Chapter 7 :Datatype Continuation
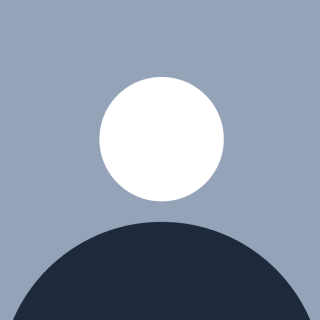
Table of contents
- Java Data Types: A Comprehensive Guide
- Introduction to Data Types
- 1. The float Data Type
- 2. The double Data Type
- Wrapper Classes
- 3. The char Data Type
- Character Data Type (char) in Java
- How char Data Type Works
- Character Encoding Systems: ASCII and Unicode
- Syntax and Rules for char in Java
- Why Java is Not Purely Object-Oriented
- 4. The boolean Data Type
- Conclusion
Java Data Types: A Comprehensive Guide
Introduction to Data Types
Data types are a crucial concept in any programming language, and Java is no exception. The main reason data types exist is to tell the compiler what kind of data to expect and how much memory to allocate for that data. Java, being a statically-typed language, mandates the declaration of data types at compile time to ensure memory efficiency and type safety. Without this declaration, Java wouldn’t know how to store or handle the data.
In the previous class, we discussed some primitive data types like byte
, int
, short
, and long
. Today, we’ll delve deeper into other fundamental data types such as float
, double
, char
, and boolean
, which are essential for real-world applications. Understanding these types will help optimize both memory usage and performance in Java applications.
1. The float
Data Type
The float
data type in Java is a primitive data type used to represent real numbers with decimal points (also called floating-point numbers). It follows the IEEE 754 standard for floating-point arithmetic, which ensures that numbers are represented in binary and stored efficiently.
Size and Memory Usage
Size: 32 bits (4 bytes)
Precision: 6 to 7 decimal digits (single precision)
The memory size allocated for float
is 32 bits, meaning it can store a wide range of real numbers but with limited precision. This limitation leads to potential precision loss when dealing with very small or very large numbers.
Maximum and Minimum Values
Maximum value:
3.4028235E38
Minimum value:
1.4E-45
These values represent the upper and lower bounds of what a float
can store. Any number beyond this range will result in an overflow or underflow, causing the program to behave unexpectedly.
System.out.println("Size of float: " + Float.SIZE); // Outputs: 32
System.out.println("Max value of float: " + Float.MAX_VALUE); // Outputs: 3.4028235E38
System.out.println("Min value of float: " + Float.MIN_VALUE); // Outputs: 1.4E-45
Common Errors
One common issue with the float
data type is that if you directly assign a decimal value like 10.5
to a float
variable, it will throw a compile-time error due to "possible loss of precision." By default, Java treats decimal numbers as double
(which we'll discuss later), so to store a floating-point number in a float
variable, you need to suffix the number with f
or F
.
Example:
// Incorrect assignment, throws a compile-time error
float a = 10.5; // Incompatible types: possible loss of precision
// Correct assignment
float a = 10.5f; // Adding 'f' tells the compiler that this is a float
Note: The reason Java treats decimal numbers as double
by default is due to the precision offered by double
compared to float
. When working with high-precision decimal numbers, double
is a safer choice, but for performance reasons, float
can be used where precision is not critical.
2. The double
Data Type
The double
data type is similar to float
, but it offers double the precision and is the default data type for real numbers. Like float
, double
also adheres to the IEEE 754 standard but in double precision, which means more bits are allocated for the number, thus allowing for higher precision.
Size and Memory Usage
Size: 64 bits (8 bytes)
Precision: Approximately 15 decimal digits (double precision)
The double
data type allocates 64 bits for each number, which allows for storing extremely small or large decimal values with a higher degree of accuracy compared to float
.
Maximum and Minimum Values
Maximum value:
1.7976931348623157E308
Minimum value:
4.9E-324
This gives the double
data type an enormous range, making it suitable for scientific computations where extreme precision is required.
System.out.println("Size of double: " + Double.SIZE); // Outputs: 64
System.out.println("Max value of double: " + Double.MAX_VALUE); // Outputs: 1.7976931348623157E308
System.out.println("Min value of double: " + Double.MIN_VALUE); // Outputs: 4.9E-324
Example:
double d = 23.456; // By default, any decimal number is treated as a double
Note: While double
offers more precision, it also consumes more memory. In memory-constrained environments or performance-critical applications, choosing between float
and double
should be based on both the memory available and the precision required.
Wrapper Classes
In Java, wrapper classes are used to treat primitive data types as objects. This is important because, in some cases, we need to work with objects rather than primitive types, particularly when interacting with Java Collections or in object-oriented programming contexts.
Java introduced wrapper classes in JDK 1.5 to allow developers to treat primitives as objects.
Here’s how the primitive types correspond to their wrapper classes:
Primitive Type | Wrapper Class |
byte | Byte |
short | Short |
int | Integer |
long | Long |
float | Float |
double | Double |
char | Character |
boolean | Boolean |
For example, if you want to convert an int
to an object, you can use the Integer
wrapper class. This also comes in handy when you want to use certain methods that are available for objects but not for primitive types.
3. The char
Data Type
Character Data Type (char
) in Java
The char
data type is a primitive type in Java, designed to store single characters. This data type is essential for storing textual data, as it represents individual characters like letters, digits, or symbols.
Why Do We Need Data Types Like char
?
To store data: Data types allow the computer to know what type of data is being stored and how much memory to allocate.
To optimize data: By using specific data types like
char
, we can efficiently store data without wasting memory.To store real-world data: Characters are a fundamental part of real-world data, such as names, symbols, and text, and they need to be stored using the appropriate data type.
How char
Data Type Works
The char
data type in Java follows a specific binary format behind the scenes. All data, including characters, is stored as a sequence of binary digits (0s and 1s) in memory. For example, integers follow the base 2 format (binary), and characters are no exception—they are stored in memory as binary values.
Binary Representation of Characters
Let's break down how characters are stored in memory using the binary format:
Character:
A
Binary Representation:
00
Character:
B
Binary Representation:
01
This representation can be extended as the number of characters increases:
For 2-bit binary combinations:
A
:00
B
:01
C
:10
D
:11
For 3-bit binary combinations:
A
:000
B
:001
C
:010
D
:011
E
:100
F
:101
G
:110
H
:111
As the number of characters increases, the number of bits used to represent each character also increases.
Character Encoding Systems: ASCII and Unicode
ASCII (American Standard Code for Information Interchange)
The ASCII encoding system was developed by Americans to represent 128 characters, which includes uppercase and lowercase letters, numbers, and symbols. Each character in ASCII is assigned a binary representation, as well as decimal and hexadecimal representations.
ASCII Range:
0
to127
(7-bit encoding, 1 extra bit for memory standardization)Memory: 1 byte = 8 bits
Example:
A
: Binary01000001
, Decimal65
, Hexadecimal41
a
: Binary01100001
, Decimal97
, Hexadecimal61
Unicode
To accommodate characters from all the languages around the world, Unicode was developed. IEEE (Institute of Electrical and Electronics Engineers) recommended finding all the characters from different languages and assigning them unique codes. This led to the creation of Unicode Transformation Format (UTF).
UTF-16 is used by Java, which allocates 2 bytes (16 bits) of memory to each character. This allows Java to store up to 65,536 unique characters or symbols.
UTF-8 is a variable-width character encoding that uses one to four bytes for each character, commonly used on the web.
Java follows the UTF-16 encoding, and each char
takes up 2 bytes of memory.
Syntax and Rules for char
in Java
The char
type allows you to store single characters, and the characters must be enclosed in single quotes ('
).
Examples:
char a = 'A'; // Valid
char b = 'i'; // Valid
Invalid Examples:
char a = "A"; // Invalid - should use single quotes
char a = 'AB'; // Invalid - only one character can be stored in a char
In Java, characters are always represented in single quotes. Using double quotes (" "
) is reserved for strings. Additionally, a char
variable can only hold one character at a time.
Why Java is Not Purely Object-Oriented
Java is considered an impure object-oriented language because it uses primitive data types like char
, int
, float
, etc. These are not objects in their default form. However, Java provides wrapper classes (like Character
, Integer
, Float
) to allow primitive types to be treated as objects when necessary.
When you use a wrapper class, everything is treated as an object, making Java closer to a purely object-oriented language.
In summary, the char
data type in Java is essential for storing characters and is backed by powerful encoding systems like ASCII and Unicode. It ensures efficient storage of textual data while being compatible with Java's object-oriented nature through the Character
wrapper class. This balance of primitive and object types allows Java to handle both performance and flexibility effectively.
4. The boolean
Data Type
The boolean
data type is the simplest of all data types in Java. It is used to store true or false values, and is integral to control flow in programming, such as conditional statements and loops.
Size and Memory Usage
Size: Not precisely defined by the Java Language Specification (JLS). However, it is often implemented using 1 bit, though the actual memory used can vary depending on the JVM and internal optimizations.
Values:
true
orfalse
The boolean
data type does not have a range like other data types, as it can only hold one of two values: true
or false
.
Example:
boolean isJavaFun = true;
boolean isFishTasty = false;
Note: In Java, boolean
does not accept numerical values like 1 or 0, which are sometimes used in other programming languages like C or Python to represent true
or false
. Java strictly requires the use of true
or false
.
Conclusion
In summary, understanding the core data types in Java—float
, double
, char
, and boolean
—is critical for writing efficient and optimized programs. Each data type serves a unique purpose, from handling real numbers with various degrees of precision to storing characters from multiple languages and managing logical conditions. With this knowledge, you can now make informed decisions when choosing the right data type for different scenarios, ensuring both memory efficiency and accuracy in your Java applications.
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
🚀 Tech Enthusiast | Full Stack Developer | System Design Explorer 💻 Passionate About Building Scalable Solutions and Sharing Knowledge Hi, I’m Rohit Gawande! 👋I am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What I’m Currently Doing 🔹 Writing an in-depth System Design Series to help developers master complex design concepts.🔹 Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.🔹 Exploring advanced Java concepts and modern web technologies. What You Can Expect Here ✨ Detailed technical blogs with examples, diagrams, and real-world use cases.✨ Practical guides on Java, System Design, and Full Stack Development.✨ Community-driven discussions to learn and grow together. Let’s Connect! 🌐 GitHub – Explore my projects and contributions.💼 LinkedIn – Connect for opportunities and collaborations.🏆 LeetCode – Check out my problem-solving journey. 💡 "Learning is a journey, not a destination. Let’s grow together!" Feel free to customize or add more based on your preferences! 😊