it again—that’s how you’ll build a real understanding. Nothing beats the hands-on approach when it comes to mastering JavaScript.
Syntax
JavaScript syntax is the set of rules that define how JavaScript programs are written and interpreted by browsers and other environments. To get a full understanding of JavaScript syntax, we’ll break it down into several core parts, covering the basics of how JavaScript code is structured and executed.
1. Variables
What are Variables?
Variables are like containers that store data values. You can assign and retrieve information using variable names.
Declaring Variables
In JavaScript, you declare variables using:
var
: Old way of declaring variables, less commonly used now.let
: Used for variables that can change.const
: Used for constants that shouldn’t change.
Key Differences: var
, let
, and const
var
: Can be updated and re-declared, but has some quirks due to being function-scoped.let
: Block-scoped (works only within its block) and can be updated but not re-declared.const
: Block-scoped, and once assigned, it can’t be changed (except for objects/arrays, where contents can still be modified).
Variable Naming Rules
Must start with a letter, underscore (
_
), or dollar sign ($
).Case-sensitive (e.g.,
myVariable
andmyvariable
are different).Cannot use reserved words like
let
,if
,function
, etc.
Uninitialized Variables
Variables declared without a value default to undefined
.
Variable Scope
Global Scope: Variables declared outside any function/block are accessible everywhere.
Local (Block) Scope: Variables declared inside a block (e.g., a loop or function) are only accessible within that block.
Hoisting
JavaScript moves variable declarations to the top of their scope before execution. However, let
and const
are not initialized until the line of code is actually executed, so they cannot be used before they are declared.
Best Practice
Use let
for variables that can change and const
for constants or values that should remain the same. Avoid using var
in modern JavaScript to prevent issues with scope and hoisting.
Example:
var x = 5;
let y = 10;
const z = 15;
x
andy
can be changed later, butz
cannot.
2. Data Types
JavaScript has various data types, and these are important for how you structure and manipulate data.
Primitive Data Types:
Number: Represents numeric values (e.g.,
5
,3.14
)String: Represents text (e.g.,
"Hello"
)Boolean: Represents logical values
true
orfalse
Undefined: A variable that has been declared but not assigned a value.
Null: Represents an intentional absence of any object value.
Symbol: Introduced in ES6, used to create unique identifiers.
Non-primitive Data Types:
Objects: Complex data structures that store collections of values.
Arrays: Special objects used to store lists of values.
Example:
let age = 30; // Number
let name = "Alice"; // String
let isStudent = true; // Boolean
let colors = ["red", "blue"]; // Array
3. Operators
Operators in JavaScript are used to perform operations on variables and values.
Arithmetic Operators:
+
: Addition-
: Subtraction*
: Multiplication/
: Division%
: Modulus (remainder)
Example:
let sum = 10 + 5; // sum is 15
let remainder = 10 % 3; // remainder is 1
Comparison Operators:
==
: Equal to (compares values, but not types)===
: Strict equal to (compares both value and type)!=
: Not equal to!==
: Strict not equal to>
: Greater than<
: Less than
Example:
let result = (5 == "5"); // true (compares only value)
let strictResult = (5 === "5"); // false (compares value and type)
4. Functions
What are Functions?
Functions are reusable blocks of code that perform specific tasks. You define a function once and can "call" or "execute" it whenever you need that task to be done.
Declaring a Function
You define a function by giving it a name, specifying any input (called parameters), and writing the code it should execute.
Calling a Function
Once a function is declared, you call (or "invoke") it by using its name followed by parentheses ()
.
Function Declaration:
function sayHello() {
console.log("Hello, world!");
}
Calling the Function:
sayHello(); // This will output: "Hello, world!"
Parameters and Arguments
Parameters are placeholders used when defining a function.
Arguments are the actual values you pass to the function when you call it.
Return Statement
Functions can optionally return a value using the return
statement. Once the function reaches return
, it stops executing and gives back a result.
Types of Functions
Regular Functions: Declared using the
function
keyword.Anonymous Functions: Functions without a name, often used in other parts of the code (like as event handlers).
Arrow Functions: A more concise way of writing functions, introduced in ES6.
Function Scope
Variables defined inside a function are local to that function and can't be accessed outside of it.
Best Practice
Name functions clearly to reflect their purpose.
Use arrow functions for concise code.
Always check whether a function needs to return something or just perform an action.
Syntax:
function functionName(parameters) {
// code to be executed
}
Example:
function greet(name) {
return "Hello, " + name;
}
console.log(greet("Alice")); // Output: "Hello, Alice"
You can also create arrow functions (introduced in ES6), which are a more concise way to write functions.
Example:
const greet = (name) => "Hello, " + name;
console.log(greet("Bob")); // Output: "Hello, Bob"
5. Conditionals
Conditionals allow JavaScript to make decisions and execute different code depending on whether a condition is true or false.
if
Statement
The most basic conditional.
Runs a block of code if a given condition is true.
else
Statement
Used with
if
.Runs a block of code if the
if
condition is false.
else if
Statement
Used when you have multiple conditions.
Runs a different block of code if the first condition is false, but another condition is true.
switch
Statement
A cleaner way to handle multiple possible conditions (instead of using many
else if
statements).Evaluates a variable or expression and matches it to a case, running the corresponding block of code.
Ternary Operator
A shorthand way to write an
if-else
statement.Syntax:
condition ? valueIfTrue : valueIfFalse;
Syntax:
if (condition) {
// code to execute if the condition is true
} else {
// code to execute if the condition is false
}
Example:
let age = 18;
if (age >= 18) {
console.log("You are an adult.");
} else {
console.log("You are a minor.");
}
6. Loops - Proceed With Coition
Loops allow you to repeat the same block of code multiple times. JavaScript has several types of loops:
for
loop: The most common loop for repeating a block of code a specific number of times.while
loop: Loops as long as the condition is true.do...while
loop: Similar towhile
, but the code runs at least once.
for
Loop Example:
for (let i = 0; i < 5; i++) {
console.log(i);
}
// Output: 0, 1, 2, 3, 4
while
Loop Example:
let i = 0;
while (i < 5) {
console.log(i);
i++;
}
Why Loops Are Important:
Efficiency: Instead of writing repetitive code manually, loops allow you to perform the same task multiple times with minimal effort.
- Example: Looping through a list of items, processing each one.
Automation: Loops help automate tasks that involve repeating operations, like calculations, data processing, or updating UI elements.
Scalability: With loops, you can handle large amounts of data efficiently by processing one item at a time without having to manually write code for each individual item.
Why Loops Can Be Dangerous:
Infinite Loops: If a loop doesn’t have a proper exit condition (a condition that stops the loop), it will run forever. This is called an infinite loop, and it can cause serious issues like:
Program Freeze: The program might stop responding because it’s stuck running the loop continuously.
Performance Problems: Infinite loops can use up memory and processing power, potentially crashing the browser or system.
Common Causes of Infinite Loops:
Forgetting to update the loop’s counter or condition.
Setting a condition that’s always true (e.g.,
while (true)
).Using incorrect comparison operators that don’t allow the loop to terminate.
How to Prevent Infinite Loops:
Always ensure that the loop’s condition will eventually become false.
Be careful with
while
loops, as they rely on the condition being met to terminate.Test your loop logic with small data sets first to ensure it behaves as expected.
Example Problem:
In a for
loop, if you forget to update the loop counter (like `i++), the loop will run forever:
for (let i = 0; i < 10;) {
console.log(i); // No update to 'i', so the loop never ends
}
Loops are powerful tools, but if not handled carefully, they can cause issues in your program’s execution. Always make sure they have a clear condition to stop running.
7. Objects
What are Objects?
Objects in JavaScript are collections of key-value pairs. They allow you to store multiple related values (data) in a structured way. An object can represent anything, like a person, a car, or a book, with various properties (keys) that describe it.
Think of an object as a box that holds different pieces of related information. Each piece of information in the box is labeled with a key (like a label on a drawer), and each key has a value (what’s inside the drawer).
For example, if you had a box that represents a car, inside the box you might have keys like "make", "model", and "year", and their corresponding values might be "Toyota", "Camry", and 2020.
Why Do We Use Objects?
Objects let us group related data together. Instead of having separate variables for every bit of information (like one for car make, one for model, etc.), we can keep everything organized inside a single object.
Imagine keeping a list of a hundred cars with their makes, models, and years. If you didn’t use objects, it would be messy! Objects help us keep everything in one place and make it easier to work with.
Syntax:
let objectName = {
key1: value1,
key2: value2,
// more key-value pairs
};
Example:
let person = {
name: "Alice",
age: 30,
isStudent: false
};
console.log(person.name); // Output: "Alice"
console.log(person.age); // Output: 30
Creating an Object
There are a few ways to create objects in JavaScript, but the most common is using object literals.
Example:
let car = {
make: "Toyota",
model: "Corolla",
year: 2020
};
In this object:
The keys are
make
,model
, andyear
.The values are
"Toyota"
,"Corolla"
, and2020
.
How to Access Information in an Object?
You can get the values from an object using dot notation or bracket notation.
Dot Notation:
car.make
gives you"Toyota"
.Bracket Notation:
car["year"]
gives you2020
.
Example:
console.log(car.make); // Output: "Toyota" (dot notation)
console.log(car["year"]); // Output: 2020 (bracket notation)
How to Add or Change Information in an Object? Modifying Objects
You can easily update the properties of an object by assigning new values.
Example:
car.model = "Camry"; // Changes the model to "Camry"
car["year"] = 2021; // Changes the year to 2021
Adding New Properties
You can add new properties to an existing object by simply assigning them.
Example:
car.color = "Red"; // Adds a new property 'color'
Deleting Properties
To remove a property from an object, you use the delete
keyword.
Example:
delete car.year; // Removes the 'year' property from the car object
Methods in Objects
Objects can also store functions (actions) that they can perform. These functions are called methods. These methods can perform actions using the object’s data.
Example:
let person = {
firstName: "John",
lastName: "Doe",
fullName: function() {
return this.firstName + " " + this.lastName;
}
};
Here, fullName
is a method, and this
refers to the current object (person
).
Calling Object Methods
You can call methods in an object just like you call a regular function, but with the object's name.
Example:
console.log(person.fullName()); // Output: "John Doe"
Nested Objects
You can have objects inside other objects, which is useful for representing more complex data.
Example:
let student = {
name: "Alice",
address: {
city: "New York",
zip: 10001
}
};
Here, address
is another object inside the student
object.
Looping Through an Object
To loop through all the properties of an object, you can use a for...in
loop.
Example:
for (let key in car) {
console.log(key + ": " + car[key]);
}
This will output all key-value pairs in the car
object.
Checking for Property Existence
You can check if a property exists in an object using the in
operator or hasOwnProperty
method.
Example:
console.log("make" in car); // true
console.log(car.hasOwnProperty("year")); // false (if 'year' was deleted earlier)
Objects vs. Arrays
Objects store data as key-value pairs (unordered), making them great for storing data where the relationships matter.
Arrays store data in an ordered list, ideal for sequences of items where position is important.
Object.keys()
and Object.values()
These methods return an array of an object’s keys or values.
Example:
let keys = Object.keys(car); // ["make", "model", "color"]
let values = Object.values(car); // ["Toyota", "Camry", "Red"]
Best Practices
Keep property names meaningful and descriptive.
Use methods to group related functionality with the object’s data.
Use dot notation for simple access and bracket notation when property names are dynamic or have special characters.
Summary of JavaScript Objects:
Objects are key-value pairs that can hold related data.
Properties can be accessed, modified, added, or deleted using dot or bracket notation.
Methods are functions that operate within the object.
Use objects to model more complex data or entities in your code.
8. Arrays
An array is like a list that can hold multiple values. Instead of having lots of separate variables, you can store all related values in one place—a list (or array).
Think of an array like a row of numbered lockers. Each locker (position in the array) holds a different item, and you can access the items by their position (starting from 0).
Syntax:
let arrayName = [item1, item2, item3];
For example, if you had an array of your favorite fruits:
let fruits = ["apple", "banana", "cherry"];
Here:
fruits[0]
is"apple"
,fruits[1]
is"banana"
,fruits[2]
is"cherry"
.
Why Do We Use Arrays?
Arrays help us organize lists of related data in a single, structured way. Instead of having multiple variables like fruit1
, fruit2
, fruit3
, you can store all your fruits in one array.
How Do We Create an Array?
You create an array by putting values inside square brackets []
, separated by commas.
Example:
let colors = ["red", "green", "blue"];
Accessing Items in an Array
You can get the values from an array by using the index number. Remember, array positions start at 0, not 1.
Example:
let firstColor = colors[0]; // "red"
let secondColor = colors[1]; // "green"
Changing or Adding Items
You can change the value of an item by assigning a new value to an index, or you can add new items to the array.
Example:
colors[1] = "yellow"; // Changes the second color to "yellow"
colors.push("purple"); // Adds "purple" to the end of the array
Now, the colors
array looks like this: ["red", "yellow", "blue", "purple"]
.
Array Length
Every array has a length, which tells you how many items are in the array. You can access it using the .length
property.
Example:
let numberOfFruits = fruits.length; // 3 (since we have 3 fruits in the array)
Looping Through an Array
You often use loops to go through all the items in an array, especially when there are many items.
Example:
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]); // Outputs each fruit in the array
}
Adding and Removing Items
push()
: Adds an item to the end of the array.pop()
: Removes the last item from the array.shift()
: Removes the first item from the array.unshift()
: Adds an item to the beginning of the array.
Example:
fruits.push("orange"); // Adds "orange" to the end
fruits.pop(); // Removes the last item (in this case, "orange")
What Can Arrays Store?
Arrays can store any type of data: numbers, strings, objects, or even other arrays! For example:
let mixedArray = [1, "hello", true, { key: "value" }, [1, 2, 3]];
Why Arrays Are Useful
They make it easy to store multiple items in one place.
Arrays are great for organizing lists of data, like names, numbers, or objects.
You can quickly add, remove, and access items by their position (index) in the array.
In Summary:
Arrays are like lists that store multiple values in a single variable.
You access items by their index number (starting from 0).
You can easily add, change, or remove items from arrays.
Arrays are useful when you have a group of related data that you want to keep together.
9. Events
An event is something that happens in the browser that JavaScript can respond to. It could be a user clicking a button, typing into a form, moving the mouse, or even when a web page finishes loading.
Think of events as triggers. When something happens, you can tell JavaScript what to do in response.
Why Are Events Important?
Events make web pages interactive. Without events, web pages would just sit there, and users wouldn't be able to interact with them. Events let you add dynamic behavior to a website, like responding to button clicks or submitting forms.
Example:
<button id="myButton">Click me</button>
<script>
document.getElementById("myButton").addEventListener("click", function() {
alert("Button was clicked!");
});
</script>
In this example, when the button is clicked, a message will pop up.
Common Types of Events
Here are a few common events you’ll deal with in JavaScript:
click
: When the user clicks on something (like a button).mouseover
: When the user moves the mouse over an element.keydown
: When the user presses a key on the keyboard.submit
: When a form is submitted.load
: When a web page has fully loaded.
How Do We Use Events?
To use events in JavaScript, you need two things:
The element you want to listen for an event on (like a button).
An event listener that tells JavaScript what to do when the event happens.
Adding an Event Listener
You can add an event listener to an element using JavaScript’s addEventListener()
method. This method "listens" for a specific event and runs a function when that event occurs.
Example:
let button = document.querySelector("button"); // Selects the button element
button.addEventListener("click", function() {
alert("Button was clicked!");
});
In this example:
We selected a button element using
document.querySelector()
.We used
addEventListener()
to listen for a"click"
event.When the button is clicked, an alert pops up saying "Button was clicked!"
Event Handler Functions
The function that runs when an event happens is called an event handler. You can either define the function directly inside addEventListener()
, or define it separately and pass it in.
Removing Event Listeners
Sometimes, you might want to stop listening for an event. You can use removeEventListener()
to stop the event handler from running.
Event Object
When an event occurs, JavaScript creates an event object that contains information about the event, like where it happened, which key was pressed, etc. You can access this information inside your event handler.
Example:
button.addEventListener("click", function(event) {
console.log(event); // Logs details about the click event
});
Event Propagation
Events can "bubble up" through the DOM tree, meaning if you click on a child element, the event can also trigger on its parent elements. This is called event bubbling. You can control how events propagate by using methods like stopPropagation()
.
Why Events Are Useful
Events are essential for building interactive web applications. They let you:
Respond to user interactions (clicks, typing, scrolling, etc.).
Handle form submissions or other changes on the page.
Create dynamic user experiences where things happen in response to what the user does.
In Summary:
Events are actions or occurrences (like clicking, typing, or loading) that happen in the browser.
You use event listeners to respond to these events.
The event handler function is the code that runs when the event occurs.
Events let you make web pages interactive and respond to what users do.
10. Comments
Comments are used to explain code or make it easier to read. They are ignored by the browser.
Single-line comment:
//
Multi-line comment:
/* ... */
Example:
// This is a single-line comment
let x = 5; // Another comment
/*
This is a multi-line comment
It can span multiple lines
*/
let y = 10;
Why Comments Are So Important in JavaScript
Comments are one of the most essential yet often underrated aspects of writing code. In JavaScript (and other programming languages), comments are lines of text in your code that JavaScript ignores when running the program. They are meant for humans reading the code, not for the computer.
Summary of JavaScript Syntax Rules:
JavaScript is case-sensitive.
let name
andlet Name
are two different variables.Statements typically end with a semicolon (
;
), though it's not always required due to automatic semicolon insertion.Curly braces
{}
define code blocks, like in functions, conditionals, and loops.Data is passed between JavaScript code and the browser using variables, arrays, and objects.
The syntax might look like just rules, but once you start practicing, it becomes second nature. It's the foundation for writing clean, efficient JavaScript!
Make Your Code Easy to Understand
When you write code, it may make perfect sense to you at the moment, but when you or someone else comes back to it days, weeks, or months later, it might be hard to remember or understand why you wrote certain pieces of code. Comments explain the "why" behind your code.
Example:
let totalPrice = itemPrice * quantity; // Calculate the total cost of items
Without the comment, someone might not immediately understand what the line is doing, but the comment makes it clear.
Help You Collaborate with Others
When working on a team, you’re often sharing code with other developers. Well-written comments can explain what certain parts of the code do and how they fit together, so team members can understand it faster and work more effectively.
Good comments help avoid miscommunication and reduce confusion.
Help You Learn
If you’re learning JavaScript, writing comments can help you reinforce your understanding of how things work. Writing comments in your code can be like taking notes—explaining what each part of the code does in plain English. This helps solidify concepts and make your thought process clear.
Make Debugging Easier
When you’re debugging code (finding and fixing errors), comments can be extremely helpful. You can use comments to explain which parts of the code you’ve tested, which are still in progress, and where you think the problem might be.
Also, you can temporarily comment out parts of your code to see how your program behaves without them, which is useful for isolating bugs.
Document Complex Logic
Sometimes your code will involve complicated logic, tricky calculations, or unusual edge cases. In these cases, comments are crucial for explaining why certain decisions were made or why you’re handling things in a particular way.
Example:
// Adding 1 to avoid division by zero error
let safeValue = value + 1;
Without this comment, someone might wonder why you’re adding 1. The comment clarifies the reasoning.
Types of Comments in JavaScript
Single-line Comments:
Single-line comments start with //
and everything after //
on that line is ignored by JavaScript.
Example:
// This is a single-line comment
let userName = "John";
Multi-line Comments:
Multi-line comments start with /*
and end with */
. Everything between /*
and */
is treated as a comment.
Example:
/*
This is a multi-line comment
It can span across multiple lines
*/
let userAge = 25;
Avoid Over-commenting
While comments are important, over-commenting can make your code messy and harder to read. You don’t need to explain every single line of code—focus on explaining why something is done rather than what each line does (if it’s obvious from the code itself).
Self-documenting Code
Good code should be as self-explanatory as possible. Using meaningful variable and function names can reduce the need for comments. For example:
Instead of:
let x = 10; // Number of items
Use:
let numberOfItems = 10;
Here, the variable name explains itself, and you might not even need a comment.
In Summary:
Comments explain what your code does and why you wrote it that way, making it easier to understand.
They help you, your future self, and others who read your code.
Comments are crucial for collaboration, learning, and debugging.
Don’t over-comment; focus on explaining why rather than what.
Comments are a small but powerful part of writing clean, understandable, and maintainable code!
Conclusion
I gave a brief history of JavaScript. I covered key concepts in JavaScript, explaining both the syntax and their meanings while giving simple examples. To sum it all up JavaScript is a dynamic, versatile programming language that's crucial for making web pages interactive and responsive. Here's a quick recap of the most important concepts:
Variables: Store data that can change, using keywords like
var
,let
, andconst
.Functions: Blocks of code that perform specific tasks. You can define them once and reuse them to keep your code clean and efficient.
Conditionals: These allow you to make decisions in your code. Using
if
,else
, andswitch
statements, you can control what your program does under different conditions.Loops: Help you repeat a block of code multiple times. However, loops need to be controlled carefully to avoid infinite loops, which can crash your program.
Objects: Structures that group related data and functions together, making it easy to work with complex information. Objects have properties (data) and methods (actions).
Arrays: Special types of objects that store lists of items. Arrays are useful for organizing multiple values in a structured way.
Events: Actions like clicks or key presses that your code can respond to. Using event listeners, you can create interactive websites by triggering code to run when these events happen.
Comments: Crucial for explaining your code and making it easier to understand for yourself and others. Comments are ignored by JavaScript but are essential for documenting your thought process and making your code readable.
Thoughts Feelings and Storytime
Lets do Storytime first. Back when I was in the computer program where I built a computer from scratch. It was one of those moments where every bit of knowledge felt like gold, and I didn’t want to miss a second. But there was one tiny problem: I really had to go to the restroom. You know that feeling—you’re so engrossed in what you're doing, you start bargaining with your bladder like it’s a negotiable contract. Plus, being a teenager, the last thing I wanted was to interrupt the flow (pun intended) of learning.
I tried to tough it out, but eventually, I reached that point where you just can’t sit still anymore. It must’ve been pretty obvious because my instructor, noticing my discomfort, asked if I was alright. I admitted to my predicament, and he graciously excused me.
Now, here’s the twist. When I returned, visibly relieved, we had a small life lesson. My instructor explained how unhealthy it is to neglect basic human needs, and I confessed how much better I felt. Cue the peanut gallery—one of the kids in class joked that I was being dramatic about how good it felt. That’s when the instructor chimed in with a nugget of wisdom: sometimes, fulfilling our most basic needs—whether it’s scratching an itch, relieving ourselves, or just cleaning out our ears—can bring immense relief and satisfaction. Moral of the story: Life’s little pleasures are not to be overlooked. Sometimes, it’s the small, simple things that make you feel the best.
Still riding the high of this journey, I attended another meetup for ColumbusJS, and this time they delved into React Native—which, let me tell you, couldn’t be more perfect for me. I’ve been wanting to dive into cross-platform app development, and React Native is a powerhouse for that. This session was especially cool because it was super interactive. We used an app called Expo, which made things next-level fun. Everyone in the room downloaded it, and the speaker was able to run his code on his laptop, which then magically appeared on all our phones—both Android and Apple—in real time! Pretty slick.
I got to meet some awesome people, including the guy who organized the meetup. He mentioned needing to update the website for future meetups but said he didn’t have the time or funds to hire someone. That’s when I stepped up and offered to do it for the experience points. We exchanged emails, and I shot him a message as soon as I got home. Now I’m waiting for him to get back to me, and I can’t wait to dive into my first real project. I’m all in—ready to give it everything I’ve got!
Final note: I was moving a bit too fast while setting up my series, but I finally figured out how to add the old articles. Like I always say, programming is all about problem-solving, and I’m getting better at it every day.
Subscribe to my newsletter
Read articles from Michael Baisden directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
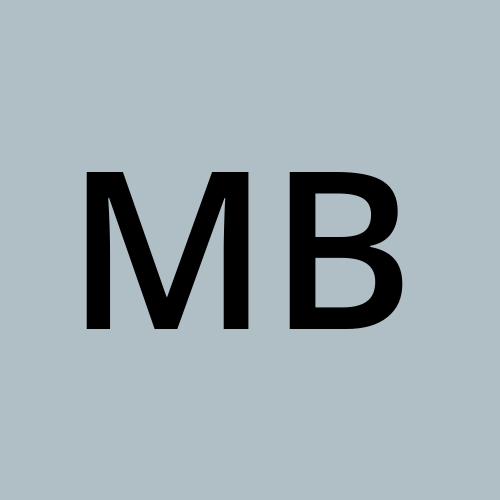
Michael Baisden
Michael Baisden
I am a developer from Columbus, Ohio, born and raised. I've always been into coding and working with computers. At the end of July 2024, I decided to teach myself how to program and write code. I love video games and music. I also love food—cooking, eating, and sharing. I am a God-loving man who keeps an open mind and loves to learn. I have a loving, caring girlfriend named Kerry and a cute dachshund named Kobe. When I'm not working or coding, I'm usually with them.