Grammars in Programming Language
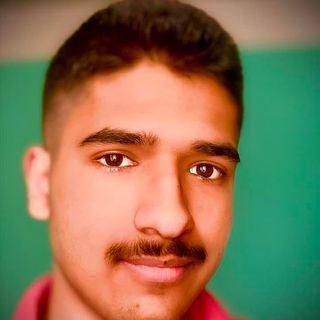
Table of contents
- The Role of Grammars in Programming Languages
- Operators and Their Impact on Syntax
- Operator Precedence and Why It Matters
- Operator Associativity: Left vs. Right
- Resolving Ambiguity in Grammars
- Abstract Syntax Trees (ASTs): Simplifying the Parse Tree
- Conclusion: The Importance of Grammar in Programming Languages
When we write code, we follow rules of syntax—how the code should look. But there's more to it than just getting the syntax right. The way the code is structured directly affects what it means and how it behaves. This intersection of syntax and semantics is fundamental to programming language design.
In this blog, we’ll dive into how grammars define both the form and meaning of programs, how operators influence the behavior of code, and how we resolve ambiguities to ensure correct interpretation by the computer.
The Role of Grammars in Programming Languages
Grammars are the foundation for defining the syntax of a programming language. They specify how to combine symbols (like keywords and operators) to form valid code. But grammars also do more than that—they shape the semantics of the code. By structuring programs in specific ways, grammars ensure that the code behaves as intended.
For example, grammars are used to build parse trees, which visually represent the structure of a program and guide how it should be executed.
Operators and Their Impact on Syntax
Operators (like +
, -
, *
) are central to programming, and the way they are used and structured in the code greatly influences the meaning of expressions.
Types of Operators
Unary operators: Operate on one operand (e.g.,
-5
).Binary operators: Operate on two operands (e.g.,
3 + 4
).Ternary operators: Operate on three operands (e.g.,
a ? b : c
in C).
In most programming languages, binary operators are the most common, and they use infix notation where the operator appears between two operands (e.g., a + b
). But operators can also appear in prefix (e.g., + a b
) or postfix notation (e.g., a b +
).
Operator Precedence and Why It Matters
In an expression with multiple operators, precedence defines the order in which operations are performed. For example, in the expression a + b * c
, should we add a
and b
first, or should we multiply b
and c
first?
Most programming languages follow a set of precedence rules. For instance:
- Multiplication (
*
) generally has higher precedence than addition (+
), soa + b * c
is interpreted asa + (b * c)
.
Fixing Precedence in Grammars
Grammars are used to enforce operator precedence. Here’s a simple grammar for handling addition and multiplication:
<exp> ::= <exp> + <mulexp> | <mulexp>
<mulexp> ::= <mulexp> * <rootexp> | <rootexp>
<rootexp> ::= ( <exp> ) | a | b | c
In this grammar, multiplication has higher precedence than addition, so expressions like a + b * c
are parsed correctly as a + (b * c)
.
Operator Associativity: Left vs. Right
Associativity refers to the direction in which operations of the same precedence are performed. For example, in a + b + c
, do we add a
and b
first, or b
and c
?
Left-associative operators: Operations are grouped from left to right (e.g.,
(a + b) + c
).Right-associative operators: Operations are grouped from right to left (e.g.,
a = (b = c)
).
Most operators in programming languages are left-associative, but some (like assignment =
or exponentiation **
) are right-associative.
Resolving Ambiguity in Grammars
One of the most challenging aspects of grammar design is resolving ambiguities. A classic example is the dangling else problem, where it’s unclear which if
statement an else
belongs to in nested if-then-else
constructs.
Example of Ambiguity: Dangling Else
Consider the following code:
if e1 then if e2 then s1 else s2
There are two possible interpretations:
The
else
belongs to the innerif
.The
else
belongs to the outerif
.
To resolve this, we modify the grammar to force else
to always pair with the nearest unmatched if
. We create a new non-terminal <full-stmt>
that generates if-then
statements with both a then
and an else
:
<stmt> ::= <if-stmt> | s1 | s2
<if-stmt> ::= if <expr> then <full-stmt> else <stmt> | if <expr> then <stmt>
<full-stmt> ::= <full-if> | s1 | s2
<full-if> ::= if <expr> then <full-stmt> else <full-stmt>
This ensures that every else
pairs with the closest if
, eliminating ambiguity in nested if-then-else
statements.
Abstract Syntax Trees (ASTs): Simplifying the Parse Tree
While grammars and parse trees are useful for defining how programs are structured, programming languages often use abstract syntax trees (ASTs) to simplify the representation of code.
An AST focuses on the essential components of the program, removing unnecessary details from the parse tree. For example, in an expression like a + b * c
, the AST might look like this:
+
├── a
└── *
├── b
└── c
This structure is simpler and more efficient for compilers to work with.
Conclusion: The Importance of Grammar in Programming Languages
Grammars are essential for defining the syntax and semantics of programming languages. By controlling operator precedence, associativity, and resolving ambiguities, grammars ensure that programs behave as expected.
Understanding how grammars work helps us write better code and provides insight into the inner workings of compilers. The way a program is parsed can greatly affect its behavior, making grammar design a critical aspect of language development.
Subscribe to my newsletter
Read articles from Dristanta Silwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
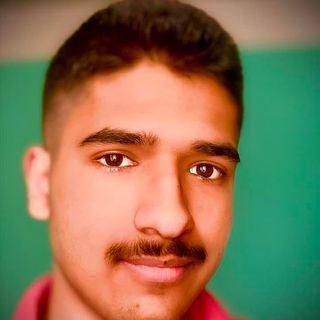
Dristanta Silwal
Dristanta Silwal
Bio: 🖥️ Computer Science Student | ✍️ Passionate Blogger 💡 Exploring the Intersection of Tech and Creativity 🎓 Currently pursuing a degree in Computer Science, I am a curious and driven student with a deep passion for all things technology. I am constantly seeking new avenues to expand my knowledge and skills in this ever-evolving field. 📚 As an aspiring computer professional, I am immersed in the world of programming languages, algorithms, and software development. However, my true excitement lies in blending my technical expertise with my creative spirit. ✏️ I have recently embarked on an exciting journey as a blogger, where I channel my love for writing to explore the fascinating world of technology, digital trends, and innovative ideas. Through my blog, I aim to share valuable insights, tutorials, and thought-provoking content that inspires others to embrace the wonders of the digital age. 🌐 When I'm not busy coding or crafting blog posts, you can find me tinkering with gadgets, experimenting with new software, or exploring the latest tech innovations. I'm always on the lookout for fresh perspectives and innovative ideas to incorporate into my work. 🤝 Let's connect and explore the limitless possibilities of the tech realm together! Feel free to reach out if you have any questions, collaboration opportunities, or just want to geek out over the latest trends. Let's shape the future of technology one blog post at a time! #ComputerScienceStudent #BloggingEnthusiast #TechGeek #CodeAndCreativity