Space Complexity Explained: Unlocking Efficient Algorithm Design

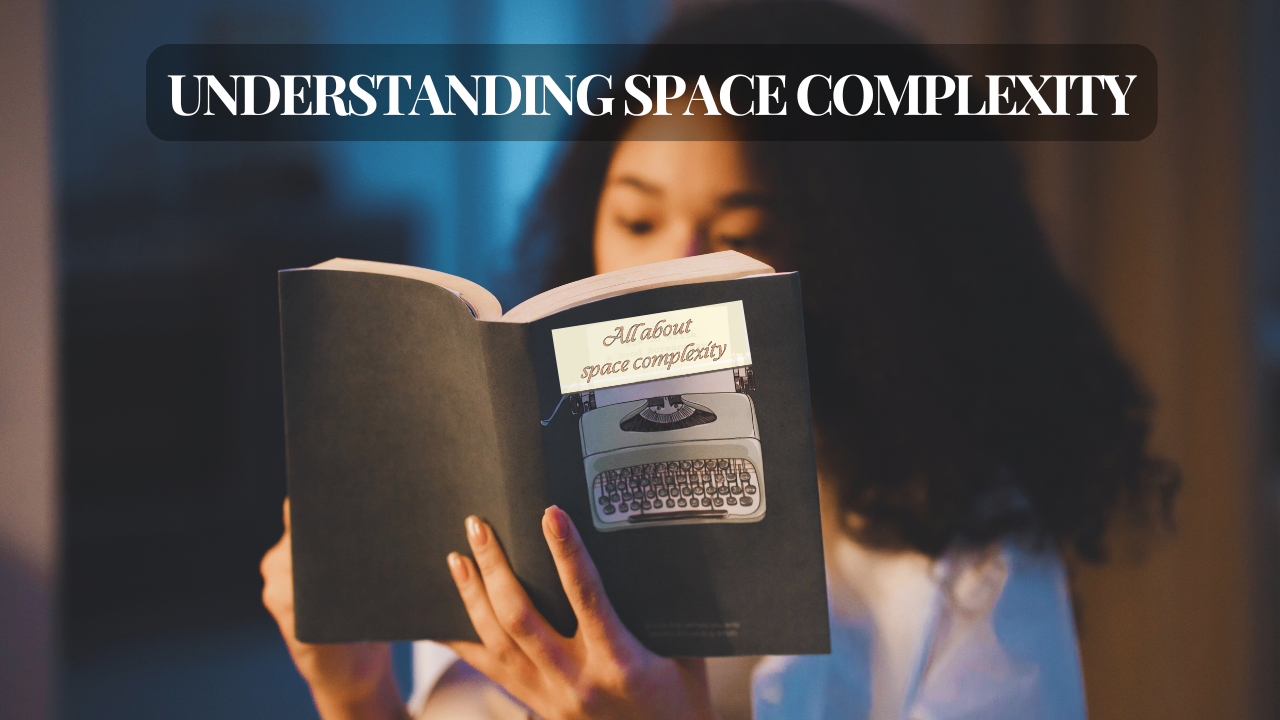
When we think of optimizing algorithms, time complexity often takes the spotlight. But equally crucial is space complexity, which measures how much memory an algorithm requires about the size of the input. In practical applications—especially with large datasets—reducing memory usage is just as vital as speeding up execution time.
What is Space Complexity?
Space complexity refers to the amount of memory an algorithm uses as a function of the input size. It includes both the memory needed for data structures and any auxiliary (extra) memory required for intermediate computations.
Space complexity can be broken down into two parts:
Fixed Space Requirement: Memory used by constants, program code, and simple variables, which remains constant regardless of input size.
Variable Space Requirement: Memory that changes with the input size, such as memory allocated for arrays, lists, or the recursive call stack.
How to Analyze Space Complexity?
To calculate space complexity, we focus on how much additional memory is consumed as the input size (denoted as n) increases. Below is a comparison of different space complexities with examples for clarity.
Complexity | Description | Example | Space Usage |
O(1) | Constant space | Swapping two numbers using a temp variable: a, b = b, a | Memory usage is constant regardless of input size. |
O(n) | Linear space | Storing values in a list: my_list = [i for i in range(n)] | Memory grows proportionally with input size. |
O(n^2) | Quadratic space | Storing values in a 2D matrix: matrix = [[0 for j in range(n)] for i in range(n)] | Memory usage increases quadratically with input. |
O(log n) | Logarithmic space | Binary search algorithm (array halved at each step): binary_search(arr, left, right, target) | Memory grows logarithmically with input size. |
O(2^n) | Exponential space | Recursive Fibonacci (many overlapping subproblems): fibonacci_recursive(n) | Memory doubles with each increase in input size. |
Example 1: O(1) Space Complexity
Consider swapping two variables. The algorithm only uses a fixed amount of memory regardless of how large the numbers are.
def swap(a, b):
temp = a
a = b
b = temp
The space complexity here is O(1) because the space required (the temp
variable) remains constant.
Example 2: O(n) Space Complexity
Storing n
elements in a list will take linear space, as the amount of memory grows with the number of elements:
def store_values(n):
my_list = []
for i in range(n):
my_list.append(i)
This algorithm has O(n) space complexity because the list size increases directly with n
.
Example 3: O(n^2) Space Complexity
Consider a 2D matrix. If the input size is n
, and we create a matrix with n
rows and n
columns, the memory usage increases quadratically.
def create_matrix(n):
matrix = [[0 for j in range(n)] for i in range(n)]
return matrix
The space complexity here is O(n^2) due to the matrix size growing quadratically with n
.
Recursive Algorithms: The Stack Factor
Recursive algorithms, while elegant, often come with higher space complexity because of the memory required to keep track of function calls on the stack.
For example, the recursive Fibonacci algorithm:
def fibonacci_recursive(n):
if n <= 1:
return n
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
This has a space complexity of O(n) due to the stack frames for each recursive call.
Why Space Complexity Matters
In today's world, memory efficiency is a key factor in designing scalable systems. High space complexity algorithms can consume too much memory, leading to performance bottlenecks, crashes, or system slowdowns. This is especially true for embedded systems and mobile devices where memory resources are limited.
Balancing both time complexity and space complexity is crucial when optimizing algorithms. In some cases, trading off one for the other is necessary—for example, using more memory to reduce time or vice versa.
Challenge for You: Apply What You’ve Learned!
Here’s a simple task to test your understanding of space complexity:
Analyze the space complexity of the following function:
def count_zeros(n):
count = 0
while n > 0:
if n % 10 == 0:
count += 1
n = n // 10
return count
Can you figure out the space complexity? What happens to the memory usage as n
increases?
Further Reading
For those interested in diving deeper into algorithm design and analysis, here are some excellent resources:
"Introduction to Algorithms" by Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, and Clifford Stein – A comprehensive guide to both time and space complexities, along with many real-world examples.
"The Algorithm Design Manual" by Steven S. Skiena – Focuses on practical algorithm design with a strong emphasis on optimization techniques.
"Data Structures and Algorithm Analysis in C++" by Mark Allen Weiss – A great resource for learning about data structures and algorithms, including how to analyze space and time complexity.
Conclusion
Understanding space complexity is crucial for building scalable and efficient software solutions. When developing algorithms, always consider not only how long they take to run but also how much memory they require. Striking the right balance between time and space efficiency can make the difference between a performant application and one that struggles with large inputs or memory constraints.
As you continue your journey in mastering DSA, keep space complexity in mind as an essential metric for evaluating algorithm efficiency.
Subscribe to my newsletter
Read articles from Keerthi Ravilla Subramanyam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Keerthi Ravilla Subramanyam
Keerthi Ravilla Subramanyam
Hi, I'm Keerthi Ravilla Subramanyam, a passionate tech enthusiast with a Master's in Computer Science. I love diving deep into topics like Data Structures, Algorithms, and Machine Learning. With a background in cloud engineering and experience working with AWS and Python, I enjoy solving complex problems and sharing what I learn along the way. On this blog, you’ll find articles focused on breaking down DSA concepts, exploring AI, and practical coding tips for aspiring developers. I’m also on a journey to apply my skills in real-world projects like predictive maintenance and data analysis. Follow along for insightful discussions, tutorials, and code snippets to sharpen your technical skills.