How to Protect Your Data with RSA Encryption and Decryption: A Cross-Platform Approach

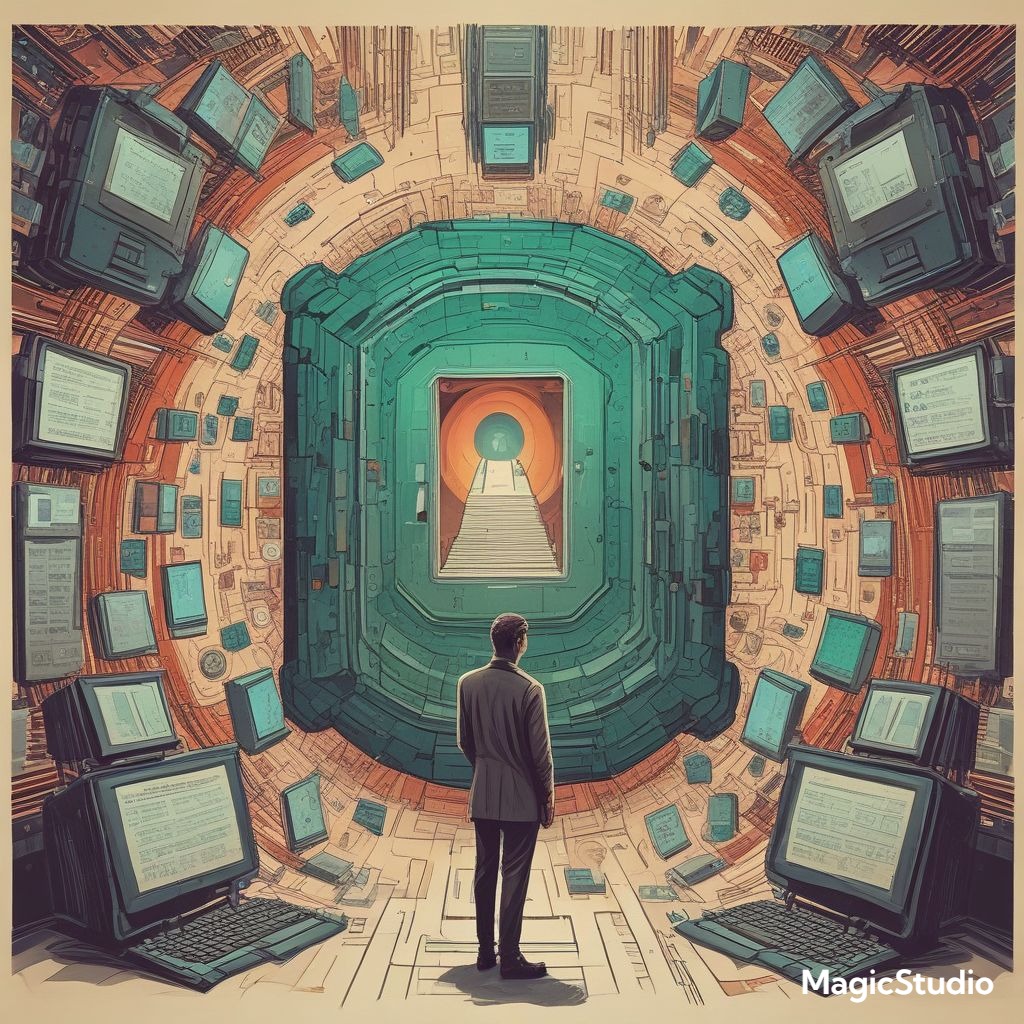
In the digital age, protecting sensitive data is more important than ever. RSA encryption is one of the most trusted ways to ensure data security. This cryptographic method is an asymmetric encryption algorithm that uses two keys — a public key for encryption and a private key for decryption. In this article, we will guide you through implementing RSA encryption and decryption in three popular programming languages: JavaScript, Python, and PHP.
Whether you're a complete beginner or an experienced developer looking to brush up your skills, this article will help you understand how to encrypt data using RSA in JavaScript and then decrypt it using Python or PHP.
What is RSA Encryption?
RSA (Rivest-Shamir-Adleman) is a widely used encryption technique based on the principle of asymmetric cryptography. The algorithm generates two keys:
A public key is used to encrypt data
A private key is used to decrypt it
The beauty of RSA is that you don’t need to share your private key with anyone — you keep it secure, while the public key can be distributed to anyone who needs to send you encrypted data.
Scenario: Encrypt in JavaScript and Decrypt in Python/PHP or vice versa
Imagine a scenario where you want to encrypt a message in JavaScript on the client side and decrypt it on the server side using Python or PHP. This technique is commonly used in web applications for secure communication.
JavaScript (Next.js with JSEncrypt)
Encryption:
import JSEncrypt from 'jsencrypt';
// Encrypt with RSA Public Key
const encryptWithPubKey = (message: string) => {
const encrypt = new JSEncrypt();
// Retrieve the public key from environment variable
const publicKey = process.env.NEXT_PUBLIC_PUBLIC_KEY!?.replace(/\\n/g, "\n");
encrypt.setPublicKey(publicKey);
// Encrypt the message using the public key
const encrypted = encrypt.encrypt(message);
return encrypted;
};
Decryption:
import JSEncrypt from 'jsencrypt';
// Decrypt with RSA Private Key
const decryptWithPrivKey = (encryptedMessage: string) => {
const decrypt = new JSEncrypt();
// Retrieve the private key from environment variable
const privateKey = process.env.PRIVATE_KEY!?.replace(/\\n/g, "\n");
decrypt.setPrivateKey(privateKey);
// Decrypt the message using the private key
const decrypted = decrypt.decrypt(encryptedMessage);
return decrypted;
};
Explanation:
Public Key Encryption: The
JSEncrypt
library is used to encrypt the message with a public key, ensuring only the holder of the corresponding private key can decrypt the message.Private Key Decryption: The same
JSEncrypt
library is used for decryption. The private key is securely stored in an environment variable, and it is used to decrypt the previously encrypted message.Security: This encryption ensures the message remains confidential, as only the private key holder can decrypt it.
Python (using rsa
library)
Encryption:
import rsa
def encrypt_with_pub_key(message: str, public_key_str: str) -> str:
# Convert the string key to an RSA public key object
public_key = rsa.PublicKey.load_pkcs1_openssl_pem(public_key_str.encode())
# Encrypt the message
encrypted_message = rsa.encrypt(message.encode(), public_key)
# Base64 encode the encrypted message
encrypted_base64 = base64.b64encode(encrypted_message).decode()
return encrypted_base64
Decryption:
import rsa
import base64
def decrypt_with_priv_key(encrypted_message: str, private_key_str: str) -> str:
# Convert the string key to an RSA private key object
private_key = rsa.PrivateKey.load_pkcs1(private_key_str.encode())
# Base64 decode the encrypted message
encrypted_bytes = base64.b64decode(encrypted_message.encode())
# Decrypt the message using the private key
decrypted_message = rsa.decrypt(encrypted_bytes, private_key)
return decrypted_message.decode()
Explanation:
Public Key Encryption: In Python, we use the
rsa
library to encrypt a message using a public key. This ensures that only the private key holder can decrypt the message.Private Key Decryption: We decrypt the message using the corresponding private key by first decoding the Base64-encoded encrypted message and then using the
rsa.decrypt()
function.Base64 Encoding: The encrypted message is encoded in Base64 to make it compatible with text-based transmission and storage.
PHP (using OpenSSL)
Encryption:
function encrypt_with_pub_key($message) {
// Retrieve the public key from environment variable
$publicKey = getenv('PUBLIC_KEY');
// Encrypt the message using the public key
openssl_public_encrypt($message, $encrypted, $publicKey);
// Return the Base64-encoded encrypted message
return base64_encode($encrypted);
}
Decryption:
function decrypt_with_priv_key($encryptedMessage) {
// Retrieve the private key from environment variable
$privateKey = getenv('PRIVATE_KEY');
// Base64 decode the encrypted message
$encryptedData = base64_decode($encryptedMessage);
// Decrypt the message using the private key
openssl_private_decrypt($encryptedData, $decrypted, $privateKey);
return $decrypted;
}
Explanation:
Public Key Encryption: In PHP,
openssl_public_encrypt
is used to encrypt the message with the public key. This ensures that only the private key holder can decrypt it.Private Key Decryption: The
openssl_private_decrypt
function decrypts the message using the private key. The message is first Base64-decoded before decryption.Environment Variables: Both the public and private keys are securely stored in environment variables for security purposes.
Best Practices for Encryption
Use Environment Variables: Store your keys securely in environment variables and never hard-code them in your application.
Encrypt Sensitive Data: Always encrypt sensitive user information such as passwords, financial data, or personally identifiable information (PII).
Use HTTPS: Ensure your application communicates over HTTPS to further protect data in transit.
Keep Keys Secure: Regularly rotate your keys and use strong key management practices.
Why Use RSA Encryption?
1. Data Security:
RSA ensures that sensitive information, such as personal data or passwords, can be securely transferred between parties without the risk of being intercepted.
2. Asymmetric Encryption:
With RSA, the public key is used to encrypt the message, but only the private key can decrypt it. This means you don’t need to share your private key with anyone, making it much more secure than symmetric encryption algorithms.
3. Cross-Platform Compatibility:
As demonstrated, RSA works across multiple programming languages, making it a versatile choice for secure communications in applications that use JavaScript on the front end and Python or PHP on the back end.
Conclusion
RSA encryption and decryption provide a solid foundation for secure communication in web applications. By encrypting messages on the client side in JavaScript and decrypting them on the server side using Python or PHP, you can ensure that sensitive data remains protected.
We’ve shown how to implement RSA encryption in JavaScript and how to handle decryption in Python and PHP, making use of environment variables for secure key management. This approach can be applied to various scenarios, such as securing form submissions, protecting sensitive API calls, or even securing authentication data.
Now that you have a clear understanding of how RSA works across these three programming languages, you can confidently integrate it into your projects to enhance security.
If you enjoyed this tutorial, feel free to share it with other developers and stay tuned for more encryption-related tips!
Subscribe to my newsletter
Read articles from Shubham Khan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shubham Khan
Shubham Khan
Full-Stack Software Engineer with over 3 years of experience in building scalable web applications using React, Spring Boot, and RDBMS. Proven ability to enhance system performance, increase efficiency, and build secure authentication systems. Skilled in developing and deploying applications with cloud services like AWS and Docker, while driving team collaboration through Agile methodologies.