Unlocking CSS: Learn Through 100 Questions and Answers
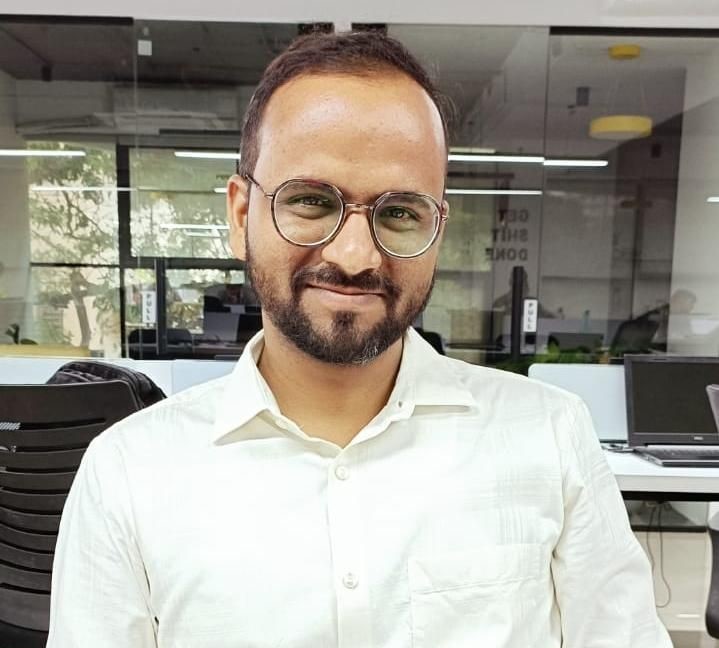

1. What does CSS stand for?
➡️ CSS stands for Cascading Style Sheets.
2. How do you add CSS to a webpage?
Inline CSS:
<h1 style="color:blue;">This is a heading</h1>
➡️ Adds CSS directly to an HTML element.
Internal CSS:
<style>
h1 { color: blue; }
</style>
➡️ Defines styles within a <style>
tag in the <head>
section.
External CSS:
<link rel="stylesheet" type="text/css" href="styles.css">
➡️ Links an external CSS file.
3. What is the CSS box model?
➡️ The box model describes how elements are structured: content, padding, border, and margin.
div {
width: 300px;
padding: 20px;
border: 5px solid black;
margin: 15px;
}
4. How do you select an element by ID in CSS?
#myId {
color: red;
}
➡️ Selects an element with the ID "myId."
5. How do you select elements by class in CSS?
.myClass {
background-color: yellow;
}
➡️ Selects all elements with the class "myClass."
6. What is a CSS selector?
➡️ A CSS selector is a pattern used to select the elements you want to style.
7. How do you apply styles to multiple elements?
h1, h2, h3 {
font-family: Arial, sans-serif;
}
➡️ Applies the same style to multiple elements.
8. What is the difference between padding
and margin
?
➡️ Padding
is the space between the content and the border, while margin
is the space outside the border.
9. How do you center a block element?
.center {
width: 50%;
margin: 0 auto;
}
➡️ Centers a block element with a specified width.
10. What is the purpose of the display
property?
➡️ The display
property defines how an element is displayed on the page.
div {
display: block; /* or inline, inline-block, flex, grid, etc. */
}
11. How do you make an element a flex container?
.flex-container {
display: flex;
}
➡️ Makes the element a flex container.
12. How do you create a responsive layout using CSS?
.responsive {
width: 100%;
max-width: 1200px;
margin: 0 auto;
}
➡️ Creates a responsive container.
13. What are media queries in CSS?
➡️ Media queries allow you to apply styles based on the viewport size or device characteristics.
@media (max-width: 600px) {
body {
background-color: lightblue;
}
}
14. How do you change the font of an element?
p {
font-family: 'Arial', sans-serif;
font-size: 16px;
}
➡️ Changes the font family and size of a paragraph.
15. What are CSS pseudo-classes?
➡️ Pseudo-classes are used to style elements based on their state or position.
a:hover {
color: green;
}
➡️ Changes the color of a link when hovered over.
16. What are CSS pseudo-elements?
➡️ Pseudo-elements allow you to style a specific part of an element.
p::first-line {
font-weight: bold;
}
➡️ Styles the first line of a paragraph.
17. How do you use the z-index
property?
.overlay {
position: absolute;
z-index: 10;
}
➡️ Controls the stacking order of elements.
18. What is the difference between absolute
, relative
, fixed
, and sticky
positioning?
➡️
absolute
: Positions the element relative to its nearest positioned ancestor.relative
: Positions the element relative to its normal position.fixed
: Positions the element relative to the viewport.sticky
: Toggles between relative and fixed, depending on scroll position.
19. How do you set a background image in CSS?
body {
background-image: url('background.jpg');
}
➡️ Sets a background image for the body.
20. How do you change the text color of an element?
h1 {
color: blue;
}
➡️ Changes the text color of an <h1>
element.
21. How do you set the width of an element?
div {
width: 300px;
}
➡️ Sets the width of a <div>
element.
22. What does the float
property do?
➡️ The float
property allows an element to be taken out of the normal flow and positioned to the left or right.
img {
float: left;
}
23. How do you clear floats in CSS?
.clearfix::after {
content: "";
display: table;
clear: both;
}
➡️ Creates a clearfix to clear floated elements.
24. How do you set a CSS variable?
:root {
--main-color: blue;
}
body {
background-color: var(--main-color);
}
➡️ Defines and uses a CSS variable.
25. How do you create a CSS transition?
button {
transition: background-color 0.5s;
}
button:hover {
background-color: red;
}
➡️ Creates a transition effect on hover.
26. What is the overflow
property?
➡️ The overflow
property controls what happens when content overflows an element's box.
div {
overflow: hidden; /* or scroll, auto */
}
27. How do you apply multiple classes to an element?
<div class="class1 class2"></div>
➡️ Applies multiple classes to a single HTML element.
28. How do you style an element when it is active?
button:active {
background-color: blue;
}
➡️ Styles a button when it is active (clicked).
29. What is the purpose of the visibility
property?
➡️ The visibility
property controls whether an element is visible or hidden without affecting the layout.
.hidden {
visibility: hidden;
}
30. How do you create a responsive image?
img {
max-width: 100%;
height: auto;
}
➡️ Makes images responsive.
31. How do you set the font size using relative units?
body {
font-size: 16px; /* Base size */
}
h1 {
font-size: 2em; /* 2 times the base size */
}
➡️ Uses relative units to set font sizes.
32. How do you create a grid layout in CSS?
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
}
➡️ Creates a simple grid layout.
33. How do you change the cursor on hover?
button {
cursor: pointer;
}
➡️ Changes the cursor to a pointer on hover.
34. What is the purpose of the opacity
property?
➡️ The opacity
property sets the transparency level of an element.
.transparent {
opacity: 0.5;
}
35. How do you set a fixed height for an element?
div {
height: 200px;
}
➡
️ Sets a fixed height for a <div>
element.
36. What is the position
property used for?
➡️ The position
property defines how an element is positioned in the document.
37. How do you change the text alignment in CSS?
p {
text-align: center;
}
➡️ Centers the text within a paragraph.
38. What is the difference between inline
, block
, and inline-block
?
➡️
inline
: Does not start on a new line; only takes up as much width as necessary.block
: Starts on a new line and takes up the full width.inline-block
: Behaves like an inline element but can have width and height.
39. How do you use the :nth-child
pseudo-class?
li:nth-child(2) {
color: red;
}
➡️ Styles the second <li>
element in a list.
40. What is the difference between rem
and em
units?
➡️
em
: Relative to the font size of the element.rem
: Relative to the font size of the root element (<html>
).
41. How do you set a list style type?
ul {
list-style-type: square;
}
➡️ Sets the bullet style for a list.
42. What is the purpose of the text-transform
property?
➡️ The text-transform
property controls the capitalization of text.
h1 {
text-transform: uppercase;
}
43. How do you create a simple CSS animation?
@keyframes example {
from {background-color: red;}
to {background-color: yellow;}
}
div {
animation: example 5s infinite;
}
➡️ Creates a simple animation that changes the background color.
44. How do you use the calc()
function in CSS?
div {
width: calc(100% - 50px);
}
➡️ Performs calculations to set CSS property values.
45. How do you style a table in CSS?
table {
width: 100%;
border-collapse: collapse;
}
th, td {
border: 1px solid black;
padding: 8px;
}
➡️ Styles a table with borders and padding.
46. What is the transform
property?
➡️ The transform
property applies a 2D or 3D transformation to an element.
div {
transform: rotate(45deg);
}
47. How do you set up a fallback font in CSS?
body {
font-family: 'Arial', sans-serif;
}
➡️ Specifies a fallback font in case the first is unavailable.
48. How do you style hyperlinks?
a {
color: blue;
text-decoration: none;
}
a:hover {
text-decoration: underline;
}
➡️ Styles links and adds an underline on hover.
49. What is the background
shorthand property?
➡️ The background
shorthand property sets multiple background properties in one declaration.
body {
background: url('image.jpg') no-repeat center center fixed;
}
50. How do you set the visibility of an element?
.invisible {
visibility: hidden;
}
➡️ Hides an element without removing it from the document flow.
51. What is the box-shadow
property?
➡️ The box-shadow
property adds shadow effects around an element's frame.
div {
box-shadow: 5px 5px 10px gray;
}
52. How do you create a sticky footer?
body {
display: flex;
flex-direction: column;
min-height: 100vh;
}
footer {
margin-top: auto;
}
➡️ Creates a footer that sticks to the bottom of the viewport.
53. How do you apply a CSS reset?
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
➡️ Resets margin and padding for all elements.
54. What is the purpose of the filter
property?
➡️ The filter
property applies graphical effects like blurring or color shifting.
img {
filter: grayscale(100%);
}
55. How do you create rounded corners with CSS?
div {
border-radius: 10px;
}
➡️ Rounds the corners of an element.
56. What does white-space
control?
➡️ The white-space
property controls how white space inside an element is handled.
p {
white-space: nowrap;
}
57. How do you add space between elements?
.element {
margin-bottom: 20px;
}
➡️ Adds space between elements.
58. What are grid-template-areas
?
➡️ grid-template-areas
defines named grid areas for easier layout management.
.grid-container {
display: grid;
grid-template-areas:
"header header"
"sidebar main"
"footer footer";
}
59. How do you create a CSS sprite?
.sprite {
background-image: url('sprite.png');
width: 50px; /* width of one sprite */
height: 50px; /* height of one sprite */
}
➡️ Combines multiple images into a single file for optimization.
60. What is the purpose of the pointer-events
property?
➡️ The pointer-events
property controls under what circumstances (if any) a particular graphic can be the target of mouse events.
.disabled {
pointer-events: none;
}
61. How do you apply styles conditionally using classes?
.active {
background-color: green;
}
➡️ Applies styles when a class is added to an element.
62. What are data-*
attributes?
➡️ data-*
attributes allow you to store custom data on HTML elements.
<div data-info="example"></div>
➡️ Stores additional information within the element.
63. How do you use CSS animations with keyframes?
@keyframes move {
from {transform: translateX(0);}
to {transform: translateX(100px);}
}
div {
animation: move 2s infinite;
}
64. What is the clip-path
property?
➡️ The clip-path
property creates a clipping region that determines what part of an element is visible.
div {
clip-path: circle(50%);
}
65. How do you change the cursor to a help icon?
.help {
cursor: help;
}
➡️ Changes the cursor to a help icon when hovering over the element.
66. What is the difference between visibility: hidden
and display: none
?
➡️
visibility: hidden
: The element is hidden but still takes up space in the layout.display: none
: The element is completely removed from the layout.
67. How do you create a shadow effect for text?
h1 {
text-shadow: 2px 2px 4px gray;
}
➡️ Adds a shadow effect to text.
68. What does the line-height
property do?
➡️ The line-height
property sets the amount of space above and below inline elements.
p {
line-height: 1.5;
}
69. How do you create a tooltip using CSS?
.tooltip {
position: relative;
}
.tooltip:hover::after {
content: "Tooltip text";
position: absolute;
background: black;
color: white;
padding: 5px;
}
70. What is the cursor
property?
➡️ The cursor
property controls the type of cursor that is displayed when pointing over an element.
71. How do you create a CSS breadcrumb?
.breadcrumb {
list-style: none;
display: flex;
}
.breadcrumb li + li:before {
content: " / ";
padding: 0 5px;
}
72. How do you use CSS transitions for color changes?
button {
background-color: blue;
transition: background-color 0.5s;
}
button:hover {
background-color: red;
}
73. What is the outline
property?
➡️ The outline
property is a shorthand for setting the outline style, color, and width.
input:focus {
outline: 2px solid blue;
}
74. How do you create a vertical navigation menu?
.nav {
display: flex;
flex-direction: column;
}
75. What is the box-sizing
property?
➡️ The box-sizing
property controls how the total width and height of an element are calculated.
* {
box-sizing: border-box;
}
76. How do you create a responsive dropdown menu?
.dropdown {
position: relative;
}
.dropdown-content {
display: none;
position: absolute;
background-color: #f9f9f9;
}
.dropdown:hover .dropdown-content {
display: block;
}
77. How do you style an active link in CSS?
a:active {
color: red;
}
➡️ Styles a link when it is actively being clicked.
78. What is a CSS preprocessor?
➡️ A CSS preprocessor is a scripting language that extends CSS and is compiled into regular CSS.
79. How do you create a media query for landscape orientation?
@media (orientation: landscape) {
body {
background-color: lightblue;
}
}
80. What is the purpose of the word-wrap
property?
➡️ The word-wrap
property allows long words to be broken and wrap onto the next line.
p {
word-wrap: break-word;
}
81. How do you set the font weight in CSS?
p {
font-weight: bold;
}
➡️ Sets the font weight for a paragraph.
82. What is the opacity
property used for?
➡️ The opacity
property sets the transparency level of an element.
83. How do you create a CSS carousel?
.carousel {
overflow: hidden;
}
.carousel-inner {
display: flex;
transition: transform 0.5s ease;
}
84. How do you create a multi-column layout in CSS?
.multi-column {
column-count: 3;
column-gap: 20px;
}
85. What is the purpose of the visibility
property?
➡️ The visibility
property determines whether an element is visible or hidden.
86. How do you use the filter
property for grayscale?
img {
filter: grayscale(100%);
}
87. How do you create a CSS sidebar?
.sidebar {
width: 250px;
position: fixed;
}
88. What does the overflow-x
and overflow-y
property do?
➡️ overflow-x
controls horizontal overflow, while overflow-y
controls vertical overflow.
89. How do you create a sticky navigation bar?
.navbar {
position: sticky;
top: 0;
}
90. What are the advantages of using CSS Grid?
➡️ CSS Grid provides a two-dimensional layout system, making it easy to create complex layouts.
91. How do you set custom properties (CSS variables)?
:root {
--main-color: blue;
}
body {
background-color: var(--main-color);
}
92. How do you create a CSS badge?
.badge {
background-color: red;
color: white;
padding: 5px 10px;
border-radius: 5px;
}
93. What is the transition
property used for?
➡️ The transition
property allows property changes in CSS values to occur smoothly over a specified duration.
94. How do you create a CSS notification?
.notification {
background-color: yellow;
padding: 10px;
border: 1px solid orange;
}
95. How do you create a CSS card layout?
.card {
border: 1px solid #ccc;
border-radius: 8px;
padding: 20px;
box-shadow: 2px 2px 10px rgba(0, 0, 0, 0.1);
}
96. How do you style a form in CSS?
form {
display: flex;
flex-direction: column;
}
input {
margin-bottom: 10px;
}
97. How do you use the aspect-ratio
property?
.box {
width: 100%;
aspect-ratio: 16 / 9;
}
98. How do you create a CSS loader?
.loader {
border: 8px solid #f3f3f3; /* Light gray */
border-top: 8px solid #3498db; /* Blue */
border-radius: 50%;
width: 40px;
height: 40px;
animation: spin 1s linear infinite;
}
@keyframes spin {
0% { transform: rotate(0deg); }
100% { transform: rotate(360deg); }
}
99. How do you create a full-screen overlay?
.overlay {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
}
100. What is the purpose of the grid-gap
property?
➡️ The grid-gap
property defines the size of the gaps (gutters) between rows and columns in a grid layout.
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-gap: 10px;
}
What
are the types of CSS?
➡️ Inline, Internal, and External CSS.
Inline CSS:
<p style="color: blue;">This is an inline styled paragraph.</p>
Internal CSS:
<!DOCTYPE html>
<html>
<head>
<style>
p {
color: blue;
}
</style>
</head>
<body>
<p>This is an internal styled paragraph.</p>
</body>
</html>
External CSS:
<!-- Link to external CSS file in HTML -->
<link rel="stylesheet" type="text/css" href="styles.css">
styles.css:
p {
color: blue;
}
- What is the difference between inline, internal, and external CSS?
➡️ Inline CSS is added directly within an HTML element, internal CSS is placed in the <style>
tag in the HTML document, and external CSS is in a separate file linked via the <link>
tag.
- What is the syntax of a CSS rule?
➡️ A CSS rule consists of a selector and a declaration block, like this: selector { property: value; }
.
h1 {
color: red;
font-size: 20px;
}
- What is a CSS selector?
➡️ A CSS selector selects the HTML element(s) to style.
/* This selector targets all <p> elements */
p {
color: green;
}
- What are the different types of selectors in CSS?
➡️ Universal (*), element, class, id, attribute, pseudo-class, and pseudo-element selectors.
/* Universal selector */
* {
margin: 0;
}
/* Class selector */
.class-name {
color: blue;
}
/* ID selector */
#unique-id {
background-color: yellow;
}
- What is the difference between class and id selectors?
➡️ Class selectors (.class) can be used multiple times, while id selectors (#id) must be unique on a page.
<!-- Class example -->
<div class="box">Class Box</div>
<div class="box">Another Class Box</div>
<!-- ID example -->
<div id="unique-box">Unique Box</div>
- What is the box model in CSS?
➡️ The CSS box model includes margins, borders, padding, and the actual content of an element.
.box {
margin: 20px;
border: 5px solid black;
padding: 15px;
width: 200px;
}
- What are the properties of the box model?
➡️ margin, border, padding, and width/height.
.box {
margin: 10px; /* space outside the box */
border: 2px solid black; /* border thickness and color */
padding: 20px; /* space inside the box */
width: 300px; /* content width */
}
- What is the display property in CSS?
➡️ The display property determines how an element is displayed (e.g., block, inline, flex, grid).
.block {
display: block; /* starts on a new line */
}
.inline {
display: inline; /* stays inline with text */
}
- What does display: block mean?
➡️ The element takes up the full width and starts on a new line.
.block {
display: block;
background-color: lightgray;
}
- What does display: inline mean?
➡️ The element does not break the flow of the document and only takes the necessary width.
.inline {
display: inline;
background-color: lightblue;
}
- What does display: inline-block do?
➡️ It allows an element to flow inline but retains block-level properties like padding and width.
.inline-block {
display: inline-block;
padding: 10px;
border: 1px solid black;
}
- What is the float property in CSS?
➡️ The float property is used to wrap text around an element (typically for images).
.img-float {
float: left;
margin: 10px;
}
- What is the clear property?
➡️ The clear property specifies whether an element should be moved below floating elements (left, right, both).
.clearfix {
clear: both; /* element will not wrap around floats */
}
- What is the position property in CSS?
➡️ The position property specifies the type of positioning for an element (static, relative, absolute, fixed, sticky).
.relative {
position: relative;
top: 10px;
left: 20px;
}
.absolute {
position: absolute;
top: 50px;
left: 50px;
}
- What is the difference between relative and absolute positioning?
➡️ Relative positions an element relative to its normal position, while absolute positions it relative to its nearest positioned ancestor.
.relative {
position: relative;
left: 10px; /* shifts from its original position */
}
.absolute {
position: absolute;
top: 20px; /* positions relative to the closest positioned ancestor */
}
- What does position: fixed do?
➡️ The element is positioned relative to the viewport and does not move when the page is scrolled.
.fixed {
position: fixed;
top: 0;
right: 0;
}
- What is z-index?
➡️ The z-index property controls the stack order of elements (which elements appear in front or behind).
.front {
position: relative;
z-index: 2; /* on top */
}
.back {
position: relative;
z-index: 1; /* behind */
}
- What is the flex layout?
➡️ Flexbox is a layout model that allows items within a container to align and distribute space.
.container {
display: flex;
justify-content: space-between;
}
- What are flex properties in CSS?
➡️ flex-direction, flex-wrap, justify-content, align-items, align-content, and flex-grow.
.container {
display: flex;
flex-direction: row; /* or column */
flex-wrap: wrap; /* allows items to wrap */
justify-content: center; /* alignment on main axis */
align-items: stretch; /* alignment on cross axis */
}
- What is the grid layout in CSS?
➡️ CSS Grid is a layout system that allows creating complex grid-based layouts using rows and columns.
.container {
display: grid;
grid-template-columns: repeat(3, 1fr); /* three equal columns */
grid-gap: 10px; /* space between grid items */
}
- What are grid properties in CSS?
➡️ grid-template-rows, grid-template-columns, grid-gap, justify-items, align-items, and grid-area.
.container {
display: grid;
grid-template-rows: 100px 200px; /* two rows */
grid-template-columns: 1fr 2fr; /* two columns with different sizes */
}
- What is a pseudo-class in CSS?
➡️ A pseudo-class selects elements based on their state or interaction (e.g., :hover, :focus, :nth-child).
a:hover {
color: red; /* changes color when hovered */
}
- What is a pseudo-element in CSS?
➡️ A pseudo-element allows you to style specific parts of an element (e.g., ::before, ::after).
p::first-line {
font-weight: bold; /* styles only the first line of the paragraph */
}
- What is the hover pseudo-class?
➡️ The :hover pseudo-class applies styles when the user hovers over an element.
.button:hover {
background-color: yellow; /* changes background color on hover */
}
- What is the nth-child() pseudo-class?
➡️ The nth-child() pseudo-class selects elements based on their order among siblings.
li:nth-child(2) {
color: green; /* styles the second <li> element */
}
- What is the first-child pseudo-class?
➡️ The :first-child pseudo-class selects the first child element of its parent.
ul li:first-child {
font-weight: bold; /* styles the first <li> in <ul> */
}
- What is the last-child pseudo-class?
➡️ The :last-child pseudo-class selects the last child element of its parent.
ul li:last-child {
color: blue; /* styles the last <li> in <ul> */
}
- What is the before pseudo-element?
➡️ The ::before pseudo-element inserts content before the content of an element.
h1::before {
content: "Note: "; /* adds text before the <h1> */
color: red;
}
- What is the after pseudo-element?
➡️ The ::after pseudo-element inserts content after the content of an element.
h1::after {
content: " !"; /* adds an exclamation mark after the <h1> */
}
- What is a media query in CSS?
➡️ Media queries are used to apply styles based on device characteristics (like screen width).
@media (max-width: 600px) {
body {
background-color: lightgray; /* changes background on smaller screens */
}
}
- What is the transition property?
➡️ The transition property allows smooth transitions between CSS property values.
.box {
transition: background-color 0.5s ease; /* smooth transition for background color */
}
.box:hover {
background-color: blue; /* changes color on hover */
}
- What is the animation property?
➡️ The animation property allows defining keyframe animations for elements.
@keyframes example {
from {background-color: red;}
to {background-color: yellow;}
}
.box {
animation: example 5s infinite; /* applies animation */
}
- What is the difference between transition and animation?
➡️ Transitions occur on property changes, while animations can define multiple keyframes and are more complex.
- What is the opacity property in CSS?
➡️ The opacity property sets the transparency level of an element (0 to 1).
.transparent {
opacity: 0.5; /* 50% transparency */
}
- What is the visibility property in CSS?
➡️ The visibility property specifies whether an element is visible or hidden (visible, hidden, collapse).
.hidden {
visibility: hidden; /* the element is hidden but takes up space */
}
- What is the overflow property in CSS?
➡️ The overflow property controls what happens when content overflows an element's box.
.container {
overflow: hidden; /* hides overflow content */
}
- What is the text-align property?
➡️ The text-align property sets the horizontal alignment of text (left, right, center, justify).
.center {
text-align: center; /* centers text */
}
- What is the font-size property?
➡️ The font-size property specifies the size of the font.
.big-text {
font-size: 24px; /* sets font size to 24 pixels */
}
Subscribe to my newsletter
Read articles from Indrajeet directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
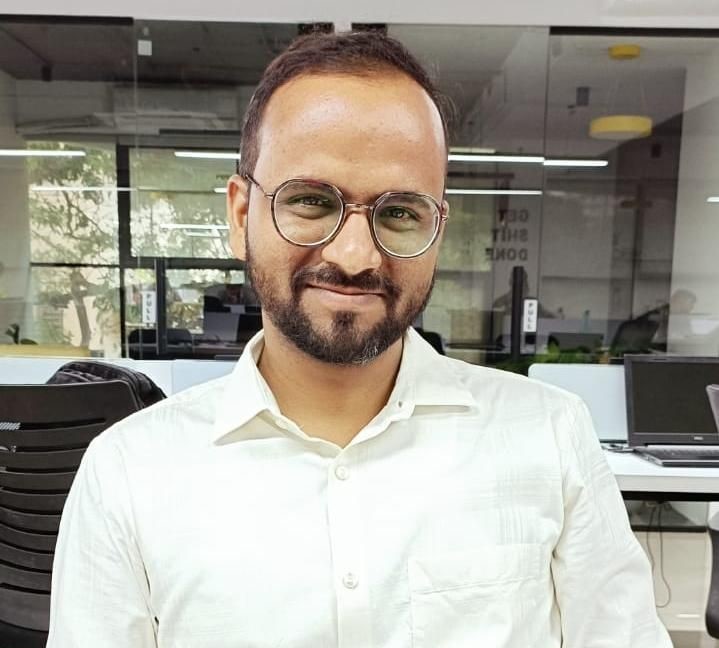
Indrajeet
Indrajeet
Hello there! My name is Indrajeet, and I am a skilled web developer passionate about creating innovative, user-friendly, and dynamic web applications. 📌My Expertise advanced proficiency in angular front-End Development. Back-End Development Leveraging the power of .NET, I build secure, robust, and scalable server-side architectures.