How to throttle your Express API using the express-rate-limit package
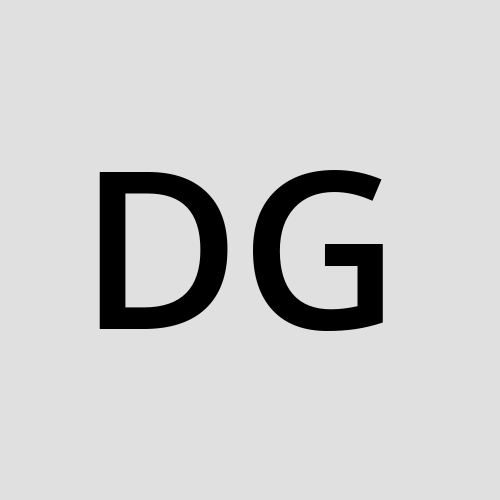
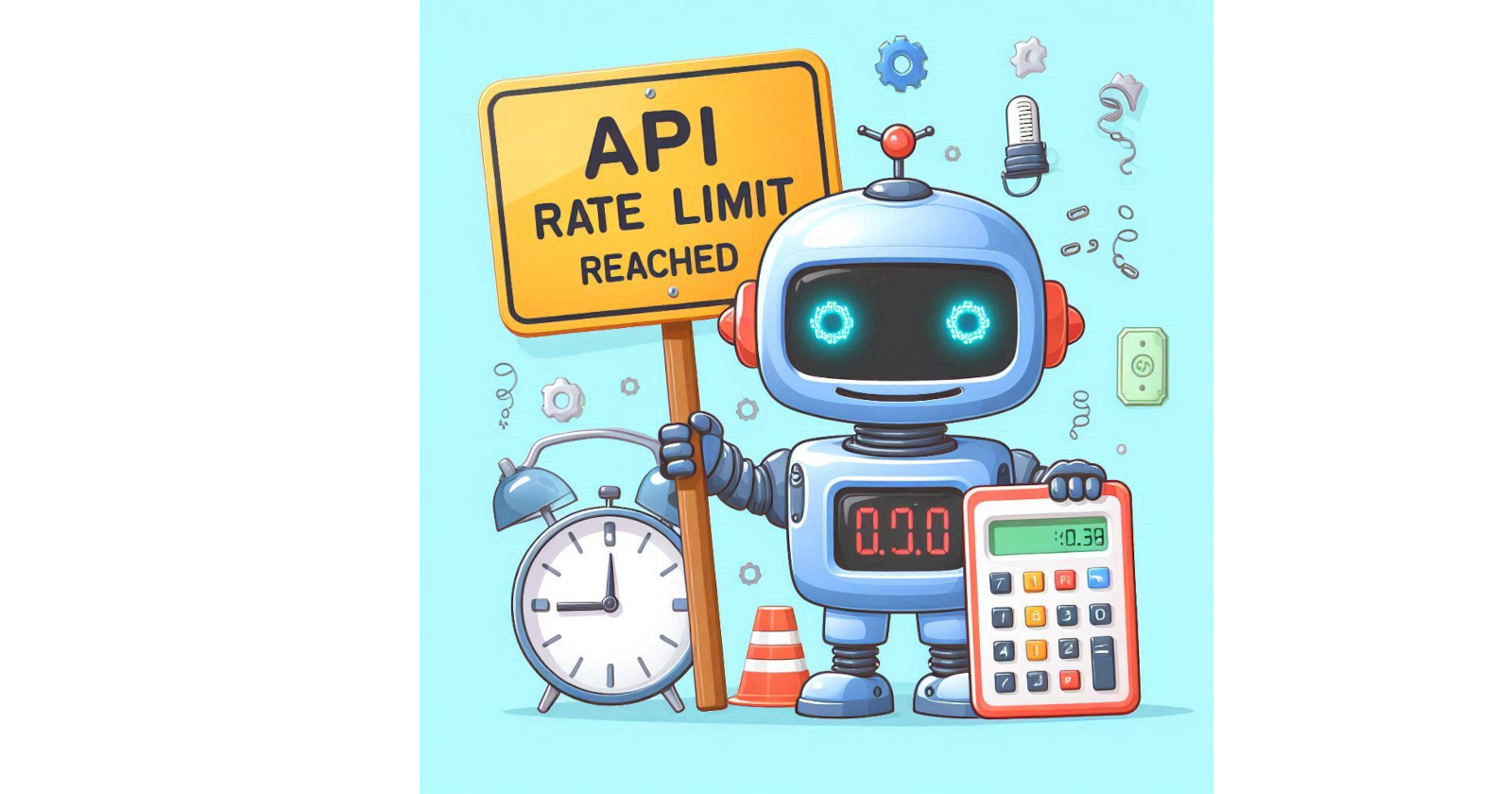
Let’s say we only want to allow 10 requests every 30 seconds to our Express API. To achieve this, you can use middleware to handle rate limiting. A popular choice for this is the express-rate-limit
package.
Steps to Throttle API Requests Using express-rate-limit
:
Install
express-rate-limit
: First, install the package in your Express project.npm install express-rate-limit
Set Up Rate Limiting Middleware: In your Express API, you can configure the
rateLimit
middleware to allow only 10 requests per 30 seconds.Example:
const express = require('express'); const rateLimit = require('express-rate-limit'); const app = express(); // Create rate limiting rule const limiter = rateLimit({ windowMs: 30 * 1000, // 30 seconds max: 10, // Limit each IP to 10 requests per `window` (30 seconds) message: 'Too many requests from this IP, please try again after 30 seconds', }); // Apply the rate limit to all requests app.use(limiter); app.get('/', (req, res) => { res.send('Hello, World!'); }); app.listen(3000, () => { console.log('Server running on port 3000'); });
Key Configuration Options:
windowMs
: This is the time window in milliseconds. For 30 seconds, set this to30 * 1000
.max
: The maximum number of requests allowed within thewindowMs
time frame (in this case, 10 requests).message
: The message sent to the client when the rate limit is exceeded. You can customize this to provide a better user experience.
Apply Rate Limiting to Specific Routes
You can apply the rate limiter to specific routes instead of globally:
// Apply rate limiter only to /api route
app.use('/api', limiter);
3. Test the API
Once set up, your API will respond with the configured message after 10 requests are made within 30 seconds. The client will need to wait for the next time window to make additional requests.
This ensures your server is protected from excessive requests and helps maintain stable performance. Let me know if you need more help with configuration or testing!
Subscribe to my newsletter
Read articles from David Gostin directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
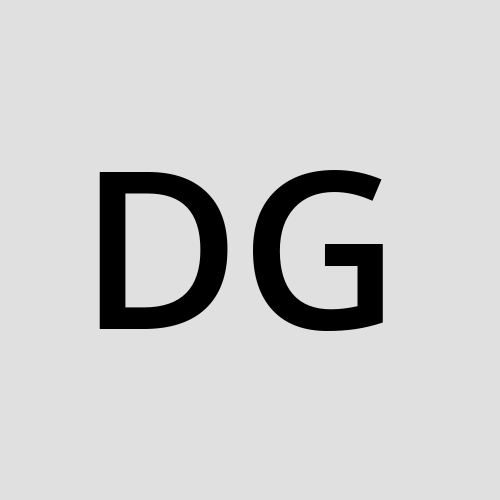
David Gostin
David Gostin
Full-Stack Web Developer with over 25 years of professional experience. I have experience in database development using Oracle, MySQL, and PostgreSQL. I have extensive experience with API and SQL development using PHP and associated frameworks. I am skilled with git/github and CI/CD. I have a good understanding of performance optimization from the server and OS level up to the application and database level. I am skilled with Linux setup, configuration, networking and command line scripting. My frontend experience includes: HTML, CSS, Sass, JavaScript, jQuery, React, Bootstrap and Tailwind CSS. I also have experience with Amazon EC2, RDS and S3.