Efficient Backtracking Strategies: A Comprehensive Guide
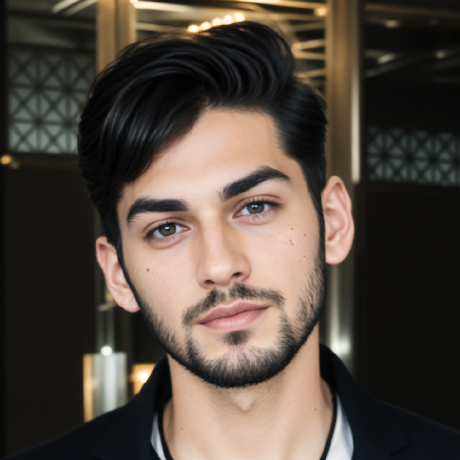
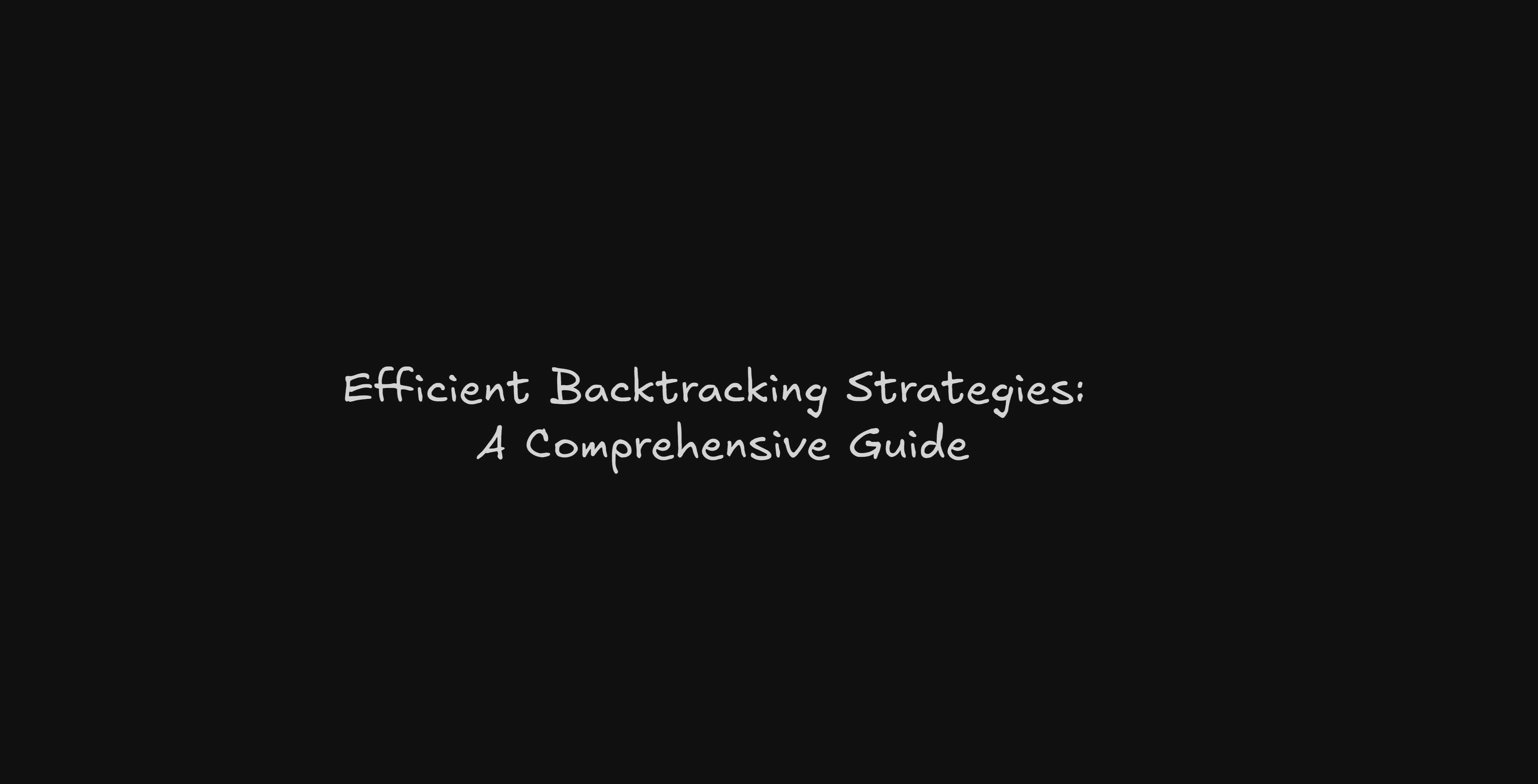
Introduction
Backtracking is a powerful algorithmic technique for solving problems incrementally by trying partial solutions and then abandoning them if they are not viable. It is particularly useful in problems involving permutations, combinations, subsets, and constraint satisfaction, such as the N-Queens problem, Sudoku solving, and the knapsack problem. In this blog, we'll explore efficient backtracking strategies step-by-step, with detailed code snippets to demonstrate each approach. By the end, you'll have a solid understanding of how to implement efficient backtracking algorithms.
Understanding the Basics of Backtracking
Before diving into efficient strategies, it's essential to understand the basic structure of a backtracking algorithm. The core idea is to build solutions piece by piece and remove those that fail to meet the criteria at any stage.
Basic Backtracking Algorithm Structure
def backtrack(solution):
if is_complete(solution):
process_solution(solution)
return
for candidate in get_candidates(solution):
make_move(solution, candidate)
backtrack(solution)
undo_move(solution, candidate)
Step 1: Problem Definition
Let’s consider a classic problem: N-Queens. The goal is to place N queens on an N×N chessboard such that no two queens threaten each other. This means no two queens can share the same row, column, or diagonal.
Step 2: Implementing the Basic Backtracking Algorithm
We will begin with a basic backtracking approach to solve the N-Queens problem.
Code Snippet: Basic Backtracking
def is_safe(board, row, col):
# Check this row on the left side
for i in range(col):
if board[row][i] == 'Q':
return False
# Check upper diagonal on left side
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 'Q':
return False
# Check lower diagonal on left side
for i, j in zip(range(row, len(board)), range(col, -1, -1)):
if board[i][j] == 'Q':
return False
return True
def solve_n_queens_util(board, col):
if col >= len(board):
print_board(board) # Function to print the board
return True
for i in range(len(board)):
if is_safe(board, i, col):
board[i][col] = 'Q' # Place queen
if solve_n_queens_util(board, col + 1):
return True
board[i][col] = '.' # Backtrack
return False
def solve_n_queens(n):
board = [['.' for _ in range(n)] for _ in range(n)]
solve_n_queens_util(board, 0)
solve_n_queens(4) # Testing the function
Step 3: Optimize with Early Pruning
To enhance our backtracking solution, we can use early pruning. This technique involves checking for conflicts before placing a queen, reducing unnecessary recursive calls.
Code Snippet: Early Pruning
def solve_n_queens_util(board, col):
if col >= len(board):
print_board(board) # Print valid solution
return
for i in range(len(board)):
if is_safe(board, i, col):
board[i][col] = 'Q'
# Recur to place rest of the queens
solve_n_queens_util(board, col + 1)
# Backtrack
board[i][col] = '.'
def print_board(board):
for row in board:
print(' '.join(row))
print()
Step 4: Using a Set to Track Columns and Diagonals
Instead of checking each column and diagonal on every call, we can maintain sets to track occupied columns and diagonals, which speeds up our solution significantly.
Code Snippet: Using Sets for Tracking
def solve_n_queens(n):
board = [['.' for _ in range(n)] for _ in range(n)]
cols = set() # Set to track columns
diag1 = set() # Set to track main diagonal
diag2 = set() # Set to track secondary diagonal
def solve(col):
if col >= n:
print_board(board)
return
for row in range(n):
if row in cols or (row - col) in diag1 or (row + col) in diag2:
continue
board[row][col] = 'Q'
cols.add(row)
diag1.add(row - col)
diag2.add(row + col)
solve(col + 1)
board[row][col] = '.'
cols.remove(row)
diag1.remove(row - col)
diag2.remove(row + col)
solve(0)
solve_n_queens(4)
Conclusion
Backtracking is an essential technique in solving complex problems like N-Queens, and by optimizing our approach with early pruning and tracking occupied columns and diagonals, we can significantly enhance performance. These strategies will help you tackle similar problems in coding interviews with more confidence and efficiency.
If you found this blog helpful, don’t forget to share it with your friends and fellow coders! Subscribe to our blog for more insightful articles on algorithmic strategies, coding techniques, and interview tips. Have any questions or topics you'd like us to cover? Leave a comment below! Happy coding!
Subscribe to my newsletter
Read articles from Akshay Parihar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
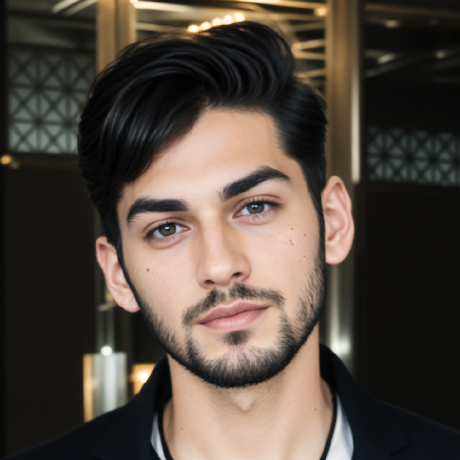