Python Guide for Rock-Paper-Scissors Game: Logic, Loops, and Random
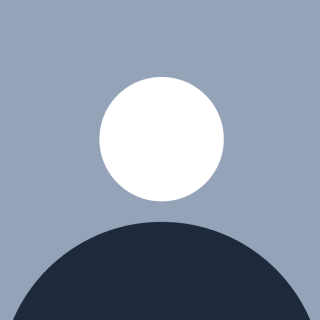
import random
The random module is used to select values randomly from a set. Here, the computer chooses random values (0, 1, 2), where 0 stands for Rock, 1 for Paper, and 2 for Scissors.
The Rule is:
1. Rock crushes Scissors
2. Scissors cuts Paper
3. Paper covers Rock
def play():
while True:
user=int(input("Enter ur choice: 0 for Rock 1 for paper 2 for scissor."))
if user>2 or user<0:
print("You entered invalid number. You lose.")
else:
computer = random.randint(0, 2)
print("Computer choose:")
print(computer)
if user == computer:
print("It's a Draw.")
elif computer == 0 and user == 2:
print("You lose.")
elif user == 0 and computer == 2:
print("You win.")
elif computer > user:
print("You lose.")
elif user > computer:
print("You win.")
again=input("If u want to play again (y/n):").lower()
if again!='y':
print("Thanks for playing..")
break
play()
In the main function contains the logic of the game. Everything inside this function is executed when the game is run. If the user enters a number other than 0, 1, or 2, it prints "Invalid number, you lose." Otherwise, the computer randomly selects a value, which is then displayed. If the user's choice matches the computer's, it results in a tie. The user wins if their choice is higher than the computer's, except when the user's choice is 2 and the computer's is 0. Conversely, the user loses if the computer's choice is higher, except when the user's choice is 0 and the computer's is 2.
After each round, the user is asked if they want to play again. If the user types anything other than ‘y’ , the game ends, and the break statement exits the loop, ending the game.
Subscribe to my newsletter
Read articles from Arunmathavan K directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by